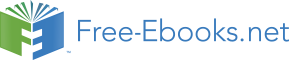

This allows you to create vector-based graphics, and looks like :
To display the results (once saved), you could use the following routine (which ignores special nodes) :
The Line drawing routine was originally written by Gernot Frisch
// --------------------------------- //
// Project: TestVector
// Start: Friday, July 23, 2010
// IDE Version: 8.036
TYPE tCoord
x
y
ENDTYPE
TYPE tGroup
pos[] AS tCoord
ENDTYPE
TYPE tObject
author$
objectName$
handleX%;handleY%
groups[] AS tGroup
ENDTYPE
TYPE TVector
vectorObject AS tObject
SECTION_OBJECT$ = "OBJECT"
KEY_AUTHOR$ = "AUTHOR"
KEY_NAME$ = "NAME"
KEY_HANDLE$ = "HANDLE"
KEY_INUSE$ = "INUSE"
KEY_DATA$ = "DATA"
FUNCTION TVector_Initialise%:
DIM self.vectorObject.groups[0]
ENDFUNCTION
FUNCTION TVector_Load%:fileName$
LOCAL vector AS tCoord
LOCAL group AS tGroup
LOCAL temp$,loop%,error$
LOCAL array$[],dataArray$[]
IF DOESFILEEXIST(fileName$)
TRY
INIOPEN fileName$
self.vectorObject.author$=INIGET$(self.SECTION_OBJECT$,self.KEY_AUTHOR$,"")
self.vectorObject.objectName$=INIGET$(self.SECTION_OBJECT$,self.KEY_NAME$,"")
DIM array$[0]
DIM self.vectorObject.groups[0]
// Get the handle positions - for information only
IF SPLITSTR(INIGET$(self.SECTION_OBJECT$,self.KEY_HANDLE$,"0,0"),array$[],",")=2
self.vectorObject.handleX%=INTEGER(array$[0])
self.vectorObject.handleY%=INTEGER(array$[1])
ELSE
// Ignore anything else and carry on
ENDIF
// Now we get a list of data sections that are in use
DIM array$[0]
IF SPLITSTR(INIGET$(self.SECTION_OBJECT$,self.KEY_INUSE$,""),array$[],",")>0
FOREACH temp$ IN array$[]
DIM dataArray$[0]
DIM group.pos[0]
IF SPLITSTR(INIGET$(self.SECTION_OBJECT$,self.KEY_DATA$+temp$,""),dataArray$[],",")>0
FOR loop%=0 TO BOUNDS(dataArray$[],0)-2 STEP 2
vector.x=INTEGER(dataArray$[loop%])*1.0
vector.y=INTEGER(dataArray$[loop%+1])*1.0
DIMPUSH group.pos[],vector
NEXT
// Push group into array
DIMPUSH self.vectorObject.groups[],group
ELSE
THROW "No data in key : "+self.KEY_DATA$+temp$
ENDIF
NEXT
ELSE
THROW "No data to be found!"
ENDIF
INIOPEN ""
RETURN TRUE
CATCH error$
DEBUG error$+"\n"
STDERR error$+"\n"
INIOPEN ""
RETURN FALSE
FINALLY
ELSE
RETURN FALSE
ENDIF
ENDFUNCTION
FUNCTION TVector_Display%:x,y,angle,scale,colour%,alpha,usePolyVector%=FALSE,useSprite%=-1
LOCAL group AS tGroup
LOCAL coords%,size%,loop%
LOCAL n%,cv,sv
cv=COS(angle)
sv=SIN(angle)
ALPHAMODE alpha
FOREACH group IN self.vectorObject.groups[]
size%=BOUNDS(group.pos[],0)
FOR loop%=0 TO size%-1
n%=self.TVector_GetNextIndex(loop%,size%)
IF usePolyVector%=TRUE
self.Line(x+((cv*group.pos[loop%].x*scale)-(sv*group.pos[loop%].y*scale)),y+((cv*group.pos[loop%].y*scale)+(sv*group.pos[loop%].x*scale)), _
x+((cv*group.pos[n%].x*scale)-(sv*group.pos[n%].y*scale)),y+((cv*group.pos[n%].y*scale)+(sv*group.pos[n%].x*scale)),colour%)
ELSE
DRAWLINE x+((cv*group.pos[loop%].x*scale)-(sv*group.pos[loop%].y*scale)),y+((cv*group.pos[loop%].y*scale)+(sv*group.pos[loop%].x*scale)), _
x+((cv*group.pos[n%].x*scale)-(sv*group.pos[n%].y*scale)),y+((cv*group.pos[n%].y*scale)+(sv*group.pos[n%].x*scale)),colour%
ENDIF
NEXT
NEXT
ENDFUNCTION
FUNCTION TVector_GetNextIndex%:current%,size%
INC current%
IF current%>=size% THEN current%=0
RETURN current
ENDFUNCTION
FUNCTION Line: x1, y1, x2, y2, col
LOCAL c,s,p, w, dx, dy, ddx, ddy, ux, uy, lg
//line width
w = 16
// direction of line
ddx = x2-x1
ddy = y2-y1
lg = SQR(ddx*ddx+ddy*ddy)
IF lg<0.5 THEN RETURN
// short caps
lg=lg*2
// dir vector
dx=ddx*w/lg
dy=ddy*w/lg
// up vector
ux=dy
uy=-dx
// cap1
STARTPOLY 0
POLYVECTOR x1+ux-dx, y1+uy-dy, 0.5, 0.5,col
POLYVECTOR x1-ux-dx, y1-uy-dy, 0.5,63.5,col
POLYVECTOR x1-ux, y1-uy, 31.5,63.5,col
POLYVECTOR x1+ux, y1+uy, 31.5, 0.5,col
ENDPOLY
// center
STARTPOLY 0
POLYVECTOR x1+ux, y1+uy, 31.5, 0.5,col
POLYVECTOR x1-ux, y1-uy, 31.5, 63.5,col
POLYVECTOR x2-ux, y2-uy, 31.5, 63.5,col
POLYVECTOR x2+ux, y2+uy, 31.5, 0.5,col
ENDPOLY
// cap2
STARTPOLY 0
POLYVECTOR x2+ux, y2+uy, 31.5, 0.5,col
POLYVECTOR x2-ux, y2-uy, 31.5, 63.5,col
POLYVECTOR x2-ux+dx, y2-uy+dy, 63.5, 63.5,col
POLYVECTOR x2+ux+dx, y2+uy+dy, 63.5, 0.5,col
ENDPOLY
ENDFUNCTION
ENDTYPE
An example would be :