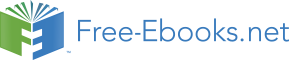

If you want to assign a value to a name defined outside the function, then you have to tell Python that the name is not local, but it is global. We do this using theglobal statement. It is impossible to assign a value to a variable defined outside a function without theglobal statement.
You can use the values of such variables defined outside the function (assuming there is no variable with the same name within the function). However, this is not encouraged and should be avoided since it becomes unclear to the reader of the program as to where that variable's definition is. Using theglobal statement makes it amply clear that the variable is defined in an outer block.
print 'x is', x
x = 2
print 'Changed global x to', x
x = 50
func()
print 'Value of x is', x
$ python func_global.py x is 50
Changed global x to 2 Value of x is 2