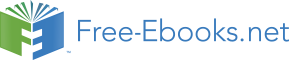

18. Deleting accounts
This next program is a relatively easy one. It’s time for the program to delete records on the account file. We could incorporate this function into the inquiry / update program but instead we will create a new program. The reason has to do with the fact that we probably only want certain people to have the ability to delete records. Another advantage is that the process will be somewhat easier if deletions are separate. Once we have this program done, we can copy it and modify it and use the result for deleting records on the zip code file. There is no sense writing a separate program when we have most of the logic in place.
The program will allow input of an account number and our program will delete the record, provided the balance is zero. It will then proceed to add the account number to the next number file. As I mentioned earlier, we may not want to use the account number over again – at least for a while – so we would just delete the account record. Adding the deleted record to the other file is just being done for the exercise. A report will be produced to list all deleted accounts. As before, in case of a serious file access, an error message will be printed on bottom of the report and listed on the screen.
Our program will be the following:
program-name: acctdel
define main-heading structure
print-month character(2)
field character value “/”
print-day character(2)
field character value “/”
print-year character(43)
field character(73) value “Account number deletion report”
field character(5) value “Page”
page-number integer(3)
define sub-heading structure
field character(54) value “account # last name first name”
field character(57) value “mi street address city state”
field character(19) value “zip balance”
define print-line structure
print-account-number integer(9)
field character(4) value spaces
print-last-name character(22)
print-first-name character(19)
print-middle-initial character(5)
print-street-address character(27)
print-city character(19)
print-state character(6)
print-zip-code integer(5)
field character(2) value spaces
print-balance signed decimal(6.2) mask($$$$,$$9.99-)
define total-line structure
field character(43) value spaces
field character(42) value “The number of account records deleted was”
print-count integer(3) mask(zz9)
define file zipfile record zip-code-record status zip-status key zip structure
zip integer(5)
city character(15)
state character(2)
define file acctfile record account-record status acct-status key account-number structure
account-number integer(9)
last-name character(18)
first-name character(15)
middle-initial character
street-address character(25)
field character(17)
zip-code integer(5)
balance signed decimal(6.2)
define file numbfile record next-number-record status numb-status key next-number structure
next-number integer(9)
indicator character
define error-msg character(60) value spaces
define work-date character(8)
define record-counter integer(5) value 0
define page-counter integer(3) value 0
define line-counter integer(2) value 54
work-date = date
print-month = work-date(5:2)
print-day = work-date(7:2)
print-year = work-date(3:2)
screen erase
screen(1,30) “account number delete”
screen(4,20) “account number:”
screen(22,20) “enter 0 for the account number to exit”
input-number: input(4,36) account-number
screen(24,1) erase
if account-number = 0
go to end-program
end-if
read acctfile update
if acct-status = 0
if balance = 0
perform process-delete
else
screen(24,20) “balance is not 0 - not deleted”
end-if
else
if acct-status = 5
screen(24,20) “account number “account-number “ is not on the file”
else
error-msg = “account file problem; program ending – press enter”
go to end-program
end-if
end-if
go to input-number
process-delete: delete acctfile
if acct-status = 3
screen(24,20) “that record is unavailable”
else
if acct-status > 0
error-msg = “problem updating file; program ending – press enter”
go to end-program
else
perform remaining-process
end-if
end-if
remaining-process: print-account-number = account-number
print-last-name = last-name
print-first-name = first-name
print-middle-initial = middle-initial
print-street-address = street-address
perform get-city-state
print-city = city
print-state = state
print-zip-code = zip-code
print-balance = balance
line-counter = line-counter + 1
if line-counter > 54
perform print-headings
end-if
print print-line
indicator = “d”
next-number = account-number
write numbfile
if numb-status > 0
screen(24,20) account-number “ was not added to the next number file”
end-if
record-counter = record-counter + 1
get-city-state: city = spaces
state = spaces
zip = zip-code
read zipfile
if zip-status = 5
screen(24,20) “zip code “ zip “ is not on the file.”
else
if zip-status > 0
error-msg = “zip file problem; program ending – press enter”
go to end-program
end-if
end-if
print-headings: page-counter = page-counter + 1
page-number = page-counter
line-counter = 5
print page main-heading
print skip(2) sub-heading
print skip
end-program: print-count = record-counter
print skip(2) total-line
print skip error-msg
screen(24,20) error-msg input
end
You may be asking why the program is so long if this is a simple process. If we had displayed data on the screen, it would have been even longer. The task is not complicated, but achieving it involves a great deal of logic. We’re producing a report of what’s happening on paper and access to the files is rather complex. A successful delete means we read the account file, read the zip code file for the city and state, deleted a record from it as long as the balance is 0 and added a record to the next number file. This could be simplified somewhat if we changed the report and only printed the account number, last name and first name, as well as not update the next number file. We chose to print out all the information on the file to help the users.
Note that for the most part, we used lines of code from other programs, such as the update program and the report program. Again, why repeat what has already been done. The main logic is to get an account number to delete and read the account file to make sure that it’s there, but also that the balance on the record is zero. It’s probably