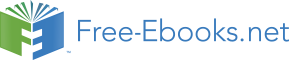

Our First Application
After installing and running Lazarus, we can start a new program from the main menu: Project/New Project/Program
We will get this code in the Source Editor window:
program Project1;
{$mode objfpc}{$H+}
uses
{$IFDEF UNIX}{$IFDEF UseCThreads}
cthreads,
{$ENDIF}{$ENDIF}
Classes
{ you can add units after this };
{$IFDEF WINDOWS}{$R project1.rc}{$ENDIF}
begin
end.
We can save this program by clicking File/Save from the main menu, and then we can name it, for example, first.lpi
Then we can write these lines between the begin and end statements: Writeln('This is Free Pascal and Lazarus');
Writeln('Press enter key to close');
Readln;
The complete source code will be:
program first;
{$mode objfpc}{$H+}
uses
{$IFDEF UNIX}{$IFDEF UseCThreads}
cthreads,
{$ENDIF}{$ENDIF}
Classes
{ you can add units after this };
{$IFDEF WINDOWS}{$R first.rc}{$ENDIF}
begin
Writeln('This is Free Pascal and Lazarus');
Writeln('Press enter key to close');
Readln;
end.
The Writeln statement displays the text on the screen (Console window). Readln halts execution to let the user read the displayed text until he/she presses enter to close the application and return to the Lazarus IDE.
Then press F9 to run the application or click the button:
After running the first program, we will get this output text:
This is Free Pascal and Lazarus
Press enter key to close
If we are using Linux, we will find a new file in a program directory called (first), and in Windows we will get a file named first.exe. Both files can be executed directly by double clicking with the mouse.
The executable file can be copied to other computers to run without the need of the Lazarus IDE.
Notes
If the console application window does not appear, we can disable the debugger from the Lazarus menu:
Environment/Options/Debugger
In Debugger type and path select (None)
Other examples
In the previous program change this line:
Writeln('This is Free Pascal and Lazarus');
to this one:
Writeln('This is a number: ', 15);
Then press F9 to run the application.
You will get this result:
This is a number: 15
Change the previous line as shown below, and run the application each time:
Code:
Writeln('This is a number: ', 3 + 2);
Output:
This is a number: 5
Code:
Writeln('5 * 2 = ', 5 * 2);
Output:
5 * 2 = 10
Code:
Writeln('This is real number: ', 7.2);
Output:
This is real number: 7.2000000000000E+0000
Code:
Writeln('One, Two, Three : ', 1, 2, 3);
Output:
One, Two, Three : 123
Code:
Writeln(10, ' * ', 3 , ' = ', 10 * 3);
Output:
10 * 3 = 30
We can write different values in the Writeln statement each time and see the result. This will help us understand it clearly.
Variables
Variables are data containers. For example, when we say that X = 5, that means X is a variable, and it contains the value 5.
Object Pascal is a strongly typed language, which means we should declare a variable's type before putting values into it. If we declare X as an integer, that means we should put only integer numbers into X during its life time in the application.
Examples of declaring and using variables:
program FirstVar;
{$mode objfpc}{$H+}
uses
{$IFDEF UNIX}{$IFDEF UseCThreads}
cthreads,
{$ENDIF}{$ENDIF}
Classes
{ you can add units after this };
var
x: Integer;
begin
x:= 5;
Writeln(x * 2);
Writeln('Press enter key to close');
Readln;
end.
We will get 10 in the application's output.
Note that we used the reserved word Var, which means the following lines will be variable declarations: x: Integer;
This means two things:
1. The variable's name is X; and
2. the type of this variable is Integer, which can hold only integer numbers without a fraction. It could also hold negative values as well as positive values.
And the statement:
x:= 5;
means put the value 5 in the variable X.
In the next example we have added the variable Y:
var
x, y: Integer;
begin
x:= 5;
y:= 10;
Writeln(x * y);
Writeln('Press enter key to close');
Readln;
end.
The output of the previous application is:
50
Press enter key to close
50 is the result of the formula (x * y).
