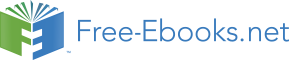

React Components: Class vs. Function
Conditional Rendering in React
React Class Component Lifecycle Methods
Understanding Higher-order Components in React
Controlled Components in React
Uncontrolled Components in React
Using the Children Prop in React
Understanding Error Boundaries in React
Server-Side Rendering with React
Static Site Generation in Next.js
Understanding useReducer in React
Component Communication in React
Optimizing Performance with useCallback
Integrating Static Typing in React
Managing Global State with Redux
Managing Side Effects in Redux
Enhancing Accessibility in React
Optimizing Bundle Sizes in React
State Management in Large Applications
Shallow vs. Deep Copy in JavaScript
Using React Native for Mobile Development
Function Components with Hooks
Enhancing Accessibility in Interactive UIs
Optimizing Render Times in React
Preventing Memory Leaks in React
Understanding useContext in React
Using useLayoutEffect in React
Modular Architecture in React Projects
Continuous Integration and Deployment in React
Managing Environments in React
Using Component Libraries in React
Clear Project Structure in React
Using Service Workers in React
Optimizing Image Handling in React
Adopting Mobile-First Design in React
Debouncing and Throttling in React
Using useEffect Dependency Arrays
Compatibility with External Libraries
Understanding React's Concurrent Mode
Implementing Code Splitting in React
Graceful Data Handling in React
Handling Asynchronous Operations in React
Functional Programming in React
◆
Welcome to a focused journey through the essentials of React, tailored specifically for those new to this powerful JavaScript library. Whether you're a seasoned programmer new to React or a veteran looking to refresh your knowledge on the latest features, this guide is crafted to streamline your learning process.
React is not just a tool, but a modern approach to building user interfaces with dynamic and interactive elements. This ebook distills the fundamental concepts you need to start building effective React applications. It avoids unnecessary complexities, focusing solely on the must-know principles and techniques.
As you progress through the pages, you will find the content rich in practical tips and core co ncepts, designed to be immediately applicable to your projects. We hope this guide proves i nvaluable in your development journey and assists you in quickly becoming proficient with R
eact.
Should this resource prove helpful, we kindly ask you to leave a review or comment. Your fee dback not only helps us improve, but also aids fellow engineers in similar situations discover this ebook. Let's grow and learn together in the ever-evolving world of software developmen t.
React Components
Components are the core building blocks of React applications, allowing you to divide the us er interface (UI) into manageable, reusable pieces.
Here is an example of a simple React component that displays a greeting message:
[Code]
import React from 'react';
function Greeting() {
return <h1>Hello, world!</h1>;
}
export default Greeting;
[Result]
The component would render "Hello, world!" inside an <h1> tag on the webpage.
In React, components are defined using JavaScript functions or classes. They return React ele ments describing what should appear on the screen. The Greeting function component abov e is simple: it returns a single <h1> element with the text "Hello, world!". This is an example of a functional component, which is favored for their simplicity and ease of use. Functional c
omponents can utilize hooks for managing state and other React features, which were introd uced in React 16.8.
[Trivia]
Understanding component composition is key in React. Larger applications are built by comp osing many small components together, similar to building with LEGO bricks. This modularity not only makes development easier but also enhances code reusability and testing.
React Props
Props are the mechanism by which components receive data from their parent, serving as rea d-only inputs that help configure their behavior or display.
Here's a simple example of a React component receiving props and using them to display da ta:
[Code]
import React from 'react';
function Welcome(props) {
return <h1>Hello, {props.name}!</h1>;
}
export default Welcome;
[Result]
If passed a prop name with the value "Alice", the component would render "Hello, Alice!" insi de an <h1> tag.
Props (short for "properties") are how data gets passed around in a React application. Each c omponent receives its own props object as a parameter, which can be used to read the prop
erties attached to it. Props are immutable within the component—meaning they cannot be c hanged by the component itself but can be replaced by new data from the parent componen t when it re-renders. This immutability helps prevent bugs and maintains data flow clarity acr oss the application.
[Trivia]
A common React pattern is "lifting state up;" that is, sharing state data across multiple comp onents by moving it up to their closest common ancestor. This technique allows components to remain pure (i.e., deterministic output based on props and state), which simplifies debuggi ng and testing.
Understanding State in React
In React, state refers to a component's local data storage that can be changed over time. Eac h component can have its own state.
Below is an example of a React class component using state. The component includes a butt on that increments a count stored in the state.
[Code]
import React, { Component } from 'react';
class Counter extends Component {
constructor(props) {
super(props);
// State initialization in the constructor
this.state = { count: 0 };
}
incrementCount = () => {
this.setState({ count: this.state.count + 1 });
};
render() {
return (
<div>
<h1>Count: {this.state.count}</h1>
<button onClick={this.incrementCount}>Increment</button>
);
}
}
export default Counter;
[Result]
Initially, the screen displays "Count: 0". Each click on the "Increment" button increases the co unt by 1.
State in React components is crucial for managing data that affects the render output. When setState() is called, React schedules an update to the component's state object and subseque ntly re-renders the component to reflect the new state. This method merges the object you p rovide into the current state, ensuring that only the components that rely on that state data r erender, which optimizes performance.The use of state enables React components to be dyn amic and responsive to user interactions or other changes. This example demonstrates a sim ple use of state with a counter. It's important to initialize the state in the constructor of a clas s component, which sets up the initial state before any interaction occurs. This setup is vital f or components to have accessible and modifiable state properties during their lifecycle.
[Trivia]
React's setState() is asynchronous, which means it schedules changes to the component state and tells React to re-render the component and its children with updated state. This is a key concept in understanding how updates are managed in React applications.
Virtual DOM in React
React uses a virtual DOM to optimize rendering by minimizing the number of updates to the actual DOM, which improves performance.
Below is a conceptual example explaining how React updates the real DOM using the virtual DOM.
[Code]
// This is a conceptual example and not executable code function updateComponent(virtualDOM) {
const actualDOM = document.getElementById('app');
const newDOM = renderToDOM(virtualDOM);
if (newDOM !== actualDOM.innerHTML) {
actualDOM.innerHTML = newDOM;
}
}
function renderToDOM(virtualDOM) {
// Simulate rendering process
return `<h1>${virtualDOM.props.title}</h1>`;
}
// Example of virtual DOM object
const virtualDOM = {
type: 'h1',
title: 'Hello, React!'
}
};
// Example of how React might update the real DOM
updateComponent(virtualDOM);
[Result]
In a real React environment, the DOM would update to display "Hello, React!" if it's different from the current content.
React's virtual DOM is a lightweight copy of the actual DOM. It is used to test and see what c hanges need to be made in the real DOM. When changes occur in the component's state or props, React updates this virtual DOM first. Then, it compares the new virtual DOM with the previous snapshot of the virtual DOM. This process is called "diffing."Once React knows exact ly which virtual DOM objects have changed, it updates only those parts in the real DOM, not the entire DOM. This selective update process significantly reduces the burden on the actual DOM and improves the performance of the application. This mechanism is essential for high-performance applications that need to handle complex updates and frequent re-rendering.
[Trivia]
The virtual DOM not only improves performance but also adds a layer of abstraction that sim plifies developer experience. React abstracts away the direct manipulation of the DOM, allowi ng developers to work at a higher conceptual level.
Understanding JSX in React
JSX is a syntax extension for JavaScript that allows you to write HTML-like code inside JavaSc ript. It is often used with React to define the structure of user interfaces.
The following example demonstrates a simple React component using JSX.
[Code]
import React from 'react';
function App() {
return (
<div>
<h1>Hello, React!</h1>
<p>Welcome to JSX.</p>
</div>
);
}
export default App;
[Result]
The code would render a web page displaying the text "Hello, React!" in a header, followed b y "Welcome to JSX." in a paragraph.
JSX allows developers to write HTML structures in the same file as JavaScript code, which sim plifies the development process by avoiding the constant switching between HTML and Java Script files. When using JSX, you can insert JavaScript expressions inside curly braces {}, which is handy for dynamic content. JSX is transformed into JavaScript calls that create React eleme nts, which are then rendered to the DOM.To use JSX effectively:Always start component nam es with a capital letter.Return a single root element in the JSX expression.Use curly braces {} t o integrate JavaScript expressions into JSX.Apply HTML attributes using camelCase notation, such as onClick for handling clicks.
[Trivia]
JSX is not a requirement for using React, but it is highly recommended for its readability and ease of integration with the UI logic. Babel compiles JSX into React.createElement() calls behi nd the scenes, which can be seen if you compile JSX in a Babel transpiler.
