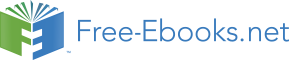

It checks the length of the value in the form element to see
if it is less than the parameter specified. It will return FALSE
if the form element is smaller than the value specified.
Example use:
$this->form_validation-
>set_rules('item1',
'Item1',
'min_length[12]');
max_length
Yes
It is the reverse of min_length; it checks if the length of the
value in the form element is greater than the parameter
specified. It will return FALSE if the form element is greater
than the value specified. Example use:
$this->form_validation-
>set_rules('item1',
'Item1',
'max_length[12]');
exact_length
Yes
It checks if the length of the value in the form element is the
exact value as compared to the specified parameter. It will
return FALSE if the form element is anything other than what
is specified. Example use:
$this->form_validation-
>set_rules('item1',
'Item1', 'exact_length
[12]');
greater_than
Yes
It checks if the value in the form element is greater than a
supplied parameter. It will return FALSE if the form element
is less than the parameter value or that value is not numeric.
Example use:
$this->form_validation-
>set_rules('item1',
'Item1',
'greater_than[12]');
104
Chapter 5
Rule
Parameter
Description
less_than
Yes
It is the reverse of greater_than. It checks if the value in the
form element is less than a supplied parameter. It will return
FALSE if the form element is greater than the parameter
value or that value is not numeric. Example use:
$this->form_validation-
>set_rules('item1',
'Item1',
'less_than[12]');
alpha
No
It checks if the value in the form element contains
alphabetical characters only. It will return FALSE if the
form element value is anything other than that.
alpha_numeric No
It checks if the value in the form element contains
alphabetical and integer values only. It will return FALSE
if the form element value is anything other than that.
alpha_dash
No
It checks if the value of the form element contains anything
other than alpha-numeric characters, underscores or dashes.
It will return FALSE if it contains any other value.
numeric
No
It checks if the value of the form element contains anything
other than numeric characters. It will return FALSE if the form
element contains anything other than that.
integer
No
It checks if the value of the form element contains anything
other than integer values. It will return FALSE if the form
element contains anything other than that.
decimal
Yes
It checks if the value of the form element contains a decimal
value, that is a number separated with a decimal point (.),
otherwise it will return FALSE.
is_natural
No
It checks if the value of the form element contains anything
other than natural numbers—that is to say anything other
than 1, 2, 3, 4, 5, and so on. It will return FALSE if the form
element contains anything other than that.
is_natural_no_ No
It checks if the value of the form element contains anything
zero
other than natural numbers, which are greater than zero.
It will return FALSE if the value is anything other than natural
numbers or zero.
valid_email
No
It checks if the value of the form element contains a valid
e-mail as calculated by Regular Expression within CodeIgniter.
It will return FALSE if the form element does not contain a
valid e-mail address.
105
Managing Data In and Out
Rule
Parameter
Description
valid_emails
No
It checks if the value of the form element contains valid
e-mail addresses as calculated by Regular Expression within
CodeIgniter. It will return FALSE if the form element does not
contain a valid e-mail address.
valid_ip
No
It checks if the supplied IP address is valid.
valid_base64
No
It returns FALSE if the supplied string contains anything other
than valid Base64 characters.
There are also some basic config changes we'll need to make before we start working through our recipes. We're going to amend the path/to/codeigniter/application/config/
config.php file.
Config Item
Change to Value Description
$config['global_xrsf_
TRUE
It specifies whether CodeIgniter
filtering']
always filters for Cross-Site
Scripting. For security purposes
it is recommended that this is set
to TRUE.
$config['csrf_protection']
TRUE
It specifies whether to use
Cross-Site Request Forgery
protection. For security purposes
it is recommended that this is
set to TRUE.
$config['csrf_token_name']
Your own string
It specifies that if the user closes
his/her browser the session
becomes void.
$config['csrf_cookie_name']
Another string of It specifies whether the cookie
your choice
should be encrypted on the user's
computer. For security purposes
this should be set to TRUE.
$config['csrf_expire']
7200
It specifies the length of time
in seconds.
How to do it...
