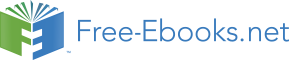

2.1 What is Pseudocode?
Pseudocode is basically a tersely worded English language description of the algorithms to be implemented later in a programming language. In days of old, you were told not to use words specific to a programming language. While that is good advice, many of the words you need to use were implemented as verbs by a large number of 3GL programming languages. Words like “if,” “do-while” and “perform” have been implemented in one fashion or another by many of the languages you will find yourself eventually programming in. As a consequence of this: Most pseudocode looks a lot like COBOL code.
You will find pseudocode still in use at many shops, but not as it was originally intended. It now exists primarily in sections of systems specifications. When an analyst writes a specification for a new and large system, there will inevitably be several areas where tricky algorithms or formulas are needed. Were this formula simply named or written as a paragraph of text, they would be open to interpretation. To remove that point of failure early on, the analyst will provide the formula or algorithm in detailed pseudocode either directly in the specification or as an appendix.
2.2 Rules of Pseudocode
There aren’t many rules for pseudocode. Each statement must end with a period just like in the COBOL programming language. You are expressly forbidden to use the word “GOTO” in your pseudocode. Paragraph names go on the left margin and the logic they contain is indented. Pages need to be numbered. If you have many pages, you need to generate a table of contents listing each paragraph name and the page it is on. External routines are to be written in all upper case.
The phrase “external routines” is one of those phrases tossed about by people who don’t like ugly details. It is a phrase like “family values,” which means something different to everyone who hears it, yet makes everyone believe they know what you are talking about. In general it means pre-existing routines or programs either provided by the operating system or previously developed by your shop. Therein lies the rub. What happens when you are writing pseudocode for a completely new system consisting of dozens of programs and functions? Each program will utilize some or all of the external functions you have written up, but which are not yet implemented. Do they qualify as external? Most would say yes, but you need to iron this definition out with whoever will be reviewing your pseudocode prior to writing.
The why and when of pseudocode have the same answer. Once you have done enough flowcharting to inherently understand the difference between good and bad logic, you will migrate to pseudocode. You cannot start learning to program and become a great programmer, just like you cannot start programming by learning the syntax of a language and jumping into coding. Flowcharting teaches you to visualize the tools of logic you will need to get from Point A to Point B. Flowcharting also takes a lot of time.
When you are first learning to flowchart, you will start over many times. This is neither right nor wrong, simply part of the learning process. After you have done a few flowcharts, you will realize you don’t have to start over as often. Pretty soon you will realize you almost never use your eraser except to fix spelling errors. Once you get to that point, you are ready for pseudocode. Your mind has expanded enough to grasp logic as a tool.
Pseudocode lets you wield that tool more quickly. While it is true that there are software packages that let you create flowcharts, they can be more cumbersome to use and learn than just using a pencil, paper and plastic flowcharting template. Indeed, most every developer educated through the mid-1980s used no more than that. Pseudocode can be written with any word processor, even the free ones like OpenOffice. You do, however, need one which will let you keep multiple documents open for editing simultaneously. One document contains the table of contents or list of functions/paragraphs you have already written; another, the pseudocode you are working on; and a third for the functions/paragraphs you have named but not yet implemented. Hopefully you won’t have to use a really crummy word processor like Microsoft Word. If you learn how to use tabbed sections correctly, Lotus Word Pro is one of the best word processors ever developed. A close second is Word Perfect. If your school only has Microsoft Word, I humbly suggest you go looking for a free word processor to use.
2.4 How Do You Learn to Write Pseudocode?
First, you learn to draw flowcharts. Not just the mechanics of connecting symbols, but how to visualize the logic. You learn what an algorithm is and the difference between a function and a subroutine. You learn that a function is a small algorithm that may use other algorithms just like a subroutine. Then you can learn to write pseudocode. You learn by doing and that is why this book focuses on in-class exercises. Your instructor will guide you by letting you make your own mistakes, then helping you correct them.
2.5 Linear Sequence
Our linear sequence example from Chapter One would read as follows:
Linear_Sequence:
Open input file.
Read Input.
Display Input.
Close File.
While this example is crude and has no earth-shattering consequences, it shows you a lot. Other than the name, nothing here lets you “see” it is a linear sequence. When we flowcharted a linear sequence you could tell by the connecting lines and arrow heads that it did not loop. As I stated earlier, you have to learn to flowchart before you can be effective with pseudocode.
2.6 Top Checking Loop
Redeveloping our top checking loop from Chapter One gives us a little more to talk about.
Top_Checking_Loop:
Let x = 0.
While x <= 10
Display x.
Add 1 to x.
End while.
If you have done any programming prior to reading this book, you will notice that many of the words used in this little example tend to be words from a language you have worked with. “Display” is typically used when you are writing to the default output device. In this day and age that is the screen a user is sitting at. This is the exact same verb COBOL uses for the exact same task.
Most every programming language you work with will have some form of the while loop as well. Just be careful when seeing the verb “while” in your programming language because it is not synonymous with a top checking loop. You will see that in our bottom checking example.
