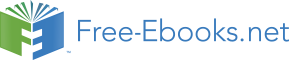

[Result]
Blog added with ID: 1
In the code above:BloggingContext class inherits from DbContext, which is a part of Entity Fr amework used to manage the database operations. This class contains a DbSet<Blog> which represents the collection of all "Blogs" in the context.OnConfiguring method in BloggingCont ext sets up the database connection using a connection string. Here, we connect to a local S
QL Server database.The Blog class represents the data model with properties BlogId and Url which correspond to the columns in the database.In the Main method, a new instance of Blo ggingContext is created and used to add a new Blog object to the database. SaveChanges m ethod commits the transaction to the database and returns the auto-generated ID of the ne wly added blog.The console output displays the ID of the new blog, demonstrating that it ha s been successfully added to the database.
[Trivia]
Entity Framework supports a feature called "migrations" which allows developers to manage changes to the database schema over time. This makes evolving your data model easier and safer as your application grows in complexity.
Introduction to ASP.NET Core
ASP.NET Core is a high-performance, open-source framework for building modern, cloud-ba sed, internet-connected applications.
Here we demonstrate how to set up a basic ASP.NET Core application that responds with "He llo, world!" on visiting the root URL.
[Code]
using Microsoft.AspNetCore.Builder;
using Microsoft.AspNetCore.Hosting;
using Microsoft.AspNetCore.Http;
using Microsoft.Extensions.Hosting;
class Program
{
static void Main(string[] args)
{
Host.CreateDefaultBuilder(args)
.ConfigureWebHostDefaults(webBuilder =>
{
webBuilder.Configure(app =>
{
app.Run(async context =>
{
await context.Response.WriteAsync("Hello, World!");
});
});
})
.Build()
.Run();
}
}
[Result]
Hello, World!
In this simple ASP.NET Core application:We use Host.CreateDefaultBuilder to set up the web host environment with default settings, which includes configuration for logging, dependenc y injection, and more.ConfigureWebHostDefaults configures the default settings for the web host, which in this case is an application that listens on the HTTP protocol.Inside the Configur e method, the app.Run middleware is set up. This middleware is a terminal server middlewar e that handles HTTP requests by sending a response directly.The lambda passed to app.Run r eceives an HttpContext object, which represents the HTTP context of the current request. It u ses this object to send "Hello, World!" as the response text.The Build and Run methods of the host actually start the web server and listen for incoming requests.This example provides a b asic demonstration of handling HTTP requests and sending responses using ASP.NET Core, s erving as a foundational model for more complex applications.
[Trivia]
ASP.NET Core is designed to run on both .NET Core and .NET Framework. This makes it a ver satile choice for developers looking to create applications that can run on Windows, Linux, or macOS.4
Xamarin for Mobile Development
Xamarin is a framework used for developing cross-platform mobile apps using C# and .NET.
This is a simple Xamarin.Forms example to create a basic "Hello World" app.
[Code]
using System;
using Xamarin.Forms;
public class App : Application
{
public App()
{
// The root page of your application
MainPage = new ContentPage
{
Content = new Label
{
Text = "Hello, Xamarin!",
VerticalOptions = LayoutOptions.Center,
HorizontalOptions = LayoutOptions.Center
}
};
}
protected override void OnStart()
{
// Handle when your app starts
}
protected override void OnSleep()
{
// Handle when your app sleeps
}
protected override void OnResume()
{
// Handle when your app resumes
}
}
public static class Program
{
public static void Main(string[] args)
{
// Initialize and run the Xamarin application
Xamarin.Forms.Forms.Init();
Application.Run(new App());
}
}
[Result]
The app displays "Hello, Xamarin!" centered on the screen.
In this code, Application represents the main entry point for a Xamarin.Forms app. MainPage is set to a new ContentPage, which acts as the container for other UI elements. Inside this Co ntentPage, we create a Label with the text "Hello, Xamarin!", which is aligned both vertically a
nd horizontally in the center. The methods OnStart, OnSleep, and OnResume are lifecycle eve nts where you can handle specific actions when the app starts, sleeps, and resumes, respectiv ely. This structure is essential for understanding the lifecycle of a mobile app in Xamarin.
[Trivia]
Xamarin apps compile into native apps that are indistinguishable from apps written in Java (
Android) or Swift (iOS). Xamarin.Forms allows for sharing of UI code across platforms, which significantly reduces development time and effort.
Visual Studio
Visual Studio is an integrated development environment (IDE) from Microsoft, widely used fo r developing software, including C# applications.
Here's an example of creating a simple console application in Visual Studio using C#.
[Code]
using System;
class Program
{
static void Main(string[] args)
{
Console.WriteLine("Hello, Visual Studio!");
}
}
[Result]
The console outputs "Hello, Visual Studio!".
The code snippet above defines a simple C# console application. The Main method is the ent ry point of any C# console application. When the program runs, it writes the string "Hello, Vis ual Studio!" to the console window. This example helps demonstrate the basic structure of a C# program, including using directives, class definitions, and static methods. It also illustrates how simple it is to execute basic output operations in C#. Understanding this fundamental c oncept is crucial for anyone starting with C# in Visual Studio.
[Trivia]
Visual Studio supports a plethora of tools and features like IntelliSense, debugging, and versi on control integration, which enhance productivity and collaboration in software developme nt. It's also highly extensible with a vast marketplace of extensions and add-ons.4
Visual Studio Code for C#
Visual Studio Code (VS Code) is a lightweight but powerful source code editor that supports C# development through extensions like the C# extension by OmniSharp.
To use VS Code for C# development, you need to install the C# extension. Here’s how you ca n do it.
[Code]
// To install the C# extension, open VS Code and follow these steps: 1. Open the Extensions view by clicking on the Extensions icon on the Sidebar or pressing Ctr l+Shift+X.
2. In the Extensions view, search for 'C#'.
3. Find the C# extension by OmniSharp and click 'Install'.
[Result]
The extension is installed and ready for use.
Once the C# extension is installed, VS Code can leverage OmniSharp to provide features like IntelliSense (auto-completion of code), debugging, and more. You can open a folder containi ng your C# project, and VS Code will automatically load the project context. This makes it ea
sy to compile and debug your application within the editor. Moreover, you can enhance your development environment by installing additional extensions such as .NET Core Test Explorer to manage unit tests or NuGet Package Manager for handling NuGet packages.
[Trivia]
VS Code is not limited to C#; it supports a wide range of programming languages through ex tensions, making it an extremely versatile tool for developers. OmniSharp is a key player in m aking C# fully functional in VS Code, as it is a cross-platform .NET tooling that powers the lan guage services behind the scenes.
NuGet
NuGet is the package manager for .NET, which helps in managing libraries and dependencies in projects.
To add a NuGet package to your C# project using the .NET CLI, here is a basic example:
[Code]
// Open your command line tool
// Navigate to your project directory
// To install the Newtonsoft.Json package, run:
dotnet add package Newtonsoft.Json
[Result]
The package Newtonsoft.Json is added to the project.
By running the above command, dotnet add package Newtonsoft.Json, you instruct the .NET
CLI to download the specified package and add it to your project file (typically a .csproj file).
This command automatically resolves and installs all dependencies required by Newtonsoft.J
son as well. This is crucial for maintaining consistent and manageable project dependencies.
The .NET CLI tool streamlines the process of managing these packages, and you can use com mands like dotnet restore to fetch all necessary packages as specified in the project file.
[Trivia]
NuGet package management is integral to modern .NET development. It supports package r estore, which means it can automatically download missing packages during build time, ensu ring that all dependencies are correctly in place. This system significantly simplifies dependen cy management, especially in larger projects where manually managing each library would b e impractical.4
Understanding MSBuild
MSBuild, Microsoft Build Engine, is a platform for building applications.
Below is a simple example to demonstrate how to write an MSBuild script to compile a basic C# project.
[Code]
<Project Sdk="Microsoft.NET.Sdk">
<PropertyGroup>
<OutputType>Exe</OutputType>
<TargetFramework>net5.0</TargetFramework>
</PropertyGroup>
<ItemGroup>
<Compile Include="Program.cs" />
</ItemGroup>
<Target Name="Build">
<Csc Sources="@(Compile)" OutputAssembly="MyApplication.exe" />
</Target>
</Project>
[Result]
The project is compiled, producing an executable named MyApplication.exe.
This MSBuild script contains several key components:<Project>: Defines the project and its S
DK.<PropertyGroup>: Specifies the type of output (Exe) and the target framework (.NET 5.0).
<ItemGroup>: Lists the files to compile.<Target>: Defines what the build does, using Csc (C#
compiler) to compile the code.MSBuild uses an XML-based format to define the steps requir ed to compile the project and produce the output. You can run this script using the msbuild command line tool by saving the script as build.proj and executing msbuild build.proj in your terminal.
[Trivia]
MSBuild scripts can also manage complex dependencies and multi-targeting scenarios which are common in large scale and enterprise-level applications.