React Components: Class vs. Function
In React, components are reusable UI elements, and they can be defined either using class sy ntax or function syntax, known as class components and function components, respectively.
Below is an example showing both a class component and a function component in React.
[Code]
import React, { Component } from 'react';
// Class component
class ClassComponent extends Component {
render() {
return <h2>Class Component Example</h2>;
}
}
// Function component
function FunctionComponent() {
return <h2>Function Component Example</h2>;
}
function App() {
return (
<div>
<ClassComponent />
<FunctionComponent />
);
}
export default App;
[Result]
This code would render a web page displaying "Class Component Example" followed by "Fun ction Component Example."
Class components provide more features than function components, such as local state man agement and lifecycle methods (e.g., componentDidMount). They are more suited for compl ex scenarios involving state or lifecycle hooks.Function components are simpler and mainly u sed for presenting static content or handling UI without internal state management. With the introduction of Hooks in React 16.8, function components can now use state and other React features without writing a class.Key distinctions include:Syntax and boilerplate: Class compon ents require more syntax and typically more code.Lifecycle methods: Available in class comp onents.Hooks: Used in function components for state and lifecycle features.Performance: Fun ction components generally have less overhead and can lead to better performance in many cases.
[Trivia]
As of recent React updates, function components with Hooks are becoming more popular th an class components due to their simplicity and reduced code complexity. React documentat ion now encourages the use of function components for new projects.
Understanding React Hooks
Hooks are special functions in React that allow you to use state and other features without w riting a class.
Here’s a simple example using the useState and useEffect hooks.
[Code]
import React, { useState, useEffect } from 'react';
function ExampleComponent() {
const [count, setCount] = useState(0);
useEffect(() => {
document.title = `You clicked ${count} times`;
}, [count]);
return (
<div>
<p>You clicked {count} times</p>
<button onClick={() => setCount(count + 1)}>
Click me
</button>
</div>
);
}
export default ExampleComponent;
[Result]
The displayed result will be a button that updates the count and the document title each tim e it is clicked.
In this example, useState is used to create count state variable. The setCount function is used to update this state. When the button is clicked, setCount increments the count by 1.The use Effect function runs after every render of the component but, due to the second argument [c ount], it only re-runs when count changes. This hook is used to update the document's title e very time the count state changes.Understanding these hooks is fundamental as they allow y ou to manage side-effects, state, and more in function components, promoting cleaner and more modular code structures.
[Trivia]
useState and useEffect are the most commonly used hooks, but there are others like useCont ext for accessing React context, useReducer for more complex state logic, and useMemo and useCallback for optimizing performance.
Conditional Rendering in React
React allows you to conditionally render components or elements using JavaScript expressio ns directly in JSX.
Below is an example showing how to use ternary operators and logical && for conditional re ndering.
[Code]
import React from 'react';
function Greeting({ isLoggedIn }) {
return (
<div>
{isLoggedIn ? (
<h1>Welcome back!</h1>
) : (
<h1>Please log in.</h1>
)}
{isLoggedIn && <button>Logout</button>}
</div>
);
}
export default Greeting;
[Result]
Depending on the isLoggedIn boolean, different elements are rendered.
In this example, the ternary expression {isLoggedIn ? <h1>Welcome back!</h1> : <h1>Pleas e log in.</h1>} is used to decide between rendering a welcome message or a login prompt based on whether the user is logged in.The expression {isLoggedIn && <button>Logout</b utton>} uses the logical && operator. This means the button will only render if isLoggedIn is true. If isLoggedIn is false, React will skip rendering the button, as the first part of the express ion evaluates to false.These techniques are very powerful for creating dynamic interfaces whe re the UI needs to adapt to different states or conditions.
[Trivia]
The ternary operator is useful for choosing between two components, while the logical && is more suited for conditionally including an element. Using these directly in JSX keeps the ren dering logic clean and readable, which is crucial for maintaining larger React applications.
Rendering Lists in React
In React, lists of items can be dynamically rendered using the JavaScript map() function comb ined with JSX.
Below is a simple example of rendering a list of names in a React component using map() an d JSX.
[Code]
function NameList() {
const names = ['Alice', 'Bob', 'Charlie'];
return (
<ul>
{names.map(name => <li key={name}>{name}</li>)}
</ul>
);
}
[Result]
An unordered list (ul) with three list items (li) displaying the names: Alice, Bob, and Charlie.
In the provided code example:We define a functional component called NameList.Inside this component, an array of names is created.The JSX <ul> element is used to define an unorder ed list.Within the <ul>, the map() function iterates over each item in the names array. For eac h item, a new <li> element is created.The key prop in each <li> element is crucial. It helps Re act maintain internal consistency and optimize re-rendering when the list changes. Each key must be unique among siblings.The expression {name} inside the <li> tags inserts the name i nto the list item.
This approach allows for efficient dynamic rendering of list elements based on data changes, which is a common pattern in React applications.
[Trivia]
The map() function is not specific to React; it's a standard JavaScript function used to transfor m arrays. When used in React, it must be combined with JSX by returning JSX elements for e ach array item. The use of key is a React-specific requirement for elements in a list or array to assist in efficient DOM updates.
Understanding Keys in React
Keys are special string attributes you need to include when creating lists of elements in React
. They help React track which items are changed, added, or removed.
Here’s a simple example demonstrating how to use keys properly in a React list component.
[Code]
function TaskList() {
const tasks = [
{ id: 1, text: 'Take out the trash' },
{ id: 2, text: 'Grocery shopping' },
{ id: 3, text: 'Read a book' }
];
return (
<ul>
{tasks.map(task => <li key={task.id}>{task.text}</li>)}
</ul>
);
}
[Result]
An unordered list (ul) with three list items (li) displaying the tasks: Take out the trash, Grocery shopping, and Read a book.
In this example:We define a component called TaskList.A tasks array is created with objects c ontaining id and text properties.The map() function iterates over each task, creating a <li> el ement for each one.The key prop for each <li> is set to task.id, ensuring that each key is uniq ue within the list. This helps React in efficiently updating and reordering list elements during dynamic changes.By providing a unique key, React can minimize DOM manipulation, which i mproves performance and reduces potential bugs in list updates.
[Trivia]
Using non-unique keys, such as indices or duplicated values, can lead to rendering errors an d performance issues in React applications. It is recommended to use unique identifiers like database IDs or unique values from your data as keys.
React Class Component Lifecycle Methods
Lifecycle methods in React class components are special functions that get called at different stages of a component's lifecycle.
Below is an example of a React class component using lifecycle methods such as component DidMount, componentDidUpdate, and componentWillUnmount.
[Code]
import React, { Component } from 'react';
class MyComponent extends Component {
constructor(props) {
super(props);
this.state = { counter: 0 };
console.log('Component constructed');
}
componentDidMount() {
console.log('Component did mount on the DOM');
this.timer = setInterval(() => {
this.setState(prevState => ({ counter: prevState.counter + 1 }));
}, 1000);
}
componentDidUpdate() {
console.log('Component did update');
componentWillUnmount() {
console.log('Component will unmount');
clearInterval(this.timer);
}
render() {
return <h1>Counter: {this.state.counter}</h1>;
}
}
export default MyComponent;
[Result]
Initially, the console logs "Component constructed" and "Component did mount on the DO
M". Then, it logs "Component did update" every second as the counter updates.
Lifecycle methods are essential for managing resources in a React component. componentDi dMount is used to perform tasks like fetching data, setting up subscriptions, or timers after t he component is initially rendered. componentDidUpdate allows you to react to changes in p rops or state. It is often used for network requests or updating the DOM in response to state changes. componentWillUnmount is crucial for cleaning up resources like event listeners or ti mers to prevent memory leaks.
[Trivia]
React 16.3 introduced new lifecycle methods and deprecated some old ones to optimize perf ormance and avoid bugs related to async rendering. For instance, getDerivedStateFromProps and getSnapshotBeforeUpdate were introduced while componentWillMount, componentWill ReceiveProps, and componentWillUpdate were deprecated.
React Context API
The Context API is a React structure that enables you to share values across different compo nents without passing props explicitly at every level.
Here's a simple example of how to use React's Context API to pass a user theme preference a cross a component tree.
[Code]
import React, { createContext, useContext, useState } from 'react'; const ThemeContext = createContext();
function App() {
const [theme, setTheme] = useState('dark');
return (
<ThemeContext.Provider value={{ theme, setTheme }}>
<Toolbar />
</ThemeContext.Provider>
);
}
function Toolbar() {
return (
<div>
<ThemedButton />
</div>
}
function ThemedButton() {
const { theme, setTheme } = useContext(ThemeContext);
return (
<button onClick={() => setTheme(theme === 'dark' ? 'light' : 'dark')}> Switch to {theme === 'dark' ? 'light' : 'dark'} theme
</button>
);
}
export default App;
[Result]
Clicking the button toggles the theme between 'dark' and 'light', demonstrating how the the me state is shared across components.
The Context API is a powerful feature for avoiding "prop drilling" — the process of passing p rops through many layers of a component hierarchy. In the example, ThemeContext.Provider wraps the components that need access to the theme state. Any component within this provi der can access the theme data using useContext, regardless of how deep it is in the compon ent tree. This simplifies component code and improves maintainability.
[Trivia]
Using the Context API can lead to performance issues if not managed properly because it ca n cause unnecessary re-renders in consumer components when the context value changes. It
's important to optimize context value updates and possibly use memoization techniques to prevent these issues.
Understanding Higher-order Components in React
Higher-order components (HOCs) are functions that take a component and return a new co mponent, enhancing the original with additional features or data.
The following example demonstrates a basic HOC that adds extra props to a component.
[Code]
// A simple React component
const SimpleComponent = props => <div>Hello, {props.name}!</div>;
// Higher-order component that adds additional props
function withExtraProps(WrappedComponent) {
return function(props) {
const extraProps = {name: 'Alice'};
return <WrappedComponent {...props} {...extraProps} />;
};
}
// Enhanced component using HOC
const EnhancedComponent = withExtraProps(SimpleComponent);
// Rendering the EnhancedComponent
ReactDOM.render(<EnhancedComponent />, document.getElementById('root'));
[Result]
The rendered output will display: "Hello, Alice!"
This example shows how a HOC (withExtraProps) takes SimpleComponent as an input and re turns a new component (EnhancedComponent). Here’s a breakdown:SimpleComponent is a basic functional component that displays a greeting message including the name passed as a prop.withExtraProps is a function that returns another function. This pattern is typical in HOC
s to allow additional customization and reusability.Inside the returned function, extraProps is defined and combined with any props passed to the enhanced component using {...props} sp read syntax.EnhancedComponent is the result of applying withExtraProps to SimpleCompone nt. It automatically receives the name prop set to "Alice" due to the HOC.This method is parti cularly useful for reusing component logic like handling subscriptions or injecting props, with out modifying the original component structure.
[Trivia]
HOCs are crucial for abstracting and isolating behaviors in React applications, such as connec ting to Redux stores or interacting with routers, without altering the original components' co de. They should be used judiciously as they can introduce complexity, particularly around pro p forwarding and ref handling.
Exploring React Router
React Router allows for the navigation among various views within a React application using a declarative approach.
Below is an example of basic routing setup with React Router in a new React application.
[Code]
import React from 'react';
import ReactDOM from 'react-dom';
import { BrowserRouter as Router, Route, Switch, Link } from 'react-router-dom';
// Component for Home page
const Home = () => <div>Home Page</div>;
// Component for About page
const About = () => <div>About Us</div>; function App() {
return (
<Router>
<div>
<nav>
<Link to="/">Home</Link>
<Link to="/about">About</Link>
</nav>
<Switch>
<Route exact path="/" component={Home} />
<Route path="/about" component={About} />
</Switch>
</div>
</Router>
);
}
// Render the App component to the DOM
ReactDOM.render(<App />, document.getElementById('app'));
[Result]
The app will display a navigation bar with links to "Home" and "About." Clicking these links w ill render the corresponding component without reloading the page.
This code illustrates a basic setup for client-side routing using React Router:BrowserRouter (a liased as Router) wraps the entire application to handle the URL logic.Inside Router, nav cont ains Link components that enable navigation without page reloads. Link changes the URL an d controls which component gets rendered based on the route.Switch is used to group Rout e components, ensuring only one route is rendered at a time. It looks through its children Ro ute elements and renders the first one that matches the current URL.Route components defi ne the mapping between the URL paths and the components to be rendered. The exact prop in the Home route ensures that it only matches when the path is exactly /.React Router enha nces the user experience by managing the navigation history internally, which supports forwa rd and back buttons natively in the browser.
[Trivia]
React Router's declarative approach aligns with React's philosophy of describing interfaces in a declarative manner. It simplifies debugging and testing as the components behave consiste
ntly based on the URL state. This library also supports dynamic routing, which can be leverag ed to load route configuration lazily.
Controlled Components in React
In React, controlled components manage form inputs where the form data is controlled by th e component's state.
Below is an example of a controlled component using a simple form with an input field and a submit button.
[Code]
import React, { useState } from 'react';
function ControlledForm() {
const [inputValue, setInputValue] = useState('');
const handleChange = (event) => {
setInputValue(event.target.value);
};
const handleSubmit = (event) => {
event.preventDefault();
alert(À name was submitted: ${inputValue}`);
};
return (
<form onSubmit={handleSubmit}>
<label>
Name:
<input type="text" value={inputValue} onChange={handleChange} />
<button type="submit">Submit</button>
</form>
);
}
export default ControlledForm;
[Result]
If the user types "John Doe" in the input field and clicks submit, an alert box pops up displayi ng "A name was submitted: John Doe".
In a controlled component, every state mutation has an associated handler function. This app roach makes it straightforward to modify or validate user input. For instance, the handleChan ge function is triggered every time the user types into the input field, updating the inputValu e state with the current value of the input field. When the form is submitted via the handleSu bmit function, since the component's state is the single source of truth, it can reliably use the state's current value. This makes controlled components very predictable and easy to debug.
The use of useState in this example initializes the state variable inputValue with an empty stri ng. This state is then bound to the input element's value and updated on every change, ensu ring the UI is always in sync with the state.
[Trivia]
Controlled components in React are similar to how forms are handled in traditional HTML for ms where every input's state is directly managed by the DOM, except React abstracts the for m state management to be handled via component state.
Uncontrolled Components in React
Uncontrolled components in React are where form data is handled by the DOM, rather than by the React state.
Here is an example of an uncontrolled component that uses a ref to access the input value di rectly from the DOM.
[Code]
import React, { useRef } from 'react';
function UncontrolledForm() {
const inputRef = useRef(null);
const handleSubmit = (event) => {
event.preventDefault();
alert(À name was submitted: ${inputRef.current.value}`);
};
return (
<form onSubmit={handleSubmit}>
<label>
Name:
<input type="text" ref={inputRef} />
</label>
<button type="submit">Submit</button>
</form>
}
export default UncontrolledForm;
[Result]
If the user types "Jane Doe" in the input field and clicks submit, an alert box pops up displayi ng "A name was submitted: Jane Doe".
Uncontrolled components are easier to integrate with non-React code as they rely less on th e component's state and more on the DOM itself. By using useRef, we create a reference (inp utRef) to the input element. This reference provides direct access to the input DOM node, all owing you to retrieve its current value at any time without needing to sync it with the compo nent's state.This approach is useful when you need quick access to the value without managi ng it through React's re-render cycle, making it ideal for cases like managing focus, text selec tion, or integrating with third-party DOM libraries.
[Trivia]
Using uncontrolled components in React can simplify certain tasks that involve direct interact ion with the DOM, similar to traditional JavaScript and HTML handling of form data. It provid es an escape hatch from the controlled component pattern when you need it.
Using React Fragments
Fragments in React allow you to group multiple elements without adding extra nodes to the DOM.
The following example shows how to use a React Fragment to return multiple elements from a component:
[Code]
import React from 'react';
function App() {
return (
<>
<h1>Hello, world!</h1>
<p>Welcome to my React app.</p>
</>
);
}
export default App;
[Result]
The browser will display the header "Hello, world!" followed by the paragraph "Welcome to my React app." without any extra parent divs in the DOM.
React Fragments are useful for wrapping lists of items or any component output without add ing unnecessary tags to the DOM. This is particularly useful for CSS styling and performance, as it reduces the depth of the DOM tree and the complexity of DOM updates. In the code, <
> and </> are shorthand syntax for <React.Fragment> and </React.Fragment>, respectively.
[Trivia]
Using React Fragments instead of additional divs can significantly simplify the DOM structure
, making your app's rendering faster and less memory-intensive, particularly on large-scale a pplications.
Using PropTypes in React
PropTypes in React is used to check the types of props passed into components to ensure th ey match expected types.
Below is an example demonstrating how to use PropTypes in a React component to validate prop types:
[Code]
import React from 'react';
import PropTypes from 'prop-types';
function UserProfile({ name, age }) {
return (
<div>
<h1>{name}</h1>
<p>Age: {age}</p>
</div>
);
}
UserProfile.propTypes = {
name: PropTypes.string.isRequired,
age: PropTypes.number
};
export default UserProfile;
[Result]
If the component receives props of incorrect types, console warnings will be displayed in the development mode.
PropTypes provides a range of validators to ensure the data you receive is valid. If name or a ge do not match the specified types (string for name and number for age), React will log a w arning in the console during development. This helps catch and debug type errors early in th e development process. Notably, PropTypes does not affect performance in production as it i s only active during development.
[Trivia]
Utilizing PropTypes effectively can prevent bugs related to unexpected data types, especially when working in large teams or with complex data structures. It can serve as a form of docu mentation, making it clearer what types of data components expect.
Using Refs in React
Refs in React allow you to access DOM nodes directly.
Here is a simple example of using refs to focus a text input element in a React component.
[Code]
import React, { useRef, useEffect } from 'react';
function TextInputWithFocus() {
const inputEl = useRef(null);
useEffect(() => {
// Automatically focus the input using the ref
inputEl.current.focus();
}, []);
return (
<div>
<input ref={inputEl} type="text" />
</div>
);
}
export default TextInputWithFocus;
[Result]
The text input field will be automatically focused when the component mounts.
Refs provide a way to directly interact with an element in the DOM. In React, you use the use Ref hook to create a ref, which is then attached to a DOM element using the ref attribute. Th e current property of the ref object points to the DOM element, allowing direct manipulation like focusing an input or changing its value. This bypasses the standard React data flow (prop s and state), so it should be used sparingly and only when necessary.
[Trivia]
Refs are not just for DOM access; they can also be used to store any mutable value that you want to persist across renders without causing a re-render. This is useful for values that need to persist but do not directly influence the output of the render method.
Using the Children Prop in React
The children prop in React allows you to pass elements directly into components.
Here's how you can create a wrapper component that accepts children elements and renders them.
[Code]
import React from 'react';
function WrapperComponent({ children }) {
return (
<div className="wrapper">
{children}
</div>
);
}
export default WrapperComponent;
[Result]
The WrapperComponent renders whatever children are passed to it within a div with a class of "wrapper".
The children prop is automatically passed to every component that receives content between its opening and closing tags in JSX. It allows you to build components that are agnostic abou t what they render inside. This is crucial for creating reusable component libraries. You can p ass multiple elements, strings, numbers, or even other components as children, making the c omponent highly flexible in different usage contexts.
[Trivia]
In addition to rendering children directly, the children prop can be manipulated, such as wra pping each child in additional markup or logic, filtering certain children, or even cloning each child with new props using React.Children utilities and React.cloneElement. This makes the ch ildren prop extremely powerful for creating high-order components and wrappers.
Understanding React.memo
React.memo is a higher order component used to optimize performance by preventing unne cessary re-renders of function components.
Below is an example where React.memo is used to prevent a functional component from re-r endering unless its props change.
[Code]
import React, { useState } from 'react';
const MyComponent = React.memo(function MyComponent(props) {
console.log('Rendering:', props.name);
return <h1>Hello, {props.name}!</h1>;
});
function App() {
const [name, setName] = useState('John');
const [counter, setCounter] = useState(0);
return (
<div>
<MyComponent name={name} />
<button onClick={() => setCounter(counter + 1)}> Increment Counter
</button>
<button onClick={() => setName('John')}>
</button>
</div>
);
}
export default App;
[Result]
In the web application, the console will log "Rendering: John" initially. When the counter is in cremented, the component does not re-render, hence no additional console log. If the name is changed to something else and then back to "John," it will log again only if the name actua lly changes.
In the given code, React.memo is wrapping MyComponent. This setup ensures that MyComp onent only re-renders if its props change. In our example, name is a prop. When the button t o increment the counter is clicked, despite the parent component's state changing, MyComp onent does not re-render because its props (name) remain unchanged. This is critical for imp roving performance in complex applications where unnecessary renders can lead to sluggish behavior.The useState hook in App manages the state for name and counter. The setName f unction is designed to update name, and similar for counter with setCounter. Notice the usa ge of console.log to demonstrate when the re-render happens.
[Trivia]
Using React.memo is particularly beneficial in projects with deep component trees or large lis ts where re-renders can be expensive in terms of performance. It does not prevent re-render s due to internal state changes or context changes.
Utilizing Custom Hooks
Custom hooks allow the reuse of stateful logic across multiple React components.
Here's an example of a custom hook called useFormInput that manages form input states, w hich can be reused in multiple components.
[Code]
import React, { useState } from 'react';
function useFormInput(initialValue) {
const [value, setValue] = useState(initialValue);
function handleChange(e) {
setValue(e.target.value);
}
return {
value,
onChange: handleChange
};
}
function LoginForm() {
const username = useFormInput('');
const password = useFormInput('');
function handleSubmit(event) {
event.preventDefault();
console.log('Login with:', username.value, password.value);
}
return (
<form onSubmit={handleSubmit}>
<label>
Username:
<input type="text" {...username} />
</label>
<label>
Password:
<input type="password" {...password} />
</label>
<button type="submit">Login</button>
</form>
);
}
export default LoginForm;
[Result]
On form submission, the console will display "Login with:" followed by the username and pas sword entered.
The useFormInput custom hook simplifies form handling by encapsulating the logic for mana ging input states (value and onChange handler). This makes it easy to manage multiple form fields with minimal repeated code. Each call to useFormInput returns an object containing pr operties and methods related to a particular input field, such as value and onChange. This ob ject can then be spread into input elements in the JSX (<input {...username}), effectively bindi ng the input’s state to the custom hook.This pattern not only reduces boilerplate but also en hances modularity and testability by isolating state management logic into reusable hooks.
[Trivia]
Custom hooks are a powerful feature introduced in React 16.8, allowing developers to extract component logic into reusable functions. This approach helps in building cleaner and more maintainable React applications, especially when handling complex stateful logic that needs t o be used across several components.
Understanding Error Boundaries in React
Error boundaries are special React components that handle errors within their child compone nts, preventing the entire application from crashing.
Below is an example of an error boundary component in React. This component catches Java Script errors in its child components, logs these errors, and displays a fallback UI instead of cr ashing.
[Code]
import React, { Component } from 'react';
class ErrorBoundary extends Component {
constructor(props) {
super(props);
this.state = { hasError: false };
}
static getDerivedStateFromError(error) {
// Update state so the next render will show the fallback UI.
return { hasError: true };
}
componentDidCatch(error, info) {
// You can also log the error to an error reporting service console.log(error, info);
}
if (this.state.hasError) {
// You can render any custom fallback UI
return <h1>Something went wrong.</h1>;
}
return this.props.children;
}
}
[Result]
When an error occurs in any child component, the error boundary catches it and displays "So mething went wrong."
Error boundaries are crucial for improving the user experience in a React application. By catc hing errors in component trees, they prevent the whole app from unmounting when an error occurs. Instead, only the component tree within the boundary is affected. This approach help s in isolating faulty components from the rest of the app, making debugging easier and impr oving overall stability.
[Trivia]
Error boundaries do not catch errors for:Event handlersAsynchronous code (e.g., setTimeout or requestAnimationFrame callbacks)Server-side renderingErrors thrown in the error boundar y itself (rather than its children)
Exploring Portals in React
React portals provide a method for rendering components into a part of the DOM outside of the parent component’s DOM hierarchy.
The following example shows how to use a portal to render a modal dialog. This dialog will b e rendered directly in the body element, outside of the root div of the React app.
[Code]
import React from 'react';
import ReactDOM from 'react-dom';
class Modal extends React.Component {
render() {
return ReactDOM.createPortal(
this.props.children,
document.body
);
}
}
function App() {
return (
<div>
<h1>Hello, world!</h1>
<Modal><p>This is a modal</p></Modal>
);
}
export default App;
[Result]
The modal content "This is a modal" is rendered directly into the body, outside the main app container.
Portals are a powerful feature in React for managing overlays, modals, and other cases where you need to break out of the DOM hierarchy. They are particularly useful for full-screen mod als and tooltips that need to escape the CSS overflow and stacking context issues caused by other components. By rendering children into a different part of the DOM, portals help maint ain proper event bubbling and state management, as they still behave like any other React c hild component.
[Trivia]
Events fired from inside a portal will propagate to ancestors in the containing React tree, eve n though those elements might not be ancestors in the DOM tree. This ensures consistent ev ent handling across your application.
Lazy Loading in React
React Lazy and Suspense simplify the process of asynchronously loading components to imp rove web application performance.
The following example demonstrates how to use React.lazy() and <Suspense> to lazy load a component.
[Code]
import React, { Suspense, lazy } from 'react';
const LazyComponent = lazy(() => import('./LazyComponent')); function App() {
return (
<div>
<Suspense fallback={<div>Loading...</div>}>
<LazyComponent />
</Suspense>
</div>
);
}
export default App;
[Result]
The screen will initially show "Loading..." until LazyComponent is loaded, then it will display t he LazyComponent's content.
When using React.lazy and <Suspense>, components are only loaded when they are needed, significantly reducing the initial load time. React.lazy takes a function that must call a dynami c import(), which returns a Promise. The <Suspense> component wraps lazy components an d uses fallback to show a UI element while the components are loading. This method is parti cularly useful for large components that are not immediately necessary, helping to keep the i nitial bundle size smaller and improve the time to interactive.
[Trivia]
React.lazy and <Suspense> can only be used in default exports of a module. They are not yet available for server-side rendering. This feature works best for improving user experience by splitting code at a finer level, which can be crucial for maintaining a fast-rendering and respo nsive interface.
Server-Side Rendering with React
Server-side rendering (SSR) involves rendering components on a server and sending the HT
ML to the client.
Here's a basic example to demonstrate how server-side rendering can be implemented in a R
eact application.
[Code]
import express from 'express';
import React from 'react';
import ReactDOMServer from 'react-dom/server';
import App from './App';
const server = express();
server.get('/', (req, res) => {
const renderedContent = ReactDOMServer.renderToString(<App />); res.send(`<html><body>${renderedContent}</body></html>`);
});
server.listen(3000);
[Result]
When a user accesses the server at the homepage, the server returns a page with the rendere d HTML of the React application.
Server-side rendering (SSR) allows a React application to render components on the server a nd send them as static HTML to the browser. This process enhances SEO as search engines ar e able to crawl the content more effectively. It also improves the perceived load time for user s because they receive content faster, though it may delay interactivity as JavaScript still need s to be loaded and run on the client. Implementing SSR requires a Node.js server environme nt, such as Express.js, to handle requests and render components.
[Trivia]
SSR can be combined with client-side rendering strategies (hydration) for an optimized balan ce of performance and interactivity. This means that while the initial render happens on the s erver to provide a quick, interactive version, React re-hydrates the application on the client si de to make it fully interactive.
Static Site Generation in Next.js
Static site generation (SSG) is a method where web pages are pre-rendered during the build process. Using Next.js, a React framework, enhances website performance and search engine optimization by generating static HTML in advance.
Below is an example demonstrating how to implement a simple static page in Next.js:
[Code]
// pages/index.js in a Next.js project
import Head from 'next/head';
export default function Home() {
return (
<div>
<Head>
<title>My Static Site</title>
<meta name="description" content="A statically generated site using Next.js" />
</Head>
<main>
<h1>Welcome to My Static Website</h1>
<p>This page is pre-rendered at build time using Next.js</p>
</main>
</div>
);
[Result]
The page is pre-rendered at build time, and the HTML is served statically.
When using SSG with Next.js, the getStaticProps or getStaticPaths functions are not needed f or simple static content. The above code generates a basic static HTML page that is pre-rend ered when you build your Next.js application. This pre-rendering means that the server does not need to render the HTML for each request, leading to faster load times and reducing serv er load. Additionally, because the HTML is already created, search engines can easily crawl an d index the pages, which improves SEO performance.
[Trivia]
SSG is particularly beneficial for websites with content that does not change often, making it ideal for blogs, documentation, and product pages. Next.js supports incremental static regen eration (ISR), which allows pages to be updated at predetermined intervals without needing t o rebuild the entire site.
Event Handling in React
React uses a synthetic event system to handle events like clicks, key presses, and other user i nteractions. This system wraps the browser's native events, providing a consistent interface a cross different browsers.
Below is an example showing how to handle a click event in a React component:
[Code]
import React from 'react';
class MyButton extends React.Component {
handleClick = () => {
alert('Button clicked!');
};
render() {
return <button onClick={this.handleClick}>Click Me!</button>;
}
}
export default MyButton;
[Result]
When the button is clicked, an alert pops up displaying "Button clicked!".
React's synthetic event system creates a wrapper around the browser's native events. This me ans that when an event like a click occurs, React interprets this event through its own event s ystem before it reaches your event handler like handleClick. This system ensures that all prop erties and behaviors of events are consistent across different browsers, regardless of their nat ive implementations. React pools these synthetic events for better performance and reuses t hem, which is why you should not rely on the event in asynchronous code without calling ev ent.persist().
[Trivia]
React's event system is designed to be efficient by using event delegation. React doesn't atta ch event handlers to the actual DOM elements but instead listens for events at the top level of the document. When an event occurs, React maps it to the component that owns the han dler and then executes the handler if applicable. This approach reduces memory overhead an d increases the performance of dynamic applications.
React Inline Styles
In React, inline styles are defined using JavaScript objects rather than CSS strings.
Here’s a simple example of applying inline styles to a component in React:
[Code]
function App() {
const style = {
color: 'blue',
backgroundColor: 'yellow',
padding: '10px'
};
return <div style={style}>Hello, styled world!</div>;
}
[Result]
A div with text "Hello, styled world!" styled with blue color, yellow background, and 10px pad ding.
When using inline styles in React, styles are not written as a string but as an object. This Java Script object maps to CSS properties where the keys are the camelCased versions of the CSS
property names, and the values are the CSS values, usually represented as strings. For exampl e, backgroundColor corresponds to the CSS background-color. This method enhances JavaSc ript’s control over style manipulation, allowing for dynamic styles and complex conditional st yling logic. It also avoids some of the pitfalls of string-based styling, such as improper escapi ng of characters or injection vulnerabilities.
[Trivia]
Using inline styles in React has benefits like scope limitation (styles apply only to the compon ent and do not leak elsewhere) and dynamic styling capabilities. However, it's not always effic ient for complex applications due to lack of reusability, inability to use pseudo-classes like :h over, and less performance optimization compared to CSS stylesheets.
JSX Rendering Behavior
In JSX, certain values like true, false, null, and undefined do not render anything.
Here’s an example demonstrating how JSX ignores false, null, undefined, and true:
[Code]
function App() {
return (
<div>
{false}
{'Hello, world!'}
{null}
{undefined}
{true}
</div>
);
}
[Result]
A div containing only the text "Hello, world!".
JSX is a syntax extension for JavaScript used in React to describe what the UI should look like.
When rendering components, JSX will ignore false, null, undefined, and true values. This beha vior is useful for conditional rendering where you might want a component or element to onl y appear under certain conditions without having to explicitly handle the falsy values' renderi ng. This also keeps the rendered DOM cleaner by not inserting unnecessary text nodes or ele ments, which can improve performance and reduce DOM clutter.
[Trivia]
Understanding JSX's handling of these values can help prevent bugs related to unexpected r ender outputs. For example, developers can use this feature for conditional rendering like {co ndition && <Component />} which will render the component only if condition is true; if con dition is false, React renders nothing, simplifying the logic without needing a ternary operato r or an if-else block.
Using StrictMode in React
StrictMode is a React component that helps you write better code by identifying potential pr oblems.
Let’s see how to wrap a React component with StrictMode.
[Code]
import React, { StrictMode } from 'react';
import ReactDOM from 'react-dom';
import App from './App';
ReactDOM.render(
<StrictMode>
<App />
</StrictMode>,
document.getElementById('root')
);
[Result]
No visible UI changes.
StrictMode is a React tool used in development mode to highlight potential problems in an a pplication. It does not impact the behavior of your application but checks for common issues like the use of deprecated APIs, unexpected side effects in lifecycle methods, and more. This tool is particularly useful when you want to ensure your application adheres to the best pract ices and prepares for future updates of React.StrictMode wraps around any component. It do esn’t render any visible UI; instead, it activates additional checks and warnings for its descend ants. These checks occur only in development mode, so they don't affect production perform ance. Using StrictMode can help detect problems early during development, potentially savin g a lot of debugging time later.
[Trivia]
StrictMode checks for the following issues: identifying components with unsafe lifecycles, wa rning about legacy string ref API usage, detecting unexpected side effects, detecting legacy c ontext API, and more. It's important to note that while StrictMode helps in detecting proble ms, it doesn't automatically fix them; it requires the developer to address the issues identifie d.
Understanding React DevTools
React DevTools is a browser extension that provides a visual interface to debug your React a pplications.
Let’s explore how to install and use React DevTools to inspect a React component.
[Code]
// To install React DevTools, run the following command in your terminal: npm install --save-dev react-devtools
// After installation, you can open the React DevTools in a separate window with: npx react-devtools
[Result]
React DevTools opens in a new window.
React DevTools is an essential tool for developers working with React. It allows you to inspec t the React component hierarchies in the Chrome Developer Tools or Firefox Developer Tools
. After installing the tool as a browser extension or using it standalone, you can view the curr ent props and state of each component you click on.The tool also allows you to observe com ponent performance, track which components re-render, and why, and explore the context a
nd hooks in use. This deeper insight into React components and their lifecycle events can sig nificantly speed up debugging and development. DevTools also features capabilities like prof iling the performance of applications, visualizing component trees, and manipulating the stat e and props without touching the source code directly.
[Trivia]
React DevTools not only helps you inspect and manage the state and props of your compon ents but also offers advanced features like profiling and tracking component performance. T
hese features are invaluable for optimizing render performance and understanding the rende ring lifecycle of your application. Another notable feature is the ability to trace updates by hi ghlighting components that re-render as a result of state changes or props updates, helping identify unnecessary renders.
Setting Up React
Creating a React app with Create React App simplifies the setup process.
To create a new React project using Create React App, run the following command in your te rminal:
[Code]
npx create-react-app my-app
cd my-app
npm start
[Result]
Your default web browser will open, displaying a new React app running at localhost:3000.
Create React App is a CLI tool that automates the setup of a new React project. It sets up the development environment so you can start developing immediately without worrying about configuring webpack, Babel, or ESLint. Here are the key components it configures for you:We bpack - A module bundler that bundles your JavaScript files and assets together.Babel - A Ja vaScript compiler that lets you use ES6+ features and JSX in your React code.ESLint - A tool f or identifying and reporting on patterns found in ECMAScript/JavaScript code, making your c
ode more consistent and avoiding bugs.Development Server - The npm start command laun ches a development server, watches your files, and refreshes the page as you edit and save y our code.Create React App also sets up a sensible project structure, suggesting a location for your files and setting up some initial templates like a root App component.
[Trivia]
Create React App comes with a .gitignore file configured, which includes common directories and files that should not be committed to a Git repository, such as node_modules/ and .env f iles.
Using useEffect Hook
The useEffect hook lets you perform side effects in function components.
Here is an example of using useEffect to fetch data from an API and display it in a React com ponent:
[Code]
import React, { useState, useEffect } from 'react';
function App() {
const [data, setData] = useState(null);
useEffect(() => {
fetch('https://api.example.com/data')
.then(response => response.json())
.then(data => setData(data))
.catch(error => console.error('Error fetching data:', error));
}, []); // The empty array ensures this effect runs only once after the initial render return (
<div>
{data ? <pre>{JSON.stringify(data, null, 2)}</pre> : 'Loading...'}
</div>
);
}
export default App;
[Result]
If successful, the fetched data will be displayed formatted in JSON. If there’s an error, it will s how "Error fetching data" in the console.
The useEffect hook is one of the most powerful features in React for managing side effects in functional components. Side effects are actions that can affect other components or can't be done during rendering, such as data fetching, timers, logging, and manually manipulating th e DOM of the page.Key points about useEffect:Dependencies Array - The second parameter of useEffect is a dependencies array. If it's empty ([]), the effect runs only once after the initial render. If it contains values, the effect will rerun when those values change.Cleaning up - If y our effect sets up a subscription or a timer, you need to return a cleanup function from the e ffect that will remove the subscription or timer when the component unmounts or before the effect runs again.Understanding useEffect is crucial for handling operations that need to be s ynchronized with the component lifecycle.
[Trivia]
useEffect replaces several lifecycle methods from class components, such as componentDid Mount, componentDidUpdate, and componentWillUnmount, offering a unified API for handli ng side effects in functional components.
Understanding useReducer in React
useReducer is a hook in React used for state management, especially useful in complex scena rios compared to useState.
Below is an example showing how to use useReducer to manage state in a component that h andles a counter with increment and decrement functionality.
[Code]
import React, { useReducer } from 'react';
function reducer(state, action) {
switch (action.type) {
case 'increment':
return { count: state.count + 1 };
case 'decrement':
return { count: state.count - 1 };
default:
return state;
}
}
function Counter() {
const [state, dispatch] = useReducer(reducer, { count: 0 }); return (
<div>
<button onClick={() => dispatch({ type: 'increment' })}>+</button>
<button onClick={() => dispatch({ type: 'decrement' })}>-</button>
</div>
);
}
export default Counter;
[Result]
The web page displays a counter with buttons to increment and decrement the count.
In this example, useReducer is particularly effective for managing state transitions in a predic table way. The reducer function defines how the state changes in response to each action dis patched. It's a pure function, meaning given the same arguments, it will always produce the s ame result without side effects. This makes the state management more predictable and easi er to debug.The useReducer hook is generally preferable over useState when you have comp lex state logic that involves multiple sub-values or when the next state depends on the previ ous one. This is because it lets you centralize the state logic in one place, making it easier to understand and manage, especially in larger components or in components that involve com plex state interactions.
[Trivia]
Understanding when to use useReducer instead of useState is crucial. A good rule of thumb i s that if you find yourself syncing states or dealing with conditions where how one state chan ges depends on another state, useReducer might be the better option. It's also great for case s where the new state depends on the old state directly, as it avoids closures problems in Jav aScript that can lead to bugs.
Using TypeScript with React
TypeScript enhances React projects by providing static type checking, which helps improve c ode quality and maintainability.
The following is an example of a simple React component with TypeScript, showing how stati c types can be applied for better code reliability and readability.
[Code]
import React from 'react';
interface Props {
message: string;
}
const Greeting: React.FC<Props> = ({ message }) => {
return <h1>Hello, {message}!</h1>;
}
export default Greeting;
[Result]
The web page displays a greeting message like "Hello, world!".
In this TypeScript example, the Props interface defines the type of props that the Greeting co mponent expects, which is a single message of type string. By using TypeScript, you ensure t hat the component receives the right type of props, reducing runtime errors and improving t he component's reliability.TypeScript provides several key benefits when used with React:Typ e Safety: Catching errors at compile time rather than at runtime, which can save time and red uce bugs in production.Readability: By providing explicit types, your code becomes more rea dable and easier to understand, especially for new developers or when returning to a project after a long time.Refactoring: Safe and easy refactoring of code, knowing that type checks wil l catch errors related to changing props, state, or other data structures.
[Trivia]
Integrating TypeScript into a React project can seem daunting at first, but the benefits in ter ms of code quality and developer productivity are significant. Many companies have adopted TypeScript for their React projects to leverage these advantages, reporting fewer bugs and b etter developer experience in maintaining large codebases.
Dynamic Imports in React
Dynamic imports in React allow you to load components as they are needed, which can signi ficantly reduce the initial loading time of your application.
Here is a simple example of how to use dynamic imports in a React application to load a com ponent only when it is required.
[Code]
import React, { Suspense } from 'react';
const LazyComponent = React.lazy(() => import('./LazyComponent')); function App() {
return (
<div>
<Suspense fallback={<div>Loading...</div>}>
<LazyComponent />
</Suspense>
</div>
);
}
export default App;
[Result]
When navigating to a page that requires LazyComponent, you would see a "Loading..." mess age until the component is fully loaded.
In this example, React.lazy is used to dynamically import a component. LazyComponent is no t loaded until it is actually rendered in the component tree. Suspense is a React component t hat wraps lazy components and displays a fallback content (e.g., a loading indicator) until the lazy component is ready to render. This approach is particularly useful for improving perform ance in large applications, where reducing the size of the initial JavaScript bundle can lead to faster load times. This can also positively impact SEO and user experience by decreasing the t ime to first meaningful paint.
[Trivia]
Dynamic imports work by splitting your code at specified points and then loading segments (
chunks) when they are needed. This is made possible by the code-splitting feature supported in modern build tools like Webpack and Parcel. Dynamic imports can thus significantly impro ve the scalability and performance of large applications.
React Reconciliation and Keys
React’s reconciliation algorithm uses keys to optimize rendering by identifying which items h ave changed, been added, or been removed.
Here is an example showing how to use keys properly in a React component that renders a li st of items.
[Code]
function ItemList({ items }) {
return (
<ul>
{items.map((item, index) => (
<li key={item.id}>{item.text}</li>
))}
</ul>
);
}
export default ItemList;
[Result]
Items in the list will maintain their identity across renders, which minimizes the amount of D
OM operations required during updates.
In this example, each item in the list is assigned a unique key prop, which is recommended to be a stable identifier like an id from your data. The key prop helps React in its reconciliation process by giving it a way to associate components with their state across updates and recog nize when elements in a list have changed, moved, or stayed the same. Using keys effectively can help avoid unnecessary re-renders and improve performance, especially in large lists or c omplex UIs. It's important to avoid using an index as a key if the order of items can change, a s this can negatively affect performance and lead to issues with component state.
[Trivia]
Keys in React are not just for performance optimization—they also help maintain state in dyn amically generated components. If keys are not properly assigned or are based on indices th at can change, React might recreate the component state unnecessarily, leading to bugs and inefficient updates.
Component Communication in React
In React, components can communicate using props or context.
Here's a simple example of parent to child communication using props:
[Code]
function ParentComponent() {
const message = "Hello from Parent!";
return <ChildComponent parentMessage={message} />;
}
function ChildComponent(props) {
return <h1>{props.parentMessage}</h1>;
}
[Result]
The child component displays: Hello from Parent!
In this example, ParentComponent passes a message to ChildComponent using props. Props are a way of passing data from parent components to child components in React. The data fl ows in one direction, from parent to child, making the state management predictable and ea
sier to understand. Here, parentMessage is the prop key assigned to pass the message "Hello from Parent!" to the child. The child component then accesses this prop and renders it within an <h1> tag, resulting in the displayed message.
[Trivia]
Props not only pass data but can also pass callback functions, allowing child components to communicate back to their parent by calling those functions. This maintains a unidirectional data flow, which is a core principle of React's design.
Optimizing Performance with useCallback
Use useCallback to prevent unnecessary re-renders in React.
This example demonstrates the use of useCallback to avoid creating new functions on each r ender:
[Code]
import React, { useState, useCallback } from 'react';
function Counter() {
const [count, setCount] = useState(0);
const increment = useCallback(() => setCount(c => c + 1), []); return (
<div>
<p>Count: {count}</p>
<button onClick={increment}>Increment</button>
</div>
);
}
[Result]
When the button is clicked, the count increments without unnecessary component re-render s.
In this code, useCallback is used to wrap the increment function. This hook takes a function a nd a dependency array as its parameters. The function inside useCallback will only be re-crea ted if the values in the dependency array change, which in this case is empty ([]), indicating t hat increment does not depend on any props or state. This means the same function object i s retained across renders, preventing unnecessary re-renders of components that rely on this function, like our button here. This is crucial for optimizing performance, especially in comple x applications where re-rendering can be costly.
[Trivia]
useCallback is particularly useful when passing callbacks to optimized child components that rely on reference equality to prevent unnecessary renders (e.g., components wrapped in Reac t.memo). This hook helps maintain the same function reference across renders unless depen dencies change, thus supporting performance optimizations.
Testing React Components
For testing React components, developers often use Jest for unit tests and React Testing Libr ary for integration tests.
Below is an example of a simple React component test using Jest and React Testing Library.
[Code]
import React from 'react';
import { render, screen } from '@testing-library/react'; import App from './App';
test('renders learn react link', () => {
render(<App />);
const linkElement = screen.getByText(/learn react/i);
expect(linkElement).toBeInTheDocument();
});
[Result]
No visible result (test output would be in the console).
This code snippet demonstrates how to set up a basic test for a React component called App
. First, the App component is imported along with necessary functions from React Testing Lib rary. The test labeled 'renders learn react link' uses the render method from React Testing Lib rary to render the App component into a virtual DOM for testing purposes.screen.getByText i s a query provided by React Testing Library that returns the first matching node for the provi ded text content, throwing an error if no elements match or if more than one match is found.
This method is particularly useful for ensuring that specific text appears in the component.Th e expect function from Jest is then used to assert that the link element is indeed present in th e document. This is a basic example of an integration test where components are tested in th e context of how they interact with each other and with their environment.
[Trivia]
Jest is a popular testing framework that provides a simple way to write tests using a rich API f or assertions. React Testing Library is a set of helpers that encourage good testing practices by focusing on testing components in a way that users actually interact with your app (i.e., vi a the UI).
React Fast Refresh
React Fast Refresh is a development feature that automatically reloads your React componen ts when changes are detected, while preserving their state.
An illustration of how React Fast Refresh works during development.
[Code]
// Normally, this is configured by default in development environments like Create React App
.
// After making changes to your component code:
console.log('Component code updated, React Fast Refresh kicks in!');
[Result]
"Component code updated, React Fast Refresh kicks in!"
React Fast Refresh seamlessly updates the UI without losing component state, which is crucia l during development for maintaining the application's runtime state across updates. This fea ture is especially helpful when working on stateful components or complex UIs, as it allows d evelopers to see changes in real-time without resetting the state of the application.This featu re works under the hood in modern React environments like Next.js or Create React App. It r
eplaces the older Hot Module Replacement (HMR) technique and is specifically optimized for React to handle both functional and class components effectively.
[Trivia]
Fast Refresh not only reloads the affected components but also tries to re-import the modul e to fetch the latest version of the components from the server. If a syntax error is detected, Fast Refresh will retain the previous version of the module, avoiding screen crashes and impr oving developer productivity by reducing manual reloads.
Integrating Static Typing in React
Using static typing in React projects helps catch bugs early, making code more robust and m aintainable.
We'll integrate Flow, a static type checker, into a basic React component to demonstrate how it improves code reliability.
[Code]
// @flow
import React, { Component } from 'react';
type Props = {
name: string,
};
type State = {
age: number,
};
class UserProfile extends Component<Props, State> {
state = {
age: 0
};
componentDidMount() {
// Simulate fetching age data and updating state
setTimeout(() => this.setState({ age: 30 }), 1000);
render() {
const { name } = this.props;
const { age } = this.state;
return (
<div>
<h1>User Profile</h1>
<p>Name: {name}</p>
<p>Age: {age}</p>
</div>
);
}
}
export default UserProfile;
[Result]
After compiling and running, you'll see a component displaying a user's name and age, upda ted after a simulated fetch.
The example uses Flow to define types for props and state, ensuring that only the correct dat a types are passed and used within the component. This prevents common errors like passin g a number where a string is expected. By checking types at compile time, Flow helps identif y problems before the code runs, improving the overall development process by reducing ru ntime errors and debugging time.
[Trivia]
Flow is maintained by Facebook, the same company that developed React. It's designed to b e gradually adopted, meaning you can start using Flow in a few components without needin g to refactor your entire project.
Managing Global State with Redux
Redux is a predictable state container for JavaScript apps, commonly used with React to man age complex application states.
Here, we'll set up a simple Redux store and connect it to a React component to manage and display global state.
[Code]
import React from 'react';
import ReactDOM from 'react-dom';
import { createStore } from 'redux';
import { Provider, connect } from 'react-redux';
// Reducer function
function counter(state = 0, action) {
switch (action.type) {
case 'INCREMENT':
return state + 1;
case 'DECREMENT':
return state - 1;
default:
return state;
}
}
const store = createStore(counter);
// React component
const Counter = ({ value, onIncrement, onDecrement }) => (
<div>
<h1>{value}</h1>
<button onClick={onIncrement}>+</button>
<button onClick={onDecrement}>-</button>
</div>
);
// Mapping state and dispatch to props
const mapStateToProps = state => ({
value: state
});
const mapDispatchToProps = dispatch => ({
onIncrement: () => dispatch({ type: 'INCREMENT' }),
onDecrement: () => dispatch({ type: 'DECREMENT' })
});
const ConnectedCounter = connect(mapStateToProps, mapDispatchToProps)(Counter);
// Rendering the component
ReactDOM.render(
<Provider store={store}>
<ConnectedCounter />
</Provider>,
document.getElementById('root')
);
[Result]
The Counter component will display "0" initially, and the number will increase or decrease as you click the "+" or "-" buttons.
In this code snippet, the Redux store manages a simple numeric state that increments or dec rements. The Provider component from react-redux wraps the entire application, allowing an y component in the app to access the Redux store if connected through connect(). This patte rn is essential for large applications where multiple components need to access or modify th e application state in a predictable manner.
[Trivia]
Redux was created by Dan Abramov and Andrew Clark. It was inspired by the Flux architectur e and the Elm programming language, focusing on simplicity and scalability in application st ate management.
Managing Side Effects in Redux
Learn how to handle asynchronous operations in Redux using middleware like Redux Thunk or Redux Saga.
Below is a simple example of using Redux Thunk to fetch data asynchronously and dispatch a ctions based on the fetch result.
[Code]
import { createStore, applyMiddleware } from 'redux';
import thunk from 'redux-thunk';
// Reducer function
function reducer(state = { data: [], loading: false }, action) {
switch (action.type) {
case 'FETCH_DATA_REQUEST':
return { ...state, loading: true };
case 'FETCH_DATA_SUCCESS':
return { ...state, data: action.payload, loading: false }; case 'FETCH_DATA_FAILURE':
return { ...state, loading: false };
default:
return state;
}
}
// Action creator that returns a function (enabled by Redux Thunk) const fetchData = () => {
return (dispatch) => {
dispatch({ type: 'FETCH_DATA_REQUEST' });
fetch('https://api.example.com/data')
.then(response => response.json())
.then(data => dispatch({ type: 'FETCH_DATA_SUCCESS', payload: data }))
.catch(error => dispatch({ type: 'FETCH_DATA_FAILURE' }));
};
};
// Create store with middleware
const store = createStore(reducer, applyMiddleware(thunk));
// Dispatch the asynchronous action
store.dispatch(fetchData());
[Result]
No direct output without running the code; expected actions to be dispatched showing loadi ng state and fetched data.
Redux Thunk allows functions instead of objects to be dispatched. These functions can execu te asynchronous operations and can dispatch multiple actions, like initiating a loading state, successfully fetching data, or handling an error. This is crucial for handling side effects in Red ux applications where state updates need to react to asynchronous events, such as API calls.R
edux Saga, another popular middleware, uses generator functions to manage side effects. It l istens for actions and can perform complex logic including asynchronous API calls, managing race conditions, and more, offering more control over side effects than Redux Thunk.
[Trivia]
Redux middleware like Thunk and Saga are essential for real-world applications where you n eed to interact with external APIs or perform complex state transitions. Understanding how t o use these tools effectively can significantly enhance your Redux application's robustness an d maintainability.
Enhancing Accessibility in React
React supports web accessibility and it's important to enhance it further using semantic HTM
L and ARIA roles.
Example of how to use semantic HTML and ARIA roles in a React component to improve acc essibility.
[Code]
import React from 'react';
function AccessibleButton() {
return (
<button aria-label="Delete item" role="button"> Delete
</button>
);
}
export default AccessibleButton;
[Result]
No output, as this is a component code; the rendered button would be accessible with ARIA roles added.
This example shows a simple use of ARIA roles in React components. ARIA (Accessible Rich In ternet Applications) roles provide additional context to assistive technologies, like screen rea ders. In this case, aria-label helps users understand the function of the button, even if the vis ual content is not clear.Using semantic HTML elements like <button>, <header>, <footer>,
<article>, and <section> also improves the accessibility as these elements provide inherent r oles that assistive technologies rely on to interpret page structure and functionality.
[Trivia]
Enhancing accessibility not only complies with web standards but also opens up your applica tions to a wider audience, including those with disabilities. Tools like the React Accessibility Li nter can help developers adhere to accessibility guidelines during development.
Using useMemo in React
Learn how to use the useMemo hook to optimize performance by memoizing expensive calc ulations in React applications.
The following example demonstrates how useMemo can be used to prevent re-computation of an expensive calculation (like a factorial) unless its dependencies change.
[Code]
import React, { useMemo, useState } from 'react';
function ExpensiveComponent() {
const [number, setNumber] = useState(5);
const factorial = useMemo(() => {
console.log('Calculating factorial');
const calculateFactorial = n => (n <= 0 ? 1 : n * calculateFactorial(n - 1)); return calculateFactorial(number);
}, [number]);
return (
<div>
<h1>Factorial of {number} is {factorial}</h1>
<button onClick={() => setNumber(number + 1)}>Increment</button>
</div>
);
}
export default ExpensiveComponent;
[Result]
Upon rendering and clicking the 'Increment' button, the console logs 'Calculating factorial' o nly when the number changes, not on every render.
The useMemo hook is essential in React to optimize performance by avoiding unnecessary c omputations. It takes a function and a list of dependencies. The function runs only when the dependencies have changed since the last render. This is particularly useful in large applicati ons where performance can be impacted by frequent updates and complex calculations. In t he example above, the factorial calculation is computationally expensive, and useMemo effici ently caches the result until the 'number' changes, thereby saving resources and enhancing p erformance.
[Trivia]
useMemo and useCallback are similar in that both cache computational results. However, use Memo returns the memoized value, while useCallback returns a memoized function. Knowing when to use which can significantly impact performance and maintainability of your applicati on.
Optimizing Bundle Sizes in React
Understand how to reduce bundle sizes in React applications by properly managing imports and employing code-splitting techniques like lazy loading.
This example showcases how to use React's lazy loading feature along with dynamic imports to split code and reduce the initial load time of your application.
[Code]
import React, { Suspense, lazy } from 'react';
const LazyComponent = lazy(() => import('./ExpensiveComponent')); function App() {
return (
<div>
<Suspense fallback={<div>Loading...</div>}>
<LazyComponent />
</Suspense>
</div>
);
}
export default App;
[Result]
When navigating to the part of the app that requires LazyComponent, it gets loaded dynami cally, and 'Loading...' is displayed until the component is ready.
React's lazy loading feature combined with Suspense allows components to be loaded dyna mically only when needed. This significantly reduces the initial load time and size of your app
's bundle, which can improve user experience, especially on slow networks. Lazy loading is im plemented using React.lazy which wraps a dynamic import statement. This tells React to load the component only when it's rendered. The Suspense component is used to handle the load ing state, showing a fallback UI until the lazy-loaded component is ready to display. It's cruci al for large applications that could be slowed down by loading unnecessary components upfr ont.
[Trivia]
Using tools like Webpack for bundle optimization can further enhance your application's perf ormance. Webpack analyzes your codebase and creates an optimized bundle by eliminating dead code and splitting your code into chunks. Techniques like tree shaking (removing unus ed code) and code splitting are fundamental to modern web development.
Using Keys in List Rendering
In React, keys help the virtual DOM manage elements efficiently when items in a list change.
Below is a simple example of rendering a list with unique keys in React.
[Code]
import React from 'react';
function ItemList() {
const items = [
{ id: 1, name: 'Item 1' },
{ id: 2, name: 'Item 2' },
{ id: 3, name: 'Item 3' }
];
return (
<ul>
{items.map(item => (
<li key={item.id}>{item.name}</li>
))}
</ul>
);
}
export default ItemList;
[Result]
The browser will display a list of items, each labeled as 'Item 1', 'Item 2', 'Item 3'.
In the example above, the map() function is used to iterate over an array of item objects, eac h with a unique id. By assigning this id as a key to each <li> element, React can efficiently up date the DOM when items are added, removed, or reordered. The key must be a unique iden tifier among siblings to help React maintain internal consistency and minimize DOM manipul ations, improving performance. If keys are duplicated, React will issue a warning and might n ot behave as expected, potentially leading to bugs in the application.
[Trivia]
It's important to avoid using indexes as keys unless the list is static and will not change. Usin g indexes can lead to performance issues and bugs during component updates because Rea ct uses keys to identify which items have changed, are added, or are removed.
State Management in Large Applications
As React applications grow, managing local state across multiple components can become c omplex.
Here's how you can use the Context API to manage state more globally across different com ponents.
[Code]
import React, { createContext, useContext, useState } from 'react'; const AppContext = createContext();
function AppProvider({ children }) {
const [user, setUser] = useState(null);
const login = (username) => {
setUser({ name: username });
};
const logout = () => {
setUser(null);
};
return (
<AppContext.Provider value={{ user, login, logout }}>
{children}
</AppContext.Provider>
);
function UserProfile() {
const { user, login, logout } = useContext(AppContext); return (
<div>
{user ? (
<div>
<p>Welcome, {user.name}!</p>
<button onClick={logout}>Logout</button>
</div>
) : (
<button onClick={() => login('Jane Doe')}>Login</button>
)}
</div>
);
}
function App() {
return (
<AppProvider>
<UserProfile />
</AppProvider>
);
}
export default App;
[Result]
The web page will display a login button initially. After logging in, it shows a welcome messa ge and a logout button.
The example uses React's Context API to provide a global state management mechanism tha t's simpler than props drilling. The AppProvider component creates a context that holds the user state and functions to manipulate it. Any component within the AppProvider can access the user state and functions via the useContext hook, allowing components to re-render whe n the state changes. This pattern is particularly useful in larger applications to avoid passing props deeply through multiple levels of components, simplifying the code and enhancing ma intainability.
[Trivia]
For even larger applications, you might consider using more sophisticated state managemen t libraries like Redux or MobX. These provide more powerful and flexible tools to handle stat e that's not just global but also has complex interactions across many components.
Immutable Props in React
In React, it's important to treat props as immutable to ensure components behave as expecte d.
Here’s a simple example showing a functional React component that receives props: