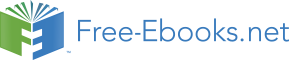

[Code]
function Greeting({ name }) {
return <h1>Hello, {name}!</h1>;
}
[Result]
If you pass a prop name="Alice" to this component, it will render: <h1>Hello, Alice!</h1>.
In React, props are inputs to components and should be considered immutable, meaning the y should not be changed by the component that receives them. This practice helps in avoidin g side effects and unexpected behaviors in applications, such as unnecessary re-renders or b ugs that are difficult to track down. When you pass props to a component, think of them as r ead-only configurations that the component can use but not alter. If you need to modify bas ed on the prop's value, use state or other forms of data handling inside the component. For
example, if a component needs to display a name in uppercase, you would not modify the pr op directly but rather use the prop to set state or compute a new value.
[Trivia]
Understanding the immutability of props in React is crucial for performance optimization. Re act's reconciliation process (the way React updates the DOM) can skip unnecessary updates when props remain unchanged, thus improving performance.
Shallow vs. Deep Copy in JavaScript
Knowing the difference between shallow and deep copying in JavaScript is essential for prop er state management in React.
Here’s a basic example of shallow copying using the spread operator and the pitfalls it might entail:
[Code]
const original = { name: 'Alice', details: { age: 25 } }; const shallowCopy = { ...original };
console.log('Original:', original);
console.log('Shallow Copy:', shallowCopy);
shallowCopy.details.age = 30;
console.log('Updated Shallow Copy:', shallowCopy);
[Result]
Original: { name: 'Alice', details: { age: 25 } }
Shallow Copy: { name: 'Alice', details: { age: 25 } }
Updated Shallow Copy: { name: 'Alice', details: { age: 30 } }
In JavaScript, shallow copying means that the outer layer of an object is copied, but any nest ed objects are still referenced. This is important in React, especially when managing state, be cause mutating nested objects within a shallow copy affects the original object, leading to po tential bugs. In the example above, changing age in the shallow copy also changes age in the original object because the nested object { details: { age: 25 } } is shared. To avoid these issue s, deep copying might be necessary where every level of nested objects is copied. This can b e achieved using libraries like Lodash with its _.cloneDeep() method or by using other techni ques like serialization with JSON.parse(JSON.stringify(object)).
[Trivia]
Using shallow copying appropriately can improve performance in React by avoiding unneces sary deep copies. However, understanding when a deep copy is needed helps prevent subtle bugs that can arise from unintended shared references in your state management.
Using React Native for Mobile Development
React Native is a framework that allows developers to build mobile applications using JavaSc ript and React.
Here is a simple example of creating a new React Native app using the React Native CLI.
[Code]
npx react-native init MyNewProject
[Result]
This command sets up a new React Native project named "MyNewProject" with all necessary dependencies.
React Native extends React's component-based architecture to mobile development, allowin g for the creation of native apps for both iOS and Android using a single codebase. This is ac hieved by mapping React components to native platform components. React Native comes with a hot-reloading feature, which speeds up development by automatically reloading your app as you make changes to the code.Using the npx react-native init command initializes a n ew project with a default template. This command installs all the necessary dependencies in a new directory with the given project name (in this case, "MyNewProject"). After initializatio n, you can navigate into the project directory and start the development server by running n
px react-native start. To view your new app, you need to use the iOS Simulator, Android Emul ator, or a physical device.
[Trivia]
React Native was introduced by Facebook in 2015. It has grown in popularity due to its abilit y to leverage existing JavaScript and React skills for mobile app development, reducing the n eed for separate native developers for iOS and Android platforms.
Optimizing React for SEO
For better search engine optimization (SEO), server-side rendering (SSR) or static site generat ion (SSG) with React can be crucial.
Below is an example of using Next.js, a React framework, for static site generation which help s in SEO.
[Code]
npx create-next-app my-static-site
cd my-static-site
npm run build
npm start
[Result]
These commands create a new Next.js project, build the static site, and start the server hostin g the static pages.
Server-side rendering (SSR) and static site generation (SSG) are techniques that can improve the SEO of React applications by rendering components into HTML on the server instead of c lient-side. This makes the content crawlable by search engines that may not execute JavaScri
pt well.Next.js is a React framework that supports both SSR and SSG out of the box. The npx create-next-app command sets up a new Next.js project. Running npm run build with a Next.
js project generates a production build of the app, optimizing performance and rendering st atic HTML files for each page. The npm start command launches a Node.js server that serves these static pages. These pages are immediately viewable by search engines, which enhances the SEO potential of your site.
[Trivia]
Google and other search engines have improved in executing JavaScript but still recommend server-side rendering for the best SEO results. Using Next.js for React applications not only o ptimizes SEO but also improves load times and overall user experience by delivering pre-ren dered content to users.
CSS-in-JS in React
CSS-in-JS libraries, such as styled-components and emotion, provide a way to write CSS that is tied directly to React components.
Below is an example using styled-components to create a button with scoped styles.
[Code]
import styled from 'styled-components';
const Button = styled.button`
background-color: blue;
color: white;
padding: 10px 20px;
border: none;
border-radius: 5px;
cursor: pointer;
&:hover {
background-color: darkblue;
}
`;
function App() {
return <Button>Click me</Button>;
}
export default App;
[Result]
A button with the text "Click me" appears, styled with a blue background, white text, and cha nges to dark blue on hover.
In this example, styled-components is used to create a Button component with specific stylin g. This method encapsulates the styles within the component, preventing any style leakage o r conflicts with other parts of the application. The &:hover selector is used to change the butt on's background color when the mouse hovers over it. This approach enhances the maintain ability and reusability of components, as each component controls its own styling, independe nt from global stylesheets.
[Trivia]
CSS-in-JS libraries not only help in scoping CSS to components but also support dynamic styl ing based on props, theming, and can significantly reduce the risk of naming conflicts by aut omatically generating unique class names for the styles.
Immutability in React State
Maintaining immutability in state management in React is essential for ensuring predictable updates and performance.
The following example shows how to handle immutability in React state using the useState h ook.
[Code]
import React, { useState } from 'react';
function ListComponent() {
const [items, setItems] = useState(['Item 1', 'Item 2']); function addItem() {
// Correct immutable update
setItems(prevItems => [...prevItems, Ìtem ${prevItems.length + 1}`]);
}
return (
<div>
<ul>
{items.map(item => <li key={item}>{item}</li>)}
</ul>
<button onClick={addItem}>Add Item</button>
</div>
);
export default ListComponent;
[Result]
The application displays a list of items with a button. Clicking the button adds a new item to the list, updating the state immutably.
In this example, the useState hook is used to manage the list of items. When adding a new it em, it's crucial not to modify the original state directly to prevent unexpected behaviors and optimize re-rendering. By using the spread operator (...), a new array is created with all previo us items and the new item, ensuring the original state array remains unchanged. This metho d is a practical application of immutability, which helps in maintaining predictable state upda tes and performance in React applications.
[Trivia]
Immutability is a key concept in functional programming that React is heavily influenced by. I t helps in easier debugging, efficient memory usage by avoiding unnecessary copies of data, and plays a significant role in optimizing React components by ensuring that only componen ts whose props or state have changed are re-rendered.
Function Components with Hooks
Function components in React allow you to build simpler and more reusable components usi ng hooks.
The following example shows a basic function component using the useState hook to manag e state.
[Code]
import React, { useState } from 'react';
function Counter() {
const [count, setCount] = useState(0);
return (
<div>
<p>You clicked {count} times</p>
<button onClick={() => setCount(count + 1)}>
Click me
</button>
</div>
);
}
export default Counter;
When the button is clicked, the displayed count increases by one each time.
In this code:We import useState from the React library, which is a Hook allowing us to keep l ocal state in a function component.useState(0) initializes the count state variable to 0. The us eState returns a pair: the current state value and a function that lets you update it.Inside the Counter function component, we display a paragraph showing the current count and a butto n that increments the count.The button's onClick handler updates the state with setCount(co unt + 1), causing the component to re-render with the new count.
This approach is more straightforward compared to using class components where you need to deal with this keyword and class methods.
[Trivia]
React's useState hook initializes the state only on the first render, making subsequent render s use the same state value until it's updated. This avoids the complexity of managing lifecycle methods like componentDidMount or componentDidUpdate that are common in class comp onents.
Type Safety in React
Using propTypes or TypeScript helps ensure type safety and enhances the reliability of your c omponents.
Here is an example of how to use propTypes in a functional React component to validate pro ps.
[Code]
import React from 'react';
import PropTypes from 'prop-types';
function Greeting({ name }) {
return <h1>Hello, {name}!</h1>;
}
Greeting.propTypes = {
name: PropTypes.string.isRequired,
};
export default Greeting;
[Result]
The component displays a greeting message incorporating the name passed to it.
