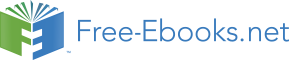

7 Numerical precision and more about variables
7.1 Entering numerical values
We have already seen how to enter simple numerical values into a program. What happens if the values need to be expressed in the form a × 10 b? F95 provides a way of doing it. For example the numbers on the left of the following table are entered in the form indicated on the right:
7.2 Numerical Accuracy
Variables in any programming language use a fixed amount of computer memory to store values. The real variables we have discussed so far have surprisingly little accuracy (about 6-7 significant figures at best). We will often require more accuracy than this, especially for any problem involving a complicated numerical routine (e.g. a NAG routine). In that case you will need to use what is called a “double precision variable”. Such variables have twice the accuracy of normal reals.
F95 in fact provides a very flexible system for specifying the accuracy of all types of variables. To do this it uses the idea of kind; this really means the accuracy of a particular type of variable. You do not need to go into this in much detail, we will give a recipe for obtaining high precision, “double precision”, variables.
Each variable type may have a number of different kinds which differ in the precision with which they hold a number for real and complex numbers, or in the largest number they can hold for integer variables. When declaring variables of a given type you may optionally specify the kind of that variable as we shall see below. A few intrinsic functions are useful:
Here we want to specify variables of high precision and there is an easy way of doing this. The notation for a double precision constant is defined; so that for instance 1.0 is designated 1.0d0 if you wish it to be held to twice the accuracy as ordinary single precision. To define variables as double precision, you first need to establish the appropriate kind parameter and then use it in the declaration of the variable. Here is a useful recipe:
! find the kind of a high precision variable, by finding
! the kind of 1.0d0
integer, parameter :: dp=kind(1.0d0)
! use dp to specify new real variables of high precision
real(kind=dp) :: x,y
! and complex variables
complex(kind=dp) :: z
! and arrays
real(kind=dp), dimension(30) :: table
The integer parameter dp can now also be used to qualify constants in the context of expressions, defining them as double precision, e.g. 0.6_dp