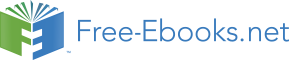

<war warfile="${dist}/hello.war" webxml="${meta}/web.xml"> <fileset dir="./HTML" />
<fileset dir="./JSP" />
<classes dir="${build}" />
<fileset dir="${lib}" includes="helloapplet.jar" /> <lib dir="${lib}" />
The fileset directive
<fileset dir="${lib}" includes="helloapplet.jar" />
tells the war task to include only the helloapplet.jar file from the lib directory. Because this file set is not a classes- or lib-type file set, helloapplet.jar goes to the root of the WAR file. In contrast, the special lib and classes file sets put their files in WEB-INF/lib and WEB-INF/classes, respectively. The end effect is that the browser is able to get the applet.
After we build the applet, we go back and rebuild the Web application and then deploy it. We run Ant in the root of both the projects' home directories (if you get a compile error, be sure you have built the model, because the Web application depends on it). After everything compiles and the appropriate JAR and WAR files are built, we deploy the Web application project by using the deploy target of the Web application build file.
Let's review what we've done so far. We've created a common Java library called model.jar. This model.jar file is used by a Web application and a regular Java application that is a stand-alone executable Java application in an executable JAR file. We've also created an applet that is loaded with a JSP page that has the jsp:plugin action. Once the applet loads into the browser, the applet communicates over HTTP to the Web application's HelloWorldServlet. HelloWorldServlet calls the getGreeting() method on the GreetingFactory, which is contained in the model.jar file.
Note
In the book Java Tools for Extreme Programming, we set up GreetingFactory so that it talks to an enterprise session bean, which in turn talks to an enterprise entity bean. The Web application build file is set up so that after we add one property file, it can talk to either the enterprise beans or to the local implementation in the model library. We achieve this magic by using Ant filters.
After we define the ejb property, the Web application project has the option of deploying/configuring whether the Web application that is deployed uses enterprise beans. Notice that the prepare_meta target, which is a dependency of the prepare target, has two dependencies: prepare_meta_ejb and prepare_meta_noejb, as shown here:
<target name="prepare_meta"<copy todir="${meta}" filtering="true">
<fileset dir="./meta-data"/>
</copy>
</target>
<target name="prepare_meta_ejb" if="ejb">
If the ejb property is set, then the target creates a filter token called Greeting.class. Here, we set the value of Greeting.class to GreetingShadow. Conversely, the prepare_meta_ejb target is executed only if the ejb property is not set, as follows:
<target name="prepare_meta_noejb" unless="ejb">Here, we set GreetingBean as Greeting.class. But how is this used by the application? You may recall that HelloWorldServlet uses the servlet parameter Greeting.class to set the system property Greeting.class (which is used by GreetingFactory to create an instance of Greeting). We put an Ant filter key in the Web application project deployment descriptor, as follows:
<servlet>
<servlet-name>HelloWorldServlet</servlet-name>
<servlet-class>xptoolkit.web.HelloWorldServlet</servlet-class> <init-param>
If we copy this file using the filter command after the filter token Greeting.class has been set, then @Greeting.class@ is replaced with the value of our token Greeting.class, which is set to xptoolkit.model.GreetingShadow in the prepare_meta_ejb target and to xptoolkit.model.GreetingBean in the prepare_meta_noejb target. Notice that the prepare_meta target copies the deployment descriptor with filtering turned on, as follows:
<copy todir="${meta}" filtering="true">
<fileset dir="./meta-data"/>
</copy>