In the next example we introduce a new data type called character:
var
c: Char;
begin
c:= 'M';
Writeln('My first letter is: ', c);
Writeln('Press enter key to close');
Readln;
end.
This type can hold only one letter, or a number as an alphanumeric character, not as value.
In the next example we introduce the real number type, which can have a fractional part: var
x: Single;
begin
x:= 1.8;
Writeln('My Car engine capacity is ', x, ' liters');
Writeln('Press enter key to close');
Readln;
end.
To write more interactive and flexible applications, we need to accept input from the user. For example, we could ask the user to enter a number, and then get this number from the user input using the Readln statement / procedure:
var
x: Integer;
begin
Write('Please input any number:');
Readln(x);
Writeln('You have entered: ', x);
Writeln('Press enter key to close');
Readln;
end.
In this example, assigning a value to X is done through the keyboard instead of assigning it a constant value in the application.
In the example below we show a multiplication table for a number entered by the user: program MultTable;
{$mode objfpc}{$H+}
uses
{$IFDEF UNIX}{$IFDEF UseCThreads}
cthreads,
{$ENDIF}{$ENDIF}
Classes
{ you can add units after this };
var
x: Integer;
begin
Write('Please input any number:');
Readln(x);
Writeln(x, ' * 1 = ', x * 1);
Writeln(x, ' * 2 = ', x * 2);
Writeln(x, ' * 3 = ', x * 3);
Writeln(x, ' * 4 = ', x * 4);
Writeln(x, ' * 5 = ', x * 5);
Writeln(x, ' * 6 = ', x * 6);
Writeln(x, ' * 7 = ', x * 7);
Writeln(x, ' * 8 = ', x * 8);
Writeln(x, ' * 9 = ', x * 9);
Writeln(x, ' * 10 = ', x * 10);
Writeln(x, ' * 11 = ', x * 11);
Writeln(x, ' * 12 = ', x * 12);
Writeln('Press enter key to close');
Readln;
end.
Note that in the previous example all the text between single quotation marks (') is displayed in the console window as is, for example:
' * 1 = '
Variables and expressions that are written without single quotation marks are evaluated and written as values.
See the difference between the two statements below:
Writeln('5 * 3');
Writeln(5 * 3);
The result of first statement is:
5 * 3
Result of the second statement is evaluated then displayed:
15
In the next example, we will do mathematical operations on two numbers (x, y), and we will put the result in a third variable (Res):
var
x, y: Integer;
Res: Single;
begin
Write('Input a number: ');
Readln(x);
Write('Input another number: ');
Readln(y);
Res:= x / y;
Writeln(x, ' / ', y, ' = ', Res);
Writeln('Press enter key to close');
Readln;
end.
Since the operation is division, it might result in a number with a fraction, so for that reason we have declared the Result variable (Res) as a real number ( Single). Single means a real number with single precision floating point.
Sub types
There are many sub types for variables, for example, Integer number subtypes differ in the range and the number of required bytes to store values in memory.
The table below contains integer types, value ranges, and required bytes in memory: Type
Min value
Max Value
Size in Bytes
Byte
0
255
1
ShortInt
128
127
1
SmallInt
32768
32767
2
Word
0
65535
2
Integer
2147483648
2147483647
4
LongInt
2147483648
2147483647
4
Cardinal
0
4294967295
4
Int64
9223372036854780000
9223372036854775807
8
We can get the minimum and maximum values and bytes sizes for each type by using the Low, High, and SizeOf functions respectively, as in the example below:
program Types;
{$mode objfpc}{$H+}
uses
{$IFDEF UNIX}{$IFDEF UseCThreads}
cthreads,
{$ENDIF}{$ENDIF}
Classes;
begin
Writeln('Byte: Size = ', SizeOf(Byte),
', Minimum value = ', Low(Byte), ', Maximum value = ',
High(Byte));
Writeln('Integer: Size = ', SizeOf(Integer),
', Minimum value = ', Low(Integer), ', Maximum value = ',
High(Integer));
Write('Press enter key to close');
Readln;
end.