Unit Testing in C# with NUnit and xUnit
NUnit and xUnit are popular unit testing frameworks used for testing C# applications.
Here is an example of a simple test class using NUnit to test a C# class.
[Code]
using NUnit.Framework;
namespace MyApplication.Tests
{
[TestFixture]
public class CalculatorTests
{
[Test]
public void TestAdd()
{
int result = Calculator.Add(5, 5);
Assert.AreEqual(10, result);
}
}
public class Calculator
{
public static int Add(int a, int b)
{
}
}
}
[Result]
The test runs successfully, showing that the Add method works as expected, returning 10.
This test example uses several key concepts:using NUnit.Framework;: Imports the NUnit fram ework.[TestFixture]: Indicates that CalculatorTests is a test class.[Test]: Marks TestAdd as a tes t method.Assert.AreEqual(10, result);: Verifies that the method returns the expected value.To run these tests, you would typically use a test runner integrated into your development envir onment or via the command line. The NUnit test framework supports various assertions to va lidate that the software behaves as expected.
[Trivia]
Both NUnit and xUnit offer similar functionalities but differ in syntax and execution methods.
xUnit, for example, does not use [TestFixture] and has slightly different attribute names, whic h promotes slightly cleaner code and better test isolation.4
Design Patterns in C#
Design patterns are reusable solutions to common software design problems that arise durin g the development of software projects.
The following example demonstrates the Singleton pattern, one of the most commonly used design patterns in C#.
[Code]
public class Singleton
{
private static Singleton instance;
private static readonly object lockObject = new object();
// Constructor is 'protected' to prevent instantiation from outside protected Singleton() { }
public static Singleton Instance
{
get
{
lock (lockObject)
{
if (instance == null)
{
instance = new Singleton();
return instance;
}
}
}
}
[Result]
The code does not produce output as it sets up a class structure.
In the Singleton pattern, a class has only one instance throughout the application and provid es a global point of access to it. In the provided code:A private static Singleton instance varia ble holds the instance of the class.The lockObject is used to lock the code block for thread sa fety, preventing multiple threads from creating multiple instances in a multi-threaded enviro nment.The constructor is protected, ensuring that the class cannot be instantiated from outsi de the class.The Instance property checks if an instance already exists. If not, it creates one a nd returns it, ensuring that only one instance is ever created.
[Trivia]
Singleton is particularly useful in scenarios where a consistent state across the application is crucial, such as in configuration settings or accessing resources like database connections.
SOLID Principles in C#
SOLID is an acronym for a set of design principles intended to make software designs more u nderstandable, flexible, and maintainable.
Here is an example demonstrating the Single Responsibility Principle (SRP), the first principle of SOLID.
[Code]
public class User
{
public string Name { get; set; }
public string Email { get; set; }
// This method is responsible for user data validation only public bool IsValid()
{
return Email.Contains("@");
}
}
public class EmailService
{
// This method is responsible for sending emails only
public void SendEmail(string email, string message)
{
Console.WriteLine($"Sending email to {email} with message: {message}");
}
}
[Result]
Sending email to [email] with message: [message]
The Single Responsibility Principle (SRP) states that a class should have only one reason to ch ange, meaning it should have only one job or responsibility:The User class is only responsible for holding user data and validating it.The EmailService class handles the sending of emails. I t does not concern itself with user validation or any other unrelated functionality.
This separation of concerns leads to easier maintenance and fewer reasons for changes.
[Trivia]
Adhering to SRP not only makes the code cleaner but also easier to test since each class has only one responsibility to focus on during testing.4
Dependency Injection in C#
Dependency Injection (DI) is a design pattern used to improve code manageability and scala bility by reducing tight coupling between components.
Here's an example demonstrating how to implement constructor injection in C#.
[Code]
using System;
public interface IMessageService
{
void SendMessage(string message);
}
public class EmailService : IMessageService
{
public void SendMessage(string message)
{
Console.WriteLine("Sending Email: " + message);
}
}
public class NotificationService
{
private IMessageService _messageService;
public NotificationService(IMessageService messageService)
_messageService = messageService;
}
public void Notify(string message)
{
_messageService.SendMessage(message);
}
}
class Program
{
static void Main(string[] args)
{
IMessageService emailService = new EmailService();
NotificationService notificationService = new NotificationService(emailService); notificationService.Notify("Hello World!");
}
}
[Result]
Sending Email: Hello World!
This code example shows how to use dependency injection via constructor injection in C#. Th e NotificationService class does not directly depend on a specific implementation of IMessag eService. Instead, it is provided (injected) with an instance of IMessageService when it is creat ed. This approach improves the flexibility and testability of the code because NotificationServ ice can work with any class that implements IMessageService, which makes it easier to switch out implementations or use mocks during testing.
Dependency Injection is heavily utilized in modern software development and is a core conce pt in many popular frameworks, including ASP.NET Core. It helps in writing decoupled and ea sily testable code.
Concurrency and Multithreading in C#
Concurrency in C# is managed through multithreading, which allows multiple threads to exec ute code simultaneously, improving application efficiency.
Below is an example demonstrating basic thread creation and synchronization in C#.
[Code]
using System;
using System.Threading;
class Program
{
static void Main(string[] args)
{
Thread thread = new Thread(new ThreadStart(Run));
thread.Start();
thread.Join(); // Waits for the thread to finish
Console.WriteLine("Thread has finished execution.");
}
static void Run()
{
for (int i = 0; i < 5; i++)
{
Console.WriteLine("Running in a thread: " + i);
Thread.Sleep(1000); // Simulates some work by sleeping
}
}
}
[Result]
Running in a thread: 0
Running in a thread: 1
Running in a thread: 2
Running in a thread: 3
Running in a thread: 4
Thread has finished execution.
In this example, we use the Thread class to execute the Run method in a separate thread. The Thread.Join() method is used to wait for the thread to complete before the main thread conti nues, ensuring that the console message "Thread has finished execution." is displayed after al l thread operations are done. This example highlights basic thread creation, execution, and sy nchronization, which are foundational for implementing concurrency in applications.
[Trivia]
Understanding threading is critical for developing high-performance applications in C#, espe cially for tasks involving I/O operations or CPU-intensive computations where operations can run in parallel to improve overall application responsiveness and speed.4
Memory Management in C#
C# uses automatic memory management, primarily through a mechanism called garbage coll ection (GC).
The following example demonstrates creating a simple object in C# and allowing the garbag e collector to manage its memory.
[Code]
using System;
public class Program
{
public static void Main()
{
// Create a new object instance
Person person = new Person("John Doe");
// Use the object
Console.WriteLine(person.Name);
// The object will be eligible for garbage collection hereafter
}
}
public class Person
{
public string Name { get; private set; }
{
Name = name;
}
}
[Result]
John Doe
In the example, a Person object is created and used. When the Main method completes, pers on goes out of scope. At this point, it becomes eligible for garbage collection, but it's not im mediately collected. The garbage collector decides the best time to reclaim memory based o n several factors, such as the total amount of memory used and system load. This automatic handling simplifies development but requires understanding to optimize memory usage effe ctively.
[Trivia]
Garbage collection in C# is non-deterministic, meaning you cannot predict exactly when the collector will run. It operates on a generational model, dividing objects into generations to o ptimize memory management efficiency.
Performance Optimization in C#
Optimizing performance in C# involves understanding and applying best practices around ef ficient coding and resource management.
Here's a basic example of using the Stopwatch class to measure the execution time of code, which is crucial for performance tuning.
[Code]
using System;
using System.Diagnostics;
public class Program
{
public static void Main()
{
Stopwatch stopwatch = new Stopwatch();
// Start measuring time
stopwatch.Start();
// Simulate some work
for (int i = 0; i < 1000000; i++)
{
int square = i * i;
}
// Stop measuring time
// Output the elapsed time in milliseconds
Console.WriteLine($"Execution time: {stopwatch.ElapsedMilliseconds} ms");
}
}
[Result]
Execution time: [time in milliseconds]
The Stopwatch class provides a precise way to measure the time it takes for a block of code t o execute, which is essential for identifying performance bottlenecks. In the example, the ela psed time to execute a simple loop that calculates squares of numbers is measured. By using performance measurement tools like Stopwatch, developers can experiment with different ap proaches (e.g., changing loop structures or data types) and directly observe the impact on pe rformance, enabling them to make informed optimizations.
[Trivia]
Performance tuning in C# often involves not just code optimizations but also choices about data structures, usage of parallel processing, and memory access patterns, all crucial for achi eving the best performance.4
Security Practices in C#
Implementing security best practices is essential for creating robust and secure C# applicatio ns, especially when dealing with sensitive data.
Here is a simple example demonstrating how to hash a password using the SHA-256 algorith m in C#. This method is commonly used to securely store user passwords.
