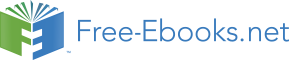

In this program, we take guesses from the user and check if it is the number that we have. We set the variable number to any integer we want, say 23. Then, we take the user's guess using the raw_input() function. Functions are just reusable pieces of programs. We'll read more about them in the next chapter.
We supply a string to the built-in raw_input function which prints it to the screen and waits for input from the user. Once we enter something and press enter, the function returns the input which in the case ofraw_input is a string. We then convert this string to an integer usingint and then store it in the variableguess. Actually, theint is a class but all you need to know right now is that you can use it to convert a string to an integer (assuming the string contains a valid integer in the text).
Next, we compare the guess of the user with the number we have chosen. If they are equal, we print a success message. Notice that we use indentation levels to tell Python which statements belong to which block. This is why indentation is so important in Python. I hope you are sticking to 'one tab per indentation level' rule. Are you?
Notice how theif statement contains a colon at the end - we are indicating to Python that a block of statements follows.Then, we check if the guess is less than the number, and if so, we inform the user to guess a little higher than that. What we have used here is theelif clause which actually combines two relatedif elseif else statements into one combinedif-elif-else statement. This makes the program easier and reduces the amount of indentation required.
Theelif andelse statements must also have a colon at the end of the logical line followed by their corresponding block of statements (with proper indentation, of course)You can have anotherif statement inside the if-block of anif statement and so on - this is called a nestedif statement.
Remember that theelif andelse parts are optional. A minival validif statement is
if True:print 'Yes, it is true'
After Python has finished executing the complete if statement along with the assocatedelif and else clauses, it moves on to the next statement in the block containing theif statement. In this case, it is the main block where execution of the program starts and the next statement is theprint 'Done' statement. After this, Python sees the ends of the program and simply finishes up.
Although this is a very simple program, I have been pointing out a lot of things that you should notice even in this simple program. All these are pretty straightforward (and surprisingly simple for those of you from C/C++ backgrounds) and requires you to become aware of all these initially, but after that, you will become comfortable with it and it'll feel 'natural' to you.