In this code:The Greeting component accepts a single prop name.PropTypes.string.isRequire d ensures that name must be provided and it must be a string. If name is not provided or is n ot a string, React will console a warning during development, helping prevent bugs related t o incorrect prop types.This approach enhances the predictability of your components and en sures that the values passed as props are of the expected type, reducing runtime errors.
[Trivia]
Using propTypes is a simple way to enforce type safety in React without adopting a full static type system like TypeScript. It's a minimal, runtime type-checking system, primarily useful in development mode to catch common type-related errors.
Handling Forms in React
Learn how to manage forms efficiently in React using libraries like Formik or React Hook For m.
Here's a basic example of using React Hook Form to create a simple registration form:
[Code]
import React from 'react';
import { useForm } from 'react-hook-form';
function RegistrationForm() {
const { register, handleSubmit, errors } = useForm();
const onSubmit = data => console.log(data);
return (
<form onSubmit={handleSubmit(onSubmit)}>
<input name="username" ref={register({ required: true })} placeholder="Username" /
>
{errors.username && <p>Username is required.</p>}
<input name="email" ref={register({ required: true })} placeholder="Email" />
{errors.email && <p>Email is required.</p>}
<input type="submit" />
</form>
);
}
export default RegistrationForm;
[Result]
Upon submission, if the fields are filled, the form data will be logged to the console. If fields are missing, error messages will appear under the respective inputs.
React Hook Form is a popular library for managing forms in React. It provides methods for re gistering input controls and handling form submission, reducing the need to manually mana ge form state and perform validation. This library optimizes performance by minimizing the n umber of re-renders, and its API is simple, making it accessible even to beginners. By using re f={register}, you link the form input to the validation rules specified, which in this case, is just checking that the input is filled ({ required: true }).
[Trivia]
React Hook Form is lighter than many other form management libraries and supports TypeSc ript, making it a good choice for projects that value performance and type safety.
Enhancing Accessibility in Interactive UIs
Improve the accessibility of your interactive UIs in React by managing focus correctly.
Below is an example of how to manage focus in a React component to enhance accessibility:
[Code]
import React, { useRef, useEffect } from 'react';
function AccessibleInput() {
const inputRef = useRef(null);
useEffect(() => {
// Automatically focus the input when the component mounts inputRef.current.focus();
}, []);
return (
<input ref={inputRef} aria-label="Search" placeholder="Type here..." />
);
}
export default AccessibleInput;
[Result]
When the component loads, the input field automatically receives focus.
This example uses the useRef and useEffect hooks in React to manage focus. The useRef hoo k creates a reference to the input element, which allows you to directly manipulate the DOM
node. Using useEffect, you can specify side effects that run after the component renders; her e, it's used to set focus to the input when the component mounts. Managing focus is crucial f or accessibility, especially for users relying on keyboard navigation and screen readers. Settin g the aria-label attribute helps screen reader users understand the purpose of the input field.
[Trivia]
Proper focus management not only helps with usability for visually impaired users but also e nhances the overall user experience by making web applications easier and more intuitive to navigate.
Optimizing Render Times in React
Learn how to monitor and optimize render times to enhance the performance of React applic ations.
The following example demonstrates how to use the React Developer Tools to monitor comp onent render times.
[Code]
// This code snippet shows a simple React component.
// It assumes that you have the React Developer Tools extension installed in your browser.
import React, { useState } from 'react';
function Counter() {
const [count, setCount] = useState(0);
const increment = () => {
setCount(count + 1);
};
console.log('Component rendered');
return (
<div>
<h1>{count}</h1>
<button onClick={increment}>Increment</button>
</div>
);
export default Counter;
[Result]
In the React Developer Tools, you would see the render timing for each update when the but ton is clicked.
In the provided code, we define a simple counter component that increments a state variable
. Every time the button is clicked, the state changes and triggers a re-render of the compone nt.Here's how to optimize further:Use React.memo to prevent unnecessary re-renders if the p rops do not change.Use the useCallback hook to memoize callback functions to prevent unn ecessary re-rendering of child components.Profile the component with React Developer Tool s to visually inspect render times and identify bottlenecks.To use React Developer Tools:Instal l the extension from your browser's web store.Open your React application, and open the de veloper tools (usually with F12).Navigate to the React tab, where you can see the component tree and profiling information.
[Trivia]
Monitoring performance in React applications is crucial because even minor inefficiencies ca n lead to significant performance issues in large-scale applications. React Developer Tools is an essential extension for any React developer, providing insights into component renders a nd allowing performance optimization.
Preventing Memory Leaks in React
Understand how to avoid memory leaks by properly cleaning up in useEffect in React applica tions.
Here is an example demonstrating how to use the cleanup function in useEffect to prevent m emory leaks.
[Code]
import React, { useState, useEffect } from 'react';
function TimerComponent() {
const [time, setTime] = useState(0);
useEffect(() => {
const timer = setInterval(() => {
setTime(prevTime => prevTime + 1);
}, 1000);
return () => {
clearInterval(timer);
console.log('Cleanup done');
};
}, []);
return <div>Timer: {time} seconds</div>;
}
export default TimerComponent;
[Result]
Every second, the timer updates the display, and when the component unmounts, "Cleanup done" is logged to the console.
In this example, the TimerComponent creates an interval timer that increments a state variabl e every second. The useEffect hook is used to set up the interval when the component moun ts and importantly, it returns a cleanup function that clears the interval when the component unmounts.It's crucial to use the cleanup function in useEffect to remove any subscriptions, ev ent listeners, or other side effects that may lead to memory leaks. Not cleaning up properly c an lead to several problems:Excessive use of memory that isn't freed.Unexpected behavior fr om old instances affecting your app's UI.Performance degradation over time as unused reso urces continue to consume system resources.To further ensure robustness:Always include de pendencies in the useEffect dependency array to control when effects run and cleanup happ ens.Consider using linters like ESLint which can help identify missing dependencies in effect hooks.
[Trivia]
Understanding and using the useEffect cleanup function is critical in React development to m anage resources efficiently and prevent memory leaks, which are common issues in single-pa ge applications (SPAs) where components frequently mount and unmount.
Understanding Redux Toolkit
Redux Toolkit simplifies the management of state in React applications by providing tools th at automate common tasks and enforce best practices.
Below is an example of how to set up a Redux store using Redux Toolkit.
[Code]
import { configureStore } from '@reduxjs/toolkit';
const store = configureStore({
reducer: {
// Reducers go here
}
});
[Result]
No direct output; this code sets up the Redux store in memory.
Redux Toolkit streamlines the configuration of your Redux store. Here's a breakdown of how it simplifies development:configureStore: This function automatically sets up the Redux DevT
ools Extension and redux-thunk middleware. This means you do not need to manually apply
these commonly used middlewares.Reducers: In the 'reducer' field of configureStore, you def ine your app’s reducers. Redux Toolkit allows you to combine reducers conveniently, managi ng different slices of state.Middleware Integration: As mentioned, Redux Toolkit automaticall y applies several middlewares that are essential for asynchronous operations and debugging.
DevTools: Redux Toolkit enables Redux DevTools by default, providing powerful tools for tra cking state changes and actions without additional setup.This setup is essential for managing state more effectively in large React applications and avoids the boilerplate code that traditio nally accompanies Redux setups.
[Trivia]
Redux Toolkit was created to address the complexity of configuring and using Redux, aiming to make Redux easier for beginners and to provide best practices out of the box. It was offici ally recommended by the Redux team as the standard way to write Redux logic since its intro duction.
Utilizing forwardRef in React
The forwardRef API in React allows you to pass refs down to child components, which is usef ul for managing focus, triggering imperative animations, and integrating with third-party DO
M libraries.
Below is an example demonstrating how to use forwardRef to pass a ref to a child componen t in React.
[Code]
import React, { forwardRef } from 'react';
const CustomInput = forwardRef((props, ref) => (
<input ref={ref} {...props} />
));
function App() {
const inputRef = React.createRef();
return <CustomInput ref={inputRef} />;
}
[Result]
No direct output; this code sets up a ref that can be used to interact with the input element i n the DOM.
Understanding forwardRef is crucial for advanced React patterns. Here’s a detailed explanatio n:forwardRef: This React API allows you to forward a ref through a component to one of its c hild components. This is particularly useful in higher-order components (HOCs) and compon ents utilizing render props, where the ref would otherwise be lost.Usage in HOCs: When creat ing a HOC, forwardRef can be used to pass refs through the HOC to the wrapped component
, ensuring that the end component can still be referenced directly.Interacting with DOM: By u sing refs, components gain direct access to DOM nodes. This is essential for managing focus, media playback, or triggering animations directly.Integration with Third-Party Libraries: Many third-party libraries interact directly with the DOM and require a ref to the DOM node to wor k properly. forwardRef makes this integration smoother.This technique enhances component reusability and encapsulation by allowing deeper control over the components.
[Trivia]
forwardRef was introduced in React version 16.3 as part of an effort to provide more flexible APIs for higher-order components, especially in libraries like Redux and MobX, which often r equire refs to be passed through to manage focus and local state more directly.