[Code]
using System;
using System.Security.Cryptography;
using System.Text;
public class SecurityDemo
{
public static string HashPassword(string password)
{
using (SHA256 sha256 = SHA256.Create())
{
byte[] hashedBytes = sha256.ComputeHash(Encoding.UTF8.GetBytes(password)); return BitConverter.ToString(hashedBytes).Replace("-", "").ToLowerInvariant();
}
}
static void Main()
{
string password = "examplePassword"; string hashedPassword = HashPassword(password);
Console.WriteLine(hashedPassword);
}
}
[Result]
The output will display the SHA-256 hash of the string "examplePassword". For instance, if th e provided password is "examplePassword", a possible output could be "ef797c8118f02b166
81235e739fe3a2d43dd0d39a43b668965206f7f6d437e68".
This code example uses the SHA256 class from the System.Security.Cryptography namespace to create a hash of a password. It converts the password string to a byte array using Encodin g.UTF8.GetBytes method, which then is hashed by the ComputeHash method of SHA256. The resulting byte array is converted back into a string in hexadecimal format using BitConverter.
ToString, and hyphens are removed for cleaner storage. This hash function is one-way, meani ng that the original password cannot be easily retrieved from the hash, making it suitable for storing passwords securely.
[Trivia]
SHA-256 is part of the SHA-2 family of cryptographic hash functions designed by the Nation al Security Agency (NSA). It is widely used in various security applications and protocols, inclu ding TLS and SSL, PGP, SSH, IPsec, and more. It's crucial to use a secure method like hashing for passwords instead of storing them in plain text to protect against data breaches.
Cross-Platform Development with .NET Core
.NET Core is a cross-platform framework that allows developers to create applications that ru n on Windows, macOS, and Linux.
The following example shows how to create a simple "Hello World" application using .NET C
ore, which demonstrates the framework's cross-platform capabilities.
[Code]
# Create a new console application
dotnet new console -n HelloWorld
# Navigate into the project directory
cd HelloWorld
# Open the Program.cs file and modify it to:
echo 'using System;
namespace HelloWorld
{
class Program
{
static void Main(string[] args)
{
Console.WriteLine("Hello World from .NET Core!");
}
}
# Run the application
dotnet run
[Result]
The output will be "Hello World from .NET Core!" when you execute the dotnet run comman d.
This set of commands first creates a new .NET Core console application using dotnet new co nsole. The -n HelloWorld specifies the name of the project. After creating the project, you na vigate into the project directory and overwrite the Program.cs file with a new version that pri nts "Hello World from .NET Core!" to the console. Finally, running dotnet run builds and runs the application, demonstrating how .NET Core applications can be developed and run on any supported operating system with the same set of commands.
[Trivia]
.NET Core was released by Microsoft as part of its efforts to make .NET a more open-source a nd cross-platform framework. It supports a wide range of development needs from web to m obile to desktop applications. Being cross-platform, it greatly simplifies the development pro cess by allowing a single code base to be deployed on different operating systems, which re duces both development time and cost.4
Code Analysis Tools in C#
Code analysis tools help improve the quality of software by detecting code issues before dep loyment.
Let's look at an example using the .NET built-in code analysis tool, Roslyn analyzers, to check for code quality and potential improvements.
[Code]
// Ensure to include the following directive to use Roslyn analyzers using System;
class Program
{
static void Main()
{
Console.WriteLine("Hello, World!");
}
}
To use Roslyn analyzers, you typically set them up in the .csproj file or enable them in the Vis ual Studio IDE under the project's properties.
[Result]
The result is the activation and output from Roslyn analyzers indicating any warnings or sugg estions based on the code provided.
Roslyn analyzers are integrated directly into the C# compiler, allowing for a seamless analysis experience as you write code. By providing live feedback on code quality and potential optim izations, they help prevent common coding errors and enforce best practices. The analyzers c an be customized to suit specific team or project requirements, and they support a wide rang e of code quality rules from style to design to performance issues.
[Trivia]
Roslyn, also known as the .NET Compiler Platform, not only provides tooling for code analysi s but also powers many of the features in Visual Studio, such as IntelliSense, refactoring, and code navigation. It allows developers to write their own custom analyzers.
Application Lifecycle Management with C#
Application Lifecycle Management (ALM) encompasses the entire process of software develo pment from planning to deployment.
Here's how to use Microsoft Team Foundation Server (TFS), now known as Azure DevOps, to manage the lifecycle of a C# application.
[Code]
# Example command to create a new project in Azure DevOps az devops project create --name "MyCSharpProject" --description "C# Application Lifecycle Management"
This command initializes a new project in Azure DevOps, setting up the environment for trac king and managing the software development lifecycle.
[Result]
The command will create a new project in Azure DevOps and provide details like project ID a nd URL.
ALM with Azure DevOps provides a comprehensive suite of tools covering project managem ent, source control, testing, and release management. By integrating directly with the develo
pment environment, it enables continuous integration and deployment pipelines, enhances c ollaboration across teams, and improves overall productivity. Azure DevOps supports both Gi t and TFVC as version control systems, catering to different team preferences and project req uirements.
[Trivia]
Azure DevOps evolved from Microsoft Team Foundation Server (TFS) and offers both cloud-based and on-premise deployment options. It's designed to support a broad range of progra mming languages and frameworks, making it an ideal choice for managing complex software projects that may use more than just C#.4
Source Control Integration in C#
Source control, also known as version control, is crucial for managing changes to your codeb ase, particularly when collaborating on software projects.
The following C# example demonstrates how to integrate Git source control into a C# projec t using the Git CLI. This is a basic operation to clone a repository.
[Code]
// This is a shell command, not C# code:
// Clone a Git repository to your local machine
git clone https://github.com/example/repository.git
[Result]
The command will download the repository contents to your local machine.
Source control systems like Git help developers manage changes to source code. Using Git, d evelopers can track revisions, revert to previous versions of the code, and handle merging ch anges from multiple sources. When you execute the git clone command, Git initializes a new directory, clones the repository into it, and sets up remote tracking branches. It automatically
checks out an initial branch, usually the main branch. This setup is essential for collaborating in team environments where code changes frequently.
[Trivia]
Understanding how to use branches and merge changes in Git is foundational in modern sof tware development. Tools like GitHub, GitLab, and Bitbucket enhance these capabilities with graphical interfaces and collaboration tools.
Continuous Integration/Continuous Deployment (C
I/CD) with C#
CI/CD is a method to frequently deliver apps to customers by introducing automation into th e stages of app development.
Below is an example of how you might set up a simple CI/CD pipeline using GitHub Actions f or a C# project. This configuration file automates building and testing the project.
[Code]
name: .NET CI
on:
push:
branches: [ main ]
pull_request:
branches: [ main ]
jobs:
build:
runs-on: ubuntu-latest
steps:
- uses: actions/checkout@v2
- name: Setup .NET
uses: actions/setup-dotnet@v1
with:
- name: Build
run: dotnet build --configuration Release
- name: Test
run: dotnet test
[Result]
The pipeline triggers on push or pull request events to the main branch, builds the applicatio n, and runs tests.
GitHub Actions provides automation directly in your GitHub repository. In the example, when you push to the main branch or create a pull request against it, the CI/CD pipeline automatic ally starts. The steps involve:Checking out the code.Setting up the .NET environment.Building the project in Release configuration to ensure it compiles without errors.Running tests to veri fy that the recent changes have not broken existing functionality. This setup helps catch error s early, leading to more reliable software releases.
[Trivia]
Advanced CI/CD setups can include additional stages like security checks, performance testin g, and automated deployment to various environments (development, staging, production).4
Database Connectivity in C#
Learn how to connect to a database using C# to perform CRUD operations.
The following C# code example demonstrates how to connect to a SQL Server database usin g ADO.NET.
[Code]
using System;
using System.Data.SqlClient;
class Program
{
static void Main()
{
string connectionString = "Server=myServerAddress;Database=myDataBase;User Id=my Username;Password=myPassword;";
using (SqlConnection connection = new SqlConnection(connectionString))
{
connection.Open();
Console.WriteLine("Connected successfully.");
// Execute operations like select, insert here
connection.Close();
}
}
[Result]
Connected successfully.
The code sample provided illustrates the basics of database connectivity in C#. Here's a brea kdown:Using Directives: System.Data.SqlClient is essential for database operations in .NET usi ng SQL Server.Connection String: This string contains vital information to connect to your dat abase, like server address, database name, user ID, and password.SqlConnection: This class is from ADO.NET framework for handling connections to a SQL Server.Using Statement: Ensure s that the connection is properly closed and disposed off, even if an exception occurs, which i s critical for not leaving connections open unintentionally.Connection.Open(): This method es tablishes the actual connection to the database. If it fails, it throws an exception.Connection.
Close(): Closes the connection to the database, freeing up resources.This example is straightf orward but foundational for any database operations you might perform in a C# application.
[Trivia]
ADO.NET is a part of the .NET Framework, providing a bridge between the front-end controls and the back-end database. The framework supports a variety of database operations, includ ing connection management, command execution, data retrieval, and transaction manageme nt.
API Development in C#
Understand the basics of creating web APIs using C# and ASP.NET Core.
