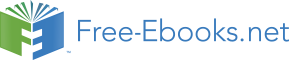

public static GreetingFactory getGreetingFactory(){
return new GreetingFactory();
}
Next we have a Greeting interface that defines the contract of a Greeting object--that is, what type of behavior it supports. The Greeting interface looks like this:
package xptoolkit.model;
public interface Greeting extends java.io.Serializable{ public String getGreeting();
}
public String getGreeting(){
return "Hello World!";
}
}
GreetingBean returns the message "Hello World!" . To create a Greeting instance, you use GreetingFactory. The default implementation of GreetingFactory gets the implementation class from a property and instantiates an instance of that class with the Class.forName().newInstance() method. It casts the created instance to the Greeting interface.
These two lines of code create the Greeting instance from GreetingFactory's getGreeting() method: String clazz = System.getProperty("Greeting.class",Thus, any class that implements the Greeting interface can substitute for the Greeting.class system property. Then, when the class is instantiated with the factory's getGreeting() method, the application uses the new implementation of the Greeting interface.
In the book Java Tools for Extreme Programming (also published by Wiley), we use this technique to transparently add support for EJBs to the Web application. In that book we also create an Ant script that can deploy the same Web application to use either enterprise beans or another bean implementation just by setting an Ant property (and some Ant filter magic). We also map the Greeting interface with the use bean action of a JSP when we implement the Model 2 using servlets and JSP.