Understanding useContext in React
The useContext hook simplifies accessing context in functional components.
Here's how to use the useContext hook in a functional component to access a value from a R
eact context.
[Code]
import React, { useContext, createContext } from 'react'; const MyContext = createContext('default value');
function MyComponent() {
const value = useContext(MyContext);
return <div>{value}</div>;
}
function App() {
return (
<MyContext.Provider value="Hello, useContext!">
<MyComponent />
</MyContext.Provider>
);
}
export default App;
The rendered result will display "Hello, useContext!" wrapped in a <div>.
In this example:We create a context using createContext and set a default value.MyCompone nt accesses the context value using useContext(MyContext). This method is far more straightf orward than using Context.Consumer, which requires a render prop pattern and makes the c ode more verbose.The App component wraps MyComponent in MyContext.Provider, allowin g us to override the context's default value with "Hello, useContext!".useContext allows comp onents that consume the context to re-render only when the context value changes, optimizi ng performance without extra coding.The useContext hook eliminates the need to wrap com ponents in Context.Consumer, simplifying the component tree and making the code cleaner and more readable.
[Trivia]
Understanding context is crucial in large-scale React applications as it provides a way to pass down data through the component tree without having to pass props manually at every level
. This pattern is particularly useful for themes, user settings, authentication statuses, and mor e.
Optimizing React Components
Utilize React’s optimization techniques to reduce unnecessary re-renders.
This example demonstrates the use of React.memo to prevent unnecessary re-renders in a fu nctional component.
[Code]
import React, { useState } from 'react';
const ExpensiveComponent = React.memo(function ExpensiveComponent({ value }) {
console.log('Rendering: ', value);
return <div>{value}</div>;
});
function App() {
const [count, setCount] = useState(0);
return (
<>
<button onClick={() => setCount(count + 1)}>Increment</button>
<ExpensiveComponent value="Static Value" />
</>
);
}
export default App;
[Result]
When clicking the "Increment" button, "Rendering: Static Value" will only log once in the con sole, indicating the component does not re-render unnecessarily.
In the provided code:ExpensiveComponent is wrapped with React.memo, which is a higher-o rder component. It tells React to only re-render if the props have changed, based on shallow comparison.Inside App, the ExpensiveComponent receives a static prop "Static Value". Theref ore, subsequent re-renders of App (triggered by updating the state with setCount) do not ca use ExpensiveComponent to re-render.This demonstrates an effective use of React.memo to avoid costly re-renders in components where the input props do not change frequently.Usin g React.memo is particularly beneficial in performance-critical applications and large compo nent trees, where unnecessary re-renders can lead to significant performance issues.
[Trivia]
shouldComponentUpdate and PureComponent are other React techniques for class compon ents similar to React.memo for functional components. These tools help in managing compo nent updates and rendering by comparing previous and new props or state.
Using useLayoutEffect in React
Learn how to synchronize changes with the DOM using the useLayoutEffect hook.
The example below demonstrates how to use the useLayoutEffect hook to update a DOM ele ment immediately after layout calculations are done, ensuring that the visual update does no t cause a noticeable flicker to the user.
[Code]
import React, { useLayoutEffect, useRef } from 'react'; function FocusedInput() {
const inputRef = useRef(null);
useLayoutEffect(() => {
// Directly manipulating the DOM to focus the input element inputRef.current.focus();
}, []); // Empty dependency array means this runs once after the initial render return <input ref={inputRef} placeholder="Focus me on load" />;
}
[Result]
The input field is focused automatically when the component mounts.
The useLayoutEffect hook works similarly to useEffect, but it fires synchronously after all DO
M mutations. This is crucial for operations that need to be visually in sync with the DOM to p revent issues like flickering or visible layout shifts. When you change a DOM element's prope rties that affect its appearance (size, focus state, etc.), useLayoutEffect ensures these changes occur between the time the DOM is updated and the screen is updated. This can be vital for animations, focus management, or adjusting elements in response to DOM changes without the user noticing any delay or layout shift.
[Trivia]
useLayoutEffect is best used when you need precise control over the timing of DOM updates
, similar to how you would use componentDidMount and componentDidUpdate in class com ponents. It’s recommended to default to useEffect unless you specifically need to make chan ges that must be visually synced with the DOM.
Modular Architecture in React Projects
Understand the importance of maintaining a modular architecture in React projects.
The following example shows a simple way to structure a React project using modular comp onents to improve maintainability and scalability.
[Code]
// File: WelcomeMessage.js
import React from 'react';
function WelcomeMessage({ name }) {
return <h1>Welcome, {name}!</h1>;
}
// File: App.js
import React from 'react';
import WelcomeMessage from './WelcomeMessage';
function App() {
return (
<div>
<WelcomeMessage name="Alice" />
</div>
);
}
[Result]
Displays a welcome message: "Welcome, Alice!"
In this example, we have divided the React application into smaller, reusable components. W
elcomeMessage is a functional component that accepts props and returns a greeting, makin g it reusable in various parts of the application or even in other projects. This approach enha nces the maintainability of your code by isolating each component's responsibilities, making it easier to debug and test individual parts. Additionally, as your application grows, a modula r architecture allows you to scale more efficiently by reusing components and ensuring that a dditions or changes to one module do not cause unexpected issues in others.
[Trivia]
A modular architecture not only helps in making the application easier to manage but also pr omotes reusability, which is a core principle of effective software development. By creating c omponents that accomplish specific tasks and can be used interchangeably throughout the a pplication, you optimize the development process and improve code quality.
Error Handling in React
Always manage errors and handle exceptional cases in React components to provide a stable and reliable user experience.
Here's a simple example of error handling in a React component using error boundaries.
[Code]
import React, { Component } from 'react';
class ErrorBoundary extends Component {
constructor(props) {
super(props);
this.state = { hasError: false };
}
static getDerivedStateFromError(error) {
// Update state so the next render will show the fallback UI.
return { hasError: true };
}
componentDidCatch(error, errorInfo) {
// You can also log the error to an error reporting service console.log(error, errorInfo);
}
render() {
if (this.state.hasError) {
// You can render any custom fallback UI return <h1>Something went wrong.</h1>;
}
return this.props.children;
}
}
// Usage of ErrorBoundary in the App component
function App() {
return (
<ErrorBoundary>
<MyComponent />
</ErrorBoundary>
);
}
[Result]
If an error occurs in MyComponent, the ErrorBoundary will catch it and display "Something w ent wrong."
Error boundaries in React are components that catch JavaScript errors anywhere in their child component tree, log those errors, and display a fallback UI instead of the component tree th at crashed. They catch errors during rendering, in lifecycle methods, and in constructors of th e whole tree below them. However, they do not catch errors for:Event handlersAsynchronous code (e.g., setTimeout or requestAnimationFrame callbacks)Server-side renderingErrors thro wn in the error boundary itself (rather than its children)
[Trivia]
Implementing an error boundary component like ErrorBoundary in React applications ensure s that even if a part of the UI crashes, the rest of the application remains operational. It's a cr ucial practice for enhancing the reliability of the application and providing a better user expe rience under unforeseen circumstances.
React Internationalization
Internationalization in React makes your application accessible to a global audience using lib raries like react-intl or i18next.
Here's an example of integrating internationalization in a React application using the react-in tl library.
[Code]
import React from 'react';
import { IntlProvider, FormattedMessage } from 'react-intl'; const messages = {
en: {
title: 'Hello World',
},
fr: {
title: 'Bonjour le monde',
}
};
function App() {
const locale = 'fr'; // Assume French is selected
return (
<IntlProvider locale={locale} messages={messages[locale]}>
<h1>
<FormattedMessage id="title" defaultMessage="Default message" />
</h1>
</IntlProvider>
);
}
[Result]
The text "Bonjour le monde" will be displayed in the application if the selected language is Fr ench.
The react-intl library provides React components and an API to format dates, numbers, and s trings, including pluralization and handling translations. IntlProvider is a component that wra ps your application's root component and provides the internationalization capabilities. The FormattedMessage component is used to display text messages, which are automatically tra nslated based on the locale provided to IntlProvider. To support multiple languages:You mus t define messages for each locale.Switch the locale dynamically based on user preference or browser settings.
[Trivia]
Internationalization not only helps in making your application accessible to a wider audience but also improves its usability and adaptability in different regions, enhancing the overall use r experience and engagement. Utilizing libraries like react-intl can greatly simplify the proces s of adding multiple languages to your React applications.
Continuous Integration and Deployment in React
Continuous integration (CI) and continuous deployment (CD) are practices designed to help developers integrate their code into a shared repository frequently and to release application s automatically and reliably.
Below is an example of a basic CI/CD pipeline using GitHub Actions for a React application.