Create the following files in your CodeIgniter install:
f
/path/to/codeigniter/application/controllers/form.php
f
/path/to/codeigniter/application/views/new_record.php
1. Add the following code into the path/to/codeigniter/application/
controllers/form.php file:
106
Chapter 5
<?php if (!defined('BASEPATH')) exit('No direct script
access allowed');
class Form extends CI_Controller {
function __construct() {
parent::__construct();
$this->load->helper('form');
$this->load->helper('url');
$this->load->helper('security');
$this->load->library('form_validation');
}
public function index() {
redirect('form/submit_form');
}
public function submit_form() {
$this->form_validation->set_error_delimiters('', '<br
/>');
$this->form_validation->set_rules('first_name', 'First
Name', 'required|min_length[1]|max_length[125]');
$this->form_validation->set_rules('last_name', 'Last
Name', 'required|min_length[1]|max_length[125]');
$this->form_validation->set_rules('email', 'Email',
'required|min_length[1]|max_length[255]
|valid_email');
$this->form_validation->set_rules('contact', 'Contact',
'required|min_length[1]|max_length[1]|
integer|is_natural');
$this->form_validation->set_rules('answer', 'Question',
'required|min_length[1]|max_length[2]|
integer|is_natural');
// Begin validation
if ($this->form_validation->run() == FALSE) { //
First load, or problem with form
$this->load->view('new_record');
}
else {
// Validation passed, now escape the data
$data = array(
'first_name' => $this->input-
>post('first_name'),
'last_name' => $this->input->post('last_name'),
'email' => $this->input->post('email'),
'contact' => $this->input->post('contact'),
107
Managing Data In and Out
'answer' => $this->input->post('answer')
);
echo '<pre>';
var_dump($data);
echo '</pre>';
}
}
}
2. Add the following code into the path /to/codeigniter/application/views/
new_record.php file:
<?php echo form_open('form/submit_form') ; ?>
<?php if (validation_errors()) : ?>
<h3>Whoops! There was an error:</h3>
<p><?php echo validation_errors(); ?></p>
<?php endif; ?>
<table border="0" >
<tr>
<td>First Name</td>
<td><?php echo form_input(array('name' => 'first_
name', 'id' => 'first_name', 'value' => '', 'maxlength' => '100',
'size' => '50', 'style' => 'width:100%')); ?></td>
</tr>
<tr>
<td>Last Name</td>
<td><?php echo form_input(array('name' => 'last_name',
'id' => 'last_name', 'value' => '', 'maxlength' => '100', 'size'
=> '50', 'style' => 'width:100%')); ?></td>
</tr>
<tr>
<td>User Email</td>
<td><?php echo form_input(array('name' => 'email',
'id' => 'email', 'value' => '', 'maxlength' => '100', 'size' =>
'50', 'style' => 'width:100%')); ?></td>
</tr>
<tr>
<td>Do you want to be contacted in the future?</td>
<td><?php echo 'Yes'.form_checkbox('contact', '1',
TRUE).'No'.form_checkbox('contact', '0', FALSE); ?></td>
</tr>
<tr>
<td>What is 10 + 5?</td>
<td><?php echo form_input(array('name' => 'answer',
108
Chapter 5
'id' => 'answer', 'value' => '', 'maxlength' => '100', 'size' =>
'50', 'style' => 'width:100%')); ?></td>
</tr>
</table>
<?php echo form_submit('submit', 'Submit'); ?>
or <?php echo anchor('form', 'cancel'); ?>
<?php echo form_close(); ?>
How it works...
CodeIgniter will first run public function index(), which will immediately redirect to public function submit_form().The submit_form() function will set our error delimiters with the line $this->form_validation->set_error_delimiters('',
'<br />'); and then list the validation rules for each form element: $this->form_validation->set_rules('first_name', 'First Name',
'required|min_length[1]|max_length[125]');
$this->form_validation->set_rules('last_name', 'Last Name',
'required|min_length[1]|max_length[125]');
$this->form_validation->set_rules('email', 'Email',
'required|min_length[1]|max_length[255]|valid_email');
$this->form_validation->set_rules('contact', 'Contact',
'required|min_length[1]|max_length[1]|integer|is_natural');
$this->form_validation->set_rules('answer', 'Question',
'required|min_length[1]|max_length[2]|integer|is_natural');
As the form is being run for the first time $this->form_validation->run() will return FALSE and so load the view file $this->load->view('new_record');, which will render the form to the user. The user can then enter his/her details into the form. Once the user clicks on the Submit button, CodeIgniter again loads public function submit_form(), but this time, as the form is being submitted the validation rules are applied to the data being submitted. CodeIgniter will compare the data submitted against the rules and return FALSE
if that data fails to match the rules in validation. If those rules are not met, the user will see error messages in the view. The following code checks if there are any validation errors, if so it will display them one by one:
<?php if (validation_errors()) : ?>
<h3>Whoops! There was an error:</h3>
<p><?php echo validation_errors(); ?></p>
<?php endif; ?>
109
Managing Data In and Out
Preparing user input
The validation rules can also be used to prepare input for you. For example, you can trim() whitespace from the input or apply htmlspecialchars(). Any PHP function can be used, as long as that function accepts one parameter as an argument by default.
