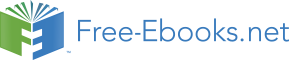

print.
Hence the first one will print out the account number, which happens to be
391023123
in this case. The remaining eight print lines will then list the remaining eight fields in the record, as shown on the listing above. Note that the last field is the account balance and it is
1620,
which indicates an amount of
$16.20.
The very last keyword is
end,
which will close the account file and end the program. That is all there is to the program with the main activity consisting of a read, nine print lines and an end. This program simply opens and reads the file into an appropriate layout, prints out the nine fields on the first record, closes the file and ends – truly exciting stuff.
There are a few concerns. For one, what about the rest of the records in the file? Second, it might be nice to have some labels for the fields on the report so we know what each is and it may be better for the environment to print the fields across the page rather than down it. While we are at it, what about a title for the report? Why doesn’t the account balance print with a decimal point and without those leading zeroes? Lastly, why do the names begin in lower case letters rather than upper case and what would happen if the account file had no records or didn’t exist at all? These are all valid questions, which need resolving.
Let us begin with the question about upper case in the names. The reason they are lower case is because someone entered them that way. We can resolve that in one of two ways by either reminding the data entry people to appropriately use capital letters in these situations or we could change our data entry program to make sure that these first characters are upper case on the file no matter what is entered. Needless to say it is important to enter correct data otherwise our file won’t have much validity. You’ve heard the expression, “If you put junk in, that’s what will eventually come out.”
Before continuing, let me clear up one point relative to upper and lower case. You may remember that I said we needed only lower case before. And yet how do we account for the first letter of the name without capital letters? The restriction on using only lower case applies only to our computer program, not to the data itself. We can certainly get along well with that limitation. However, we probably need to allow both upper and lower case in the data that will be on our file.
We could print a label before the account number by the statement:
print “account number: ” account-number
and the output would then be
account number: 391023123
which is an improvement over what we had before. The double quote enables us to print literals or strings of values that can be helpful in reports. It may be hard to see, but note that there is a space after the colon, which keeps the label and the actual account number from running together.
This won’t put all the fields on one line but we could do it by two print statements. The first would print all the field labels and the second would print the actual field values. Since a line on our report has space for about 130 characters and our record layout is 99 characters, we should have enough room on one line to print all the fields and have room for spaces between the fields as separators. This we can do by the following print lines:
print “account # last name first name mi street address ”
“city state zip balance”
print account-number “ ” last-name “ ” first-name “ ” middle-initial “ ” street-address
“ ” city “ ” state “ ” zip-code “ ” (balance, mask($$$$,$$9.99))
The output would now be:
account # last name first name mi street address city st zip balance
391023123 Smith Chris T 396 Main Street Buffalo NY 14225 $16.20
Note that the line on this page does not have 132 characters so what you see above is not exactly what you would see on the actual report. The word balance and the value of it would all be on the same line with the other data and there would be more spacing between the fields. Also notice on this page that the headings for each field with the appropriate value don’t exactly line up. This is due to limitations in the word processing software that I am using, which I can’t do much about. Nobody said computers are perfect. If the page you are reading does not have this discrepancy, it means that the publisher took care of the problem. In any case, I think you get the idea.
There would be three spaces between the fields and more depending on the values, specifically the name fields, which allow for more than the number of characters that each name actually has. Thus there will be exactly four spaces between the # sign and the label last and exactly three spaces between the last digit of the account number and the first letter of the name, Smith. Note that we have our upper case designation for the names, which means someone entered them correctly on the file.
Though our first print statement takes up two lines in our program, it will all print on one line of our report. The same is true of the second print statement. The reason that we have two lines each is because they physically don’t fit on one line. If we had put the keyword
before the literal city, then the labels of the fields would have printed on two lines which is not what we want. On the second statement which print the values of all the fields, we have a specific literal,
“ ”
printing after each field to separate the fields. This string consists of exactly three spaces. I mentioned the keywords,
character,
integer,
signed
and
decimal,
before, so you have a good idea what they stand for. In summery, the first can be just about anything, any letter of the alphabet, a number or special character.
integer
is any single digit, positive number, including zero.
decimal
allows us to talk about the numbers between whole numbers, such as 3.23, and
signed
expands our system so that we have negative as well as positive numbers.
The line handling our balance needs some explanation and it ends in
(balance, mask($$$$,$$9.99)).