[Code]
name: React CI/CD Pipeline
on:
push:
branches: [ main ]
pull_request:
branches: [ main ]
jobs:
build:
runs-on: ubuntu-latest
steps:
- uses: actions/checkout@v2
- name: Set up Node.js
uses: actions/setup-node@v2
with:
node-version: '14'
run: npm install
- name: Run tests
run: npm test
- name: Build
run: npm run build
deploy:
needs: build
runs-on: ubuntu-latest
if: github.ref == 'refs/heads/main'
steps:
- uses: actions/checkout@v2
- name: Set up Node.js
uses: actions/setup-node@v2
- name: Install dependencies
run: npm install
- name: Build
run: npm run build
- name: Deploy to Production
run: scp -r build/* username@yourserver.com:/path/to/website env:
DEPLOY_KEY: ${{ secrets.DEPLOY_KEY }}
[Result]
No direct output as this is a configuration file.
This YAML file defines a workflow in GitHub Actions. When code is pushed to the 'main' bran ch or when a pull request is made to it, this triggers the workflow. The jobs are divided into '
build' and 'deploy'.Build Job: This job installs Node.js and the project's dependencies, runs te
sts, and builds the production-ready code. It uses the official GitHub Actions to set up Node.j s and to check out the code from the repository.Deploy Job: This job depends on the success ful completion of the build job. It repeats some steps like setting up Node.js and installing de pendencies because each job runs in a fresh environment. Finally, it deploys the built applicat ion to a production server using scp (secure copy protocol). Note that it uses a secret key DE
PLOY_KEY to authenticate to the server, which needs to be set up in the repository's secrets.T
his pipeline ensures that every change made to the application is automatically tested and d eployed if the tests pass. It reduces the chance for human error and streamlines the develop ment process.
[Trivia]
CI/CD not only automates the process but also provides immediate feedback to developers o n their code changes, which enhances code quality and reduces the time to detect and corre ct issues.
Managing Environments in React
Environment variables allow you to manage different configurations for your React applicatio n across various environments like development, testing, and production without hard-codin g any sensitive data.
Below is an example of using environment variables in a React application.
[Code]
console.log('Environment:', process.env.REACT_APP_STAGE);
[Result]
"Environment: development" (Assuming the environment variable REACT_APP_STAGE is set t o 'development' in the local environment)
This example simply logs the value of the environment variable REACT_APP_STAGE. In React, environment variables need to be prefixed with REACT_APP_ to ensure they are included in t he build when using Create React App.How to Set Environment Variables:In your local develo pment environment, you can create a .env file in the root of your project.Add variables like th is: REACT_APP_STAGE=development.In production, these variables can be set in the environ ment where the server or hosting platform runs your application.Using environment variables
is crucial for security and flexibility. It prevents sensitive data from being pushed to version c ontrol and allows different behaviors across environments without changing the code.
[Trivia]
Using environment variables is a best practice in modern application development. It not onl y aids in maintaining security by keeping sensitive data out of your source code but also pro vides the flexibility to change settings without code changes across multiple stages of develo pment.
Using GraphQL with React
Learn how to fetch data efficiently in React using GraphQL.
Here's a basic example of using GraphQL with React to fetch user data:
[Code]
import React from 'react';
import { useQuery, gql } from '@apollo/client';
const GET_USER = gql`
query GetUser($userId: ID!) {
user(id: $userId) {
id
name
}
}
`;
function UserComponent({ userId }) {
const { loading, error, data } = useQuery(GET_USER, {
variables: { userId },
});
if (loading) return <p>Loading...</p>;
if (error) return <p>Error :(</p>;
<div>
<h1>{data.user.name}</h1>
<p>Email: {data.user.email}</p>
</div>
);
}
export default UserComponent;
[Result]
Displays the user's name and email if successful, or shows loading/error messages.
In this example, useQuery is a hook provided by Apollo Client to execute a GraphQL query. T
he query GET_USER is defined with a parameter $userId to fetch user-specific data. The comp onent UserComponent consumes the userId prop to make the query. When the data is being fetched, a loading message is shown. If there's an error in fetching data, an error message is displayed. Once the data is successfully fetched, it renders the user's name and email.The use of GraphQL eliminates unnecessary data fetches (over-fetching) and missing data scenarios (
under-fetching) often seen in REST APIs, as it allows clients to request exactly what they need
.
[Trivia]
GraphQL, developed by Facebook, is a query language for APIs that provides a more efficient and powerful alternative to REST. It allows clients to specify the exact data needed, reducing the amount of data transferred over the network.
Using Component Libraries in React
Speed up your React development with pre-built component libraries.
Here’s an example of using the Material-UI library to create a simple user interface in React:
[Code]
import React from 'react';
import { Button, TextField, Typography } from '@material-ui/core'; function LoginForm() {
return (
<div style={{ margin: '50px' }}>
<Typography variant="h4">Login</Typography>
<TextField label="Username" variant="outlined" />
<TextField label="Password" type="password" variant="outlined" style={{ marginTop: '20
px' }} />
<Button variant="contained" color="primary" style={{ marginTop: '20px' }}> Submit
</Button>
</div>
);
}
export default LoginForm;
[Result]
Renders a login form with username and password fields and a submit button.
Material-UI is a popular React UI framework that offers a wide range of pre-designed compo nents, such as Buttons, TextFields, and Typography elements. In the example above, LoginFor m uses these components to build a styled form quickly without the need to write detailed C
SS or structure. Each component comes with props like variant and color, providing customiz ation options that help align the design closer to your application's theme.Using such librarie s accelerates the development process, especially for prototyping, by providing high-quality, customizable UI components out of the box.
[Trivia]
Component libraries like Material-UI follow Material Design principles established by Google, ensuring that applications not only look good but also have consistent, usability-optimized d esigns.
Clear Project Structure in React
Maintaining a clear and consistent project structure is vital for managing and navigating a Re act codebase efficiently.
Let's explore a basic folder structure for a React application:
[Code]
src/
|-- components/
| |-- Header.js
| |-- Footer.js
|-- containers/
| |-- UserProfile.js
|-- pages/
| |-- Home.js
| |-- About.js
|-- App.js
|-- index.js
[Result]
This structure organizes files into specific directories for components, containers, and pages.
components/: This directory holds all the reusable UI components like buttons, headers, foot ers, etc. Each component has its own file, making it easier to find and maintain them.contain ers/: Containers are components that connect directly with the Redux store (if used) or mana ge significant parts of state. They are typically parent components that handle logic and state management and pass data down to child components.pages/: Represents the distinct pages of the app, each corresponding to a route in the application. For instance, 'Home.js' might be your landing page, and 'About.js' might provide company information.App.js: Serves as the r oot component that contains routing logic and global configurations.index.js: The entry poin t of the React application that renders the App component into the HTML.This separation of components, containers, and pages helps in maintaining a modular and scalable codebase, f acilitating easier updates and maintenance.
[Trivia]
The distinction between components and containers is part of a design pattern called "smart and dumb components" or "container and presentational components." This pattern encoura ges separating the UI (presentational components) from the business logic (containers).
Custom Middleware in Redux
Implementing custom middleware in Redux can enhance functionality, such as handling asyn chronous actions or logging for debugging.
Here’s how to create a simple logging middleware in Redux:
[Code]
const loggerMiddleware = store => next => action => {
console.log('dispatching', action);
let result = next(action);
console.log('next state', store.getState());
return result;
};
export default loggerMiddleware;
[Result]
This middleware logs every action dispatched, along with the state after the action is process ed.
store: The Redux store object that holds the application state.next: A function that calls the n ext middleware in the chain. If this is the last middleware, it will call the actual reducer.action: The action object being dispatched. Middleware can read or modify actions.The process flow in the middleware:The loggerMiddleware captures each dispatched action.It logs the action d etails before passing the action to the next middleware or reducer.After the next function, it l ogs the new state of the application.The result of the next function (usually the action) is retu rned.This middleware is particularly useful for debugging, as it allows developers to see the s equence of actions and their impact on the app's state in real-time.
[Trivia]
Redux middleware is powerful because it provides a third-party extension point between dis patching an action and the moment it reaches the reducer. This is ideal for logging, crash rep orting, performing asynchronous tasks, etc.
Using Service Workers in React
Implement service workers in React to enhance web applications with performance improve ments and offline capabilities.
The following example demonstrates how to register a service worker in a React application.
[Code]
// In your React application, you typically handle the registration of service workers in your in dex.js file.
if ('serviceWorker' in navigator) {
window.addEventListener('load', () => {
navigator.serviceWorker.register('/service-worker.js').then(registration => {
console.log('ServiceWorker registration successful with scope: ', registration.scope);
}, err => {
console.log('ServiceWorker registration failed: ', err);
});
});
}
[Result]
ServiceWorker registration successful with scope: [scope of the service worker]
Service workers are scripts that your browser runs in the background, separate from a web p age, enabling features that don't need a web page or user interaction. They are a powerful fe ature for creating reliable and fast web applications, particularly useful for handling offline ca pabilities and background syncs.In React, service workers can be integrated to cache assets a nd API responses, which is particularly useful in creating progressive web applications (PWAs)
. The registration of a service worker starts by checking if the browser supports it, followed b y registering it during the window's 'load' event to ensure that the registration process does not affect the initial page load performance. This code snippet will output a console message indicating successful registration or an error if it fails.It's important to handle the registration process correctly to ensure that updates to your service worker are processed correctly, allow ing new versions of your service worker to take over seamlessly.
