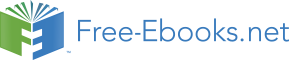

2
3
4
5
Displaying:
2
3
4
5
This page contains examples and source code on arrays and pointers. To understand all program on this page, you should have
knowledge of following array and pointer topics:
1. Arrays
2. Multi-dimensional Arrays
3. Pointers
4. Array and Pointer Relation
5. Call by Reference
6. Dynamic Memory Allocation
Array and Pointer Examples
C Programming Examples And Source Code
C Programming Examples And Source Code
C Program to Calculate Average Using Arrays
C Program to Find Largest Element of an Array
C Program to Calculate Standard Deviation
C Program to Add Two Matrix Using Multi-dimensional Arryas
C Program to Multiply to Matrix Using Multi-dimensional Arrays
C Program to Find Transpose of a Matrix
C Program to Multiply two Matrices by Passing Matrix to Function
C Program to Sort Elements of an Array
C Program to Access Elements of an Array Using Pointer
C Program Swap Numbers in Cyclic Order Using Call by Reference
C Program to Find Largest Number Using Dynamic Memory Allocation
C Programming Pointers
Pointers are the powerful feature of C and (C++) programming, which differs it from other popular programming languages like: java and Visual Basic.
Pointers are used in C program to access the memory and manipulate the address.
Reference operator(&)
If var is a variable then, &var is the address in memory.
/* Example to demonstrate use of reference operator in C programming. */
#include <stdio.h>
int main(){
int var=5;
printf("Value: %d\n",var);
printf("Address: %d",&var); //Notice, the ampersand(&) before var.
return 0;
}