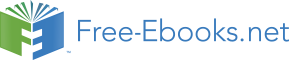

<target name="package" depends="compile">
<war warfile="${dist}/hello.war" webxml="${meta}/web.xml"> <!-
Include the html and jsp files.
Put the classes from the build into the classes directory
of the war.
/-->
<fileset dir="./HTML" />
<fileset dir="./JSP" />
<classes dir="${build}" />
<!-- Include all of the jar files except the ejbeans and applet.
/-->
<lib dir="${lib}" />
</war>
As you can see, this package target is much larger than the other two we've discussed (model and application). For now we'll offer a detailed discussion of the second and third lines of code: <mkdir dir="${meta}" />
These lines do some processing on the web.xml deployment descriptor file and put the file in the directory defined by the ${meta} directory (note that the meta property is set in the init target). Next, the package target calls the war task:
<war warfile="${dist}/hello.war" webxml="${meta}/web.xml"> <fileset dir="./HTML" />
<fileset dir="./JSP" />
<classes dir="${build}" />
<fileset dir="${lib}" includes="helloapplet.jar" /> <lib dir="${lib}" />
The WAR file hello.war is put in the distribution directory (dist), which is specified by the war task's warfile attribute (warfile="${dist}/hello.war"). The dist directory is another common directory that is used by the main project build file later to build an enterprise archive (EAR) file; the dist property is defined in the init target. The webxml attribute of the war task defines the deployment descriptor to use; it's the one we processed at the beginning of the package target. The web.xml file is put in the WAR file's WEB-INF/ directory.
In addition, the war task body specifies three file sets. One file set includes the helloapplet.jar file (which we discuss in the section "HelloWorld.jsp Applet Delivery" later in this chapter) and all the files in the HTML and JSP directories. The war task body also specifies where to locate the classes using
<classes dir="${build}" />This command puts the classes in the WEB-INF/classes directory.
The Web application project build file defines a slightly more complex compile target: <target name="compile" depends="prepare"
<fileset dir="${lib}">
<include name="**/*.jar"/>
</fileset>
<fileset dir="${build_lib}"> <include name="**/*.jar"/>
</fileset>
Notice that this compile target defines two file sets. One file set (<fileset dir="${build_lib}">) is used to include the classes needed for servlets (such as import javax.servlet.*). The other file set (<fileset dir="${lib}">) is used to include the Greeting interfaces and the GreetingFactory class. The only real difference from the application compile target is the inclusion of the JAR file for servlets. The build_lib property is defined in the Web application project's init target, as shown here:
<property name="build_lib" value="./../lib" />The good thing about this approach is that if we need additional JAR files, we can put them in build_lib. The second file set (<fileset dir="${build_lib}">) grabs all the JAR files in the ./../lib directory.
The Web application project build file adds a few convenience targets geared toward Web applications. The deploy target copies the WAR file that this build file generates to the webapps directory of Tomcat and Resin. (Resin is an easy-to-use Java application server that supports JSPs, EJBs, J2EE container specification, XSL, and so on. We show it here to show that the build script would work with more than one app server since it genretates J2EE compliant war files) Without further ado, here is the deploy target:
<target name="deploy" depends="package">
<copy file="${dist}/hello.war" todir="${deploy_resin}" /> <copy file="${dist}/hello.war" todir="${deploy_tomcat}" />
Both Tomcat and Resin pick up the WAR files automatically, in the interest of doing no harm and cleaning up after ourselves. The Web application project build file adds an extra clean_deploy target that deletes the WAR file it deployed and cleans up the generated directory:
<target name="clean_deploy" >
<delete file="${deploy_resin}/hello.war" />
<delete dir="${deploy_resin}/hello" />
<delete file="${deploy_tomcat}/hello.war" />
<delete dir="${deploy_tomcat}/hello" />
"Great," you say. "But what if the application server I am deploying to is on another server that is halfway around the world?" No problem; you could use the following FTP task:
<ftp server="ftp.texas.austin.building7.eblox.org"
remotedir="/deploy/resin/webapps"
userid="kingJon"
password="killMyLandLord"
depends="yes"
binary="yes"
<fileset dir="${dist}"> <include name="**/*.war"/>
</fileset>
The key lesson here is that Ant is powerful and extensible and if you can think of a whiz-bang task that would be great, there is a good chance it may already exist. Be sure to read the Ant online docs--they are a great reference.