This example demonstrates how to create a simple web API in C# that returns a list of items when accessed via HTTP GET.
[Code]
using Microsoft.AspNetCore.Mvc;
using System.Collections.Generic;
namespace WebApiExample
{
[ApiController]
[Route("[controller]")]
public class ItemsController : ControllerBase
{
[HttpGet]
public IEnumerable<string> Get()
{
return new List<string> { "Item1", "Item2", "Item3" };
}
}
}
[Result]
When accessed, the API returns: ["Item1", "Item2", "Item3"]
This sample provides a quick look at setting up a basic web API using ASP.NET Core:Namesp ace Imports: Microsoft.AspNetCore.Mvc is crucial for all functionalities related to ASP.NET Co re MVC, which includes API controllers.ApiController Attribute: This attribute indicates that th e class is used as a controller in an API. This allows for automatic HTTP error status responses
, model validation, etc.Route Attribute: Defines the route at which this API is accessible. "[con troller]" automatically uses the controller's name for the route.HttpGet Attribute: Specifies th at this particular action responds to HTTP GET requests.Return Type: The action returns an IE
numerable of strings, just a simple list in this case.This example sets the foundation for buildi ng APIs that can handle various HTTP methods and return types, crucial for modern web dev elopment.
[Trivia]
ASP.NET Core is a redesign of ASP.NET 4.x, aimed at creating more modular, scalable applica tions that integrate seamlessly with various operating systems. It’s highly favored for building robust, high-performance web APIs.4
Cloud Services Integration in C#
Understanding how to integrate cloud services in C# applications.
Here, we'll explore a simple example of integrating Azure Storage services using C# to uploa d a file to an Azure Blob container.
[Code]
using Azure.Storage.Blobs;
using System;
using System.IO;
using System.Threading.Tasks;
public class AzureStorageExample
{
public static async Task UploadFileToBlobAsync(string connectionString, string containerN
ame, string filePath)
{
// Create a BlobServiceClient
var blobServiceClient = new BlobServiceClient(connectionString);
// Get a reference to a container
var blobContainer = blobServiceClient.GetBlobContainerClient(containerName);
// Create the container if it does not exist
await blobContainer.CreateIfNotExistsAsync();
// Get a reference to a blob
var blobClient = blobContainer.GetBlobClient(Path.GetFileName(filePath));
// Upload file to the blob
await blobClient.UploadAsync(filePath, true);
}
}
[Result]
File successfully uploaded to Azure Blob Storage.
This code snippet demonstrates the essential steps to integrate Azure Blob Storage in a C# a pplication:Using Azure SDK: The Azure.Storage.Blobs namespace is used to interact with the Azure Blob Storage.Creating BlobServiceClient: This client is the starting point for working wit h a storage account.Container and Blob Management: The example shows how to get a cont ainer, create it if it doesn't exist, and how to manage blobs within.Async Methods: Notice the use of asynchronous methods like UploadAsync, which helps in non-blocking UI and efficient resource usage.Exception Handling: In a real-world scenario, include try-catch blocks to hand le potential errors during network operations.
[Trivia]
Azure Blob Storage is a service for storing large amounts of unstructured data, such as text o r binary data, which is highly scalable and available. It's a part of Microsoft Azure's cloud serv ices, and integrating it into C# applications involves using the Azure SDK which provides a se t of libraries pre-configured to interact with Azure resources.
Windows Presentation Foundation (WPF)
Creating user interfaces in C# using Windows Presentation Foundation (WPF).
Below is a simple example of a WPF application in C# that creates a basic window with a butt on.
[Code]
using System.Windows;
using System.Windows.Controls;
public partial class MainWindow : Window
{
public MainWindow()
{
InitializeComponent();
// Create a Button
Button clickButton = new Button
{
Content = "Click Me!",
Width = 100,
Height = 50,
HorizontalAlignment = HorizontalAlignment.Center,
VerticalAlignment = VerticalAlignment.Center
};
clickButton.Click += ClickButton_Click;
// Add Button to Window
this.Content = clickButton;
}
private void ClickButton_Click(object sender, RoutedEventArgs e)
{
MessageBox.Show("Button clicked!");
}
}
[Result]
A window with a central "Click Me!" button appears; clicking the button shows a message bo x saying "Button clicked!".
This code introduces the basics of a WPF application:XAML and Code-behind: While the exa mple here is purely in C#, normally WPF uses XAML for UI design and C# for the backend log ic.Event Handling: It demonstrates how to handle events like button clicks.UI Elements: Eleme nts like Button and properties like HorizontalAlignment and VerticalAlignment control the pl acement and appearance.Message Box: Using MessageBox.Show to display messages to the user.Initialization and Component Loading: The InitializeComponent method in the construct or is crucial for loading components defined in XAML (not shown here but typically used in WPF).
[Trivia]
WPF is part of the .NET framework and uses DirectX for rendering, making it powerful for co mplex UIs. It supports advanced UI features such as animations, templating, and binding, ma
king it suitable for desktop applications that require a high degree of customization and inte ractivity.4
Windows Forms in C#
Windows Forms is a GUI (Graphical User Interface) class library included in the .NET Framewo rk, used for creating desktop applications on the Windows platform.
The following example demonstrates creating a simple Windows Forms application with a bu tton that, when clicked, shows a message.
[Code]
using System;
using System.Windows.Forms;
public class HelloWorldForm : Form
{
private Button button1;
public HelloWorldForm()
{
button1 = new Button();
button1.Size = new System.Drawing.Size(100, 50);
button1.Location = new System.Drawing.Point(100, 100); button1.Text = "Click me!";
button1.Click += Button1_Click;
this.Controls.Add(button1);
}
private void Button1_Click(object sender, EventArgs e)
MessageBox.Show("Hello, World!");
}
[STAThread]
static void Main()
{
Application.EnableVisualStyles();
Application.SetCompatibleTextRenderingDefault(false);
Application.Run(new HelloWorldForm());
}
}
[Result]
When the button is clicked, a message box displaying "Hello, World!" appears.
This code initializes a new form containing a single button. The Button1_Click method is an e vent handler that responds to the button's click event by displaying a message box. Key poin ts for beginners:using System.Windows.Forms;: This line includes the Windows Forms library needed to create forms and controls.public class HelloWorldForm : Form: This defines a class that inherits from the Form class, allowing us to create windows.button1 = new Button();: Thi s creates a new button object.Event handling with button1.Click += Button1_Click;: This subs cribes to the button's click event.Application.Run(new HelloWorldForm());: This line starts the form's message loop, necessary for processing events like clicks.
[Trivia]
Windows Forms uses a coordinate system for positioning controls. The Location property spe cifies the coordinates of the upper-left corner of the control relative to the upper-left corner of its container.
Blazor for Web Development
Blazor is a framework for building interactive web UIs using C# instead of JavaScript. It allows developers to write client-side logic in C# that runs on WebAssembly.
Here is a simple Blazor server-side application example, showing a button that updates a cou nter when clicked.
[Code]
@page "/"
@using Microsoft.AspNetCore.Components
<h1>Counter</h1>
<p>Current count: @count</p>
<button @onclick="IncrementCount">Click me</button>
@code {
private int count = 0;
private void IncrementCount()
{
count++;
}
}
[Result]
Each click on the button increments the counter and updates the display of the current count
.
This Blazor page includes:Razor syntax with @page "/" indicating the component's routing p ath.@code block where C# code is written, separate from the HTML, allowing clean separatio n of markup and logic.IncrementCount method to handle the button click, demonstrating ho w Blazor facilitates using C# for client-side interactions, avoiding JavaScript.The @onclick dir ective binds the button's click event to the IncrementCount method.
[Trivia]
Blazor can run on the server (Blazor Server) or on the client (Blazor WebAssembly). In Blazor Server, the code runs on the server and interacts with the client-side UI via SignalR connectio ns, while Blazor WebAssembly runs the code directly in the browser using WebAssembly.4
Universal Windows Platform (UWP) Basics
UWP is a platform for building universal apps that run on Windows 10 devices using a comm on API set.
The following example demonstrates creating a simple UWP app displaying "Hello World" us ing XAML and C#.
[Code]
using Windows.UI.Xaml.Controls;
using Windows.UI.Xaml;
public sealed partial class MainPage : Page
{
public MainPage()
{
this.InitializeComponent();
TextBlock txtBlock = new TextBlock
{
Text = "Hello World",
VerticalAlignment = VerticalAlignment.Center,
HorizontalAlignment = HorizontalAlignment.Center,
FontSize = 24
};
this.Content = txtBlock;
}
[Result]
A window displaying the text "Hello World" centered on the screen.
This code snippet is a simple example of a UWP application written in C#. It involves creating a MainPage class that inherits from Page. The MainPage constructor initializes the page com ponents and sets up a TextBlock to display "Hello World". The TextBlock is configured for cen ter alignment both vertically and horizontally, and the font size is set to 24 points. The TextBl ock is then assigned to the Content property of the page, which makes it the visual element displayed by the page. UWP apps use XAML for layout and C# for behaviors, enabling a pow erful way to build interactive applications for Windows devices.
