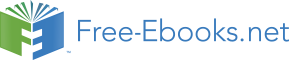

Suppose, the programmer want pointer pc to point to the address of c. Then,
int c, *pc;
pc=c; /* pc is address whereas, c is not an address. */
*pc=&c; /* &c is address whereas, *pc is not an address. */
In both cases, pointer pc is not pointing to the address of c.
Pointers and Arrays
Arrays are closely related to pointers in C programming. Arrays and pointers are synonymous in terms of how they use to access memory. But, the important difference between them is that, a pointer variable can take different addresses as value whereas, in case of array it is fixed. This can be demonstrated by an example:
#include <stdio.h>
int main(){
char c[4];
int i;
for(i=0;i<4;++i){
printf("Address of c[%d]=%x\n",i,&c[i]);
}
return 0;
}
Address of c[0]=28ff44
Address of c[1]=28ff45
Address of c[2]=28ff46
Address of c[3]=28ff47
Notice, that there is equal difference (difference of 1 byte) between any two consecutive elements of array.
Note: You may get different address of an array.
Relation between Arrays and Pointers
Consider and array:
int arr[4];
In arrays of C programming, name of the array always points to the first element of an array. Here, address of first element of an array is &arr[0]. Also, arr represents the address of the pointer where it is pointing. Hence, &arr[0] is equivalent to arr.
Also, value inside the address &arr[0] and address arr are equal. Value in address &arr[0] is arr[0] and value in addressarr is *arr.
Hence, arr[0] is equivalent to *arr.
Similarly,
&a[1] is equivalent to (a+1) AND, a[1] is equivalent to *(a+1).
&a[2] is equivalent to (a+2) AND, a[2] is equivalent to *(a+2).
&a[3] is equivalent to (a+1) AND, a[3] is equivalent to *(a+3).
.
.
&a[i] is equivalent to (a+i) AND, a[i] is equivalent to *(a+i).
In C, you can declare an array and can use pointer to alter the data of an array.
//Program to find the sum of six numbers with arrays and pointers.
#include <stdio.h>
int main(){
int i,class[6],sum=0;
printf("Enter 6 numbers:\n");
for(i=0;i<6;++i){
scanf("%d",(class+i)); // (class+i) is equivalent to &class[i]
sum += *(class+i); // *(class+i) is equivalent to class[i]
}
printf("Sum=%d",sum);
return 0;
}