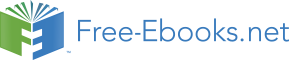

Quite often it is the simple things that we often miss out while programming. That results in errors, difficulty in maintenance of the code that we write, increase in the efforts of finishing modules, delivery slippage, etc. But these can be avoided with some simple tips that follow.
Exceptions
1. Avoid Unhandled Exceptions
2. Catch errors and give proper messages to the user
Variables
3. Follow proper naming conventions
4. Always initialize variables and reset them to their initial or final state
5. Always reset variables if you are using them in loops
6. Avoid long variable names
7. Avoid static variables unless it is really required
Methods
8. Method names should be meaningful and convey what it is trying to do
9. Method names should not be too long
10. Avoid long methods, typically it should be only around 30-40 lines
11.Design methods for reusability, don’t have any dependencies in the method
12. Always think of plug and play methods
Parameters
13. Avoid too many parameters for a method, if so you are missing an object or a structure
14. Avoid more than one return variable, if so you are missing something
Checking
15. Check if NULL
16. Check if empty
17. Always check for array length before processing to avoid index out of bounds exception
18. Always cast properly to avoid invalid cast exception
19. Always check boundary conditions
Memory Management
20. Always dispose objects after use
21. Always have long running methods in separate thread
22. Always give a response UI to the user, it should never say “Not Responding”
General
23. Always follow this principle while programming
Initialize -> Do the work -> Cleanup
24. Always abstract the functionalities
25. Avoid using = instead of == for equality comparison
26. Always check the interfaces for errors (Interactions between two modules)
27. Use a Version Control system which can solve major headaches
28. Always check-in your code very frequently, it can save a lot of time
29. Refactor the code very often till it becomes a habit
30. Use break statements only when needed, use them carefully
31. Database connections should be opened only when needed, and should be closed as long as it is not needed
32. Do not fetch all the records from the database, especially if the records are huge in size. Use paging concepts.
33. Have all environment specific variables in a config file, for eg. Connecting to a database server, Default file path, etc.
34. Follow proper coding standards
35. Always review your own code
36. Always read good programming books