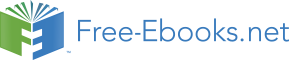

// Create a FileSystem object
FileSystem fs = FileSystem.get(conf);
// Create a Path object for the input file
Path inputPath = new Path("/user/hadoop/input.txt");
// Create a Path object for the output file
Path outputPath = new Path("/user/hadoop/output.txt");
// Create an FSDataInputStream object to read data from the input file FSDataInputStream in = fs.open(inputPath);
// Create an FSDataOutputStream object to write data to the output file FSDataOutputStream out = fs.create(outputPath);
// Read data from the input file and write it to the output file String line = in.readLine();
while (line != null) {
out.writeBytes(line + "\n");
line = in.readLine();
}
// Close the files
in.close();
out.close();
62
3.2 How to perform MapReduce operations on ad data using Java MapReduce is a programming paradigm that is based on paral el processing of large datasets and is using the key-value pairs. MapReduce consists of two phases: chart and merge. In the map phase an input is taken as a key-value pair and it produces an intermediate key-value pair as its output. The second phase, reduce, is where the multiple pairs of intermediate key-value pairs with the same key are combined to produce one nal output key-value pair. MapReduce is among the most popular tools for batch processing and o ine analytics of ad data, which include, but are not limited to, ad impressions, clicks and conversions, revenue and so on.
To perform MapReduce operations on ad data using Java, we need to fol ow these steps: 1. De ne a Mapper class that extends the Mapper class of the org.apache.hadoop.mapreduce package. The Mapper class has four generic types: KEYIN, VALUEIN, KEYOUT, and VALUEOUT. These represent the input key, input value, output key, and output value types of the map function. The Mapper class has a map() method that takes an input key-value pair and produces a set of intermediate key-value pairs. We need to override this method and implement our own logic for the map function.
2. De ne a Reducer class that extends the Reducer class of the org.apache.hadoop.mapreduce package. The Reducer class has four generic types: KEYIN, VALUEIN, KEYOUT, and VALUEOUT. These represent the input key, input value, output key, and output value types of the reduce function. The Reducer class has a reduce() method that takes a set of intermediate key-value pairs with the same key and produces a nal output key-value pair.
We need to override this method and implement our own logic for the reduce function.
3. De ne a Driver class that contains the main method of the MapReduce application. The Driver class is responsible for con guring and running the MapReduce job. The Driver class needs to create a Job object that represents the MapReduce job. The Job object has various methods to set the job name, the input and output paths, the mapper and reducer classes, the output key and value classes, the number of reducers, etc. The Driver class also needs to cal the waitForCompletion() method of the Job object to submit the job and wait for its completion.
63
Here is an example of how to perform MapReduce operations on ad data using Java. The example calculates the total revenue for each advertiser from a given ad data le. The ad data le has the fol owing format:
ad_id,advertiser_id,impressions,clicks,conversions,revenue
1,
101,
1000,
100,
10,
1000
2,
102,
2000,
200,
20,
2000
3,
103,
3000,
300,
30,
3000
4,
101,
4000,
400,
40,
4000
5,
102,
5000,
500,
50,
5000
6,
103,
6000,
600,
60,
6000
The MapReduce application has the fol owing classes:
// Import the required classes
import org.apache.hadoop.conf.Configuration;
import org.apache.hadoop.fs.Path;
import org.apache.hadoop.io.IntWritable;
import org.apache.hadoop.io.Text;
import org.apache.hadoop.mapreduce.Job;
import org.apache.hadoop.mapreduce.Mapper;
import org.apache.hadoop.mapreduce.Reducer;
import org.apache.hadoop.mapreduce.lib.input.FileInputFormat; import org.apache.hadoop.mapreduce.lib.output.FileOutputFormat;
// Define Mapper that extends the Mapper class
public class AdRevenueMapper extends Mapper< Object, Text, IntWritable, IntWritable> {
// Define output key and value objects
private IntWritable advertiserId = new IntWritable();
private IntWritable revenue = new IntWritable();
// Override map() method
public void map(Object key, Text value, Context context) throws IOException, InterruptedException {
// Split input value by comma
String[] fields = value.toString().split(",");
// Skip the header line
if (fields[0].equals("ad_id")) {
return;
}
// Parse advertiser id and revenue from the input value
int advertiserId = Integer.parseInt(fields[1]);
int revenue = Integer.parseInt(fields[5]);
64
// Set output key and value objects
this.advertiserId.set(advertiserId);
this.revenue.set(revenue);
// Write output key-value pair to the context
context.write(this.advertiserId, this.revenue);
}
}
// Define Reducer that extends the Reducer class
public class AdRevenueReducer extends Reducer< IntWritable, IntWritable, IntWritable, IntWritable> {
// Define output value object
private IntWritable totalRevenue = new IntWritable();
// Override reduce() method
public void reduce(IntWritable key, Iterable<IntWritable> values, Context context) throws IOException, InterruptedException {
// Initialize the total revenue to zero
int totalRevenue = 0;
// Iterate over the input values and sum them up
for (IntWritable value : values) {
totalRevenue += value.get();
}
// Set output value object
this.totalRevenue.set(totalRevenue);
// Write output key-value pair to the context
context.write(key, this.totalRevenue);
}
}
// Define Driver that contains main method of the MapReduce application public class AdRevenueDriver {
// Define main method
public static void main(String[] args) throws Exception {
// Create Configuration object
Configuration conf = new Configuration();
// Create Job object
Job job = Job.getInstance(conf, "Ad Revenue Calculator");
// Set jar by class
job.setJarByClass(AdRevenueDriver.class);
// Set mapper and reducer classes
job.setMapperClass(AdRevenueMapper.class);
job.setReducerClass(AdRevenueReducer.class);
65
// Set output key and value classes
job.setOutputKeyClass(IntWritable.class);
job.setOutputValueClass(IntWritable.class);
// Set input and output paths
FileInputFormat.addInputPath(job, new Path(args[0]));
FileOutputFormat.setOutputPath(job, new Path(args[1]));
// Submit job and wait for its completion
System.exit(job.waitForCompletion(true) ? 0 : 1);
}
}
66
3.3 How to create and run Spark applications on ad data using Java Spark is a uni ed analytics engine that o ers an advanced processing speed for working with large amounts of data, which can be distributed across clusters of computers. Spark al ows for the development of multiple language programs, such as Java, and provides numerous libraries for streaming, SQL, machine learning, graph, and natural language processing. Spark is a common tool in AdTech, providing the possibility of extremely fast ad data processing and analysis, such as for example impression, clicks, conversions, revenue calculation, etc.
To create and run Spark applications on ad data using Java, we need to fol ow these steps: 1. De ne a SparkSession object that represents the entry point to the Spark application. The SparkSession object al ows us to create and manipulate DataFrames and Datasets, which are the main abstractions for working with structured and semi-structured data in Spark.
The SparkSession object also al ows us to access various Spark features, such as Spark SQL, Spark Streaming, Spark MLlib, etc.
2. De ne a JavaRDD object that represents a resilient distributed dataset (RDD), which is the main abstraction for working with unstructured and raw data in Spark. The JavaRDD
object al ows us to apply transformations and actions on the data, such as map, lter, reduce, count, etc. The JavaRDD object can be created from various sources, such as les, col ections, databases, streams, etc.
3. De ne a JavaSparkContext object that represents the connection to the Spark cluster. The JavaSparkContext object al ows us to con gure and manage the Spark application, such as setting the master URL, the application name, the number of cores, the memory, etc. The JavaSparkContext object can be obtained from the SparkSession object by cal ing the sparkContext() method.
4. De ne a StructType object that represents the schema of the ad data. The StructType object al ows us to de ne the elds and types of the ad data, such as ad_id, advertiser_id, impressions, clicks, conversions, revenue, etc. The StructType object can be created by using the createStructType() method of the DataTypes class and passing a list of StructField objects as a parameter.
5. De ne a JavaBean class that represents the ad data as a Java object. The JavaBean class al ows us to encapsulate the ad data as a Java object with getters and setters for each eld.
The JavaBean class must implement the Serializable interface and have a public no-argument constructor. The JavaBean class can be used to convert the JavaRDD object to a Dataset object by using the createDataset() method of the SparkSession object.
6. De ne a Dataset object that represents a distributed col ection of data organized into named columns. The Dataset object al ows us to perform various operations on the data, such as selecting, ltering, grouping, aggregating, joining, etc. The Dataset object can be created from various sources, such as les, col ections, databases, streams, etc. The Dataset 67
object can also be created from a JavaRDD object by using the createDataFrame() or createDataset() methods of the SparkSession object and passing the JavaRDD object and the schema or the JavaBean class as parameters.
7. De ne a SQLContext object that represents the context for working with structured and semi-structured data using SQL queries. The SQLContext object al ows us to register the Dataset object as a temporary view and execute SQL queries on it. The SQLContext object can be obtained from the SparkSession object by cal ing the sqlContext() method.
8. De ne a StreamingContext object that represents the context for working with streaming data using Spark Streaming. The StreamingContext object al ows us to create and manipulate DStreams, which are the main abstractions for working with streaming data in Spark. The StreamingContext object can be created by passing the JavaSparkContext object and the batch duration as parameters.
9. De ne a DStream object that represents a discretized stream of data. The DStream object al ows us to apply transformations and actions on the streaming data, such as map, lter, reduce, count, etc. The DStream object can be created from various sources, such as les, sockets, Kafka, etc. The DStream object can also be converted to a Dataset object by using the foreachRDD() method and passing a function that creates a Dataset object from a JavaRDD object as a parameter.
10. De ne a SparkConf object that represents the con guration settings for the Spark application. The SparkConf object al ows us to set various parameters for the Spark application, such as the master URL, the application name, the number of cores, the memory, etc. The SparkConf object can be created by using the SparkConf() constructor and passing the key-value pairs as parameters.
11. De ne a JavaStreamingContext object that represents the connection to the Spark Streaming cluster. The JavaStreamingContext object al ows us to con gure and manage the Spark Streaming application, such as setting the master URL, the application name, the number of cores, the memory, etc. The JavaStreamingContext object can be obtained from the StreamingContext object by cal ing the javaStreamingContext() method.