[Trivia]
UWP apps can run across all Windows 10 device families, using a single app package. This in cludes PCs, tablets, phones, and more, allowing developers to reach a wide audience with re duced effort on adapting the app for different device types.
Exploring C# Interactive (CSI)
C# Interactive, or CSI, is a powerful tool for running C# code snippets interactively in a comm and-line interface.
Below is a basic example of using C# Interactive to perform a simple calculation.
[Code]
> csi
Microsoft (R) Roslyn C# Compiler version 4.0.0.0
> 1 + 2
[Result]
3
The example provided uses the C# Interactive shell, which is part of the .NET toolset, specific ally leveraging the Roslyn compiler. When you type csi in a command prompt, it launches the C# Interactive shell. You can then directly input C# code like 1 + 2 and get the result immedi ately. C# Interactive is useful for testing small code snippets, learning C#, or doing complex c alculations on the fly without the need to compile a full project. It's an ideal tool for quick pr ototyping and iterative development processes.
[Trivia]
C# Interactive supports most of the features of the C# language, including using namespaces
, creating variables, and defining methods. It can be particularly helpful for developers transit ioning from other languages, as it provides a low-barrier way to experiment with C# syntax a nd features.4
REPL in Visual Studio
REPL stands for Read-Eval-Print Loop, a simple, interactive programming environment.
The following C# example demonstrates using the C# Interactive Window in Visual Studio, a REPL tool.
[Code]
// Open Visual Studio
// Go to View -> Other Windows -> C# Interactive
// Type the following line and press Enter
Console.WriteLine("Hello, World!");
[Result]
Hello, World!
The C# Interactive Window in Visual Studio provides a powerful environment for quickly testi ng snippets of C# code. Here's a step-by-step explanation of how to use it:Opening the C# In teractive Window: From the Visual Studio interface, navigate through the menus: View -> Ot her Windows -> C# Interactive.Using the Window: Once open, you can type any valid C# stat ement and press Enter to execute it. The environment reads the statement (Read), evaluates i
ts result (Eval), prints the output (Print), and then loops back to wait for more input (Loop).Be nefits: This tool is particularly useful for experimenting with new libraries, debugging code sn ippets, or learning C# syntax without the need to create a new project or write a full applicati on.This interactive tool saves time and simplifies the process of learning and testing C# code.
[Trivia]
The REPL environment in Visual Studio supports not only C# but also other .NET languages, making it versatile for developers working across different .NET frameworks.
Code Refactoring Tools in Visual Studio
Code refactoring tools help developers restructure existing code without changing its extern al behavior.
The example below shows how to use the "Extract Method" refactoring tool in Visual Studio t o improve code readability.
[Code]
// Original code before refactoring
public class Calculator
{
public void DisplaySum()
{
int a = 5;
int b = 10;
int sum = a + b;
Console.WriteLine("Sum: " + sum);
}
}
// Steps for refactoring in Visual Studio:
// 1. Highlight the code: int sum = a + b; Console.WriteLine("Sum: " + sum);
// 2. Right-click and select "Quick Actions and Refactorings"
// 3. Choose "Extract Method" and name the new method "CalculateAndDisplaySum"
[Result]
// Refactored code
public class Calculator
{
public void DisplaySum()
{
CalculateAndDisplaySum();
}
private void CalculateAndDisplaySum()
{
int a = 5;
int b = 10;
int sum = a + b;
Console.WriteLine("Sum: " + sum);
}
}
Refactoring is a critical skill for improving and maintaining the quality of code. The "Extract M
ethod" tool in Visual Studio allows you to take a block of code within a method and turn it in to a new method. This is useful for several reasons:Improves Readability: Smaller, well-named methods are easier to understand than long blocks of code.Reduces Complexity: By isolating sections of code, you reduce the complexity of individual methods.Enhances Reusability: Isol ated code can be reused in different parts of the program without duplication.Using refactori ng tools effectively can lead to more maintainable and error-free code.
[Trivia]
Visual Studio's refactoring tools are part of a larger suite of features known as IntelliSense, w hich also includes code completion, code hints, and powerful debugging tools that enhance developer productivity.4
Static Code Analysis in C#
Static code analysis is the process of analyzing source code for potential errors and issues be fore the program is run.
Below is an example using the popular C# static analysis tool "Roslyn Analyzers" to detect po tential issues in code.
[Code]
using System;
public class Example
{
public static void Main()
{
int a = 10;
int b = 0;
Console.WriteLine(a / b);
}
}
[Result]
This code, if analyzed, would alert a potential division by zero error.
Static code analysis tools, like Roslyn Analyzers, scrutinize your code by examining it without executing it. These tools help in identifying common coding flaws such as syntax errors, type checking errors, and potential runtime exceptions like the division by zero error shown above
. Using static analysis, developers can enhance code quality, adhere to coding standards, and reduce potential runtime errors.In C#, integrating static code analysis tools can be done thro ugh the Visual Studio IDE, where you can configure these tools to run automatically during b uild or manually upon request. This practice helps in maintaining a high standard of code he alth and reduces bug introduction during the development phase.
[Trivia]
Static analysis tools not only detect potential errors but also help in enforcing coding standar ds and practices. They can be configured to ensure that code adheres to organizational or co mmunity standards, such as naming conventions and layout guidelines.
Code Profiling in C#
Code profiling is the technique used to measure the runtime performance of a program, iden tifying bottlenecks and inefficiencies.
Here is how you might set up a simple profiling session using the Visual Studio Diagnostic To ols to analyze the performance of a C# application.
[Code]
using System;
using System.Diagnostics;
using System.Threading;
public class PerformanceTest
{
public static void Main()
{
Stopwatch stopwatch = new Stopwatch();
stopwatch.Start();
// Simulate some workload
Thread.Sleep(1000);
stopwatch.Stop();
Console.WriteLine("Execution time in milliseconds: " + stopwatch.ElapsedMilliseconds);
}
}
[Result]
Execution time in milliseconds: 1000
In this example, Stopwatch from System.Diagnostics is used to measure the time taken for a section of code to execute, simulating a simple profiling scenario. Code profiling in real-worl d applications involves more sophisticated tools and methodologies, often integrated into d evelopment environments like Visual Studio. These tools not only measure time but also anal yze CPU usage, memory consumption, and other important performance metrics.In Visual St udio, you can use the Diagnostic Tools during debugging to get a detailed view of your appli cation’s performance. This includes real-time CPU and memory usage, and you can even take snapshots of execution at various points to analyze performance issues deeply. Profiling is es sential for optimizing application performance and is especially crucial in environments wher e resources are limited or costly.
[Trivia]
Profiling tools in C# and .NET can be extremely granular, allowing developers to pinpoint exa ctly where the most time or resources are being spent. This capability is crucial for optimizing applications to run faster and more efficiently, especially in large-scale or high-load scenarios
.4
Code Documentation in C#
Code documentation is crucial in software development for maintaining code readability and facilitating understanding among developers.
Below is an example of C# code with comments explaining the functionality of each part.
[Code]
using System;
namespace DocumentationExample
{
/// <summary>
/// Main Program class that contains the entry point of the application.
/// </summary>
class Program
{
/// <summary>
/// The Main method is the entry point of any C# application.
/// </summary>
/// <param name="args">An array of command-line arguments</param> static void Main(string[] args)
{
Console.WriteLine("Hello, World!");
}
}
[Result]
Hello, World!
In the provided code, the 'using System;' directive includes the System namespace which con tains fundamental classes for handling I/O operations among others. The 'namespace Docum entationExample' organizes classes under a named scope to avoid name conflicts. The Progr am class serves as a container for the application's entry point, which is the Main method. Co mments in the code use XML tags like <summary> and <param> which are used by various tools to generate documentation automatically. This approach not only improves the readabi lity of the code but also allows for better maintenance and upscaling of the software develop ment project.
[Trivia]
XML documentation comments in C# are used by tools like Visual Studio to provide IntelliSe nse features, such as type descriptions and parameter information directly in the IDE, making the development process faster and more intuitive.
Assembly Versioning in C#
Assembly versioning is essential for managing dependencies in .NET applications, ensuring c ompatibility across different versions.
The example below demonstrates how to specify assembly versioning in a C# application usi ng AssemblyInfo.cs.
[Code]
using System.Reflection;
[assembly: AssemblyTitle("VersioningExample")]
[assembly: AssemblyDescription("A simple C# application to demonstrate assembly versionin g.")]
[assembly: AssemblyConfiguration("")]
[assembly: AssemblyCompany("Example Company")]
[assembly: AssemblyProduct("VersioningProduct")]
[assembly: AssemblyCopyright("Copyright 2023 Example Company")]
[assembly: AssemblyTrademark("")]
[assembly: AssemblyCulture("")]
[assembly: AssemblyVersion("1.0.0.0")]
[assembly: AssemblyFileVersion("1.0.0.0")]
[Result]
// This is a metadata configuration file, so there is no direct execution output, but the assem bly will have these version attributes.
AssemblyInfo.cs file is a part of the project where metadata about the assembly is specified.
This metadata includes the title, description, company, product, and most importantly, the ve rsion of the assembly. The 'AssemblyVersion' attribute dictates the version used by the runti me for binding, whereas the 'AssemblyFileVersion' is for file system and display purposes onl y. It’s crucial for managing dependencies in large applications where assemblies might need to interact with each other and specific version dependencies need to be maintained to ensu re compatibility.
[Trivia]
In .NET, different versions of the same assembly can coexist in the Global Assembly Cache (G
AC), allowing applications to specify and use the exact version they need, thus avoiding the "
DLL Hell" that can occur when multiple applications require different versions of the same as sembly.4
Localization and Globalization in C#
Understanding how to adapt your software for different languages and regions.
The following C# example demonstrates how to format dates and numbers according to the culture of the user.
[Code]
using System;
using System.Globalization;
public class Example
{
public static void Main()
{
// Set the culture to German (Germany)
CultureInfo ci = new CultureInfo("de-DE");
// Display a formatted date
Console.WriteLine(DateTime.Now.ToString("D", ci));
// Display a formatted number
Console.WriteLine((1234567.89).ToString("N", ci));
}
}
Montag, 20. April 2024
1.234.567,89
In this code:CultureInfo ci = new CultureInfo("de-DE"); creates a CultureInfo object for Germa n (Germany). This object encapsulates information about the culture-specific settings.DateTi me.Now.ToString("D", ci) formats the current date in a long date pattern specific to the Germ an culture, e.g., "Montag, 20. April 2024".(1234567.89).ToString("N", ci) formats the number w ith grouping separators and a decimal symbol appropriate for German culture, resulting in "1
.234.567,89".This example demonstrates the significance of using the CultureInfo class to for mat strings in ways that are appropriate for specific cultures, which is essential for creating gl obally aware applications.
[Trivia]
Localization involves adapting software for specific cultures and languages, while globalizatio n is the broader process of designing software that can be adapted to various cultures. C# pr ovides comprehensive support for these processes through classes in the System.Globalizati on namespace.
Data Types and Variables in C#
Understanding basic data types and variable declarations in C#.
Here's a simple C# example to demonstrate variable declaration and usage.
[Code]
using System;
public class DataTypes
{
public static void Main()
{
int integerNumber = 123;
double floatingPointNumber = 123.456;
string text = "Hello, world!";
bool booleanValue = true;
Console.WriteLine("Integer: " + integerNumber); Console.WriteLine("Double: " + floatingPointNumber); Console.WriteLine("String: " + text);
Console.WriteLine("Boolean: " + booleanValue);
}
}
Integer: 123
Double: 123.456
String: Hello, world!
Boolean: true
In the provided code:int integerNumber = 123; declares an integer variable.double floatingP
ointNumber = 123.456; declares a variable for floating-point numbers.string text = "Hello, w orld!"; declares a string variable.bool booleanValue = true; declares a boolean variable.These are examples of some basic data types in C#. Variables in C# are strongly typed, which mean s the type of a variable is known at compile time. This allows the compiler to perform type ch ecking before the code is executed, reducing runtime errors and improving performance.
[Trivia]
C# supports various data types, including built-in types (like int, double, bool, and string), us er-defined types (like classes and structs), and reference types. Understanding these is crucial for programming in C# as it affects memory management, performance, and type safety.4
Control Structures in C#
Control structures are fundamental elements in programming that allow you to control the fl ow of execution based on conditions. In C#, these include if-else statements, switch cases, lo ops like for, while, and foreach.
The following is a simple C# program demonstrating the use of an if-else statement to perfor m conditional logic based on user input.
[Code]
using System;
class Program {
static void Main() {
Console.WriteLine("Enter a number:");
int number = Convert.ToInt32(Console.ReadLine());
if (number > 0) {
Console.WriteLine("The number is positive.");
} else if (number < 0) {
Console.WriteLine("The number is negative.");
} else {
Console.WriteLine("The number is zero.");
}
}
}
[Result]
Depending on the input, the output will be "The number is positive.", "The number is negativ e.", or "The number is zero."
In the provided C# code, we start by including the System namespace which gives us access t o basic functionalities like console input and output. The Main method is the entry point of a C# application. Here, the program first prompts the user to enter a number and reads it as a string using Console.ReadLine(). This string is then converted to an integer with Convert.ToIn t32(). The if-else statement evaluates the number. If it's greater than zero, it prints that the nu mber is positive. If it's less than zero, it prints that the number is negative. Otherwise, it prints that the number is zero. The control structures allow the program to make decisions and exe cute different blocks of code based on the condition's result.
[Trivia]
Understanding how control structures work is critical as they form the backbone of most pro gramming tasks involving decision making and looping. Knowing when to use which type of control structure can significantly impact the readability and efficiency of your code.
Object-Oriented Programming in C#
Object-oriented programming (OOP) is a programming paradigm based on the concept of "
objects", which can contain data and code: data in the form of fields (often known as attribut es), and code, in the form of methods (actions).
Here is a simple example in C# that demonstrates the definition of a class with fields and met hods, and creating an object of that class.
[Code]
using System;
class Car {
public string color; // Field
public Car(string color) { // Constructor
this.color = color;
}
public void DisplayColor() { // Method
Console.WriteLine("The color of the car is " + color);
}
}
class Program {
static void Main() {
Car myCar = new Car("Red");
myCar.DisplayColor();
}
[Result]
The output will be "The color of the car is Red."
In the given C# example, a Car class is defined with one field (color) and one method (Displa yColor()). The Car constructor initializes the color field when a new object of Car is created. T
he Main method in the Program class creates an instance of Car named myCar, passing "Red
" as an argument to the constructor, which sets the car's color. The myCar.DisplayColor() met hod is then called to print the color to the console. This example demonstrates encapsulation
, one of the core concepts of OOP, by keeping the car's color data within the car object and u sing a method to access it.
[Trivia]
OOP concepts such as encapsulation, inheritance, and polymorphism are essential for creatin g scalable and maintainable software. Understanding these principles can help you better or ganize your code and reuse it across different parts of your programs or even in different pro jects.4
Interfaces in C#
Interfaces in C# define a contract that classes or structs can implement. They specify method s, properties, or events that implementing types must provide.
The following example demonstrates an interface ITransport and a class Car that implements this interface.
[Code]
using System;
interface ITransport
{
void Start();
void Stop();
}
class Car : ITransport
{
public void Start()
{
Console.WriteLine("Car is starting");
}
public void Stop()
{
Console.WriteLine("Car is stopping");
}
class Program
{
static void Main()
{
ITransport myCar = new Car();
myCar.Start();
myCar.Stop();
}
}
[Result]
Car is starting
Car is stopping
In the above code:The ITransport interface declares two methods: Start() and Stop(). These m ethods are declared but not implemented within the interface itself.The Car class implements the ITransport interface by providing concrete implementations of the Start and Stop metho ds.In the Main method, an instance of Car is created and assigned to an ITransport type varia ble. This demonstrates polymorphism where the interface type is used to interact with the im plementing object, allowing the same interface to be implemented by different classes with p otentially different behaviors for the methods.
[Trivia]
Interfaces are crucial in C# for creating flexible and scalable systems. They allow developers t o separate the definition of tasks from their implementation, facilitating dependency injectio n and mocking in unit tests, which are key components of test-driven development (TDD).
Events and Delegates in C#
Events and delegates in C# provide a way to handle notifications and implement the observe r pattern. Delegates are types that safely encapsulate methods, while events are messages se nt by an object to signal the occurrence of an action.
This example shows how to define a delegate, use it to declare an event, and trigger that eve nt.
[Code]
using System;
public delegate void NotificationEventHandler(string message); class EventPublisher
{
public event NotificationEventHandler NotificationReceived; public void Notify(string message)
{
NotificationReceived?.Invoke(message);
}
}
class EventSubscriber
{
public void OnNotificationReceived(string message)
{
Console.WriteLine("Received message: " + message);
}
}
class Program
{
static void Main()
{
EventPublisher publisher = new EventPublisher();
EventSubscriber subscriber = new EventSubscriber();
publisher.NotificationReceived += subscriber.OnNotificationReceived; publisher.Notify("Hello, this is an event notification!");
}
}
[Result]
Received message: Hello, this is an event notification!
In this code:A delegate NotificationEventHandler is defined that describes a method taking a single string parameter and returning void.EventPublisher has an event NotificationReceived of type NotificationEventHandler.The Notify method of EventPublisher triggers the event, but only if there are subscribers (checked with ?.Invoke which is a null-conditional operator preve nting a NullReferenceException).EventSubscriber defines a method OnNotificationReceived t hat matches the delegate signature and handles the event by writing a message to the conso le.In the Main method, an instance of EventSubscriber subscribes to the NotificationReceived event of an EventPublisher instance. When Notify is called, it leads to the output on the cons ole through the subscriber's method.
[Trivia]
Events and delegates are fundamental for designing extensible and maintainable application s in C#. They allow objects to communicate without being tightly coupled, which is essential f or implementing designs such as Model-View-Controller (MVC) architectures.4
File I/O in C#
Understanding file operations in C# is essential for manipulating data stored in files.
Here we demonstrate how to write and read text to and from a file using C#.
[Code]
using System;
using System.IO;
class FileIOExample
{
static void Main()
{
string filePath = @"example.txt";
// Write text to a file
string contentToWrite = "Hello, C#! This is file writing example."; File.WriteAllText(filePath, contentToWrite);
// Read text from a file
string contentRead = File.ReadAllText(filePath);
Console.WriteLine(contentRead);
}
}
Hello, C#! This is file writing example.
In the provided example, we use File.WriteAllText to write a string to a file. This method takes two arguments: the file path and the content to write. It automatically handles opening the fil e, writing the content, and then closing the file. If the file specified does not exist, it creates a new one. Conversely, if the file exists, this method will overwrite the existing content.Next, we use File.ReadAllText to read the contents of the file. This method takes the file path as an arg ument and returns the content of the file as a string. It handles opening the file, reading the contents, and closing the file efficiently.This example is a straightforward demonstration of w riting to and reading from a text file, showing both the simplicity and power of file I/O operat ions in C#. This is crucial for tasks such as data logging, configuration management, and pers isting data between application sessions.
[Trivia]
The methods File.WriteAllText and File.ReadAllText are part of the System.IO namespace, whi ch provides tools for file and data stream manipulation. These methods are efficient and suit able for small to medium-sized files. For handling larger files or more complex file operations
, one might consider using StreamWriter or StreamReader for more control and better perfor mance.
Error Handling in C#
Error handling in C# is primarily done using exceptions, a method of responding to errors in a controlled way.
This example shows how to handle exceptions that may occur during file I/O operations in C
#.
[Code]
using System;
using System.IO;
class ErrorHandlingExample
{
static void Main()
{
try
{
string filePath = @"nonexistent.txt";
string content = File.ReadAllText(filePath);
Console.WriteLine(content);
}
catch (FileNotFoundException ex)
{
Console.WriteLine("File not found: " + ex.Message);
catch (Exception ex)
{
Console.WriteLine("An error occurred: " + ex.Message);
}
}
}
[Result]
File not found: Could not find file '...\nonexistent.txt'.
The code demonstrates how to handle exceptions using a try block that encloses code which might throw an exception, and catch blocks to handle the exceptions. Multiple catch blocks a llow for handling different types of exceptions in different ways. In this example, if the file do es not exist, a FileNotFoundException is caught and handled by printing a specific error mess age. Any other type of exception that might be thrown (handled by the generic Exception cla ss) will be caught by the subsequent catch block.Using structured exception handling allows developers to manage errors gracefully while keeping the application running smoothly. It al so helps in debugging by providing clear information on what went wrong and where.
[Trivia]
Structured exception handling in C# provides a robust framework for dealing with errors syst ematically. FileNotFoundException is a specific type of IOException that occurs when an atte mpt to access a file that does not exist is made. Handling exceptions specifically, like FileNotF
oundException, helps in implementing more precise and user-friendly error handling. Additio nally, it’s a good practice to log errors for later diagnosis which can be invaluable during mai ntenance and further development.4
Data Access in C#
Understanding how to interact with databases using C#.
Here, we'll look at a simple example of connecting to a SQL database using C# and performi ng a query.
[Code]
using System;
using System.Data.SqlClient;
class Program
{
static void Main()
{
string connectionString = "Server=myServerAddress;Database=myDataBase;User Id=my Username;Password=myPassword;";
using (SqlConnection connection = new SqlConnection(connectionString))
{
connection.Open();
SqlCommand command = new SqlCommand("SELECT TOP 10 * FROM Customers", c onnection);
SqlDataReader reader = command.ExecuteReader();
while (reader.Read())
{
Console.WriteLine(reader["CustomerName"].ToString());
}
}
}
}
[Result]
The output will display the names of the top 10 customers from the Customers table in the d atabase.
In this code:We import necessary namespaces. System.Data.SqlClient is used for SQL Server d atabase operations.The connectionString contains details needed to connect to the database
. Adjust Server, Database, User Id, and Password as per your setup.SqlConnection manages t he connection to the database. It's wrapped in a using statement to ensure that resources ar e freed automatically when the connection is closed.SqlCommand represents a SQL comman d to be executed against the database.ExecuteReader executes the command and returns a S
qlDataReader to read data from the database.Inside the while loop, data is read row-by-row.
reader["CustomerName"] fetches the customer name from the current row.It's critical to han dle database connections carefully to avoid resource leaks and ensure security.
[Trivia]
Always ensure to handle exceptions and manage connections properly. Using parameterized queries or stored procedures can help prevent SQL injection attacks.
Web Development with C#
Creating web applications using C# and ASP.NET.
Below is a basic example of a C# ASP.NET application that displays "Hello, world!" on a webp age.
[Code]
using System;
using Microsoft.AspNetCore;
using Microsoft.AspNetCore.Hosting;
using Microsoft.AspNetCore.Builder;
using Microsoft.AspNetCore.Http;
public class Program
{
public static void Main(string[] args)
{
BuildWebHost(args).Run();
}
public static IWebHost BuildWebHost(string[] args) => WebHost.CreateDefaultBuilder(args)
.Configure(app =>
{
app.Run(async (context) =>
await context.Response.WriteAsync("Hello, world!");
});
})
.Build();
}
[Result]
When run, this code will host a web server displaying "Hello, world!" on the webpage.
In this code:We set up a simple ASP.NET Core web application.Microsoft.AspNetCore namesp aces are used for building web applications.IWebHost and WebHost manage the web server'
s lifecycle.The Configure method configures the app's request processing pipeline using a mi ddleware that handles all HTTP requests and responds with "Hello, world!".app.Run is a termi nal middleware because it does not call a next middleware in the pipeline.This example is a f oundation for more complex web applications and illustrates how middleware components a re used to handle HTTP requests.
[Trivia]
ASP.NET Core supports dependency injection out of the box, which helps in building loosely coupled and easily testable applications.4
Mobile Development with C#
Learning how to develop mobile applications using C# with Xamarin.
Below is a simple example of how to create a basic mobile app with a single button that displ ays a message when clicked.
[Code]
using System;
using Xamarin.Forms;
namespace SimpleMobileApp
{
public class App : Application
{
public App()
{
var button = new Button { Text = "Click Me!" }; button.Clicked += OnButtonClicked;
MainPage = new ContentPage
{
Content = button
};
}
private void OnButtonClicked(object sender, EventArgs e)
MainPage.DisplayAlert("Alert", "Button was clicked!", "OK");
}
static void Main(string[] args)
{
Xamarin.Forms.Platforms.Windows.Application.Start(App.Main);
}
}
}
[Result]
An alert box with the message "Button was clicked!" appears when the button is clicked.
This code snippet demonstrates the basic structure of a Xamarin.Forms mobile app in C#. Th e App class inherits from Application, which is a fundamental part of the Xamarin.Forms fram ework. The MainPage property of Application is set to a new ContentPage that contains a sin gle Button. The Clicked event of the button is wired to an event handler OnButtonClicked, wh ich triggers a display alert when the button is clicked. The Main method initializes the applica tion on a platform-specific basis, in this case, assumed for Windows with Xamarin.Forms.The key components of this example include:Xamarin.Forms: A framework that allows developers to create user interfaces in C# that are rendered into native controls on iOS, Android, and Wi ndows.Event Handling: Demonstrates how to handle user interactions using event handlers (
Clicked event).UI Elements: Introduction to basic UI elements like Button and ContentPage.
[Trivia]
Xamarin uses a single code base to deploy on multiple platforms, which includes the use of n ative APIs and UI controls. This approach provides a seamless user experience similar to that of applications written in native platform languages.
Game Development with Unity and C#
How to start creating games using C# in Unity.
Here's a basic example showing how to create a simple script in Unity that moves an object when the arrow keys are pressed.
[Code]
using UnityEngine;
public class MoveObject : MonoBehaviour
{
void Update()
{
float moveX = Input.GetAxis("Horizontal") * Time.deltaTime * 10; float moveY = Input.GetAxis("Vertical") * Time.deltaTime * 10; transform.Translate(moveX, 0, moveY);
}
}
[Result]
The object moves in the game scene based on the arrow key inputs.
This script demonstrates using Unity's MonoBehaviour class to create game behavior. The Up date method is called once per frame and checks for user input. Input.GetAxis retrieves the m ovement input from the horizontal and vertical axes, which are typically linked to arrow keys or a joystick. The movement values are scaled by Time.deltaTime to make the movement sm ooth and frame rate independent, and transform.Translate is used to move the object in the game world.Key concepts include:MonoBehaviour: The base class from which every Unity scri pt derives, allowing the script to behave like a component of a GameObject.Frame Update Cy cle: The Update function is crucial as it is executed every frame, providing the ability to handl e real-time changes in game states or player inputs.Input Handling: Unity provides the Input class to handle user input from various sources including the keyboard, mouse, and game co ntrollers.Movement and Transformation: Demonstrating the use of the transform object to c hange the position of game elements. The example makes the object move according to use r input, illustrating basic interactivity in a game.
