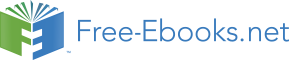

public void init(){
URL uGreeting;
String sGreeting="Bye Bye";
try{
uGreeting = new URL(
getDocumentBase(), "HelloWorldServlet");
sGreeting = getGreeting(uGreeting);
}
catch(Exception e){
getAppletContext()
.showStatus("Unable to communicate with server.");
e.printStackTrace();
}
text.setText(sGreeting);
The init() method gets the document base URL (the URL from which the applet's page was loaded) from the applet context. Then, the init() method uses the document base and the URI identifying HelloWorldServlet to create a URL that has the output of HelloWorldServlet:
uGreeting = new URL( getDocumentBase(), "HelloWorldServlet");The init() method uses a helper method called getGreeting() to parse the output of HelloWorldServlet. It then displays the greeting in the applet's JLabel (text.setText(sGreeting);). The helper method looks like this:
private String getGreeting(URL uGreeting)throws Exception{ String line;
int endTagIndex;
BufferedReader reader=null;
if (line.startsWith("<h1>")){
getAppletContext().showStatus("Parsing message."); endTagIndex=line.indexOf("</h1>");
line=line.substring(4,endTagIndex);
break;
Basically, the method gets the output stream from the URL (uGreeting.openStream()) and goes through the stream line by line looking for a line that begins with <h1>. Then, it pulls the text out of the <h1> tag.
The output of HelloServlet looks like this:
<html>
<head>
<title>Hello World</title>
</head>
<body>
<h1>Hello World!</h1>
</body>
package xptoolkit.applet;
import javax.swing.JApplet;
import javax.swing.JLabel;
import java.awt.Font;
import java.awt.BorderLayout;
import java.applet.AppletContext;
import java.net.URL;
import java.io.InputStreamReader;
import java.io.BufferedReader;
JLabel text; public HelloWorldApplet() {
this.getContentPane().setLayout(new BorderLayout()); text = new JLabel("Bye Bye");
text.setAlignmentX(JLabel.CENTER_ALIGNMENT); text.setAlignmentY(JLabel.CENTER_ALIGNMENT); Font f = new Font("Arial", Font.BOLD, 20); text.setFont(f);
getContentPane().add(text,BorderLayout.CENTER);
public void init(){
URL uGreeting;
String sGreeting="Bye Bye";
try{ uGreeting = new URL(
this.getDocumentBase(), "HelloWorldServlet");
sGreeting = getGreeting(uGreeting); }
catch(Exception e){
getAppletContext()
.showStatus("Unable to communicate with server.");
e.printStackTrace();
}
text.setText(sGreeting);
private String getGreeting(URL uGreeting)throws Exception{ String line;
int endTagIndex;
BufferedReader reader=null;
uGreeting.openStream()));
while((line=reader.readLine())!=null){
System.out.println(line);
if (line.startsWith("<h1>")){
getAppletContext().showStatus("Parsing message."); endTagIndex=line.indexOf("</h1>");
line=line.substring(4,endTagIndex);
break;
}
}
}
finally{
if (reader!=null)reader.close(); }
return line;