68
Here is an example of how to create and run a Spark application on ad data using Java. The example calculates total revenue for each advertiser from a given ad data le. The ad data le has the same format as previous example.
The Spark application has the fol owing classes:
// Import required classes
import org.apache.spark.SparkConf;
import org.apache.spark.api.java.JavaRDD;
import org.apache.spark.api.java.JavaSparkContext;
import org.apache.spark.sql.Dataset;
import org.apache.spark.sql.Row;
import org.apache.spark.sql.SparkSession;
import org.apache.spark.sql.types.DataTypes;
import org.apache.spark.sql.types.StructField;
import org.apache.spark.sql.types.StructType;
// Define JavaBean class that represents the ad data as a Java object public class AdData implements Serializable {
// Define fields and types of the ad data
private int ad_id;
private int advertiser_id;
private int impressions;
private int clicks;
private int conversions;
private int revenue;
// Define public no-argument constructor
public AdData() {
}
// Define getters and setters for each field
public int getAd_id() {
return ad_id;
}
public void setAd_id(int ad_id) {
this.ad_id = ad_id;
}
public int getAdvertiser_id() {
return advertiser_id;
}
public void setAdvertiser_id(int advertiser_id) {
this.advertiser_id = advertiser_id;
}
69
return impressions;
}
public void setImpressions(int impressions) {
this.impressions = impressions;
}
public int getClicks() {
return clicks;
}
public void setClicks(int clicks) {
this.clicks = clicks;
}
public int getConversions() {
return conversions;
}
public void setConversions(int conversions) {
this.conversions = conversions;
}
public int getRevenue() {
return revenue;
}
public void setRevenue(int revenue) {
this.revenue = revenue;
}
}
// Define a Driver class that contains the main method of the Spark application public class AdRevenueSparkDriver {
// Define main method
public static void main(String[] args) throws Exception {
// Create SparkConf object
SparkConf conf = new SparkConf();
// Set master URL
conf.setMaster("local[*]");
// Set application name
conf.setAppName("Ad Revenue Spark Calculator");
// Create JavaSparkContext object
JavaSparkContext sc = new JavaSparkContext(conf);
// Create SparkSession object
SparkSession spark = SparkSession.builder().config(conf).getOrCreate(); 70
// Create StructType object that represents schema of the ad data StructType schema = DataTypes.createStructType(new StructField[]{
DataTypes.createStructField("ad_id", DataTypes.IntegerType, true),
DataTypes.createStructField("advertiser_id",
DataTypes.IntegerType, true),
DataTypes.createStructField("impressions", DataTypes.IntegerType, true),
DataTypes.createStructField("clicks", DataTypes.IntegerType, true),
DataTypes.createStructField("conversions", DataTypes.IntegerType, true),
DataTypes.createStructField("revenue", DataTypes.IntegerType, true)
});
// Create JavaRDD object that represents the ad data file
JavaRDD<String> adDataFile = sc.textFile(args[0]);
// Filter out the header line
adDataFile = adDataFile.filter(line -> !line.startsWith("ad_id"));
// Create JavaRDD object that represents the ad data as Java objects JavaRDD<AdData> adDataRDD = adDataFile.map(line -> {
// Split the line by comma
String[] fields = line.split(",");
// Parse fields and create an AdData object
AdData adData = new AdData();
adData.setAd_id(Integer.parseInt(fields[0]));
adData.setAdvertiser_id(Integer.parseInt(fields[1]));
adData.setImpressions(Integer.parseInt(fields[2]));
adData.setClicks(Integer.parseInt(fields[3]));
adData.setConversions(Integer.parseInt(fields[4]));
adData.setRevenue(Integer.parseInt(fields[5]));
// Return AdData object
return adData;
});
// Create Dataset object that represents the ad data as a distributed collection of data
Dataset<Row> adDataDF = spark.createDataFrame(adDataRDD, AdData.class);
// Register Dataset object as a temporary view
adDataDF.createOrReplaceTempView("ad_data");
String query = "SELECT"
+ " advertiser_id, SUM(revenue) AS total_revenue"
+ " FROM ad_data"
71
// Execute a SQL query to calculate the total revenue for each advertiser Dataset<Row> resultDF = spark.sql(query);
// Show the result
resultDF.show();
// Stop SparkSession and SparkContext
spark.stop();
sc.stop();
}
}
This is how we can create and run a Spark application on ad data using Java. In the next section, we wil learn how to produce and consume data streams from Kafka using Java.
72
3.4 How to produce and consume data streams from Kafka Kafka is a distributive streaming platform that enables the publication and subscription of streams of records in an error-tolerant and scalable way. Kafka is designed to handle large volume and high velocity data from multiple sources and to deliver them to multiple destinations. Kafka is a popular tool for AdTech companies to ingest, integrate, and deliver data in real-time like receiving, sending, and gathering ad requests, responses, events, metrics, and more.
To produce and consume data streams from Kafka using Java, we need to fol ow these steps: 1. De ne a Producer object that represents a Kafka producer that can send records to a Kafka topic. The Producer object al ows us to con gure various parameters for the producer, such as the bootstrap servers, the key and value serializers, the acks, the retries, etc. The Producer object also al ows us to create and send ProducerRecord objects, which represent the records to be sent to the Kafka topic. The ProducerRecord objects have various elds, such as the topic name, the partition number, the key, the value, the timestamp, etc.
2. De ne a Consumer object that represents a Kafka consumer that can receive records from a Kafka topic. The Consumer object al ows us to con gure various parameters for the consumer, such as the bootstrap servers, the key and value deserializers, the group id, the auto o set reset, etc. The Consumer object also al ows us to subscribe to one or more Kafka topics and pol for records. The pol () method returns a ConsumerRecords object, which represents a col ection of ConsumerRecord objects, which represent the records received from the Kafka topic. The ConsumerRecord objects have various elds, such as the topic name, the partition number, the o set, the key, the value, the timestamp, etc.
3. De ne a KafkaStreams object that represents a Kafka Streams application that can process and transform data streams from Kafka topics. The KafkaStreams object al ows us to con gure various parameters for the Kafka Streams application, such as the application id, the bootstrap servers, the default key and value serdes, the state directory, etc. The KafkaStreams object also al ows us to de ne and execute a Topology object, which represents the logical processing graph of the Kafka Streams application. The Topology object consists of various nodes, such as source nodes, processor nodes, sink nodes, etc., that are connected by streams, which represent the data ows between the nodes.
73
Here is an example of how to produce and consume data streams from Kafka using Java. The example simulates a simple ad exchange system that receives ad requests from publishers, sends ad responses to publishers, and records ad events from users.
The example uses three Kafka topics: ad_request, ad_response, and ad_event.
The ad_request topic contains the ad requests from publishers, which have the fol owing format: publisher_id,page_url,page_category,user_id,user_agent,user_ip
The ad_response topic contains the ad responses to publishers, which have the fol owing format: publisher_id,ad_id,advertiser_id,ad_creative,ad_url,ad_price
The ad_event topic contains the ad events from users, which have the fol owing format: user_id,ad_id,advertiser_id,event_type,event_time
The example has the fol owing classes:
// Import required classes
import org.apache.kafka.clients.producer.KafkaProducer;
import org.apache.kafka.clients.producer.Producer;
import org.apache.kafka.clients.producer.ProducerRecord;
import org.apache.kafka.clients.consumer.KafkaConsumer;
import org.apache.kafka.clients.consumer.Consumer;
import org.apache.kafka.clients.consumer.ConsumerRecords;
import org.apache.kafka.clients.consumer.ConsumerRecord;
import org.apache.kafka.streams.KafkaStreams;
import org.apache.kafka.streams.StreamsBuilder;
import org.apache.kafka.streams.StreamsConfig;
import org.apache.kafka.streams.Topology;
import org.apache.kafka.streams.kstream.KStream;
import org.apache.kafka.common.serialization.StringSerializer; import org.apache.kafka.common.serialization.StringDeserializer; import java.util.Properties;
import java.util.Arrays;
import java.util.Random;
import java.util.Date;
import java.text.SimpleDateFormat;
// Define Producer that simulates the ad requests from publishers public class AdRequestProducer {
// Define main method
74
public static void main(String[] args) throws Exception {
// Create Properties object
Properties props = new Properties();
// Set bootstrap servers
props.put("bootstrap.servers", "localhost:9092");
// Set key and value serializers
props.put("key.serializer", StringSerializer.class.getName()); props.put("value.serializer", StringSerializer.class.getName());
// Set acks
props.put("acks", "all");
// Set retries
props.put("retries", 0);
// Create Producer object
Producer<String, String> producer = new KafkaProducer<>(props);
// Create Random object
Random random = new Random();
// Create SimpleDateFormat object
SimpleDateFormat sdf = new SimpleDateFormat("yyyy-MM-dd HH:mm:ss");
// Define publisher ids
String[] publisherIds = {"101", "102", "103", "104", "105"};
// Define page urls
String[] pageUrls = {"https://www.example.com/news",
"https://www.example.com/sports", "https://www.example.com/entertainment",
"https://www.example.com/technology", "https://www.example.com/business"};
// Define page categories
String[] pageCategories = {"News", "Sports", "Entertainment",
"Technology", "Business"};
// Define user ids
String[] userIds = {"201", "202", "203", "204", "205"};
// Define user agents
String[] userAgents = {"Mozilla/5.0 (Windows NT 10.0; Win64; x64) AppleWebKit/537.36 (KHTML, like Gecko) Chrome/90.0.4430.93 Safari/537.36",
"Mozilla/5.0 (iPhone; CPU iPhone OS 14_4_2 like Mac OS X) AppleWebKit/605.1.15
(KHTML, like Gecko) Version/14.0.3 Mobile/15E148 Safari/604.1", "Mozilla/5.0
(Macintosh; Intel Mac OS X 10_15_7) AppleWebKit/537.36 (KHTML, like Gecko) Firefox/88.0", "Mozilla/5.0 (Linux; Android 11; SM-G998B) AppleWebKit/537.36
(KHTML, like Gecko) Chrome/90.0.4430.91 Mobile Safari/537.36", "Mozilla/5.0
(Windows Phone 10.0; Android 6.0.1; Microsoft; RM-1152) AppleWebKit/537.36
(KHTML, like Gecko) Chrome/52.0.2743.116 Mobile Safari/537.36 Edge/15.14977"};
// Define user ips
String[] userIps = {"192.168.0.1", "192.168.0.2", "192.168.0.3",
"192.168.0.4", "192.168.0.5"};
// Loop indefinitely
while (true) {
// Generate random publisher id
