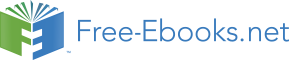

if guess == number:
print 'Congratulations, you guessed it.' running = False # this causes the while loop to stop
elif guess < number:
print 'No, it is a little higher than that.'
else:
else: print 'No, it is a little lower than that.'
$ python while.py
Enter an integer : 50
No, it is a little lower than that. Enter an integer : 22
No, it is a little higher than that. Enter an integer : 23
Congratulations, you guessed it. The while loop is over.
Done
In this program, we are still playing the guessing game, but the advantage is that the user is allowed to keep guessing until he guesses correctly - there is no need to repeatedly execute the program for each guess as we have done previously. This aptly demonstrates the use of thewhile statement.
We move the raw_input andif statements to inside thewhile loop and set the variablerunning toTrue before the while loop. First, we check if the variablerunning isTrue and then proceed to execute the corresponding while-block. After this block is executed, the condition is again checked which in this case is therunning variable. If it is true, we execute the while-block again, else we continue to execute the optional else-block and then continue to the next statement.
The else block is executed when thewhile loop condition becomesFalse - this may even be the first time that the condition is checked. If there is anelse clause for awhile loop, it is always executed unless you have awhile loop which loops forever without ever breaking out!
The True andFalse are called Boolean types and you can consider them to be equivalent to the value 1 and0 respecitvely. It's important to use these where the condition or checking is important and not the actual value such as1.
The else-block is actually redundant since you can put those statements in the same block (as thewhile statement) after thewhile statement to get the same effect.