Conditional Branching
One of the most important features of intelligent devices (like computers, programmable devices) is that they can take actions in different conditions. This can be done by using conditional branching. For example, some cars lock the door when the speed reaches or exceeds 40 K/h. The condition in this case will be:
If speed is >= 40 and doors are unlocked, then lock door.
Cars, washing machines, and many other gadgets contains programmable circuits like micro controllers, or small processors like ARM. Such circuits can be programmed using assembly, C, or Free Pascal according to their architecture.
The If condition
The If condition statement in the Pascal language is very easy and clear. In the example below, we want to decide whether to turn on the airconditioner or turn it off, according to the entered room temperature:
AirConditioner program:
var
Temp: Single;
begin
Write('Please enter Temperature of this room :');
Readln(Temp);
if Temp > 22 then
Writeln('Please turn on air-condition')
else
Writeln('Please turn off air-condition');
Write('Press enter key to close');
Readln;
end.
We have introduced the if then else statement, and in this example: if the temperature is greater than 22, then display the first sentence::
Please turn on air-conditioner
else, if the condition is not met (less than or equal to 22) , then display this line: Please turn off air-conditioner
We can write multiple conditions like:
var
Temp: Single;
begin
Write('Please enter Temperature of this room :');
Readln(Temp);
if Temp > 22 then
Writeln('Please turn on air-conditioner')
else
if Temp < 18 then
Writeln('Please turn off air-conditioner')
else
Writeln('Do nothing');
You can test the above example with different temperature values to see the results.
We can make conditions more complex to be more useful:
var
Temp: Single;
ACIsOn: Byte;
begin
Write('Please enter Temperature of this room : ');
Readln(Temp);
Write('Is air conditioner on? if it is (On) write 1,',
' if it is (Off) write 0 : ');
Readln(ACIsOn);
if (ACIsOn = 1) and (Temp > 22) then
Writeln('Do nothing, we still need cooling')
else
if (ACIsOn = 1) and (Temp < 18) then
Writeln('Please turn off air-conditioner')
else
if (ACIsOn = 0) and (Temp < 18) then
Writeln('Do nothing, it is still cold')
else
if (ACIsOn = 0) and (Temp > 22) then
Writeln('Please turn on air-conditioner')
else
Writeln('Please enter a valid values');
Write('Press enter key to close');
Readln;
end.
In the above example, we have used the new keyword ( and) which means if the first condition returns True (ACIsOn = 1), and the second condition returns True (Temp > 22), then execute the Writeln statement. If one condition or both of them return False, then it will go to the else part.
If the airconditioner is connected to a computer via the serial port for example, then we can turn it on/off from that application, using serial port procedures/components. In this case we need to add extra parameters for the if condition, like for how long the airconditioner was operating. If it exceeds the allowable time (for example, 1 hour) then it should be turned off regardless of room temperature. Also we can consider the rate of losing coolness, if it is very slow (at night), then we could turn if off for longer time.
We
W ight program
In this example, we ask the user to enter his/her height in meters, and weight in Kilos. Then the program will calculate the suitable weight for that person according to the entered data, and then it will tell him/her the results:
program Weight;
{$mode objfpc}{$H+}
uses
{$IFDEF UNIX}{$IFDEF UseCThreads}
cthreads,
{$ENDIF}{$ENDIF}
Classes, SysUtils
{ you can add units after this };
var
Height: Double;
Weight: Double;
IdealWeight: Double;
begin
Write('What is your height in meters (e.g. 1.8 meter) : ');
Readln(Height);
Write('What is your weight in kilos : ');
Readln(Weight);
if Height >= 1.4 then
IdealWeight:= (Height - 1) * 100
else
IdealWeight:= Height * 20;
if (Height < 0.4) or (Height > 2.5) or (Weight < 3) or (Weight > 200) then
begin
Writeln('Invalid values');
Writeln('Please enter proper values');
end
else
if IdealWeight = Weight then
Writeln('Your weight is suitable')
else
if IdealWeight > Weight then
Writeln('You are under weight, you need more ',
Format('%.2f', [IdealWeight - Weight]), ' Kilos')
else
Writeln('You are over weight, you need to lose ',
Format('%.2f', [Weight - IdealWeight]), ' Kilos');
Write('Press enter key to close');
Readln;
end.
