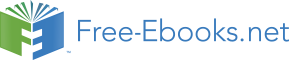

for i in range(1, 5): print i
else:
print 'The for loop is over'
$ python for.py
1
2
3
4
The for loop is over
What we do here is supply it two numbers and range returns a sequence of numbers starting from the first number and up to the second number. For example,range(1,5) gives the sequence[1, 2, 3, 4]. By default,range takes a step count of 1. If we supply a third number torange, then that becomes the step count. For example,range(1,5,2) gives[1,3]. Remember that the range extends up to the second number i.e. it does not include the second number.
The for loop then iterates over this range for i in range(1,5) is equivalent tofor i in [1, 2, 3, 4] which is like assigning each number (or object) in the sequence to i, one at a time, and then executing the block of statements for each value ofi. In this case, we just print the value in the block of statements.
Remember that theelse part is optional. When included, it is always executed once after thefor loop is over unless a break statement is encountered.Remember that the for..in loop works for any sequence. Here, we have a list of numbers generated by the built-inrange function, but in general we can use any kind of sequence of any kind of objects! We will explore this idea in detail in later chapters.
The Python for loop is radically different from the C/C++for loop. C# programmers will note that thefor loop in Python is similar to theforeach loop in C#. Java programmers will note that the same is similar tofor (int i : IntArray) in Java 1.5 .
In C/C++, if you want to write for (int i = 0; i < 5; i++), then in Python you write justfor i in range(0,5). As you can see, thefor loop is simpler, more expressive and less error prone in Python.