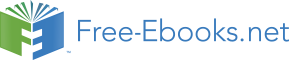

I can almost guarantee that you will be using strings in almost every Python program that you write, so pay attention to the following part. Here's how you use strings in Python:
• Using Single Quotes (') You can specify strings using single quotes such as'Quote me on this' . All white space i.e. spaces and tabs are preserved as-is.
• Using Double Quotes (") Strings in double quotes work exactly the same way as strings in single quotes. An example is "What's your name?"
• Using Triple Quotes (''' or""") You can specify multi-line strings using triple quotes. You can use single quotes and double quotes freely within the triple quotes. An example is
'''This is a multi-line string. This is the first line. This is the second line.
"What's your name?," I asked.
He said "Bond, James Bond."
'''
Suppose, you want to have a string which contains a single quote ( '), how will you specify this string? For example, the string isWhat's your name?. You cannot specify'What's your name?' because Python will be confused as to where the string starts and ends. So, you will have to specify that this single quote does not indicate the end of the string. This can be done with the help of what is called an escape sequence. You specify the single quote as\' - notice the backslash. Now, you can specify the string as'What\'s your name?'.
Another way of specifying this specific string would be "What's your name?" i.e. using double quotes. Similarly, you have to use an escape sequence forusing a double quote itself in a double quoted string. Also, you have to indicate the backslash itself using the escape sequence\\.
What if you wanted to specify a two-line string? One way is to use a triple-quoted string as shown above or you can use an escape sequence for the newline character \n to indicate the start of a new line. An example isThis is the first line\nThis is the second line . Another useful escape sequence to know is the tab \t. There are many more escape sequences but I have mentioned only the most useful ones here.
One thing to note is that in a string, a single backslash at the end of the line indicates that the string is continued in the next line, but no newline is added. For example,"This is the first sentence.\ This is the second sentence."
is equivalent to "This is the first sentence. This is the second sentence."
• Raw Strings
If you need to specify some strings where no special processing such as escape sequences are handled, then what you need is to specify a raw string by prefixingr orR to the string. An example isr"Newlines are indicated by \n".
• Unicode StringsUnicode is a standard way of writing international text. If you want to write text in your native language such as Hindi or Arabic, then you need to have a Unicode-enabled text editor. Similarly, Python allows you to handle Unicode text - all you need to do is prefixu orU. For example,u"This is a Unicode string.".
Remember to use Unicode strings when you are dealing with text files, especially when you know that the file will contain text written in languages other than English.• Strings are immutable
This means that once you have created a string, you cannot change it. Although this might seem like a bad thing, it really isn't. We will see why this is not a limitation in the various programs that we see later on.
• String literal concatenationIf you place two string literals side by side, they are automatically concatenated by Python. For example, 'What\'s' 'your name?' is automatically converted in to "What's your name?".