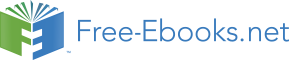

C Programming Examples And Source Code
C Program to Check Whether a Number is Even or Odd
C Programming Examples And Source Code
C Program to Check Whether a Character is Vowel or consonant
C Program to Find the Largest Number Among Three Numbers Entered by User
C program to Find all Roots of a Quadratic equation
C Program to Check Whether the Entered Year is Leap Year or not
C Program to Check Whether a Number is Positive or Negative or Zero.
C Program to Checker Whether a Character is an Alphabet or not
C Program to Find Sum of Natural Numbers
C Program to Find Factorial of a Number
C program to Generate Multiplication Table
C Program to Display Fibonacci Series
C Program to Find HCF of two Numbers
C Program to Find LCM of two numbers entered by user
C Program to Count Number of Digits of an Integer
C Program to Reverse a Number
C program to Calculate the Power of a Number
C Program to Check Whether a Number is Palindrome or Not
C Program to Check Whether a Number is Prime or Not
C Program to Display Prime Numbers Between Two Intervals
C program to Check Armstrong Number
C Programming Examples And Source Code
C Program to Display Armstrong Number Between Two Intervals
C program to Display Factors of a Number
C program to Print Pyramids and Triangles in C programming using Loops
C program to Make a Simple Calculator to Add, Subtract, Multiply or Divide Using
switch...case
C Programming Functions
Function in programming is a segment that groups a number of program statements to perform specific task.
A C program has at least one function main( ). Without main() function, there is technically no C program.
Types of C functions
Basically, there are two types of functions in C on basis of whether it is defined by user or not.
Library function
User defined function
Library function
Library functions are the in-built function in C programming system. For example:
main()
- The execution of every C program starts from this main() function.
printf()
- prinf() is used for displaying output in C.
scanf()
- scanf() is used for taking input in C.
Visit this page to learn more about library functions in C programming language.
User defined function
C provides programmer to define their own function according to their requirement known as user defined functions. Suppose, a programmer wants to find factorial of a number and check whether it is prime or not in same program. Then, he/she can create two separate user-defined functions in that program: one for finding factorial and other for checking whether it is prime or not.
How user-defined function works in C Programming?
#include <stdio.h>
void function_name(){
................
................
}
int main(){
...........
...........
function_name();
...........
...........
}
As mentioned earlier, every C program begins from main() and program starts executing the codes inside main() function. When the control of program reaches to function_name() inside main() function. The control of program jumps to void function_name() and executes the codes inside it. When, all the codes inside that user-defined function are executed, control of the program jumps to the statement just after function_name() from where it is called. Analyze the figure below for understanding the concept of function in C
programming. Visit this page to learn in detail about user-defined functions.
Remember, the function name is an identifier and should be unique.
Advantages of user defined functions
1. User defined functions helps to decompose the large program into small segments which makes programmar easy to
understand, maintain and debug.
2. If repeated code occurs in a program. Function can be used to include those codes and execute when needed by calling that function.
3. Programmar working on large project can divide the workload by making different functions.
C Programming User-defined functions
This chapter is the continuation to the function Introduction chapter.
Example of user-defined function
Write a C program to add two integers. Make a function add to add integers and display sum in main() function.
/*Program to demonstrate the working of user defined function*/
#include <stdio.h>
int add(int a, int b); //function prototype(declaration)
int main(){
int num1,num2,sum;
printf("Enters two number to add\n");
scanf("%d %d",&num1,&num2);
sum=add(num1,num2); //function call
printf("sum=%d",sum);
return 0;
}
int add(int a,int b) //function declarator
{
/* Start of function definition. */
int add;
add=a+b;
return add; //return statement of function
/* End of function definition. */
}
Function prototype(declaration):
Every function in C programming should be declared before they are used. These type of declaration are also called function prototype.
Function prototype gives compiler information about function name, type of arguments to be passed and return type.
Syntax of function prototype
return_type function_name(type(1) argument(1),....,type(n) argument(n));
In the above example,int add(int a, int b); is a function prototype which provides following information to the compiler:
1. name of the function is add()
2. return type of the function is int.
3. two arguments of type int are passed to function.
Function prototype are not needed if user-definition function is written before main() function.
Function call
Control of the program cannot be transferred to user-defined function unless it is called invoked).
Syntax of function call
function_name(argument(1),....argument(n));
In the above example, function call is made using statement add(num1,num2); from main(). This make the control of program jump from that statement to function definition and executes the codes inside that function.
Function definition
Function definition contains programming codes to perform specific task.