This will give us the account balance in a way that we’d like to view it. The keyword
mask
is used to reformat the balance so that it will have the appropriate dollar sign, commas if the amount is a thousand dollars or more as well as the decimal point in all cases. The dollar sign used the way it is here allows it to float to just left of the significant digit in the amount. Recall that the record has
00001620
for the balance, so with the mask, the leading zeroes on the left are not printed and the dollar sign winds up just to the left of 16.20. The
9
in the mask forces the number to print, even if it is a zero. Hence a balance of a quarter would print as
$0.25
using this mask. The decimal amount will always print with this mask. Note also that we need two right parentheses to end the statement in order to balance those two parentheses on the left and the mask is enclosed within one set of parentheses. The outer parentheses are needed to assure that the mask goes with the variable,
balance.
Using this same mask, an amount of three thousand dollars and ten cents would print as $3,000.10. We could choose to not print the dollar sign or leave out the comma and this we could do with a change to the mask, using
mask(999999.99).
Our values would then be
000016.20
and
003000.10
respectively. To suppress the leading zeroes we could change it to
mask(zzzzzz.99)
and now we would get
16.20
and
3000.10
for our formatted amounts. The character z here just suppresses the leading zeroes.
To create a main title for our report we could simply add another print statement. It might be
print “ Account balance report”
and note the few spaces to the left of the word Account we used to guarantee that the heading is centered on the page. We shall talk more about headings in another discussion but now we must accommodate those other records on the file that we didn’t list on the report and the possibility of an empty file or none at all.
We do this by introducing more keywords,
status,
if,
go to
and
end-if.
We do have three possibilities here, that is we could have a normal account file or an empty one or none at all so the keyword
status
will enable us to see which of the three comes into play in our program. The last three keywords will give us the ability to make decisions and even branch if necessary, which we will need to do in many cases. The main logic of our program (the code after the structure and its definition) now is:
define acct-status status acctfile
print “ Account Balance Report”
print “account # last name first name mi street address ”
“city state zip balance”
account-number = 9
read-file: readnext acctfile
if acct-status = 0
print account-number “ ” last-name “ ” first-name “ ” middle-initial
“ ” street-address “ ” city “ ” state “ ” zip-code “ ”
(balance, mask($$$$,$$9.99))
go to read-file
end-if
if acct-status not = 9
print “the account file is not integral – program ending”
end-if
end-program: end
The first line
define acct-status status acctfile
defines a two position numeric field for
acct-status
which refers only to the file
acctfile.
The status – which we don’t define – can be anything from 0 to 99. This is done by the keyword
status,
which is always a two-digit number, or
integer(2),
that we will use to verify that any processing of a file has no problems, whether it is a read, write or delete. Here
acct-status
will be used to see if we have a successful read. A value of 0 will indicate that the read was error free. In fact we shall see later that other accesses to the file such as a write will also result in 0, provided they are successful. If we read the file and there are no more records left, the record status will be 9, indicating we have reached the end of the file. Any other value that results means that the file has a problem and we can’t continue in our program.
Let’s look at the lines
account-number = 9
read-file: readnext acctfile.
The first is an assign statement, where the variable on the left is given the value 9. The smallest account number is 10, so the
readnext
verb will try to read a record with an account number of 9, but since it can’t find it, it will read the next record. In the second line, the first part
read-file
is a label, which is used since we need to get back here over and over. We could have called it “xyz” but the name we assigned is much more meaningful. Labels are followed by a colon.
program-name
was also followed by a colon, but since it is a keyword, it is in bold.
The next six lines work hand in hand.
if acct-status = 0
print account-number “ ” last-name “ ” first-name “ ” middle-initial
“ ” street-address “ ” city “ ” state “ ” zip-code “ ”
(balance, mask($$$$,$$9.99))
go to read-file
end-if
The keyword
if
gives us the ability to do something depending on a comparison. In this case we are looking at the field
acct-status
to see if it has a value of 0. This means that the read of the file was successful. If it is, the new line or two indicates what action is to be taken. In this case we have the fields we need to print a single line of our report. We print that line and then proceed to do another read. This is accomplished because of the next keyword,
go to,
which allows us to branch to the label
read-file.
We can now try another read and proceed as before. The keyword
end-if
is used to indicate that our if statement is complete.
The next three lines
if acct-status not = 9
print “the account file is not integral – program ending”
end-if
interrogate or check the value of the field
acct-status
and if it is not equal to 9, there is a problem with the read of the file. In this case we cannot proceed so we print out an error message. The
end-if
again means that this particular if statement is done. You might say that we should end the program and that’s exactly what will happen since that’s the last line of the program. If the
acct-status
is 9, indicating the end of the file, we will wind up in the same place – exactly what we want. Note that if the
acct-status
is 0, we won’t get to this point since we will have branched back to the label
read-file.