String publisherId =
publisherIds[random.nextInt(publisherIds.length)];
// Generate random page url
String pageUrl = pageUrls[random.nextInt(pageUrls.length)];
// Generate random page category
75
pageCategories[random.nextInt(pageCategories.length)];
// Generate random user id
String userId = userIds[random.nextInt(userIds.length)];
// Generate random user agent
String userAgent = userAgents[random.nextInt(userAgents.length)];
// Generate random user ip
String userIp = userIps[random.nextInt(userIps.length)];
// Generate a current timestamp
String timestamp = sdf.format(new Date());
// Create a record value by concatenating the fields with comma
String recordValue = publisherId + "," + pageUrl + "," + pageCategory
+ "," + userId + "," + userAgent + "," + userIp;
// Create a ProducerRecord object
ProducerRecord<String, String> record = new
ProducerRecord<>("ad_request", timestamp, recordValue);
// Send the record to the Kafka topic
producer.send(record);
// Print the record
System.out.println("Sent record: " + record);
// Sleep for one second
Thread.sleep(1000);
}
}
}
// Define a Consumer class that simulates the ad responses to publishers public class AdResponseConsumer {
// Define the main method
public static void main(String[] args) throws Exception {
// Create a Properties object
Properties props = new Properties();
// Set the bootstrap servers
props.put("bootstrap.servers", "localhost:9092");
// Set the key and value deserializers
props.put("key.deserializer", StringDeserializer.class.getName()); props.put("value.deserializer", StringDeserializer.class.getName());
// Set the group id
props.put("group.id", "ad_response_consumer_group");
// Set the auto offset reset
props.put("auto.offset.reset", "latest");
// Create a Consumer object
Consumer<String, String> consumer = new KafkaConsumer<>(props);
// Subscribe to the Kafka topic
consumer.subscribe(Arrays.asList("ad_response"));
// Loop indefinitely
while (true) {
// Poll for records
76
ConsumerRecords<String, String> records = consumer.poll(1000);
// Iterate over the records
for (ConsumerRecord<String, String> record : records) {
// Print record
System.out.println("Received record: " + record);
// Split record value by comma
String[] fields = record.value().split(",");
// Parse fields and extract publisher id and ad response
String publisherId = fields[0];
String adResponse = fields[1];
// Simulate sending ad response to publisher
System.out.println("Sending ad response to publisher " +
publisherId + ": " + adResponse);
}
// Commit the offsets
consumer.commitSync();
}
}
}
// Define KafkaStreams that simulates the ad exchange system that processes and transforms data streams from Kafka topics
public class AdExchangeKafkaStreams {
// Define main method
public static void main(String[] args) throws Exception {
// Create Properties object
Properties props = new Properties();
// Set application id
props.put(StreamsConfig.APPLICATION_ID_CONFIG,
"ad_exchange_kafka_streams");
// Set bootstrap servers
props.put(StreamsConfig.BOOTSTRAP_SERVERS_CONFIG, "localhost:9092");
// Set default key and value serdes
props.put(StreamsConfig.DEFAULT_KEY_SERDE_CLASS_CONFIG,
Serdes.String().getClass().getName());
props.put(StreamsConfig.DEFAULT_VALUE_SERDE_CLASS_CONFIG,
Serdes.String().getClass().getName());
// Set state directory
props.put(StreamsConfig.STATE_DIR_CONFIG, "/tmp/kafka-streams");
// Create StreamsBuilder object
StreamsBuilder builder = new StreamsBuilder();
// Create KStream object that represents ad request stream from the ad_request topic
KStream<String, String> adRequestStream = builder.stream("ad_request");
// Create KStream object that represents ad response stream to the ad_response topic
KStream<String, String> adResponseStream =
adRequestStream.mapValues(value -> {
// Split the value by comma
String[] fields = value.split(",");
77
// Parse fields and extract the publisher id, the page category, and the user id
String publisherId = fields[0];
String pageCategory = fields[2];
String userId = fields[3];
// Generate random ad id
int adId = new Random().nextInt(100) + 1;
// Generate random advertiser id
int advertiserId = new Random().nextInt(10) + 1;
// Generate random ad creative
String adCreative = "This is an ad for " + pageCategory;
// Generate random ad url
String adUrl = "https://www.example.com/ad/" + adId;
// Generate random ad price
int adPrice = new Random().nextInt(10) + 1;
// Create an ad response by concatenating the fields with comma
String adResponse = publisherId + "," + adId + "," + advertiserId +
"," + adCreative + "," + adUrl + "," + adPrice;
// Return the ad response
return adResponse;
});
// Send the ad response stream to the ad_response topic
adResponseStream.to("ad_response");
// Create KStream object that represents ad event stream from the ad_event topic
KStream<String, String> adEventStream = builder.stream("ad_event");
// Create KStream object that represents ad revenue stream to the ad_revenue topic
KStream<String, String> adRevenueStream = adEventStream.mapValues(value
-> {
// Split the value by comma
String[] fields = value.split(",");
// Parse fields and extract user id, ad id, advertiser id, event
// type, and the event time
String userId = fields[0];
String adId = fields[1];
String advertiserId = fields[2];
String eventType = fields[3];
String eventTime = fields[4];
// Define revenue factor for each event type
int revenueFactor = 0;
switch (eventType) {
case "impression":
revenueFactor = 1;
break;
case "click":
revenueFactor = 10;
break;
78
revenueFactor = 100;
break;
}
// Calculate revenue by multiplying the revenue factor and the ad price
int revenue = revenueFactor * Integer.parseInt(adId);
// Create an ad revenue by concatenating the fields with comma
String adRevenue = userId + "," + adId + "," + advertiserId + "," +
eventType + "," + eventTime + "," + revenue;
// Return ad revenue
return adRevenue;
});
// Send ad revenue stream to the ad_revenue topic
adRevenueStream.to("ad_revenue");
// Create Topology object
Topology topology = builder.build();
// Create KafkaStreams object
KafkaStreams streams = new KafkaStreams(topology, props);
// Start KafkaStreams object
streams.start();
// Add a shutdown hook to stop the KafkaStreams object
Runtime.getRuntime().addShutdownHook(new Thread(streams::close));
}
}
79
3.5 How to connect and query Cassandra using Java The Cassandra database is a distributed one which ensures high availability and scalability for the huge data volumes with semi-structured and structured formats. Cassandra is a column-based store that does not impose columnar layout on data; it al ows for dynamic and varying data modeling.
The most widely used technology in AdTech is Cassandra which is used for storing and querying ad related data.
To connect and query Cassandra using Java, we need to fol ow these steps: 1. Add the dependency for the DataStax Java driver for Apache Cassandra to our project. The DataStax Java driver is a high-level API that al ows Java applications to communicate with Cassandra clusters. If we are using Maven, we can add the fol owing dependency to our pom.xml le:
<dependency>
<groupId>com.datastax.oss</groupId>
<artifactId>java-driver-core</artifactId>
<version>4.12.0</version>
</dependency>
2. Create a Cluster object that represents the Cassandra cluster we want to connect to. The Cluster object al ows us to con gure various parameters for the connection, such as the contact points, the port, the load balancing policy, the retry policy, etc. We can use the Cluster.builder() method to create a Cluster object and set the parameters. For example, we can create a Cluster object that connects to a local Cassandra cluster running on port 9042
with the fol owing code:
Cluster cluster = Cluster.builder()
.addContactPoint("127.0.0.1")
.withPort(9042)
.build();
3. Create a Session object that represents the connection to the Cassandra cluster. The Session object al ows us to execute queries and statements on the Cassandra cluster. We can use the connect() method of the Cluster object to create a Session object. For example, we can create a Session object that connects to the default keyspace with the fol owing code: Session session = cluster.connect();
80
4. Execute queries and statements on the Cassandra cluster using the Session object. We can use various methods of the Session object to execute queries and statements, such as execute(), executeAsync(), prepare(), prepareAsync(), etc. We can use the SimpleStatement class to create simple queries and statements, or the PreparedStatement class to create prepared queries and statements. For example, we can create and execute a simple query that selects al the data from a table cal ed ad_data with the fol owing code: SimpleStatement statement = new SimpleStatement("SELECT * FROM ad_data"); ResultSet resultSet = session.execute(statement);
5. Process the results of the queries and statements using the ResultSet object. The ResultSet object represents a col ection of rows returned by the queries and statements. We can use various methods of the ResultSet object to process the results, such as al (), one(), iterator(), isExhausted(), wasApplied(), etc. We can use the Row class to access the data in each row.
For example, we can iterate over the rows in the ResultSet object and print the data with the fol owing code:
for (Row row : resultSet) {
System.out.println(row.toString());
}
6. Close the Session and the Cluster objects when we are done with the connection. We can use the close() method of the Session and the Cluster objects to close the connection and release the resources. For example, we can close the Session and the Cluster objects with the fol owing code:
session.close();
cluster.close();
This is how we can connect and query Cassandra using Java.
81
4 Security Considerations in AdTech Development
Safety takes the rst place among others in the advertising technology. Al three components – namely advertising companies, publishers, and users- depend on the authenticity of the advertisement server. The security topics elaborated in this chapter include the applicable security measures as we explore Java’s AdTech development. We are going to discuss the fol owing areas of specialization: data privacy, compliance, secure communication, and techniques for anti-fraud campaign and safety measures.
4.1 Data Privacy and Compliance in AdTech
4.1.1 Protecting User Data
The data from users forms the basis of targeted ads in the AdTech sector. Advertisers and publishers stand on di erent data bases such as demographic data, search history and behavioral data to structure personalized advertisements for the target customer. While on the one hand, enterprises strive to col ect data in order to gain understanding of customer needs, on the other hand, security of user data has become a priority issue with data privacy concerns and data breach.
Anonymization and Pseudonymization
Java professionals who work in AdTech must possess a thorough understanding of data anonymization as wel as de-identi cation methods. Pseudonymization and information replacement is the techniques in which the PII is either deleted or substituted in such a way that even if the data is leaked, there would be no way of traced back to particular users.
Data anonymization refers to the act of stripping o data of identi ers that can directly identify a person by name and e-mail address among others. Say, just for one example, you don't store user's name, but his/her identi er which is linked to that user. The protection of the sensitive information is maximized since the risk of exposing it during a security attack is eliminated.
Anonymization is one method that replaces PII with an irreversible token that ensures unidenti ability, whereas pseudonymization is similar to anonymization, but with a reversible token or pseudonym. It wil provide a solution to some AdTech processes like ad targeting, but at the same time wil give a person control of personal data. Java is known to provide links which developers can and do use to implement techniques to anonymize their data as wel such as using pseudonyms.
82
The fundamental concept of "privacy by design" is one of the most basic rules in AdTech's development. It is based on the principle of privacy by design by considering privacy issues throughout each step of the development process from the prototype design to the operations and after deployment. Security requirements in Java development need to go beyond a traditional attitude of bolting on safety features as an afterthought. The system components that consider the mandated security levels must be an integrated part of the system.
When implementing privacy by design, consider adopting the fol owing principles: Data Minimization: Col ect only the data that is necessary for the intended purpose. This reduces the amount of sensitive information that needs to be protected.
User Consent: Obtain explicit consent from users before col ecting and processing their data.
Implement mechanisms for users to withdraw their consent at any time.
Data Encryption: Use strong encryption techniques to protect data both in transit and at rest.
Java provides robust encryption libraries to secure data e ectively.
Privacy by design also involves conducting privacy impact assessments to identify potential risks and vulnerabilities related to data privacy and security throughout the development lifecycle. By systematical y addressing privacy concerns, AdTech developers can build trust with users and ensure compliance with data protection regulation.
83
Consent Management Platforms (CMPs)
To maneuver the complex data privacy regulations such as GDPR and CCPA, AdTech solutions leverage Consent Management Platforms (CMPs). These platforms enable users to give or cancel consent easily and control their data preferences.
Developers in Java can incorporate CMPs in their systems to ensure the users about the usage of their data in the eld of advertisement. CMPs in compliant with regulations because they register the user’s consent and o er the user hands-on mechanisms to exert his or her rights regarding data privacy.
CMPs o er several key features:
Consent Recording: CMPs record user consent choices, including the type of data that can be col ected and the purposes for which it can be used. This information is crucial for compliance and transparency.
Consent Revocation: Users should have the ability to revoke their consent at any time. CMPs facilitate this process, ensuring that user preferences are respected.
Consent Logs: CMPs maintain logs of consent changes and user interactions, which can be invaluable in demonstrating compliance during audits or investigations.
By integrating CMPs into AdTech solutions, developers can streamline compliance e orts and provide users with a transparent and user-friendly way to manage their data preferences.
84
Consent mechanisms are critical within the AdTech realm. They serve to help the users understand how the data should be used in respect to promotion. Robust consent mechanisms, in addition to providing compliance with privacy legislations, create trust between users and AdTech platforms.
Granular Consent
The Java developers should design a exible system that wil al ow certain enough granular consent options. This implies that users must have the option to determine which categories of information they approve sharing and to identify the areas where they would like to keep their data for themselves. Take, for example, a case where an individual would approve of the usage of demographic data for ad targeting but would refuse to provide location data in return.
To the users though, granular consent is the power to have ful and sweeping control over how their data is handled, therefore, they wil be able to have al their doubts be washed away by the trust the AdTech platforms have in them. Java apps is capable of giving access to user interface which is very clear in presentation of group of data categories and purposes that helps users to personalized the preferences.
Transparency
Transparency is the ultimate factor that a ects the majority of users’ trust. Systems of AdTech should strategical y convey the data transactions clearly and precisely. Java programs have the ability to display the corresponding user oriented consent dialog which elaborates the plain language by submitting how data wil be operated, and therefore people are now empowered to make correct choice.
Besides stating the matter precisely, the transparency sense includes also giving a chance for the user to read privacy policies, data retention policies and about third-party data sharing. The Java developers' responsibility should be to provide easy access to the information supplied by the third-party Ad Server through the applications they built for AdTech.
Consent Management Interfaces
The consent management function can be implemented in AdTech applications using Java which leads to easy-to-use interfaces. These interfaces supply users with the opportunities to review and correct their consent options as often as they want. Users should have the ability to change preferences, revoke consent or delete their data at any time according to the in the data.
Consent management interfaces should be user-friendly and navigate, since these interfaces are created to assist users in managing their data preferences. In addition, they should give users a choice of either subscribing to alerts or noti cations about changes made to data col ection rules or privacy policies.
85
Through opting for granular consent, transparency and usability through the interface, Java developers build up the AdTech applications that comply with data privacy regulations as wel as respect the users' options and gain the trust of the ad ecosystem.
Consent Auditing and Reporting
The AdTech systems also have to elaborate on consent auditing and reporting for the sake of observance of data privacy regulations. Java developers can make the implementation of this feature to note and log the user consent and data processing activities possible.
Auditing: Implementing auditing mechanisms al ows AdTech platforms to record consent events, data processing activities, and changes in user preferences. Audit logs provide a trail of evidence that can be used to prove compliance during regulatory audits or investigations.
Reporting: Reporting features enable AdTech platforms to generate compliance reports that summarize consent data, data processing activities, and adherence to privacy policies. These reports can be invaluable in demonstrating accountability to regulatory authorities and stakeholders.
Javàs adaptability implies that it can design an advanced audit and reporting system. Developers can rely on established libraries and frameworks in order to e ciently accommodate the consent-related data and generate reports on compliance.
Data Subject Rights
Data privacy regulations grant individuals certain rights regarding their personal data. AdTech developers should be aware of these rights and provide mechanisms for users to exercise them.
Some of the key data subject rights include:
Right to Access: Users can request access to their personal data that AdTech platforms have col ected. Java developers can create interfaces for users to make these requests and retrieve their data.
Right to Recti cation: Users have the right to correct inaccurate or incomplete personal data.
Java applications can include functionality for users to update their information.
Right to Erasure (Right to Be Forgotten): Users can request the deletion of their personal data.
AdTech systems developed in Java should have processes in place to handle data deletion requests.
Right to Data Portability: Users can request a copy of their personal data in a structured, commonly used, and machine-readable format. Java developers can build export features to facilitate data portability.
Right to Object: Users can object to the processing of their personal data, particularly in cases of direct marketing. AdTech platforms should provide mechanisms for users to opt out of data processing for speci c purposes.
Automated Decision-Making and Pro ling: Some regulations restrict automated decision-making and pro ling without user consent. Java developers should implement controls to ensure compliance with these restrictions.
86
AdTech systems must have processes and interfaces in place to accommodate these data subject rights. Java's exibility al ows developers to create user-friendly interfaces for users to exercise their rights and for AdTech platforms to respond promptly and appropriately to these requests.
Cross-Border Data Transfers
The transfer of data back and forth across borders is one of the key features of AdTech systems.
Regarding international data transfers, developers ought to consider data protection laws and regulations not only than the source country but also that in the destination country.
One of the most commonly employed mechanisms for granting legitimacy to international data transfer is the incorporation of Standard Contractual Clauses (SCCs) or the usage of Binding Corporate Rules (BCRs). Java applications can be designed with consideration to these procedures so that they are compliant with global data protection law.
Furthermore, developers should understand data localization’s requirements that might dictate where speci c data processing can take place. Compliance with the data localization mandate may include certain architectural considerations which may necessitate using speci c Java-based tools and services.
4.1.3 Conclusion
Privacy and compliance, which are an important basic of developing responsible AdTech are two of fundamental elements. Developers are an indispensable component in guaranteeing that AdTech platforms respect the privacy of the users, fol ow regulatory and legislative requirements, and strive to maintain the trust of the users and other stakeholders.
By applying strict data anonymization and pseudonymization techniques, fol owing the principle of privacy by design, integrating CMPs for stamping the user consent and the data sharing mechanisms, creating a very transparent and user friendly interface, Java developers can build AdTech solutions that not only meet the legal obligations but also the user privacy and con dentiality.
In the next section, we are going to delve into the ways to build secure communication channels in AdTech systems developed using Java. The security feature and encryption of data make it more secure when it operates between various components of AdTech and across networks, ensuring the proper security and compliance of the entire AdTech solutions.
87
4.2 Implementing Secure Communication in Java
As far as information security is concerned, encrypted communication is a must-have feature, particularly if the data is private. Whether it's a banner impression, user data, or a bid, the modi cation and privacy when being sent become the key elements. Java imparts the tools that are able to ensure safe transit of information within the AdTech ecosystems, and with that the data is protected.
4.2.1 Secure Sockets Layer (SSL) and Transport Layer Security (TLS) Use of protocols such as SSL and TLS becomes a critical role in the process of securing overal communication in AdTech. These protocols thus, guarantee the protection of messages were maybe exchanged or maybe passed from one system to another is encrypted or kept intact and private throughout the transmission of the data. Java uses data encryption mechanisms (SSL/TLS) to build a solid cryptography background that could be used for integrated secure communication.
88
4.2.2 Authentication and Authorization
Authentication and authorization procedures are the key elements enforcing the security of the communication. By doing this, they enable the systems to enact access control, so that only authorized users and systems can get access to the sensitive data. The Java language encompasses a variety of ways of implementing the proposed mechanisms.
Authentication: Implementing robust authentication process with these libraries is possible for the entire team, so they don't want to create their own security system. To implement these security measures, authentication methods are general y based on either username-password authentication, token-based authentication or certi cates and public-key infrastructure (PKI).
Simple authentication tokens are the types where users and system obtain secure tokens after a successful login. Therefore, this token wil enable users to employ them for protected assets by excluding the need of providing credentials in every request. It heightens the level of security and the password exposure is minimized. Write the best words you can and be wil ing to share them with others.
Certi cate of this type and PKI-based authentication presents high security level as they use trustable authorities' issued digital certi cates. Java shows us the process of validating the certi cates, which wil make sure that the identity of the communicating parties is true.
