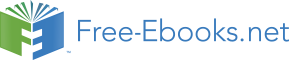

3
Enter a number
-2
Enter a number
0
sum=1
In this C program, user is asked a number and it is added with sum. Then, only the test condition in the do...while loop is checked. If the test condition is true,i.e, num is not equal to 0, the body of do...while loop is again executed until num equals to zero.
C Programming break and continue Statement
There are two statement built in C, break; and continue; to interrupt the normal flow of control of a program. Loops performs a set of operation repeately until certain condition becomes false but, it is sometimes desirable to skip some statements inside loop and terminate the loop immediately without checking the test expression. In such cases, break and continue statements are used.
break Statement
In C programming, break is used in terminating the loop immediately after it is encountered. The break statement is used with conditional if statement.
Syntax of break statement
break;
The break statement can be used in terminating all three loops for, while and do...while loops.
The figure below explains the working of break statement in all three type of loops.
Example of break statement
Write a C program to find average of maximum of n positive numbers entered by user. But, if the input is negative,
display the average(excluding the average of negative input) and end the program.
/* C program to demonstrate the working of break statement by terminating a loop, if user
inputs negative number*/
# include <stdio.h>
int main(){
float num,average,sum;
int i,n;
printf("Maximum no. of inputs\n");
scanf("%d",&n);
for(i=1;i<=n;++i){
printf("Enter n%d: ",i);
scanf("%f",&num);
if(num<0.0)
break; //for loop breaks if num<0.0
sum=sum+num;
}
average=sum/(i-1);
printf("Average=%.2f",average);
return 0;
}