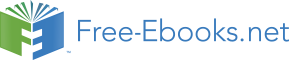

JavaScript
JavaScript is a simple scripting language created for use in web browsers to make web- sites more dynamic. On its own, HTML is capable of putting out static pages. Once you load them the visitor's view doesn't change much until they click a link to go to a new page. Adding JavaScript to your code allows you to change how the document looks completely, from changing text, changing colors, the options available in a drop-down list, and more.
JavaScript is a client-side language, which means all the action occurs on the visitor's side of things. No trips to the web server are required for scripts to work. Usually they work because the page is refreshed, or you have programmed in times or responses to a visitor's actions.
You can easily add a bit of interaction to your website with bits of JavaScript. For the real thing, you will want to study JavaScript, get a book, and do tutorials on it. You will also want to look into JavaScript libraries you can lean on in your website like JQuery, MooT- ools, or Yui. However, you can easily do a few simple things without being an expert and add that little dash to your website that can make an impact.
Let's just look at a few examples of simple javascript you can apply to make your site a bit more dynamic. You can find tons of javascript tutorials and scripts on the Web.
Add A Dynamic Date
<head>
<script type=”text/javascript”>
var myDate = new Date();
var myYear = myDate.getFullYear();
</script>
</head>
<body>
Use somewhere, like in the footer.
©<script type=”text/javascript”>document.write(myYear);</script>
</body>
Add A Protected Email Address
<head>
<script type=”text/javascript”>
function getEmailAddy(displayName, emailName, emailHostName, ext)
return “<a href=’” + “mail” + “to:” + emailName + “@” + emailHostName + “.”
+ ext + “’>” + displayName + “</a>”;
</script>
</head>
<body>
<p><script type=”text/javascript”>document.write( getEmailAddy(“Email
L.J.”, “lbothe01”, “seattlecentral”, “edu”) );</script></p>
</body>
Add a Changing Phrase
<body>
<script type=”text/javascript”> var Quotation=new Array() Quotation[0] = “I want a mocha.”; Quotation[1] = “I want some sleep.”;
Quotation[2] = “I want to be in the sun.”;
var Q = Quotation.length;
var whichQuotation=Math.round(Math.random()*(Q-1));
function showQuotation()document.write(Quotation[whichQuotation]);
showQuotation();
</script>
</body>
Create a Rollover Image
<head>
<script type=”text/javascript”>
<!--
if (document.images) button1 = new Image button2 = new Image button1.src = ‘img1.gif’ button2.src = ‘img2.gif’
//-->
</script>
</head>
<body>
<a href=”http://domainname.com” onmouseover=”document.rollover. src=button2.src” onmouseout=”document.rollover.src=button1.src”><img src=”img1.gif” border=0 name=”rollover”></a>
</body>
Open a New Window
<body>
<script type=”text/javascript”>
<!--
window.open (‘titlepage.html’, ‘newwindow’, config=’height=100, width=400, toolbar=no, menubar=no, scrollbars=no, resizable=no, location=no, directories=no, status=no’)
-->
</script>
</body>
Print This Page
<body>
<a href=”javascript:window.print()”>Click to Print This Page</a>
</body>
OR
<body>
<form>
<input type=”button” onClick=”window.print()”>
</ form >
</body>
Redirect Page
<head>
<script type=”text/javascript”>
<!--
var time = null function move()
window.location = ‘http://www.yourdomain.com’
//-->
</script>
</head>
<body onload=”timer=setTimeout(‘move()’,2000)”>
</body>
Back to Previous Page
<body>
<a href=”javascript: history.go(-1)”>Back</a>
</body>
Hide Javascript From Browsers
Older browsers that do not support javascript will tend to show the code in the visitor's view of your site. You can comment out your script so this does not happen.
<script language=”JavaScript”>
<!--
Put JS code here. Old browsers will treat it as an HTML comment.
-->
</script>