Authorization: Authorization provides speci c control over AdTech resource or functions in a system by limiting access or authorizing speci c instances. Java developers can use role-based access control (RBAC), or attribute-based access control (ABAC), to decide the particular actions authorize under certain attributes.
RBAC systems identify roles within a system, and users or systems are then assigned roles that de ne privileges of accessibility to certain features. Consequently, ABAC which adjusts attribute includes (e.g., user attributes, request parameters) and determines access rights dynamical y. It o ers more focused control over access, thus bugs less.
The integration of ne-grained authorization in the context of AdTech denotes one of the measures which aims to safeguard information on the set of critical functions and prevent unauthorized access.
89
4.2.3 Securing APIs and Endpoints
In AdTech, APIs act as a central communication channel through which data is exchanged between di erent components. Not only must endpoints and APIs be adequately secured, but unauthorized access must also be prevented to ensure data security. Java provides an arsenal of tools and techniques for handling exceptions.
OAuth and OpenID: OAuth and OpenID Connect are widely adopted protocols for securing APIs and enabling single sign-on (SSO). Java developers can leverage OAuth and OpenID Connect libraries to implement robust authentication and authorization mechanisms for their APIs.
OAuth al ows applications to obtain access tokens, which can be used to access protected resources on behalf of a user or system. OpenID Connect builds on OAuth and adds identity veri cation capabilities. These protocols are especial y useful when building AdTech platforms with multiple integrated services and user interactions.
API Key Management: Ending up with API key management systems is a good way to prevent breaches in APIs and endpoints. API keys are the same as the keys that enable access to the APIs or endpoints that are speci c. Through creating and automating the use of API keys, developers secure the access to their APIs and keep track of the key usage.
Another feature of API key management solutions which incorporate rate-limiting is that prevents misuse of APIs by imposing a limit on the number of requests that can be made from a singular source. Rate limiting plays the essential role to keep the stable API online and to decrease the API performance deterioration.
JSON Web Tokens (JWT): JSON Web Token is a lightweight self-contained token bearer that can be used to sign and validate the statements that consist the user or system authorization. The Java programming language al ows the developers to use JWT tokens to verify the accuracy of API requests and also ensure that the cal er of the request has the rightful permissions.
Stateless authentications are strongly based on the use of JWTs. JWTs can be used to avoid storing the session data whenever the customers input their usernames and passwords to verify their identity. Such data records imply the target users’ and systems’ identi cation and permission claims. Go java libraries for JWT valiation enable one to check the integrity of the tokens by making sure that they have not been edited.
It is crucial to bolster API and endpoint security in AdTech systems to guarantee data con dentiality and to avoid unauthorized access to the data. Having various authentication and authorization means available and ability to use third-party libraries makes it possible for Java developers to create a security opus that lies at the heart of their APIs.
90
4.2.4 Security Audits and Penetration Testing
Performing ongoing security audits and penetration tests is strongly recommended to discover vulnerability points and de ciencies of AdTech systems. Java applications can be subjected to thorough testing making them fault less and stick to the security best practices.
Security Audits
Security audits performed in this manner, review the architecture, code, and con gurations of al AdTech systems, in-search for any security-related vulnerabilities. Col aboration between Java developers and security experts via regular audits and problem solving for any issues arising there likewise should be the case.
Key areas of focus during security audits include:Key areas of focus during security audits include: Code Review: Developing the code for inevitability of loses like injection attacks, misuse of user inputs, and insecure nature.
Architecture Assessment: Reviewing the total architecture for an innundate of attack paths, data movements, and security loopholes.
Con guration Checks: Tackling miscon gurations for security, for instance access controls being set too permissively or connections not being encrypted and so on.
Penetration Testing
Penetration testing, also referred to as as ethical hacking, is a type of attack that is aimed at discovering system vulnerabilities. Aiming to simulate the behavior of malicious actors, seasoned security experts watch to see if the implemented defenses fail and, hence, they provide information about enhancement for them.
Network Vulnerabilities: Identifying the a rmation of the weaknesses in network con gurations, rewal rules and intrusion detection systems.
Web Application Vulnerabilities: Registering accumulation of secure web applications, involving examination for SQL injection, XSS (cross-site-scripting), and also other frequent vulnerabilities.
Authentication and Authorization Testing: Analyzing the performance of authentication and authorization methods that have been used, including the ways to dodge them.
API Security Assessment: To verify the security of APIs and endpoints the assessment of existing vulnerabilities is required.
Developers should col aborate with certi ed penetration testers to perform these assessments.
Penetration testing helps uncover vulnerabilities that might not be apparent in code reviews or architecture assessments and al ows for targeted remediation.
91
4.2.5 Regular Updates and Patch Management
The IT infrastructure of an AdTech system may have vulnerabilities in software components or libraries used in the system that are discovered from time to time. Java programmers are always at risks for security breaches and thus need to be more vigilant concerning their Java runtime environments and dependencies by keeping them updated at al times.
Java Updates: Java providers release regular updates with the security patches and bug xes integrated in it. It is imperative to carry out these xes in a timely manner to prevent the public from being exploited by the known vulnerabilities. Moreover, programmers can adhere to secure JVM coding practices by setting security policies and implementing the right privileges.
Dependency Scanning: AdTech applications often rely on third-party libraries and components.
Java developers should use dependency scanning tools to identify and track vulnerabilities in these dependencies. Regularly monitoring for security advisories related to used libraries al ows developers to take timely action, such as updating or replacing vulnerable components.
Incident Response Plan
Preventative approaches are not the only important and one should be ready for emergency events.
Java developers need to create a contingency plan as the rst step towards reacting to security incidents quickly and e ectively. The plan should go through the process of identifying and con ning incidents, information of a ected parties, and keep up with the recovery from the security breaches.
Security vulnerabilities are addressed proactively, systems and dependencies are up to date, and incident response plan is cohesive, al of these measures help to strengthen the security posture of an AdTech systems and protect the data from spying.
4.2.6 Conclusion
Emplacing secure communication is a vital part for furthering the AdTech development world.
Java in terms of the built-in support for the SSL/TLS protocols, authentication methods, and also authorization mechanism, can be regarded as having a lot power in building a secure system for AdTech solutions.
Current consideration is regular audits and checks, penetration testing and timely patching of applications being fundamental to the security of AdTech services. Through securing the codes from the beginning, Java developers can preserve al con dentiality in a ected data and can create a trustworthy relationship with other people who are directly involved.
In the next section, we wil explore strategies for preventing ad fraud and security threats within the AdTech ecosystem.
92
4.3 Preventing Ad Fraud and Security Threats
Ad fraud and cyber security are major hurdles for the AdTech industry and can lead to issues like brand safety, malware propagation, and nancial loss. Fake clicks, impressions, and even ad views by fraudsters lead to a loss of bil ions of dol ars which in turn hurts both advertisers as wel as publishers, while the user privacy is compromised in the event of security breaches. The impact of cybercrime in Java development for AdTech sector prompts Java developers to come up with a defensive approach in order to save their companies from the unlawful tactics being used by the cybercriminals.
4.3.1 Understanding Ad Fraud
Ad fraud incorporates a variety of the mockeries focused on the systems of digital advertisement. It is done in order to get income. Some common types of ad fraud include:Some common types of ad fraud include:
Click Fraud: Fraudsters, by clicking on advertisers's e-ads, make falsi ed views of these ads and thus in ate the charges of advertisers.
Impression Fraud: Sites or Microbots reporting fake ad impressions increase ad income to fraudsters
Ad Injection: The software of bad character adds ads to websites without permission and not good.
Domain Spoo ng: The forgers use the source of an ad to a genuine domain that make them to be regarded as a genuine ad.
4.3.2 Recognizing Security Threats
Security breaches in AdTech may have signi cant consequences from spoilt software and compromised data of users.Common security threats include:
Malware Attacks: Imminent threats to AdTech systems could be viruses that hack into the infrastructure and infringe upon the integrity of data.
Data Theft: The malicious actors may try to obtain the user information that comprises the personal data (Personal y identi able information, PII).
Distributed Denial of Service (DDoS) Attacks: Adversaries in cyber space bury the target infrastructure in the tra c overwhelming it and thus causing service disruptions.
Phishing Attacks: Such emails and websites for the purpose of il egal y obtaining private accounts and personal information exist.
93
Java developers in the AdTech industry can employ various strategies and best practices to prevent ad fraud and security threats e ectively.
Fraud Detection and Prevention Tools: Putting in place proven fraud detection and prevention systems is indispensable. Such tools exploit the pattern recognition and machine learning algorithms to detect carry out and block fraudulent activities. Java developers may use these tools to implement AdTech environment where ad fraud is easily identi able and blocked in real-time.
Ad Veri cation Services: Leveraging ad veri cation services helps ensure that ads are displayed on reputable and brand-safe websites. These services analyze ad placements to verify their legitimacy.
Java developers can integrate ad veri cation APIs into AdTech systems to validate the quality and authenticity of ad placements.
Bot Detection and Mitigation: Bots are a signi cant source of ad fraud and security threats. Java developers can implement bot detection and mitigation techniques to distinguish between legitimate users and automated scripts. Captcha chal enges, behavioral analysis, and device ngerprinting are common methods used to detect and mitigate bot activity.
User Behavior Analysis: Through the use of behavioral analysis, you can detect abnormalities resulting from fraud. Developers have the ability to create algorithms which let them analyse user interactions and they also detect unusual patterns like high click-through rates or quick ad impressions. Deviations from the norm of everyday user behavior can indicate the raise of alerts for investigation.
Secure Ad Tags and Headers: Ad tags and headers are commonly used to deliver ads to websites and applications. Java developers should ensure that ad tags and headers are implemented securely to prevent tampering or injection of malicious code. Implementing content security policies (CSP) and validating ad tags at runtime can help protect against ad injection attacks.
Data Encryption and Access Controls: Protecting sensitive data is paramount. Java developers should implement strong data encryption techniques for data at rest and in transit. Additional y, access controls should be enforced to restrict unauthorized access to user data and AdTech systems.
Regular Security Audits: Periodical security audits and vulnerability assessments are fundamental in discovering and rectifying the weak spots of security. Java developers are recommended to exploit ful - edged code reviews and security scans to precautionary identify and mitigate the vulnerabilities.