[Trivia]
Service workers primarily cache network requests to allow web applications to access resourc es offline, but they also enable other advanced features such as push notifications and backg round data synchronization. Using service workers can significantly enhance the user experie nce by improving load times and providing content availability offline.
React Keys in Lists
Use unique and stable keys for list items in React to maintain state consistency and performa nce.
Below is an example of using unique ids as keys in a React component that renders a list.
[Code]
import React from 'react';
function ItemList({ items }) {
return (
<ul>
{items.map(item => (
<li key={item.id}>{item.text}</li>
))}
</ul>
);
}
// Example usage:
const todoItems = [
{ id: 1, text: 'Learn React' },
{ id: 2, text: 'Read about Service Workers' }
];
function App() {
return <ItemList items={todoItems} />;
}
export default App;
[Result]
<ul>
<li key="1">Learn React</li>
<li key="2">Read about Service Workers</li>
</ul>
When rendering lists in React, it's crucial to use keys that uniquely identify each element in th e list. Thèkey` prop helps React identify which items have changed, been added, or removed
, which greatly aids in efficient updates of the user interface.Using indices as keys may lead t o issues if the order of items changes, as React may incorrectly assume that the item with the same key is the same element. Instead, use a unique and stable identifier like an id from your data. This approach ensures that the list and its state are maintained correctly across re-rend ers and updates, leading to better performance and fewer bugs in your application.
[Trivia]
Incorrect usage of keys in React lists can cause unexpected behavior such as improper render ing, lost state, and performance issues. This is because keys should give React the hint to uni quely identify elements independently from their order in the dataset. This practice not only helps in performance optimizations but also prevents issues during updates when the items’
order changes.
Optimizing Image Handling in React
Learn how to handle images in React to improve loading times and enhance the user experie nce.
The following example demonstrates how to use the react-lazy-load-image-component for l azy loading images in a React application.
[Code]
import React from 'react';
import { LazyLoadImage } from 'react-lazy-load-image-component'; import 'react-lazy-load-image-component/src/effects/blur.css'; const ImageComponent = ({ imageUrl }) => (
<div>
<LazyLoadImage
src={imageUrl} // URL of the image
effect="blur" // Apply a blur effect while the image is loading alt="An example image"
width="100%" // Responsive image width
height="auto" // Maintain aspect ratio
/>
</div>
);
export default ImageComponent;
[Result]
The image is loaded lazily as it comes into the viewport, initially displayed with a blur effect.
In this example, the LazyLoadImage component from the react-lazy-load-image-component library is used to lazy load an image. This means that the image is only loaded when it beco mes visible in the user's viewport, which significantly reduces initial load times and saves ban dwidth. The effect prop is set to "blur", providing a pleasant aesthetic effect that transitions s moothly to the full image once loaded. This approach is particularly useful in React applicatio ns where large numbers of images or media files might be displayed, such as in image galleri es or product listings.The width is set to 100% to ensure the image is responsive, adjusting to the width of its container, and the height is set to auto to maintain the image's aspect ratio r egardless of the display size. This setup contributes to a better user experience by adapting t o various device screens and orientations.
[Trivia]
Lazy loading is a technique that delays the loading of resources until they are actually neede d, which can improve the performance of a webpage by reducing initial load time and page weight. This is particularly effective in environments where bandwidth is a bottleneck.
Adopting Mobile-First Design in React
Understand the importance of mobile-first design in React projects to better serve mobile us ers.
Here we illustrate how to use CSS modules in React to implement a responsive design, focusi ng on mobile-first strategies.
[Code]
import React from 'react';
import styles from './App.module.css';
const App = () => (
<div className={styles.container}>
<h1 className={styles.header}>Welcome to Our Mobile-First React App!</h1>
<p className={styles.paragraph}>
This text is optimized for mobile viewing first, scaling up smoothly for larger screens.
</p>
</div>
);
export default App;
[Result]
The webpage displays text that is optimized for mobile devices, with styles that adapt as the screen size increases.
In this code snippet, CSS modules are utilized to apply styles from a local CSS file (App.modu le.css) to a React component. The mobile-first approach is evident in the way the CSS is struc tured: styles are defined with the assumption that the user is on a mobile device, with additio nal styles (media queries) added to adjust the layout for larger screens as needed. This meth odology ensures that the application is optimized for mobile users from the start, which is cr ucial given the increasing prevalence of mobile internet usage.The classes container, header, and paragraph in the styles object are imported from the CSS module, ensuring that these st yles are scoped locally to the component, avoiding conflicts with other styles in the applicati on. This approach enhances maintainability and modularity in large React applications.
[Trivia]
A mobile-first design strategy starts with designing an online experience for mobile devices fi rst before scaling up designs for larger screens. This approach is beneficial not only for the us er experience but also for SEO, as Google prioritizes mobile-friendly websites in its search ra nkings.
React Router Basics
Learn how to keep your routes organized and use dynamic routing in React.
Below is an example of how to use React Router to organize routes and implement dynamic routing.
[Code]
import React from 'react';
import { BrowserRouter as Router, Route, Switch } from 'react-router-dom'; const Home = () => <div>Home Page</div>; const About = () => <div>About Us</div>; const User = ({ match }) => <div>User ID: {match.params.id}</div>; function App() {
return (
<Router>
<Switch>
<Route path="/" exact component={Home} />
<Route path="/about" component={About} />
<Route path="/user/:id" component={User} />
</Switch>
</Router>
);
}
[Result]
No direct output, as this is a React component setup.
In this example, we use BrowserRouter (aliased as Router) to wrap our routing components. S
witch is used to render only the first Route that matches the current location's pathname.Rou te Paths: Defined using the Route component. Each Route has a path prop specifying the UR
L pattern and a component prop defining what component to render when the path matches
. For example, the / path renders the Home component.Dynamic Routing: The route /user/:id demonstrates dynamic routing. Here, :id is a placeholder for any user ID passed in the URL. T
he User component receives the matched parameters as a prop (match), which it uses to disp lay the user ID.This setup ensures that the application only loads the component necessary f or the visible route, optimizing performance and resource utilization.
[Trivia]
Dynamic routing in React Router makes it possible to build highly efficient and scalable appli cations that handle varying content needs based on URL parameters. By structuring your rou tes with dynamic segments, you can greatly reduce the redundancy of components and enha nce user navigation experiences.
React State Updates
Understand asynchronous state updates in React and using a function with setState.
The following example demonstrates how to update component state in React, accounting f or its asynchronous nature.
[Code]
import React, { Component } from 'react';
class Counter extends Component {
state = { count: 0 };
increment = () => {
this.setState(prevState => ({
count: prevState.count + 1
}));
};
render() {
return (
<div>
<p>Count: {this.state.count}</p>
<button onClick={this.increment}>Increment</button>
</div>
);
}
export default Counter;
[Result]
No direct output, as this is a React component setup.
This example introduces a Counter class component with a state that tracks a count value. Th e increment method updates the state based on the previous state, which is crucial because s tate updates in React are asynchronous.Asynchronous State Updates: React may batch multi ple setState() calls into a single update for performance reasons, which means this.state may not immediately reflect the new state after calling setState().Function in setState(): Passing a f unction to setState() (as shown with prevState => ({ count: prevState.count + 1 })) provides y ou with the previous state at the time the update is applied. This pattern ensures reliable stat e updates, particularly in cases where the new state depends on the old state.This approach prevents bugs that could arise from outdated state references and is a best practice in compl ex React applications.
[Trivia]
Understanding the asynchronous nature of setState() is crucial for managing complex states i n React. This knowledge is particularly important when state updates depend on previous val ues, as it ensures consistency across all state transitions and re-renders.
Debouncing and Throttling in React
Debouncing and throttling are techniques to optimize performance by controlling how often a function can execute.
Here is an example of implementing debouncing in a React component that handles input ch anges.
[Code]
import React, { useState, useEffect } from 'react';
// Debouncing function
function useDebounce(value, delay) {
const [debouncedValue, setDebouncedValue] = useState(value); useEffect(() => {
const handler = setTimeout(() => {
setDebouncedValue(value);
}, delay);
return () => {
clearTimeout(handler);
};
}, [value, delay]);
return debouncedValue;
}
function SearchInput() {
const [input, setInput] = useState(''); const debouncedInput = useDebounce(input, 500); // 500 ms delay useEffect(() => {
console.log(`Search for: ${debouncedInput}`);
}, [debouncedInput]);
return (
<input
type="text"
value={input}
onChange={(e) => setInput(e.target.value)}
placeholder="Type to search..."
/>
);
}
export default SearchInput;
[Result]
The console will log "Search for: [value]" only after the user has stopped typing for 500 millis econds.
Debouncing in React using a custom hook (useDebounce) helps in delaying the execution of function calls until a certain amount of inactivity (e.g., 500 ms) is detected. This is particularly useful in search inputs where you don't want to fire an API request with every keystroke, thus saving on performance and API call costs. The debounced value is updated only after the spe cified delay, and this update triggers the useEffect that logs the value, showing the effect of debouncing.
[Trivia]
Debouncing is different from throttling. Debouncing postpones the function call until after a period of inactivity, while throttling limits the number of times a function can be executed ov er time, ensuring it only happens at every specified interval.
Preventing XSS in React
React helps prevent XSS (Cross-Site Scripting) attacks, but additional security practices are ne cessary to further secure applications.
Below is an example demonstrating how to validate props to prevent unsafe content renderi ng in a React component.
[Code]
import React from 'react';
import PropTypes from 'prop-types';
const SafeContent = ({ content }) => {
// Simple content validation to prevent XSS
const isValidContent = (content) => {
const pattern = /<script\b[^<]*(?:(?!<\/script>)<[^<]*)*<\/script>/gi; return !pattern.test(content);
};
return (
<div>
{isValidContent(content) ? content : 'Unsafe content detected!'}
</div>
);
};
SafeContent.propTypes = {
content: PropTypes.string.isRequired,
};
export default SafeContent;
[Result]
If content includes a <script> tag, the component will render "Unsafe content detected!".
In the SafeContent component, a simple regular expression is used to detect <script> tags in the content passed as a prop. If such tags are found, indicating potential XSS risks, the comp onent renders a safe message instead of the untrusted content. This example highlights the i mportance of validating external inputs, especially when they might include HTML or JavaScri pt code that can execute. Always ensure that any content rendered in your components is sa nitized or validated if it originates from untrusted sources.
[Trivia]
XSS attacks can occur when an attacker manages to insert malicious scripts into web pages vi ewed by other users. These scripts can steal cookies, log keystrokes, and perform other harm ful actions. React automatically escapes content rendered in JSX before it's added to the DO
M, which prevents a wide range of XSS attacks by default. However, developers must still be vigilant about prop validation and avoiding direct HTML rendering with dangerouslySetInner HTML unless absolutely necessary and safe.
Animating in React
React can use both CSS transitions and JavaScript libraries to handle animations.
Here's a simple example of using CSS transitions in React to animate a div element.
[Code]
import React, { useState } from 'react';
function AnimatedDiv() {
const [toggle, setToggle] = useState(false);
const style = {
width: '100px',
height: '100px',
backgroundColor: 'blue',
transition: 'transform 0.3s ease-in-out',
transform: toggle ? 'scale(1.5)' : 'scale(1)'
};
return (
<div>
<button onClick={() => setToggle(!toggle)}>Animate</button>
<div style={style}></div>
</div>
);
}
[Result]
A blue square that scales up when you click the "Animate" button, and scales back down on a subsequent click.
This example demonstrates a basic use of CSS transitions in a React component. The useStat e hook is used to toggle the state of the component between true and false. This state dictat es whether the div should be scaled up (scale(1.5)) or remain at its original size (scale(1)). The CSS property transition specifies that the transformation should occur over 0.3 seconds with an "ease-in-out" timing function, providing a smooth animation effect. This method leverage s CSS for animations, which is generally more performant than JavaScript-based animations, especially for simple effects.
[Trivia]
Using CSS for animations offloads the graphical processing to the browser's rendering engin e, optimized for these tasks, rather than relying on JavaScript, which might be handling more logical or structural functions of your application.
Data Immutability in React
Understanding mutable vs immutable data is crucial for managing state in React.
Here’s a basic example demonstrating the importance of immutability in React state manage ment.
[Code]
import React, { useState } from 'react';
function DataList() {
const [items, setItems] = useState(['Item 1', 'Item 2']); const addItem = () => {
// Incorrect way: Directly mutating state
// items.push(Ìtem ${items.length + 1}`);
// setItems(items);
// Correct way: Using immutability
setItems([...items, Ìtem ${items.length + 1}`]);
};
return (
<div>
<button onClick={addItem}>Add Item</button>
{items.map((item, index) => <div key={index}>{item}</div>)}
</div>
);
export default DataList;
[Result]
Each click on "Add Item" will append a new item to the list displayed on the screen.
In the example, the addItem function demonstrates the incorrect and correct ways to update state in React. Directly mutating the state (commented out in the code) by pushing to the ite ms array won't trigger a re-render because React's state update mechanism won't detect cha nges made through direct mutation. In contrast, creating a new array with the spread operat or ([...items, newItem]) ensures that React recognizes the state change and triggers a re-rend er. This immutability pattern helps in predictable state management and efficient UI updates.
[Trivia]
Immutability is a key concept in functional programming and highly emphasized in React. It not only helps in predictable state management but also plays a crucial role in optimizing pe rformance through shouldComponentUpdate and React.memo, which rely on shallow compa rison to detect changes in data.
Using useEffect Dependency Arrays
Learn how to manage component side-effects in React using the useEffect hook.
Here is a simple example that demonstrates the use of the dependency array in a useEffect h ook to control the execution of side effects.
[Code]
import React, { useState, useEffect } from 'react';
function ExampleComponent() {
const [count, setCount] = useState(0);
// useEffect with dependency array
useEffect(() => {
// This code runs only when `count` changes.
console.log(`You clicked ${count} times`);
}, [count]); // Dependency array includes `count`
return (
<div>
<p>You clicked {count} times</p>
<button onClick={() => setCount(count + 1)}>Click me</button>
</div>
);
}
[Result]
Each time the button is clicked, the console logs "You clicked X times", where X is the current count.
In this example, useEffect is used to perform a side effect, which is logging to the console ea ch time the count state changes. The second argument to useEffect is an array of dependenci es—values that, when changed, will trigger the effect to run again. If this array is omitted, the effect runs after every render. If it's an empty array ([]), the effect runs only once after the initi al render, acting like componentDidMount. Here, by including [count] in the dependency arr ay, we ensure that the effect only runs when count changes, thus avoiding unnecessary exec utions that could occur on re-renders triggered by other state changes or props.
[Trivia]
Using dependency arrays correctly prevents memory leaks and unnecessary computations, i mproving performance. Misusing dependency arrays, such as omitting values that are used i n the effect, can lead to bugs where the effect operates on stale data.
Compatibility with External Libraries
Ensure external libraries used in your React project are compatible with your version of React.
Here is an example showing how to check for library compatibility with your React version.
[Code]
npm view [library-name] peerDependencies
[Result]
Displays the peer dependency requirements for the chosen library, which includes compatibl e React versions.
When adding an external library to your React project, it’s crucial to check its compatibility wi th the React version you are using. Using the command npm view [library-name] peerDepen dencies, you can see the library’s dependencies, including which versions of React it is compa tible with. This check helps prevent issues that arise from incompatibilities, such as unexpecte d behavior, crashes, or deprecated feature usage. Always make sure to update your libraries and React version accordingly to avoid security vulnerabilities and to use the latest features a nd performance improvements.
Regularly updating dependencies in a React project not only ensures compatibility but also r educes the risk of security vulnerabilities found in older versions. Always consult the library's documentation and change logs for detailed information on compatibility and known issues.
Understanding React's Concurrent Mode
React’s concurrent mode is an advanced feature designed to enhance the responsiveness an d performance of React applications by allowing multiple tasks to be executed in parallel.
Below is a simplified example showing how to enable and utilize Concurrent Mode in a React application. This example does not represent a real-world complex application, but gives you a basic idea of how to start using Concurrent Mode.
[Code]
import React from 'react';
import ReactDOM from 'react-dom';
import { unstable_createRoot as createRoot } from 'react-dom'; function App() {
return <h1>Hello, Concurrent Mode!</h1>;
}
// Assuming your HTML file has a div with id 'root'
const container = document.getElementById('root');
const root = createRoot(container); // Create a root.
root.render(<App />);
[Result]
The text "Hello, Concurrent Mode!" is displayed on the web page.
In the provided code:We import the necessary modules from React and ReactDOM.unstable_
createRoot is used instead of ReactDOM.render. Note that unstable_createRoot is part of the experimental API, indicating that concurrent mode is still experimental and subject to change s.createRoot creates a root container in which the React app is loaded. This method prepares your app to run in concurrent mode, which supports interruptible rendering allowing the app to pause, resume, and prioritize different updates more effectively.The App component simpl y renders a <h1> tag.This example is very basic and serves only as an introduction. Concurre nt mode can handle much more complex scenarios involving data fetching, state updates, an d interaction with large datasets more responsively.
[Trivia]
Concurrent Mode in React is not yet stable and is considered experimental. As of the latest u pdates, it's advisable to keep track of the React team's releases and announcements for chan ges or updates to the APIs used here. Using concurrent mode could significantly enhance the user experience by improving the responsiveness of an application, but it should be used wit h caution in production environments.
Implementing Code Splitting in React
Code splitting is a technique used in modern web development to split your code into variou s bundles, which can then be loaded on demand, improving the performance of the applicati on.
Here is an example of how to implement code splitting in a React application using React Ro uter and dynamic imports.
[Code]
import React from 'react';
import { BrowserRouter as Router, Route, Switch } from 'react-router-dom'; const Home = React.lazy(() => import('./Home'));
const About = React.lazy(() => import('./About'));
function App() {
return (
<Router>
<React.Suspense fallback={<div>Loading...</div>}>
<Switch>
<Route exact path="/" component={Home} />
<Route path="/about" component={About} />
</Switch>
</React.Suspense>
</Router>
}
export default App;
[Result]
When a user navigates to "/", the Home component loads. When navigating to "/about", the About component loads, each after displaying "Loading..." temporarily.
In the provided code:React.lazy is a function that allows you to render a dynamic import as a regular component. It is used here to defer loading of the components until they are actually needed, which reduces the initial load time.React.Suspense is a component that lets you spec ify the loading indicator in case the component takes time to load. In this example, it's a sim ple <div> with text "Loading...".BrowserRouter, Switch, and Route are components provided by React Router to handle routing. This setup ensures that only the necessary components ar e loaded and rendered based on the current route, effectively implementing code splitting.T
his example demonstrates how to apply route-level code splitting, which is crucial for improv ing the load time and performance of large-scale applications.
[Trivia]
Code splitting not only helps in reducing the initial load time but also in conserving bandwid th by loading only what is necessary as per user interactions. It's particularly beneficial in larg e-scale applications where the size of the application can significantly impact the load time a nd performance. React Router and React.lazy are two essential tools for implementing efficie nt code splitting in React applications.
Testing Strategies in React
To build reliable React applications, it is crucial to implement a robust testing strategy that in cludes unit, integration, and end-to-end tests.
Below is an example of how to set up a simple unit test in a React application using Jest, a po pular testing framework.
[Code]
import React from 'react';
import { render, screen } from '@testing-library/react'; import App from './App';
test('renders learn react link', () => {
render(<App />);
const linkElement = screen.getByText(/learn react/i);
expect(linkElement).toBeInTheDocument();
});
[Result]
If the test finds the text "learn react" in the document, it will pass. Otherwise, it will fail.
In the provided example, @testing-library/react is used for rendering components in a testin g environment. This library provides a set of methods (like render and screen.getByText) that allow you to query the DOM in the same way users would. Using expect(linkElement).toBeInT
heDocument(); asserts that the element is found in the document, helping ensure that your a pplication is rendering as expected.When developing React applications, it's beneficial to cat egorize tests into:Unit tests - These test individual components or functions.Integration tests
- These tests check how multiple components work together.End-to-end tests - These simula te user interactions from start to finish.This layered approach ensures comprehensive covera ge and helps catch issues at different levels of your application.
[Trivia]
Jest is commonly used for testing React applications due to its simplicity and support for co mplex testing needs. It integrates well with React's component-based architecture, making it a preferred choice among developers.
Optimizing React with Caching
Utilizing browser caching strategies with service workers can significantly improve the loadin g time of your React applications.
Below is an example of how to register a service worker in a React application to leverage br owser caching.
[Code]
// In your React application's src/index.js file
if ('serviceWorker' in navigator) {
window.addEventListener('load', () => {
navigator.serviceWorker.register('/service-worker.js')
.then(registration => {
console.log('ServiceWorker registration successful with scope: ', registration.scope);
}, err => {
console.log('ServiceWorker registration failed: ', err);
});
});
}
[Result]
On successful registration, the console will display "ServiceWorker registration successful wit h scope: followed by the scope of the service worker. On failure, it will show "ServiceWorker r egistration failed:" followed by the error message.
Service workers act as a proxy between the web application and the network. By intercepting network requests, they can manage how resources are handled, such as serving content from cache when offline or on slow networks.To optimize a React application:Precaching: Store im portant assets during the install phase of the service worker so they can be quickly loaded fr om cache on repeat visits.Runtime caching: Cache assets fetched during normal operation, w hich can be updated as needed.Implementing service workers requires handling various life c ycle events like installation, activation, and fetching. This example shows the basic registratio n process, which is typically included in the entry point of your React application, often in src
/index.js.
[Trivia]
While service workers can dramatically enhance performance, they also introduce complexity in handling offline scenarios and resource management. Testing and proper error handling a re crucial to avoid caching issues that can lead to outdated or incorrect data being served to users.
Accessibility in React
Making your React applications accessible ensures that they are usable by everyone, includin g individuals with disabilities.
The following code example demonstrates how to implement an accessible button compone nt in React:
[Code]
import React from 'react';
function AccessibleButton() {
return (
<button aria-label="Click me" onClick={() => alert('Button clicked!')}> Click me
</button>
);
}
export default AccessibleButton;
[Result]
When you click the button, it triggers an alert saying "Button clicked!"
This code snippet is a basic implementation of an accessible button in a React application. Th e aria-label attribute is crucial as it provides a text label for screen readers, which is essential for users who are visually impaired. The onClick event handler demonstrates how to add inte ractivity. When designing accessible React components, it's important to utilize ARIA (Accessi ble Rich Internet Applications) attributes to enhance the accessibility of elements that might not otherwise have sufficient context for assistive technologies.Here are a few additional poi nters:Semantic HTML: Use semantic HTML tags like <button>, <form>, <header>, which pro vide inherent accessibility features.Keyboard Navigation: Ensure that all interactive elements are navigable via keyboard in a logical order.Visual Indicators: Provide clear focus styles for in teractive elements to help users with limited vision or cognitive disabilities.Testing with Scree n Readers: Test your application using tools like NVDA, JAWS, or VoiceOver to experience ho w your application is presented to users with screen readers.
[Trivia]
Web Content Accessibility Guidelines (WCAG) are developed through the W3C process in co operation with individuals and organizations around the world, aiming to provide a single sh ared standard for web content accessibility that meets the needs of individuals, organizations
, and governments internationally.
Profiling React Performance
Profiling your React application helps you identify performance bottlenecks.
Below is a demonstration of how to use React DevTools to profile a React component:
[Code]
// This is a hypothetical command to open your React application's profiling tab in React Dev Tools
"Open your browser's developer tools, switch to the React DevTools tab, and click on the 'Pro filer' tab to start recording performance."
[Result]
You would see a flame graph showing the rendering time of each component.
React DevTools is an essential browser extension for Chrome and Firefox that helps develope rs inspect the React component hierarchy, including props, state, and more. The Profiler tab within React DevTools provides a powerful interface for recording and examining the perfor mance of your React application.To effectively use the Profiler:Start a profiling session: Hit th e record button in the Profiler tab before performing the actions in your application you wan t to analyze.Analyze performance data: After stopping the recording, you'll see a flame graph
that visualizes the render times of your components. Components that take longer to render are potential bottlenecks.Optimize based on findings: Focus on components with longer ren der times and consider optimizing them by checking their props, reducing re-renders, or usin g React.memo if applicable.Using profiling tools early and often in development can prevent performance issues from becoming deeply integrated into your application, making them ha rder to resolve later on.
[Trivia]
React 16.5 added a new feature called "Profiler" in the developer tools. This Profiler helps yo u measure the "cost" of rendering, identify which components re-render frequently or take lo ng to render, thereby helping you optimize your application effectively.
React List Virtualization
Using virtualization libraries like react-window or react-virtualized to enhance performance in React applications with large lists or tables.
The following example demonstrates how to use react-window to render a large list efficientl y.
[Code]
import React from 'react';
import { FixedSizeList as List } from 'react-window';
const MyLargeList = () => {
const itemCount = 1000; // Number of items in the list
const itemSize = 35; // Height of each row (in pixels)
const renderItem = ({ index, style }) => (
<div style={style}>Row {index}</div>
);
return (
<List
height={150}
itemCount={itemCount}
itemSize={itemSize}
width={300}
>
</List>
);
}
export default MyLargeList;
[Result]
The list component will efficiently render only the items that are currently visible on the scree n, improving performance for large datasets.
Virtualization is a technique where only a subset of the total items in a list are rendered at an y given time. This approach drastically reduces the amount of DOM nodes created and mana ged by the browser, which enhances overall performance and user experience. react-window is a popular library for implementing virtualization in React applications. It provides several c omponents to handle different types of lists and grids. The FixedSizeList component used her e is suitable for lists where each item has the same size. It requires the height of the visible p art of the list, the total itemCount, the itemSize, and the width of the list.
[Trivia]
React-window and react-virtualized differ primarily in their approach and flexibility. React-wi ndow is a lighter and simpler library ideal for simpler cases, while react-virtualized offers mor e features and flexibility but at a cost of greater complexity and size.
Graceful Data Handling in React
Properly managing data fetching and errors when integrating third-party APIs to improve us er experience in React apps.
The code snippet below illustrates handling data fetching and error states gracefully using Ax ios and React hooks.
[Code]
import React, { useState, useEffect } from 'react';
import axios from 'axios';
const FetchDataComponent = () => {
const [data, setData] = useState(null);
const [error, setError] = useState(null);
const [loading, setLoading] = useState(false);
useEffect(() => {
setLoading(true);
axios.get('https://api.example.com/data')
.then(response => {
setData(response.data);
setLoading(false);
})
.catch(error => {
setError('Failed to fetch data');
});
}, []);
return (
<div>
{loading && <p>Loading...</p>}
{error && <p>{error}</p>}
{data && <div>{JSON.stringify(data)}</div>}
</div>
);
};
export default FetchDataComponent;
[Result]
When loaded, the component displays either a loading message, an error message if the dat a fetch fails, or the data itself.
Using Axios for HTTP requests in React applications provides a robust way to handle asynchr onous data fetching. The example utilizes React's useEffect hook to perform the API call whe n the component mounts. The useState hook manages the state for data, error, and loading.
This setup ensures that the user interface always reflects the current state of the data fetch o peration. Handling errors gracefully is crucial for maintaining a good user experience, especia lly when network requests can fail or return unexpected results.
[Trivia]
Error handling in React applications often involves anticipating different types of errors that c an occur during data fetching, such as network errors, data format errors, or issues specific to the third-party service or API. Advanced patterns might include using error boundaries, a Rea
ct feature that allows components to catch JavaScript errors anywhere in their child compone nt tree.
Memoization in React
Use memoization to improve performance by caching results of expensive function calls.
Below is an example of using React.memo for component memoization and useMemo hook to memoize expensive calculations within a component.
[Code]
import React, { useMemo } from 'react';
// A memoized component
const ExpensiveComponent = React.memo(({ value }) => {
// Simulate an expensive calculation
const computeExpensiveValue = (val) => {
console.log('Calculating...');
return val * 2; // Example of an expensive operation
};
const result = useMemo(() => computeExpensiveValue(value), [value]); return <div>Result: {result}</div>;
});
export default function App() {
return <ExpensiveComponent value={10} />;
}
Upon the first render, the console logs 'Calculating...' and displays "Result: 20". Subsequent r enders with the same props do not re-calculate or log to the console.
React.memo is a higher order component for memoizing the result of a render based on pro ps and state, preventing unnecessary re-renders. The useMemo hook is used inside a compo nent to remember the result of an expensive calculation if the dependencies ([value] in this c ase) haven't changed. This saves processing time and resources, especially useful in large ap plications with complex computations or frequent updates.When value remains the same acr oss re-renders, ExpensiveComponent does not re-calculate the result, improving performanc e. The console log only occurs when the calculation is actually performed, which is a good wa y to track unnecessary computations.
[Trivia]
Memoization is a programming technique used extensively in optimization problems, particu larly useful in React to avoid expensive recalculations on every render. The dependencies arra y in hooks like useMemo and useCallback precisely defines when re-computation should occ ur.
CSS Organization in React
Implement CSS methodologies to enhance maintainability and scalability in large projects.
Example using the BEM (Block, Element, Modifier) methodology in a React project.
[Code]
import React from 'react';
import './App.css'; // Assuming CSS is defined in App.css function App() {
return (
<div className="app">
<header className="app__header">
<h1 className="app__title">Welcome to My React App!</h1>
<button className="app__button app__button--primary">Click Me!</button>
</header>
</div>
);
}
export default App;
/* App.css */
.app {}
.app__header {
background: #f0f0f0;
}
.app__title {
color: #333;
}
.app__button {
padding: 10px 20px;
border: none;
background-color: #007bff;
color: white;
cursor: pointer;
}
.app__button--primary {
background-color: #0056b3;
}
[Result]
The webpage displays a header with a title "Welcome to My React App!" and a primary blue button saying "Click Me!".
Using BEM in React helps to avoid CSS naming collisions and enhances component-based st yling. Each class name follows the block__element--modifier format, making the structure an d purpose of CSS clear. This organization facilitates easier maintenance, scalability, and reuse of styles across components in large projects.The .app class represents the block, .app__head er, and .app__title are elements, and .app__button--primary is a modifier indicating a variatio n of the app__button element. This structure helps developers quickly understand which part of the application UI is styled by each rule.
BEM is not the only methodology for organizing CSS. Other popular methods include SMAC
SS (Scalable and Modular Architecture for CSS) and OOCSS (Object-Oriented CSS), each with their own principles aimed at reducing style conflicts and fostering reusability in increasingly complex web projects.
React Batched Updates
React’s batched updates optimize performance by grouping multiple setState() calls into a si ngle re-render for better user experience.
The following example demonstrates how React batches multiple setState() calls from within the same event handler.
[Code]
import React, { useState } from 'react';
function Counter() {
const [count, setCount] = useState(0);
const [increment, setIncrement] = useState(1);
const handleClick = () => {
setCount(count + increment);
setIncrement(increment + 1);
};
return (
<div>
<p>Current count: {count}</p>
<button onClick={handleClick}>Increment</button>
</div>
);
}
[Result]
The displayed count increases by an increasing value each time the button is clicked.
In this example, when the button is clicked, the handleClick function is triggered, which conta ins two setState() calls. React intelligently batches these calls, meaning the component only r e-renders once after both states (count and increment) are updated. This avoids unnecessary re-renders and thus improves performance. If React did not use batched updates, each setSt ate() call would cause a separate re-render, which would be less efficient.
[Trivia]
Batching is automatic in React 18 and newer for updates triggered within React event handle rs and lifecycle methods. It uses a scheduling model called "concurrent mode" to prioritize u pdates and manage heavy computations more efficiently.
Using React Children Prop
Avoid passing children as props if possible; instead, use React’s built-in children prop to man age component contents.
This example shows how to correctly use React's built-in children prop to pass elements bet ween components.
[Code]
import React from 'react';
function Page({ children }) {
return <div className="page">{children}</div>;
}
function App() {
return (
<Page>
<h1>Welcome to My App</h1>
<p>This is the content of the page.</p>
</Page>
);
}
export default App;
The page will display a heading and a paragraph within a div container.
In this example, Page is a component that receives children as a prop. This prop is a special p rop in React, automatically passed to components that represent whatever is included betwe en the opening and closing tags when invoking a component. By using the children prop, the App component can pass structured HTML directly into the Page, keeping the composition fl exible and maintainable. It promotes better reuse and separation of concerns compared to p assing children directly as props.
[Trivia]
React’s children prop is part of React’s top-level API. It allows components to be passed as d ata to other components, providing a powerful tool for creating dynamic and flexible compo nent hierarchies.
Handling Asynchronous Operations in React
Asynchronous operations in React, such as fetching data or timers, should be managed caref ully to avoid issues like race conditions when updating the state.
Here is a simple example using useEffect to handle asynchronous data fetching with state up dates.
[Code]
import React, { useState, useEffect } from 'react';
function UserData() {
const [userData, setUserData] = useState(null);
const [loading, setLoading] = useState(true);
useEffect(() => {
const fetchData = async () => {
const response = await fetch('https://api.example.com/user'); const data = await response.json();
setUserData(data);
setLoading(false);
};
fetchData();
return () => {
console.log('Cleanup on component unmount or update');
};
}, []); // Empty dependency array ensures the effect runs only once if (loading) {
return <div>Loading...</div>;
}
return (
<div>
<h1>User Details</h1>
<p>Name: {userData.name}</p>
<p>Email: {userData.email}</p>
</div>
);
}
export default UserData;
[Result]
If the fetch operation completes successfully, the component displays user details. Otherwise, it shows a loading message until the data is ready.
In this example, useState is used to manage the userData and loading state variables. useEffe ct is used for side effects, in this case, fetching data from an API. An empty dependency array
[] means the effect runs once after the initial render, similar to componentDidMount in class components. The cleanup function logged in the console helps avoid memory leaks or unwa nted behavior by cleaning up resources or subscriptions when the component unmounts or before the effect runs again. This is crucial to prevent race conditions where a previous state update might override a newer one if not handled properly.
[Trivia]
Race conditions in React occur when multiple state updates happen in a way that the output depends on the sequence of these updates, which can be non-deterministic if not properly managed. Using asynchronous operations without cleanup can lead to situations where state updates are attempted on unmounted components, leading to memory leaks and errors like
"Can't perform a React state update on an unmounted component."
Optimizing React Bundle Size
Monitoring and optimizing the bundle size of your React application can improve load times and overall performance.
Here is how you can use Webpack Bundle Analyzer to visualize and analyze the size of your Webpack output files.
[Code]
# Install Webpack Bundle Analyzer
npm install --save-dev webpack-bundle-analyzer
# