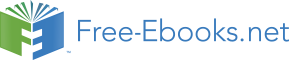

Allocates requested size of bytes and returns a pointer first byte of allocated
malloc()
space
Allocates space for an array elements, initializes to zero and then returns a
calloc()
pointer to memory
free()
dellocate the previously allocated space
realloc()
Change the size of previously allocated space
malloc()
The name malloc stands for "memory allocation". The function mal oc() reserves a block of memory of specified size and return a pointer of type void which can be casted into pointer of any form.
Syntax of malloc()
ptr=(cast-type*)malloc(byte-size)
Here, ptr is pointer of cast-type. The mal oc() function returns a pointer to an area of memory with size of byte size. If the space is insufficient, allocation fails and returns NULL pointer.
ptr=(int*)malloc(100*sizeof(int));
This statement will allocate either 200 or 400 according to size of int 2 or 4 bytes respectively and the pointer points to the address of first byte of memory.
calloc()
The name calloc stands for "contiguous allocation". The only difference between malloc() and calloc() is that, malloc() allocates single block of memory whereas calloc() allocates multiple blocks of memory each of same size and sets all bytes to zero.
Syntax of calloc()
ptr=(cast-type*)calloc(n,element-size);
This statement will allocate contiguous space in memory for an array of n elements. For example:
ptr=(float*)calloc(25,sizeof(float));
This statement allocates contiguous space in memory for an array of 25 elements each of size of float, i.e, 4 bytes.
free()
Dynamically allocated memory with either calloc() or malloc() does not get return on its own. The programmer must use free() explicitly to release space.
syntax of free()
free(ptr);
This statement cause the space in memory pointer by ptr to be deallocated.
Examples of calloc() and malloc()
Write a C program to find sum of n elements entered by user. To perform this program, allocate memory dynamically
using malloc() function.
#include <stdio.h>
#include <stdlib.h>
int main(){
int n,i,*ptr,sum=0;
printf("Enter number of elements: ");
scanf("%d",&n);
ptr=(int*)malloc(n*sizeof(int)); //memory allocated using malloc
if(ptr==NULL)
{
printf("Error! memory not allocated.");
exit(0);
}
printf("Enter elements of array: ");
for(i=0;i<n;++i)
{
scanf("%d",ptr+i);
sum+=*(ptr+i);
}
printf("Sum=%d",sum);
free(ptr);
return 0;
}
Write a C program to find sum of n elements entered by user. To perform this program, allocate memory dynamically
using calloc() function.
#include <stdio.h>
#include <stdlib.h>
int main(){
int n,i,*ptr,sum=0;
printf("Enter number of elements: ");
scanf("%d",&n);
ptr=(int*)calloc(n,sizeof(int));
if(ptr==NULL)
{
printf("Error! memory not allocated.");
exit(0);
}
printf("Enter elements of array: ");
for(i=0;i<n;++i)
{
scanf("%d",ptr+i);
sum+=*(ptr+i);
}
printf("Sum=%d",sum);
free(ptr);
return 0;
}
realloc()
If the previously allocated memory is insufficient or more than sufficient. Then, you can change memory size previously allocated using realloc().
Syntax of realloc()
ptr=realloc(ptr,newsize);
Here, ptr is reallocated with size of newsize.
#include <stdio.h>
#include <stdlib.h>
int main(){
int *ptr,i,n1,n2;
printf("Enter size of array: ");
scanf("%d",&n1);
ptr=(int*)malloc(n1*sizeof(int));
printf("Address of previously allocated memory: ");
for(i=0;i<n1;++i)
printf("%u\t",ptr+i);
printf("\nEnter new size of array: ");
scanf("%d",&n2);
ptr=realloc(ptr,n2);
for(i=0;i<n2;++i)
printf("%u\t",ptr+i);
return 0;
}
C Programming Array and Pointer Examples
This page contains examples and source code on arrays and pointers. To understand all program on this page, you should have
knowledge of following array and pointer topics:
1. Arrays
2. Multi-dimensional Arrays
3. Pointers
4. Array and Pointer Relation
5. Call by Reference
6. Dynamic Memory Allocation
Array and Pointer Examples
C Programming Examples And Source Code
C Programming Examples And Source Code
C Program to Calculate Average Using Arrays
C Program to Find Largest Element of an Array
C Program to Calculate Standard Deviation
C Program to Add Two Matrix Using Multi-dimensional Arryas
C Program to Multiply to Matrix Using Multi-dimensional Arrays
C Program to Find Transpose of a Matrix
C Program to Multiply two Matrices by Passing Matrix to Function
C Program to Sort Elements of an Array
C Program to Access Elements of an Array Using Pointer
C Program Swap Numbers in Cyclic Order Using Call by Reference
C Program to Find Largest Number Using Dynamic Memory Allocation
C Programming String
In C programming, array of character are called strings. A string is terminated by null character /0. For example:
"c string tutorial"
Here, "c string tutorial" is a string. When, compiler encounters strings, it appends null character at the end of string.
Declaration of strings
Strings are declared in C in similar manner as arrays. Only difference is that, strings are of char type.
char s[5];
Strings can also be declared using pointer.
char *p
Initialization of strings
In C, string can be initialized in different number of ways.
char c[]="abcd";
OR,
char c[5]="abcd";
OR,
char c[]={'a','b','c','d','\0'};
OR;
char c[5]={'a','b','c','d','\0'};
String can also be initialized using pointers
char *c="abcd";
Reading Strings from user.
Reading words from user.
char c[20];
scanf("%s",c);
String variable c can only take a word. It is beacause when white space is encountered, the scanf() function terminates.
Write a C program to illustrate how to read string from terminal.
#include <stdio.h>
int main(){
char name[20];
printf("Enter name: ");
scanf("%s",name);
printf("Your name is %s.",name);
return 0;
}