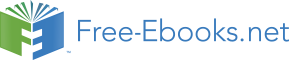

Object Oriented Programming is a design and programming methodology, using which we design and develop a software system which resembles a real life model. When we say we are developing an software application for inventory management using OOPs, what we mean is that we are using a real life model to design and develop the software application.
A real life model is what we see and live among. The cars we see and the pens we use and tables we write on, are called objects in Object Oriented terminology. Every object in a real life situation or model can be said to belong to a Class. Cars belong to the Class automobile.
The real life model can be extended. Suppose, we say that automobiles are classified into four wheelers and two wheelers, then car becomes the instance of the four wheeler sub class and a jeep another instance of the four wheeler sub class. So, the Object Oriented methodology is a classifying system in order to better understand a system. The benefit of OOPs is that we can understand and design a software application in a logical manner.
In other words, in OOPs, we use the concept of Classes and Objects, and if required Sub Classes, to define a system. If we define the Class automobile as a class consisting of things which transport people, then the next step is to define the characteristics. The characteristics could be, the four wheels, and the seating, and the steering wheel. In OOPs terminology we call these as attributes or properties. Methods and attributes together are known as the interface of the class.
In a real life model we say the automobile class has the behavior of transporting people. In OOPs terminology, behaviour is referred to as service or method.In the domain (that is the Inventory domain) which we have considered, two of the classes and their objects are:
Class Object
a. ItemClass Pens, Pencils
b. TradeClass Purchase Invoice, Sales Invoice etc.
The success of the Object Oriented approach lies in the fact that we can commence the design and analysis of the application by using real life situations and approach.
Basic elements of an Object Oriented System
The four basic elements of an object oriented system are:
a. Abstraction. b. Encapsulation. c. Inheritance. d. Polymorphism.
These are key requirements and are often used as evaluation criteria when defining a system.Abstraction
Abstraction is a technique that we all use to manage the complexity of the information. Using abstraction we can focus on the objects of an application and not on the implementation. This lets us think about what needs to be done and not how the computer will do it.
EncapsulationEncapsulation is the ability to contain and hide the information about an object such as internal data structures and code. Encapsulation isolates the internal complexity of an object’s operation from the rest of the application.
PolymorphismTwo or more classes having the same behavior or methods that are named the same and have the same basic purpose but different implementations, is polymorphism. For example, ItemClass has Save behavior or method and TradeClass also has Save behavior. But, the implementation of that behavior is completely different in each case. When the Save button is hit, the object knows the class it belongs to and automatically calls the appropriate Class save method.
OOPS Features in Visual BasicThere are many features in VB which support Object Oriented Programming. Some of them are detailed below:
a. Defining a Class.
b. Property Procedures. c. Polymorphic Methods. d. Generating Events. e. Viewing a Class.
f. Creating Objects.
g. Using Forms as Objects.
A Class is defined using a feature called Class Module. The Class Module can be added into the project using the Add Class Module option in the Project Menu. Using the Class Module, properties and property procedures can be defined. Behaviors or methods of the class can be implemented using sub and function procedures.
b. Property ProceduresProperty procedures provide the public interface to the private properties in a class. With property procedures we can get and set the value of object property.
c. Polymorphic Methods
Methods are the sub and function procedures in a class that provide the implementation of the object behaviors. Same names can be used for similar properties and methods in different classes which is polymorphism.
d. Generating EventsIn VB events can be defined in a Class. Events are the means to communicate between components.
e. Viewing a Class The object browser provides a list of all classes within the current project and the properties, methods and events for each class. You can use the Object Browser to quickly review the interface of a class. The Object Browser also provides a convenient online reference information.
f. Creating ObjectsObjects can be created from the classes. The reference to the created object is stored in an object variable. The object variable is then used to set or retrieve the object’s properties and invoke object’s methods.
g. Using Forms as ObjectsIn VB, forms are treated as classes. Many of the features provided for classes are available in forms as well. You can add public properties and methods to a form class defined in a form module. A form object is created by VB when the form is loaded.
The above seven points explain the OOPs features of VB. Implementation of these features is explained in the coming topics.