How to do it...
Let's assume that we want to trim() whitespaces from the beginning and end of the input and generate an md5 hash of the input:
$this->form_validation->set_rules('input_name', 'Input Name',
'trim|md5');
Sticky form elements in CodeIgniter
It is good for user experience to offer feedback; we do this in the preceding sections with validation_errors(), but it is also useful to keep user data in form elements to save them having to re-type everything, should there be an error. To do this, we need to use CodeIgniter's set_value() function.
Getting ready
Make sure that you load $this->load->helper('form'); from within the __constructor() of the controller; however, you can always autoload the helper from /path.to/codeigniter/application/config/autoload.php.
How to do it...
We're going to edit the /path/to/codeigniter/application/views/new_record.
php file.
3. Amend the file to show the following (changes in bold):
<?php echo form_open('form/submit_form') ; ?>
<?php if (validation_errors()) : ?>
<h3>Whoops! There was an error:</h3>
<p><?php echo validation_errors(); ?></p>
<?php endif; ?>
<table border="0" >
<tr>
<td>First Name</td>
<td><?php echo form_input(array('name' => 'first_
110
Chapter 5
name', 'id' => 'first_name', 'value' => set_value('first_
name', ''), 'maxlength' => '100', 'size' => '50', 'style' =>
'width:100%')); ?></td>
</tr>
<tr>
<td>Last Name</td>
<td><?php echo form_input(array('name' => 'last_name',
'id' => 'last_name', 'value' => set_value('last_name', ''),
'maxlength' => '100', 'size' => '50', 'style' => 'width:100%'));
?></td>
</tr>
<tr>
<td>User Email</td>
<td><?php echo form_input(array('name' => 'email',
'id' => 'email', 'value' => set_value('email', ''), 'maxlength' =>
'100', 'size' => '50', 'style' => 'width:100%')); ?></td>
</tr>
<tr>
<td>Do you want to be contacted in the future?</td>
<td><?php echo 'Yes'.form_checkbox('contact', '1',
TRUE).'No'.form_checkbox('contact', '0', FALSE); ?></td>
</tr>
<tr>
<td>What is 10 + 5?</td>
<td><?php echo form_input(array('name' => 'answer',
'id' => 'answer', 'value' => set_value('answer', ''), 'maxlength'
=> '100', 'size' => '50', 'style' => 'width:100%')); ?></td>
</tr>
</table>
<?php echo form_submit('submit', 'Submit'); ?>
or <?php echo anchor('form', 'cancel'); ?>
<?php echo form_close(); ?>
How it works...
Essentially, it is exactly the same functionality as the Validating User Input recipe, except that now the CodeIgniter function, set_value(), populates the form element value with the data submitted previously by the user.
111
Managing Data In and Out
Displaying errors next to form items
In the preceding example, we displayed errors one by one at the top of the HTML page; however, you may wish to display each individual error closer to the form element to which it refers.
How to do it...
We're going to amend the /path/to/codeigniter/application/views/new_record.
php file.
1. Amend the code to reflect the following (changes in bold):
<?php echo form_open('form/submit_form') ; ?>
<?php if (validation_errors()) : ?>
<h3>Whoops! There was an error:</h3>
<?php endif; ?>
<table border="0" >
<tr>
<td>First Name</td>
<?php if (form_error('first_name')) : ?>
<?php echo form_error('first_name') ; ?>
<?php endif ; ?>
<td><?php echo form_input(array('name' => 'first_
name', 'id' => 'first_name', 'value' => set_value('first_
name', ''), 'maxlength' => '100', 'size' => '50', 'style' =>
'width:100%')); ?></td>
</tr>
<tr>
<td>Last Name</td>
<?php if (form_error('last_name')) : ?>
<?php echo form_error('last_name') ; ?>
<?php endif ; ?>
<td><?php echo form_input(array('name' => 'last_name',
'id' => 'last_name', 'value' => set_value('last_name', ''),
'maxlength' => '100', 'size' => '50', 'style' => 'width:100%'));
?></td>
</tr>
<tr>
<td>User Email</td>
<?php if (form_error('email')) : ?>
<?php echo form_error('email') ; ?>
<?php endif ; ?>
<td><?php echo form_input(array('name' => 'email',
'id' => 'email', 'value' => set_value('email', ''), 'maxlength' =>
'100', 'size' => '50', 'style' => 'width:100%')); ?></td>
</tr>
<tr>
112
Chapter 5
<?php if (form_error('contact')) : ?>
<?php echo form_error('contact') ; ?>
<?php endif ; ?>
<td>Do you want to be contacted in the future?</td>
<td><?php echo 'Yes'.form_checkbox('contact', '1',
TRUE).'No'.form_checkbox('contact', '0', FALSE); ?></td>
</tr>
<tr>
<td>What is 10 + 5?</td>
<td><?php echo form_input(array('name' => 'answer',
'id' => 'answer', 'value' => set_value('answer', ''), 'maxlength'
=> '100', 'size' => '50', 'style' => 'width:100%')); ?></td>
</tr>
</table>
<?php echo form_submit('submit', 'Submit'); ?>
or <?php echo anchor('form', 'cancel'); ?>
<?php echo form_close(); ?>
How it works...
Essentially, it's exactly the same validation functionality as the preceding recipe; the only change is how we're displaying the errors. We have removed the line <p><?php echo validation_errors(); ?></p>, as we're not listing the errors one by one. We have added the CodeIgniter's form_error() statement, passing it the name of the HTML form element so that if CodeIgniter's validation class discovers that the posted form data does not meet the parameters assigned to it as validation rules, an error will be displayed above the form element.
Reading files from the filesystem
Although you're probably going to be writing and reading data in a database you will certainly come in contact with the requirement to write something to the disk, and read from files stored on it. CodeIgniter can support several methods for interacting with files.
Getting ready
There are no configuration options to change here, but ensure that you load the file helper in your controller constructor (and also the url helper):
function __construct() {
parent::__construct();
$this->load->helper('url');
$this->load->helper('file');
}
113
Managing Data In and Out
How to do it...
We're going to read files from the disk and display details about them to a view.
Firstly, we're going to create two files:
f
/path/to/codeigniter/application/controllers/file.php
f
/path/to/codeigniter/application/views/file/view_file.php
1. Add the following code into /path/to/codeigniter/application/
controllers/file.php:
<?php if (!defined('BASEPATH')) exit('No direct script access
allowed');
class File extends CI_Controller {
function __construct() {
parent::__construct();
$this->load->helper('url');
$this->load->helper('file');
}
public function index() {
redirect('file/view_all_files');
}
public function view_all_files() {
$data['dir'] = '/full/path/to/read';
$data['files'] = get_dir_file_info($data['dir']);
$this->load->view('files/view_file', $data);
}
}
2. Add the following code into /path/to/codeigniter/application/views/
files/view_file.php:
<html>
<head>
<title>Viewing Files</title>
</head>
<body>
<?php echo anchor('file/create_file', 'Create File'); ?>
<?php echo anchor('file/read_file', 'Read File'); ?>
<?php echo anchor('file/view_all_files', 'View Files'); ?>
<table border="1">
<tr>
<td><b>Filename</b></td>
<td><b>Size</b></td>
<td><b>Created</b></td>
<td colspan="3">Actions</td>
114
Chapter 5
</tr>
<?php foreach ($files as $file) : ?>
<tr>
<td>
<?php if (is_dir($file['server_path'])) :
?>
<b><?php echo $file['name']; ?></b>
<?php else : ?>
<?php echo $file['name']; ?>
<?php endif; ?>
</td>
<td>
<?php echo $file['size']; ?>
</td>
<td>
<?php echo date("d/m/Y H:i:s",
$file['date']); ?>
</td>
<td>
<?php echo anchor('file/edit_file/' .
$file['name'], 'Edit'); ?>
<?php echo anchor('file/delete_file/' .
$file['name'], 'Delete'); ?>
<?php echo anchor('file/view_file/' .
$file['name'], 'View'); ?>
</td>
</tr>
<?php endforeach; ?>
</table>
</body>
</html>
How it works...
The business end of this is the controller, function view_all_files(). We're doing three things. First, is setting the target directory with which we wish to read the line $data['dir']
= '/full/path/to/read'; o