[Trivia]
Unity supports C# because it is a powerful, type-safe, object-oriented language that integrat es well with the engine’s extensive APIs and, crucially, with the .NET Framework, enhancing g ame development with a vast range of libraries and tools.4
IoT Development with C#
Learn how to develop Internet of Things (IoT) applications using C# on platforms like Windo ws 10 IoT Core.
The following C# code example demonstrates how to read temperature data from a sensor c onnected to a Raspberry Pi running Windows 10 IoT Core.
[Code]
using System;
using Windows.Devices.Gpio;
using Windows.Devices.I2c;
public class TemperatureSensor
{
private I2cDevice sensor;
public TemperatureSensor()
{
InitSensor();
}
private void InitSensor()
{
var settings = new I2cConnectionSettings(0x40); // Sensor I2C address settings.BusSpeed = I2cBusSpeed.FastMode;
var controller = await GpioController.GetDefaultAsync();
sensor = I2cDevice.FromIdAsync(controller.GetDeviceSelector(), settings);
}
public double ReadTemperature()
{
byte[] tempData = new byte[2];
sensor.Read(tempData);
int rawTemp = tempData[0] << 8 | tempData[1];
return (rawTemp & 0xFFF) / 16.0;
}
}
[Result]
The code reads the temperature data from the sensor and returns it as a double value.
In this example, I2cDevice class is used to communicate with an I2C device, in this case, a te mperature sensor. The I2C connection settings are defined with the device's address and bus speed. The ReadTemperature method reads two bytes from the sensor, combines them to fo rm an integer, and converts it to a temperature in Celsius by following the sensor's data form at specification.This setup requires the Windows IoT Extension SDK and depends on the spec ific hardware capabilities of the Raspberry Pi and the type of temperature sensor used (like th e commonly used TMP102). Make sure your device pinouts and I2C address are correctly con figured to match those in the code.Additionally, proper error handling and asynchronous ma nagement should be considered for real-world applications to handle potential communicati on errors or data corruption.
[Trivia]
The Windows 10 IoT Core operating system is specifically designed for small, secured smart devices, making it an ideal choice for developing IoT solutions. Using C# and the Universal W
indows Platform (UWP), developers can create powerful, cross-device applications.
Machine Learning with ML.NET
Introduction to implementing machine learning models in C# using ML.NET.
This C# example showcases how to build and train a simple regression model using ML.NET t o predict house prices.
[Code]
using Microsoft.ML;
using Microsoft.ML.Data;
public class HouseData
{
[LoadColumn(0)] public float Size { get; set; }
[LoadColumn(1)] public float Price { get; set; }
}
public class Prediction
{
[ColumnName("Score")] public float PredictedPrice { get; set; }
}
class Program
{
static void Main(string[] args)
{
var mlContext = new MLContext();
var data = mlContext.Data.LoadFromTextFile<HouseData>("./data/house_data.csv", has Header: true, separatorChar: ',');
var pipeline = mlContext.Transforms.Concatenate("Features", "Size")
.Append(mlContext.Regression.Trainers.LbfgsPoissonRegression()); var model = pipeline.Fit(data);
var predictionEngine = mlContext.Model.CreatePredictionEngine<HouseData, Predictio n>(model);
var prediction = predictionEngine.Predict(new HouseData { Size = 2.5f }); Console.WriteLine($"Predicted Price: {prediction.PredictedPrice}");
}
}
[Result]
Predicted Price: [The output will display the predicted price based on the model training.]
In this code snippet, the MLContext class is instantiated to start the machine learning job. Lo adFromTextFile method is used to load training data from a CSV file, mapping it to a user-de fined HouseData class with Size and Price properties marked by the LoadColumn attribute.Th e Concatenate method in the pipeline combines specified columns into a single feature vect or which is crucial for ML algorithms. LbfgsPoissonRegression is a regression algorithm used here to predict numerical values.For prediction, a PredictionEngine is created from the traine d model to predict house prices for new data. This example demonstrates how ML.NET can b e used to train and consume machine learning models directly in C#.It is important to note t hat the accuracy of predictions largely depends on the quality and quantity of the training da ta, and appropriate feature engineering.
[Trivia]
ML.NET is an open-source and cross-platform machine learning framework for .NET develop ers. It allows developers to integrate machine learning into their applications without needin g to switch to other programming languages traditionally associated with machine learning, l ike Python.4
True and False Values in C#
In C#, the Boolean data type (bool) is used to represent true and false values, which are fund amental in controlling flow with conditions and loops.
The following example demonstrates how C# treats different values as true or false when con verted to a Boolean context.
[Code]
bool trueValue = true;
bool falseValue = false;
bool defaultBool = new bool(); // Default value for bool Console.WriteLine($"True Value: {trueValue}"); Console.WriteLine($"False Value: {falseValue}"); Console.WriteLine($"Default Bool Value (should be false): {defaultBool}");
[Result]
True Value: True
False Value: False
Default Bool Value (should be false): False
In C#, the Boolean type (bool) only accepts the values true or false. The default value for a bo ol variable that hasn't been explicitly assigned is false. This behavior is crucial in programmin g because it ensures that Boolean variables that are uninitialized will behave predictably in co nditional statements, typically not triggering if statements or loops like while. Understanding this default setting helps in debugging and writing more reliable code, especially in conditio ns where multiple Boolean variables interact.
[Trivia]
It's important to note that unlike some other programming languages (like JavaScript or Pyth on), C# does not treat numbers (like 0 or 1), strings, or null values as inherently true or false.
An explicit conversion or comparison must be performed to use these types in Boolean cont exts.
Boolean Logic in C#
Boolean logic in C# involves using logical operators to combine or invert Boolean values, ess ential for complex conditions and decision-making in code.
Here we demonstrate the use of logical AND (&&), logical OR (||), and logical NOT (!) operato rs in a simple C# program.
[Code]
bool a = true;
bool b = false;
bool andResult = a && b; // Logical AND
bool orResult = a || b; // Logical OR
bool notResult = !b; // Logical NOT
Console.WriteLine($"AND Result: {andResult}"); Console.WriteLine($"OR Result: {orResult}"); Console.WriteLine($"NOT Result: {notResult}");
[Result]
AND Result: False
OR Result: True
NOT Result: True
The logical AND operator (&&) returns true only if both operands are true. The logical OR op erator (||) returns true if at least one operand is true. The logical NOT operator (!) inverts the Boolean value, turning true into false and vice versa. These operators are foundational in crea ting conditional logic in programs, such as determining if multiple conditions are satisfied be fore executing a block of code or ensuring at least one of several conditions is met. Understa nding these operators allows for precise control over the flow of the program, particularly in handling complex decision-making scenarios.
[Trivia]
A useful tip for optimizing performance in C# is to use short-circuit evaluation with the && a nd || operators. For example, in a && b, if a is false, C# will not evaluate b because the result cannot be true regardless of b's value. This can prevent unnecessary computation, especially when the second condition involves method calls or heavy calculations.4
Nullable Boolean Types in C#
In C#, a nullable boolean type can hold three values: true, false, and null. This additional null option allows for representing scenarios where a value is undefined or unknown.
Here is an example that demonstrates how to declare and use nullable booleans in C#:
[Code]
bool? isCompleted = null;
if (isCompleted == true)
{
Console.WriteLine("Task is completed.");
}
else if (isCompleted == false)
{
Console.WriteLine("Task is not completed.");
}
else
{
Console.WriteLine("Completion status is unknown.");
}
[Result]
Completion status is unknown.
In this code:bool? is the nullable boolean type in C#. The question mark ? makes any value ty pe nullable, indicating that it can also hold a null value.The variable isCompleted is initially se t to null, which represents an undefined state.The if statement checks the value of isComplet ed. Since it is null, neither the true nor false condition is met, so it falls to the else block, print ing "Completion status is unknown."This example is useful in real-world applications where a binary true/false response may not suffice, and an unknown state needs to be handled.
[Trivia]
Nullable types are particularly useful in database interactions where table columns can be op tional (i.e., can contain null values), reflecting the importance of being able to handle the abs ence of data gracefully in software applications.
Truth Tables in C#
A truth table lists all possible logical relations between boolean variables. In programming, u nderstanding truth tables can help in designing complex conditional logic.
Below is a simple C# program that displays a truth table for the logical AND operator:
[Code]
bool[] values = { true, false };
Console.WriteLine("A\tB\tA AND B");
foreach (bool a in values)
{
foreach (bool b in values)
{
Console.WriteLine($"{a}\t{b}\t{a && b}");
}
}
[Result]