Incident Response Plan: The essential point is that you need to make arrangements for security incidents. Developer should arrange for an Incident Response plan, that documents measures for detection, mitigation and recovery of security vulnerabilities. Implementing a professional plan can lead to a high-level of protection against security problems.
94
4.3.4 Industry Standards and Regulations
AdTech professionals should stay informed about industry standards and regulations related to ad fraud prevention and data security. Familiarity with guidelines such as the Interactive Advertising Bureau (IAB) standards and data protection regulations like GDPR and CCPA is essential for compliance and best practices.
4.3.5 Conclusion
Ad fraud defense and ad security are among key priorities that require AdTech industry players to remain vigilant, proactive and wel -responsive. The Java developers wil certainly be indispensable in processing security best practices , fraud detection tools and though to educate the users but this role wil be aimed at ensuring the security of the digital advertising and user privacy.
Through learning about potential risks, abiding by the set standards, working with other players in your industry, and devoting security to your products' life cycle, AdTech security is successful y achieved by java developers.
4.4 Conclusion
Security limitations remain an indispensable factor in AdTech creation and are not negotiable.
Developers of Java wil be able to solve security concerns through priority data ow, secure communication as wel as developing the security system and preventing ad fraud by accepting to assist. This chapters wil deal with how to optimize performance, reliability of testing and outcome quality for AdTech applications.
In Chapter 5, we wil explore strategies for optimizing the performance of AdTech applications developed in Java, ensuring that they deliver ads e ciently and at scale.
95
5 Optimizing Performance in AdTech Applications
Implementing the e ciency of AdTech apps is crucial with the dynamic application of advertising technology (AdTech). Advertisers and publishers use the apps in the rst place to e ciently reach their target audiences with advertising. This section discusses the di erent strategies and techniques that can be used by Java developers to be at the top in the AdTech applications performance.
5.1 Pro ling and Performance Tuning in Java
Performance optimization is a pivotal aspect of developing robust and e cient AdTech applications. In the dynamic and competitive landscape of advertising technology (AdTech), where mil iseconds matter and user experience is paramount, Java developers face the constant chal enge of ensuring their applications run at peak performance. This section delves deep into the critical practices of pro ling and performance tuning in Java, empowering developers to identify bottlenecks, enhance e ciency, and deliver advertisements swiftly and e ectively to target audiences.
5.1.1 The Importance of Performance Optimization
In the AdTech ecosystem, performance optimization is not merely a desirable feature; it is a necessity. Advertisers and publishers rely on AdTech applications to deliver ads seamlessly, and users expect swift and unobtrusive ad experiences. In this context, performance optimization encompasses a range of practices aimed at maximizing the e ciency of AdTech systems.
96
5.1.2 Pro ling: Gaining Insights into Application Behavior Pro ling is the systematic analysis of an application's behavior with the aim of identifying performance bottlenecks and areas that require improvement. Java developers have at their disposal a set of powerful pro ling tools, each designed to provide speci c insights into an application's performance and resource utilization.
Pro ling Tools
VisualVM: VisualVM-the part of JDK which is yours for free as wel -is a powerful and clean tool for in-pro ling tasks. In addition to a powerful feature set (e.g., CPU and memory pro ling, thread analysis, and heap dump analysis), it serves as an alternative to popular debuggers with a built-in pro ler and disassembler, with more capabilities. VisualVM is an integrated tool that may be the right way to start Java development activities for developers who need to know more about the applications.
YourKit: YourKit is a,comercial Java pro ler, with the up-to-date pro leothe worlds. This pro ler is known for it low overhead and advanced pro ling capabilities. It has features that al ow developers to grab total CPU and memory usage. The function of it makes its usage in this regard highly important. It can aid in the speedy resolving of performance problems.
Java Mission Control: Java Mission Control (JMC) in addition to Oracle, is another useful tool for evaluation of Java applications. JMC provides in-depth views of what goes on during a program's execution. It stands for features that is for monitoring, diagnosing, and pro ling the system problems so as for programmers to be able to have thorough comprehension regarding the source of bottlenecks.
Applications resource consumption, execution ow, and memory usage, can be pro led with VisualVM, YourKit, and Java Mission Control tools, which aids developers to visualize the tools.
Armed with the acquired knowledge, the developers are able to determine certain areas that need xing and improvements.
Pro ling Work ow
E ective pro ling requires a structured approach:E ective pro ling requires a structured approach:
Pro ling Preparation: Greater e ect is created before a performance evaluation by creation of a clear objectives and parameters. Ascertain what it is that your application needs and check what pro ler system works best for your needs.
Pro ling Execution: Create the new pro le task and link it to the ongoing application. The virtual theatre performance you intend to examine is embedded in the reality of life; how true to life is it? This can be done while emulating user behaviours, ad deliveries, or other fundamental procedures within the developed AdTech application.
97
Analysis and Optimization: Pro le the results: for performance teddy bears. These stal s can manifest as many ways: robust CPU usage, memory leaks, threading issues, and slow database queries. You must identify each bottleneck, and then take immediate remedial action to make it work.
5.1.3 Performance Tuning: Strategies for Optimization
Pro ling is the rst step in the journey towards performance optimization. After identifying bottlenecks, Java developers can employ various performance tuning techniques to enhance the e ciency of AdTech applications.
Memory Optimization
E cient memory management is an essential component of performance improvement. In AdTech applications, where heavy data processing takes place, the ability to manage memory wel is crucial. Developers should consider the fol owing memory optimization techniques: Minimize Unnecessary Object Creation: In Java, the object creation on every instance is an act of memory and CPU usage. Do not clutter the program with items which may be repeated within loops and/or frequently executed code objects. Use reuse instead of create and destroy new objects or apply object pooling mechanisms to decrease the additional load on process.
Employ Object Pooling: With the object pooling, the pre-initialized objects are kept in an object pool rather than being made new each time. The AdTech apps in this context are not to create new objects; they can instead borrow objects from the shared pool and then they should return those objects when they are not needed anymore. This behavior means the overhead on object creation and destruction is reduced, however, as it is more memory e cient.
Optimize Data Structures: Selecting the correct data structures assures memory footprint that is unobtrusive to the code. Such as HashMaps, in which the average cost of an operation is constant, are suitable anytime you want to obtain a constant-time access. Among these ones, HashMaps and HashSets might be the best choice when you need a constant time. Consequently, it can be also chal enging to use complicated data types, because they are known to be bigger than primitive ones with fewer memory al ocation areas.
Thread Management
E cient multithreading is essential for AdTech applications that must handle concurrent requests and processing. Java developers can employ various strategies for e ective thread management: Leverage Thread Pools: Thread pools are pivotal idea in threaded programming, because without them it would be impossible to run multiple threads. This is the feature that enables the developers to manage a xed amount of threads so that, while start- ing new threads more e ciently, their running is made much better, as wel . Applying thread pool approach, AdTech applications al ow 98
the threads to pre - determine the number of raw threads to handle the requests without the extra cost of constantly creating and kil ing threads.
Implement Asynchronous Processing: Asynchronous processing is speci cal y important in AdTech, where some jobs may require a greater time to be completed (for instance, data gathering from external sources, or complex computations). AdTech applications can continue to run other tasks while waiting for the completion of time-consuming operations, thanks to asynchronous programming techniques. Thus, this approach makes the product more exible.
Avoid Excessive Thread Contention: Thread contention is the phenomenon when many threads simultaneously want to access a concurrent shared resource, which leads to possible performance slowdown. On the part of AdTech applications, ubiquity of rivalry comes into being, when number threads is trying to use the shared data in a paral el manner. One of the ways in which developers could iron out contention is through the means of techniques such as accurate locking, thread safe data structures, or resorting to thread-safe alternatives.
Caching Strategies
Caching is a fundamental technique in performance optimization, especial y in AdTech applications. E ective caching reduces the load on databases, ad servers, and external services while ensuring rapid access to frequently requested data. Java developers can employ various caching strategies tailored to the unique demands of AdTech platforms
Database Optimization
Databases are a fundamental entity of most AdTech applications, as they mainly store campaign inventory, audience pro les, and data of the marketing campaign. It is vital to optimize the databases for enhancing the system e ciency. Strategies to optimize database performance: Fine-Tune Database Queries: Database queries should be ne-tuned for e ciency.
1. Ensure that queries are properly indexed to accelerate data retrieval.
2. Analyze query execution plans to identify performance bottlenecks.
3. Consider denormalization for speci c use cases to reduce the complexity of queries.
By optimizing database queries, AdTech applications can deliver ad content and user-speci c information swiftly.
Utilize Indexing: E ective use of indexing is key to fast data retrieval. Developers should: 1. Identify columns frequently used in WHERE clauses and JOIN operations and create indexes on them.
2. Be mindful of the trade-o between read performance and write performance when adding indexes.
Wel -placed indexes signi cantly improve the speed of data retrieval, bene ting ad delivery and user targeting.
Consider NoSQL Databases: For certain use cases, NoSQL databases can provide advantages over traditional relational databases. NoSQL databases, such as MongoDB, Cassandra, or Redis, 99
are designed to handle large volumes of data with high write and read throughput. AdTech applications can bene t from NoSQL databases when dealing with real-time data processing, user pro les, and ad serving.
Optimize Connection Pooling: E cient connection pooling is essential for AdTech applications that interact with databases. Developers should:
1. Con gure connection pools to ensure an optimal number of connections are available.
2. Implement connection pool monitoring to detect and address connection leaks.
Wel -optimized connection pooling strategy ensures that AdTech applications can establish and manage database connections e ciently.
100
5.2 Caching Strategies for AdTech Platforms
Caching is a mainstay of performance tweaking with AdTech applications. Through cleverly caching data and assets, Java developers can drastical y reduce the load on databases, ad servers, and external sources, while providing accelerated fetching of frequently requested information. This part shows the multifaceted caching techniques that are crafted to accommodate the speci c requirements of AdTech platforms, and hence developers can serve their ads faster and adequately.
5.2.1 E ective Caching Strategies
AdTech caching plays the mission reducing the latency, and speeding up the response of applications. E cient caching ensures these procedures – from delivering ad content to personalizing user data – in AdTech. Here are some e ective caching strategies for AdTech platforms.
Ad Content Caching
In the sphere of Advertising Technology (AdTech), ad creatives and visuals such as pictures and multimedia impact the e ectiveness of the advertisements. Caching these assets can signi cantly reduce latency in ad rendering and enhance user experience:
Image and Multimedia Caching: Image and Multimedia Caching: Caching frequently used ad creatives, images, and multimedia elements can be done for better performance. Basical y, this helps to fetch the set of assets from external servers just once. This in turn guarantees that ads are delivered quickly and smoothly without users needing to wait for ad loading to be done.
HTML and JavaScript Caching: For ad creatives, these usual y contain HTML templates and JavaScript libraries. These parts are cached so they are easily rendered in ad cal requests. AdTech applications diminish latency in loading these ancil ary materials thereby enhancing seamless ad viewer experience.
User Data Caching
Personalization is among the key elements of digital advertising which is designed to inform the users about the products and services they need or have the highest demand through displaying of ads which are targeted based on their preferences and behavior. Caching user-related data is instrumental in achieving this goal:
User Pro le Caching: AdTech utilizes user pro le caching and preference storage as a key approach to monitor online user activity. In this way, AdTech apps can avoid having to make queries to their databases repeatedly to personalize the advertising exposure. Targeted user 101
information stored as caches improves the relevance of advertising and better tailors it to audiences, thus increasing the e ciency of the ads.
Session Data Caching: RTB and ad auction processes require participants to transfer essential data with regard to Big Data, for example, session-related data, including bid requests and responses. Caching session data also cuts burden to backend servers and databases, thereby reducing the strain faced by the real-time processing infrastructure. E cient handling of a high number of concurrent bidding operations in AdTech platforms is based on the optimization, which is critical to e ectively manage complicated bid scenarios.
Ad Inventory Caching
E cient ad inventory management is essential for AdTech platforms that serve ads across various channels and formats. Caching ad inventory data accelerates the ad selection and placement process. Ad inventory data includes information about available ad slots, targeting criteria, and pricing. Caching this data al ows AdTech platforms to make ad placement decisions swiftly.
Whether it's selecting the right ad for a speci c user or determining optimal ad positioning, ad inventory caching streamlines the decision-making process.
Real-Time Bidding (RTB) Caching
The decision making process in RTB is performed instantaneously via an on-the- y response from the demand-side platforms (DSPs) and ad Exchanges. Temporal storage of bid responses is crucial as one of the techniques to enhance the speed of exchanges in Real-time auction bidding. In the case of RTB, ads spending progress reports are kept in browser memory. This has let the bidding strategy save the time required for processing bid responses, therefore ensuring that the ad placements are done in a near real-time fashion. Frequent auctions ensure that slots are sold to the most engaged audience in the most optimal sales circumstances.
Content Delivery Network (CDN) Caching
A vast amount of AdTech solutions has a Content Delivery Network (CDN) usage for an ad assets deployment on a worldwide basis. CDNs have fast-caching abilities and thus can easily deliver ad content to users. Content Delivery Networks (CDNs) are responsible for serving a- les, such as images, videos, and other multimedia formats to users from di erent locations around the globe.
CDNs cache ad content on edge servers which are as close as possible to the users, and guarantee successful delivery of low-latency content alone. This technique prevents disruptions in the ad viewer experience so that a user is not troubled by crashing ads, irrespective of his geographical location.
102
5.2.2 Cache Invalidation and Refresh Strategies
E ective caching requires thoughtful cache invalidation and refresh strategies to maintain data accuracy and consistency:
Time-Based Expiry: One common approach to cache management is setting the expiration times for stored items and the second one removes invalid data. Data explicitly with intrinsic instability, for example, update in real time, might have cache lifetime which is shorter than the more static data, which can have longer lifetimes. Caching mechanism time-out guarantees that loaded data remains current.
Event-Driven Invalidation: Event-driven cache invalidation mechanism is one of the incisive mechanisms to provide cache consistency. By way of example, when images (ads) are modi ed or user pro les evolve, AdTech hardware can lead to structure invalidation events. Such activities are the precursor to the removal of the outdated data from the cache, e ectively delivering the most current information to the end users.
5.2.3 Lazy Loading
In situations where the refresh overhead to cache should be reduced, lazy loading is used as technique. Cached items wil not be refreshed unless they are being requested due to the lazy loading. Meaning less resources are wasted which is stil relevant when updates are necessary.
The adoption of such caching strategies and cache management techniques that meet the demands of AdTech applications by the Java developers thus provides a tremendous boost to application performance. E cient caching not only al eviates the server traction but also minimizes ad replaying time and improves ad presentation.
5.2.4 Challenges in Caching
Though caching provides tremendous advantages for performance increases, the caching of ads within AdTech needs careful consideration to address unique chal enges. Developers must be aware of these chal enges and adopt strategies to address them e ectively: Cache Consistency: Implementing a process that discriminate transactions that have out of date cache data is a daunting task in AdTech because many updates and dynamic content occurs in Real-Time. Developer have to insert caching invalidation strategies, that respond very quickly to the receiving of an updated records, to keep consistency.
Cache Size Management: The amount of cache can turn up to become essential in AdTech surveys, where many tasks are involved and datasets or throughput is relatively big. Developers should come up with algorithms to manage their cache size, for example, through eviction policies so that they do not use exorbitant amounts of memory.
103
Cache Eviction Policies: What items are deleted and retained from the cache become the source of debate under various eviction policies. Picking up an eviction policy that would have crucial data in it along with less important data is therefore necessary because it wil ensure data remains in the cache.
Distributed Caching: Caching plays an important role even in di erent environments, in particular, with AdTech, where data is scattered. Juggling cache consistency and synchronization across numerous nodes makes the management even more complicating; development team is required to deal with this issue additional y.
Cold Start: Caching is a tool that has helped to improve the overal performance of websites.
When information is purged or expires, a "hot start" situation comes about and data retrieved normal y from a stored cache must be loaded from the source afresh. Di erent patterns of cold start may impose a temporary loss of performance levels. Hence, the developers should work on relieving their e ect by implementing caching approaches.
104
5.2.5 Best Practices for E ective Caching
To navigate the chal enges of caching in AdTech successful y, Java developers should adhere to best practices:
Monitor Cache Performance: Let’s add monitoring and a log to the cache to grab cache performance details. Trough tracking can help developers to detect cache related problems, and also to redesign cahceg con gurations.
Use a Dedicated Caching Solution: Try the use of speci c caching solutions and libraries which include Redis, Memcached and Ehcache that cater to speedy programming. These solutions support functions such as distributed caching, replication, eviction policies and other distributed external storage arrays among others.
Test Cache Behavior: Testing extensively the cache behavior under di erent situations, namely huge tra c, updates of objects and cache expiration. The test wil verify that the cache work correctly as its intended purpose and keep the data consistent between the cache and the main memory.
Employ a Cache-Aside Pattern: The cache-aside pattern combines the assumption that applications are left with the job of loading and updating the data stored in caches. This regularity gives granular options to regulate caching styles and is useful for the AdTech scenarios that require a high level of data consistency.
Implement Distributed Caching Patterns: In the storing at a distributed system, try the distributed caching patterns, for example, the cache replication and cache partitioning to ensure consistent and scale cache.
Evolve Caching Strategies: It is important to acknowledge that the caching strategy may require updates over time when your application changes its objectives. Despite the developments in technology, be nimble and constantly upgrade your caching strategies in accordance with changing demands of the AdTech platform.
105
The whole success of Adtech platform performance is dependent on caching strategies. For developers, the main goal of e ective caching is to strategical y cache advertising content, user data, session information, and anything else that might be important. This can signi cantly reduce latency and improve the overal user experience. E cient cache management gives aftercare with calculated implications for cache invalidation and booking is achieved to preserve accuracy and currentness of cached data.
Nevertheless, adtech caching can be very powerful, but it does have associated chal enges. Hence, developers have to deal with the problems of cache consistency, cache size management, distribution and eviction policies. By fol owing best practices and be watchful at the pace of caching system, it is possible to resolve the problems and get the advantages of the caching mechanism.
In the next chapter, we explore the critical aspects of load balancing and scaling AdTech systems. As AdTech platforms grow and handle increasing tra c loads, e ective load balancing and scalability become paramount for maintaining optimal performance and reliability.
106
5.3 Load Balancing and Scaling AdTech Systems
For AdTech speci cal y, where users consume ads at lightning speed and the ad delivery has to be seamless, load distribution and scaling are very critical and essential practices. This part is focused on the essentials of tra c management, which is the process of balancing the resources while maintaining the reliability and performance of AdTech systems.
5.3.1 The Importance of Load Balancing and Scaling
The AdTech applications due to their activities within the constantly evolving environments have to manage tra c load ups and oods of ads requests. The users of AdTech demand that ad servings be fast and the service to be uninterrupted. In order for AdTech systems to accomplish the requirement, deployment of load balancing and scaling is vital. These practices o er several crucial bene ts:
Improved Performance: Load balancing makes sure that al the incoming ad requests are distributed in a balanced way which is more spread across numerous servers or instances. To this end, this distribution of is one that would address this balance by which lowering the latency and increase the quality of experience is the main goal. Viewers see ads upon a short time what causes greater clicks.
High Availability: Load balancers are developed to discover failed servers and path the tra c away from outages of their service instances. Providing such cover guarantees that AdTech systems to keep on their work with no chances tool server breakdowns, however, this secures to minimize downtime and provides high service reliability.
Scalability: The demand for AdTech services rises during certain periods or events driven by higher web tra c. Scaling creates the condition where more and more resources are supplied automatical y, based on the current systems limits. Scalable systems can satisfy tra c surges, that doing do not have place ads not be served properly.
Cost Optimization: Load balance and scaling strategies are utilitarian in terms of favorable resource distribution. As a decentralized platform, AdTech is capable of balancing tra c and adjusting the size provisions to t to the scale of demands, thus leading the overal costs as the operational costs are reduced.
107
5.3.2 Load Balancing Strategies
E ective load balancing represents the core competency of AdTech systems for providing best-in-class performance and availability. A friendly technique to distribute requests is a load balancer that intel igently al ocates incoming ad requests to take the best advantage of available resources. Here are some load balancing techniques tailored to AdTech: Round Robin Load Balancing: By this means, al accessible server populations would be serviced without bias via the circular distribution of ad requests. Round robin load balancing is extremely convenient and e cient in instances where servers are composed of similarly capable ones and have similar response times.
Weighted Round Robin Load Balancing: Scenarios in which servers' inbuilt capacities di er or have varying response rates are taken care of by assigning a weight to each server in weighted round robin load balancing. The servers with higher weights amongl them wil get more ads requests and as a result, they assure the resources are al ocated per their capabilities.
Least Connections Load Balancing: By selecting only the server with fewest active ad requests, this approach aims to resolve overloading issues. It is super helpful in servers of variable processing capacities and when the requests varied in complexity. Least connections load balancing with its direct goal to successful y distribute workload of al servers involved.
IP Hash Load Balancing: IP hash load balancing, respectively, distributes the request to the server depending upon the client's IP address. It determines such situations when the same client is redirected to the particular server. It speeds up stateful aesthetics applications and helps in preserving the session continuity in the AdTech area.
Content-Based Load Balancing: In AdTech scenarios, it may be some ad requests would be more resource-intensive, speci cal y due to the complexity of the ad creatives or the case of real-time bidding (RTB) Content-based load balancing looks into the content of ads requests and routes them to the servers which are (already) enabled to handle (already) the given requirements. This way, resources are harvested by ad requests of many kinds.
108
The capacity to scale up in regard to the upsurge in the Adtech tra c is as vital as can be. Tra c can spike beyond measure during an event, going live with a new product or running the speci c campaign. Developers have to do the scaling with AdTech systems that could operate either on the contracting or growth process depending on the amount of responding demand. Here are some scalable approaches:Here are some scalable approaches:
Vertical Scaling: Also named as "scaling up" horizontal scaling include the expansion of the capacity of servers. The number of core CPU units, memory, and other resources wil be added to the server to make this possible. Also, vertical scaling is able to extend the current provision over the near term however, stacking pods on one host increases costs.
Horizontal Scaling: Horizontal scaling, or stated scaling, is a process where more servers or instances are added to facilitate quicker and smoother experience in AdTech system. Distributing jobs among di erent servers in this way is a load balancing mechanism, which puts them in a position to tackle undue tra c ow. Horizontal scaling is one of the main methods to get elasticity and tra c peaks' load.
Auto-Scaling: Auto-scaling is a dynamic facility which automatic scaling the number of server instances based upon prede ned intrinsic elements, such as CPU utilization, memory usages, or the incoming ad request number. Most of the deployment is cloud-based, and auto-scaling options al ow AdTech applications to self-adjust in order to meet workload uctuations.
Microservices Architecture: Microservices Architecture is the new way of designing applications by where rather than the whole application, which could be served through a monolithic design, the latter is broken down into smal er, independently deployable services. Each of the services can be scaled out to provide tailor made ways for tackling speci c issues with the example of ad rendering, user data processing and real-time bids. Microservices provide exibility and scalability making AdTech a good t among other things.
Serverless Computing: One of the most signi cant changes introduced by the cloud providers is the serverless computing that lets developers focus on writing code and application logic without requiring them to be in charge of the server infrastructure. Therefore, such technologies as AdTech can stand to nd their application in the serverless functions that are able to automatical y scale depending upon the number of inbound ad cal s, consequently diminishing the operational niche.
109
5.3.4 Challenges in Load Balancing and Scaling
While load balancing and scaling o er signi cant advantages, they also come with chal enges that AdTech developers must address:
State Management: AdTech applications often require stateful interactions, such as maintaining user sessions or tracking ad impressions. Load balancing strategies must consider how to manage and synchronize session data across servers to ensure a seamless user experience.
Data Consistency: AdTech systems rely on consistent data, including user pro les and ad inventory. Ensuring data consistency across distributed servers can be chal enging and requires robust synchronization mechanisms.
Monitoring and Alerts: E ective load balancing and scaling strategies depend on real-time monitoring of server health, resource utilization, and tra c patterns. Implementing monitoring solutions and alerting mechanisms is crucial to identifying and addressing issues promptly.
Cost Management: Scaling can incur additional costs, especial y when utilizing cloud-based resources. AdTech platforms must strike a balance between ensuring scalability and managing operational expenses e ectively.
Security Considerations: Load balancers and scaled instances must be secured to protect AdTech applications from security threats, including distributed denial-of-service (DDoS) attacks and unauthorized access.
110
5.3.5 Best Practices for Load Balancing and Scaling To overcome the chal enges and harness the bene ts of load balancing and scaling in AdTech, developers should adhere to best practices:
Conduct Load Testing: Before deployment, do load testing to check for the system's response by simulating tra c higher than normal. The tra c test wil check the system's responsiveness when it is receiving a higher-than-usual tra c load. Determine the points where the system slows down and reprogram load balancing and scaling con gurations to avoid any interferences with performance thereby eliminating them.
Implement Redundancy: Implement least-tra c load-balancers and backup server in order to maximize uptime. Redundancy makes abandoning at single point failure and strengthens overal system reliability.
Use Load Balancer Health Checks: Set up load balancers to verify the health of the backend servers perform health checks. Hence, tra c is redirected from destinations that are not working or slow in responding.
Leverage Cloud Services: The cloud provider provides the managed solutions, which include load balancing and auto-scaling that simplify their implementation. Using cloud service on-demand scale al ows for a cost-e ective scalability.
Employ Containerization: Docker and Kubernetes are main players in container technology space which makes it easier and scalable to deploy microservices. Container orchestration platforms are the means to have the services as part of their AdTech infrastructure better managed if they use streaming containers.
Prioritize Security: Implement security measures, such as rewal rules and access controls, to protect load balancers and scaled instances from security threats. Regularly update and patch system components to address vulnerabilities.
5.3.6 Conclusion
The dynamic features such as loading balancing and scaling are two of the crucial parts of the infrastructure of resilient and high-performance AdTech projects. These techniques, therefore, help AdTech software solutions to withstand di erent stress loads, send ads on time, and stay online. By integrating perfect load balancing and scalable structures, java developers enhance AdTech platforms to perform wel given the dynamic and competitive nature of the marketing domain.
AdTech wil continue to grow and advanced progress and load balancing and scaling wil remain as major chal enges. With the help of exible algorithms and software capabilities, AdTech platforms can easily ful l the requirements of both the advertisers and publishers, regardless of the nature of changes in tra c patterns and user preferences. Through utilization of load balancing and scaling practices, we strive to keep up the website performance at its peak level.
111
By the end of the paper, we have come to the conclusion that AdTech is the amalgamation of technology, strategy, and market dynamics which are at the helm of the digital space. Our discourse started with an in-depth study of the AdTech ecosystem covering its components as wel as the crucial role of Java in it. This chapter wil draw the main conclusions of our orientation, contemplate the future route of AdTech, as wel as consider Java's further prospect in this developing area.
Recapitulation of Key Concepts
We began with an investigating in the AdTech ecosystem in which we looked at deeply. We studied many aspects of the eco-system, including DSP which makes it economical for advertisers to buy ad space, and SSP, which provides publishers the ability to sel their spaces to the highest bidder. The point of emphasis was on the manifestation of data management platforms (DMPs) that act as basic tools in ordering and reactivating data for e cient targeted advertising. Another broad area of coverage was ad exchanges, header bidding, and how important Creative Management Platforms (CMPs) and Dynamic Creative Optimization (DCO) become for future use.
The abilities of Java were listed that pertain to the reproduction of di erent AdTech solutions, the serving of mass input ad operations, and the ability in delivering dependable ad-serving systems. In addition, the role of Java in real-time bidding, data processing and analytics, which are known for being the heart and soul of technology behind AdTech, was stressed, emphasising its absolute necessity.
Evolution and Future Trajectory of AdTech
The technological changes that occur as wel as changing consumer behaviors across the market plus regulatory landscapes are continual y developing factors that in uence the AdTech industry.
Introducing AI and machine learning technologies leads to a new epoch of smart targeting through the development of models that deliver the ads so precisely. Yet, the growth in itself implies some issues, encompassing the issue on how to participate in the technology evolution without con icting on the privacy of the users.
The new trend that appears in the TV programmatic advertising and the growth of the coordinated campaigns indicate that advertising shifts to the integrated approach. These trends speak to the future where the advertisement is more no-impression, intuitive, and and natural y syncing between consumer online habits and tastes.
112
Java’s Continued Relevance in AdTech
Despite its current reign of dominance in AdTech, Java's day of reckoning looms.
Java has returned to the roots of Adtech since of the day, and that this relation is projected to go stronger in the foreseeable future. Java's outstanding performance has been further improved ensuring it always remains ready for current AdTech market that requires a massive ow of data to be processed at the maximum possible speed.
Future Java versions (version updates) are probably going to have an emphasis on improving the program run speeds and work e ectiveness with the new tools that have been brought up – cloud computing and microservices which have been getting popular in the Adtech industry. Despite we are witnessing a constant stream of new technologies, the community, libraries, and platform of Java wil always be an essential foundation for building smart applications.
Thank you for joining on this educational journey, and I wish you success in your endeavors in the ever-evolving eld of AdTech and Java development!
113
S28
S28
Java in Ad Tech / Semeniuk V. – Odesa : Oldi+, 2024. – 114 p.
ISBN 978-966-289-848-4
UDK 004.056.55
_______________________________________________________________
Навчальне видання
Семенюк Вадим
Java в рекламних технологіях
Англ. мовою
Підписано до друку 28.03.2024 р.
Формат 60х84/8. Папір офсетний.
Цифровий друк. Ум. друк. арк. 13,25. Наклад 10.
Замовлення № 0324-033.
Видавництво та друк: Олді+
65101, м. Одеса, вул. Інглезі, 6/1,
тел.: +38 (095) 559-45-45, e-mail: office@oldiplus.ua
Свідоцтво ДК № 7642 від 29.07.2022 р.
Замовлення книг:
тел.: +38 (050) 915-34-54, +38 (068) 517-50-33
e-mail: book@oldiplus.ua