The line “Add 1 to x” is also a COBOL syntax. As I said earlier, most of your pseudocode will read like COBOL. It is not an accident. COBOL was developed in the 1970s to be an English-like programming language. Pseudocode is an English-like program description language. The two are going to overlap a lot.
2.7 Middle Checking Loop
The one thing you will learn by reading pseudocode for our middle checking loop example from Book One is that pseudocode doesn’t give you a good method for describing some of the realities you will face in the programming world. Indeed, the middle checking loop is so frowned upon by the goto-less programming purists, that they made it difficult to implement in pseudocode.
Middle_checking_loop:
Set eof to false.
Open input file.
Loop_top:
Read a record.
If eof <> true
Perform do_something.
else
Close input file.
Exit program.
End if.
next loop_top.
You get forced into the middle checking loop by reality some times. With some programming languages, you cannot call or test for end of file until you have performed at least one read from the file. Simply having it open isn’t good enough. Other languages, like COBOL, completely hide the middle checking aspect by providing an AT END clause on the read statement where you would put the logic to close the file and exit the program. Purists maintain that you shouldn’t take into consideration the implementation language when writing pseudocode. The reality is that programming shops only support a limited number of languages and the programmers tend to think in terms of those languages.
2.8 Bottom Checking Loop
Bottom_checking_loop:
Let x = 0.
Do
Add 1 to x.
Display x.
while x <= 10.
The pseudocode for our example from Chapter One is pretty straight forward. You may notice throughout this book that I use “set,” “let” and “assign” interchangeably when initializing variables. This is a common practice with pseudocode. When it comes to actual implementation, the language will dictate how you do this. Some have very different meanings for “set” and “assign.” “Let” is a keyword from the BASIC programming language. Most versions of BASIC either no longer support it or simply skip over it. In the early days of programming, it helped students to read “LET X = 0" so the compilers forced this syntax. Later, compiler writers realized that the compiler only cared about the “X=0" part so the syntax was relaxed.
2.9 Multiple Decisions
When showing you decisions implemented as nested “if” statements or conditions for flowcharting, I was able to create an empty flowchart to emphasize the point of not giving your decision symbols “wings.” Pseudocode doesn’t allow for an easy example of that because you have to have words for everything. The developer writing the pseudocode is free to keep indenting if statements to their hearts content. If they choose to use a text editor instead of a word processor to write their pseudocode, they won’t even be stopped by the right margin wrapping function built into most word processors. This is yet another reason you have to learn flowcharting before you learn pseudocode. It teaches you how to avoid nesting decisions too deeply.
Chapter One did give us a nice example of the select-case logic tool. Here it is in pseudocode.
Evaluate customer_type
When “NEW”
Display offer for new credit card.
Break.
When “GOOD”
Display offer for 10% discount.
Break.
When “BIG”
Display offer for 10% discount.
Offer gift.
Break.
When “DELINQUENT”
Send to manager.
Break.
Default
Enter into sweepstakes.
End Evaluate.
I chose to use the evaluate-when combination, but could have just as easily used select-case or switch-case. The syntax for this logic structure didn’t appear in pseudocode until after it had appeared in several programming languages so in this case, the languages defined the syntax for pseudocode.
Please take note of the break statement. When writing this logic structure in pseudocode, always use the break statement if you don’t wish a programmer to allow for execution from one case to fall through to the next. Some languages, most notably C, allow for cases to fall through each other. While that technique can be handy, it can get you into a lot of trouble if the compiler optimization decides to change the order of the statements it generates. Let me show you.
Select_case_example_with_fal _through:
Evaluate customer_type
When “NEW”
Display offer for new credit card.
Break.
When “BIG”
Offer gift.
When “GOOD”
Display offer for 10% discount.
Break.
When “DELINQUENT”
Send to manager.
Break.
Default
Enter into sweepstakes.
End Evaluate.
Notice the subtle changes. We removed the duplicate logic to display a 10% discount by moving the when “BIG” condition above “GOOD” and allowing it to fall through the “GOOD” condition. When drawing logic with a flowchart, you don’t have to worry about this kind of ambiguity. The lines and arrows only go to one place and it is the responsibility of the developer to implement what is drawn. Words are open to interpretation. If you leave out the break and really mean for the cases to be independent of each other, then you are at the mercy of the programmer and the programming language when it comes to implementation.
2.10 Pseudocode Followup
Pseudocode is a quick and powerful program specification tool. With it’s speed comes a lot of opportunity for error. As was demonstrated with the select-case example, assumptions or simple omissions can have drastic results during implementation. Once you get into the real world as a programmer, you will only use pseudocode to work out intricate details of tough routines. In truth, if the problem is really difficult, you will see seasoned developers reaching for pencil and paper to actually flowchart that little section.
Pseudocode allows for assumptions, flowcharting does not. When you become good at flowcharting, you will be ready to write pseudocode. One of the biggest crimes that occur with pseudocode is the omission of detail. It is not uncommon to see a statement like “Compute Interest Rate” in pseudocode without any hint or direction about how to do it. Things like that are much more difficult to hide when someone looks at a flowchart.