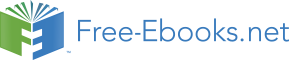

String clazz = config.getInitParameter("Greeting.class") ; It uses the value of the initialization parameter "Greeting.class" to set the System property "Greeting.class", as follows:
System.setProperty("Greeting.class",clazz);
You will recall from earlier that GreetingFactory uses the system property "Greeting.class" to decide which implementation of the Greeting interface to load. Now let's get to the real action: the doGet() and doPost() methods.
When the doGet() or doPost() method of HelloWorldServlet is called, the servlet uses GreetingFactory to create a greeting:Greeting greet = (Greeting)
session.setAttribute("greeting", greet);
Finally, HelloWorldServlet forwards processing of this request to the JSP file HelloWorld.jsp by getting the request dispatcher for HelloWorldServlet from the servlet's context:
/* Redirect to the HelloWorld.jsp */
context = getServletContext();
dispatch = context.getRequestDispatcher("/HelloWorldJSP"); dispatch.forward(request, response);
You may notice that the context.getRequestDispatcher call looks a little strange. This is because HelloWorld.jsp is mapped to /HelloWorldJSP in the deployment descriptor for the servlet. Next, let's examine HelloWorld.jsp.