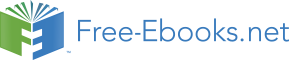

<project name="model" default="all" >
The all target is executed by default, unless we specify another target as a command-line argument of Ant. The all target depends on the clean and package targets. The clean target depends on the init target; thus, the init target is executed first.
Next, the init target is executed. The init target is defined in build.xml as follows: <target name="init"<property name="local_outdir" value="${outdir}/model" /> <property name="build" value="${local_outdir}/classes" /> <property name="lib" value="${outdir}/lib" />
<property name="model_jar" value="${lib}/greetmodel.jar" />
The init target defines several properties that refer to directories and files needed to compile and deploy the model project. Let's discuss the meaning of these properties because all the other build files for this example use the same or similar properties. The init target defines the following properties:
local_outdir --Defines the output directory of all the model project's intermediate files (Java class files). build--Defines the output directory of the Java class files.
lib--Defines the directory that holds the common code libraries (JAR files) used for the whole Model 2 Hello World example application.model_jar--Defines the output JAR file for this project.
Note
As a general rule, if you use the same literal twice, you should go ahead and define it in the init target. You don't know how many times we've shot ourselves in the foot by not following this rule. This build file is fairly simple, but the later ones are more complex. Please learn from our mistakes (and missing toes).
Now that all the clean target's dependencies have executed, the clean target can execute. The clean target deletes the intermediate files created by the compile and the output common JAR file, which is the output of this project. Here is the code for the clean target:
<target name="clean" depends="init"Remember that the all target depends on the clean and package targets. The clean branch and all its dependencies have now executed, so it is time to execute the package target branch (a branch is a target and all its dependencies). The package target depends on the compile target, the compile target depends on the prepare target, and the prepare target depends on the init target, which has already been executed.
NoteDuring development you would probably not use the all target; instead, you would use the compile or package target. However, as a general rule, the default target is preferred as the most complete target with the fewest side effects. You can always override this during development by passing the desired target on the command line when invoking Ant.
Thus, the next target that executes is prepare, because all its dependencies have already executed. The prepare target creates the build output directory, which ensures that the lib directory is created. The prepare target is defined as follows:
<target name="prepare" depends="init"The next target in the package target branch that executes is the compile target--another dependency of the package target. The compile target compiles the code in the src directory to the build directory, which was defined by the build property in the init target. The compile target is defined in this way:
<target name="compile" depends="prepare"Now that all the target dependencies of the package target have been executed, we can run the package target. The package target packages the Java classes created in the compile target into a JAR file in the common lib directory. The package target is defined as follows:
<target name="package" depends="compile"