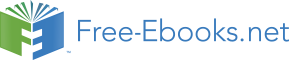

Introduction to C Language
C is a general purpose, high level programming language and is a widely used language. This language has facilities for structured programming and allows to efficiently provide machine instructions. Hence, the language is used for coding assembly language too. The system software like Unix operating system has been coded using C. C is a procedural language. It was designed to be compiled using a relatively straightforward compiler, to provide low-level access to memory, to provide language constructs that map efficiently to machine instructions, and to have a have need of minimal run-time support. C is useful for many applications that had formerly been coded in assembly language, such as in system programming.
Software programs required for a C program
As no computer can understand C, you need several programs that help the computer understand the instructions. The programs are:
Here Turbo C editor is used for C programming.
The table below explains the purpose of the various programs.
The basics of a C programming language needs an understanding of the basic
building blocks of the programming language, the bare minimum C program contains the following parts:
Every C program consists of one or more functions, one of which must be main().
Let us look at a simple code that would print the words "My First Program in C" followed by an explanation of the various parts of the C program:
Structure of a C program
The various parts of the program given above are explained below:
Preprocessor Commands
1. #include<stdio.h>
Start writing a C program with a preprocessor command #include<stdio.h>.
This informs a C compiler to include a header file stdio.h before compiling the program.
Refer Library Functions to know about the list of other library functions that can be included in a C program.
2. main() function
The next line int main() is the main function where program execution begins.
All programs in C should have a main() function. There are two variations of main(). They are void main() and int main(). void main() denotes a function does not return a value, int main() denotes a function that is of a numeric return type.
3. Starting Brace
All C program start with a left brace {.and the program code always ends with a closing brace - }.
4. Statements
Comment Statement
The next line /*...*/ will be ignored by the compiler and it has been put to add additional comments in the program. So such lines are called comments in the program. You can give a brief description about the program within the /*…..*/ comment line statements.
Input and Output statements
Output statement
printf(...)
The next line printf(...) is another function available in C which causes the message "My First Program in C” to be displayed on the screen. All the statements that you want to display on the screen should start with the printf( ) function.
Input Statement
scanf(“%d”, &a);
The data type of the input is specified within double quotes, if it is of integer type then it is % d and &a represents a variable data item.
scanf(…..) function can be used to enter any combination of numerical values, single characters and strings.
Compile & Execute C Program
Let’s look at how to save the source code in a file, and how to compile and run it. Following are the simple steps:
1. Open a text editor and add the above-mentioned code.
2. Save the file as First.c in the TC\Bin directory. The directory where you have the C compiler.
3. Open a command prompt and go to the directory where you saved the file.
4. Type TC First.c and press enter.
5. This opens up the First.c program in the Turbo C window.
6. To compile your code press Alt + F9 key simultaneously.
7. If there are no errors there will be no error message.
8. You have to execute the file to view the output. To execute the file press Ctrl + F9 keys simultaneously.
9. You will be able to see "My First Program in C" printed on the screen.
Output screen of First.c
C uses structured programming with variables and recursive statements. In C, all executable code is contained within "functions" All C program use the semicolon as a statement terminator and curly braces({ }) for grouping blocks of statements.
The following are the key points of C language:
The basic characters that are included in a program are:
Data types
C supports different types of data, the basic C data types are as listed below:
The array types and structure types are referred to collectively as the aggregate The type of a function specifies the type of the function's return value. We will see basic types in the following section.
The void Type
The void type specifies that no value is available. It is used in the following kinds of situations:
Variables
Variable store values and they are used to perform calculations and evaluate A variable refers to values that are not fixed, they keep changing. C programming language also allows defining various other types of variables, other those defined in the following type:
Variable Definition in C
A variable definition means to tell the compiler where and how much to create the storage for the variable. A variable definition specifies a data type and contains a list of one or more variables of a specific data type.
Variable Initialization
Assigning value to the variable is called Variable Initialization. A variable can be initialized at the place where the variable is declared.
Syntax
data_type