In this example, we have used new keywords:
1. Double: which is similar to Single. Both of them are real numbers, but Double has a double precision floating point, and it requires 8 bytes in memory, while single requires only 4 bytes.
2. The second new thing is the keyword ( Or) and we have used it to check if one of the conditions is met or not. If one of condition is met, then it will execute the statement. For example: if the first condition returns True (Height < 0.4), then the Writeln statement will be called: Writeln('Invalid values'); . If the first condition returns False, then it will check the second one, etc. If all conditions returns False, it will go to the else part.
3. We have used the keywords begin end with the if statement, because if statement should execute one statement. Begin end converts multiple statements to be considered as one block (statement), then multiple statements could be executed by if condition. Look for these two statemetns:
Writeln('Invalid values');
Writeln('Please enter proper values');
It has been converted to one statement using begin end:
if (Height < 0.4) or (Height > 2.5) or (Weight < 3) or (Weight > 200) then
begin
Writeln('Invalid values');
Writeln('Please enter proper values');
end
4. We have used the procedure Format, which displays values in a specific format. In this case we need to display only 2 digits after the decimal point. We need to add the SysUtils unit to the Uses clause in order to use this function.
What is your height in meters (e.g. 1.8 meter) : 1.8
What is your weight in kilos : 60.2
You are under weight, you need more 19.80 Kilos
Note:
This example may be not 100% accurate. You can search the web for weight calculation in detail. We meant only to explain how the programmer could solve such problems and do good analysis of the subject to produce reliable applications.
Case .. of statement
There is another method for conditional branching, which is the Case .. Of statement. It branches execution according to the case ordinal value. The Restaurant program will illustrate the use of the case of statement:
Restaurant program
var
Meal: Byte;
begin
Writeln('Welcome to Pascal Restaurant. Please select your order');
Writeln('1 - Chicken (10$)');
Writeln('2 - Fish (7$)');
Writeln('3 - Meat (8$)');
Writeln('4 – Salad (2$)');
Writeln('5 - Orange Juice (1$)');
Writeln('6 - Milk (1$)');
Writeln;
Write('Please enter your selection: ');
Readln(Meal);
case Meal of
1: Writeln('You have ordered Chicken,',
' this will take 15 minutes');
2: Writeln('You have ordered Fish, this will take 12 minutes');
3: Writeln('You have ordered meat, this will take 18 minutes');
4: Writeln('You have ordered Salad, this will take 5 minutes');
5: Writeln('You have ordered Orange juice,',
' this will take 2 minutes');
6: Writeln('You have ordered Milk, this will take 1 minute');
else
Writeln('Wrong entry');
end;
Write('Press enter key to close');
Readln;
end.
If we write the same application using the if condition, it will become more complicated, and will contain duplications:
Restaurant program using If condition
var
Meal: Byte;
begin
Writeln('Welcome to Pascal restaurant, please select your meal');
Writeln('1 - Chicken (10$)');
Writeln('2 - Fish (7$)');
Writeln('3 - Meat (8$)');
Writeln('4 - Salad (2$)');
Writeln('5 - Orange Juice (1$)');
Writeln('6 - Milk (1$)');
Writeln;
Write('Please enter your selection: ');
Readln(Meal);
if Meal = 1 then
Writeln('You have ordered Chicken, this will take 15 minutes')
else
if Meal = 2 then
Writeln('You have ordered Fish, this will take 12 minutes')
else
if Meal = 3 then
Writeln('You have ordered meat, this will take 18 minutes')
else
if Meal = 4 then
Writeln('You have ordered Salad, this will take 5 minutes')
else
if Meal = 5 then
Writeln('You have ordered Orange juice,' ,
' this will take 2 minutes')
else
if Meal = 6 then
Writeln('You have ordered Milk, this will take 1 minute')
else
Writeln('Wrong entry');
Write('Press enter key to close');
Readln;
end.
In the next example, the application evaluates students' marks and converts them to grades: A, B, C, D, E, and F:
Students' Grades program
var
Mark: Integer;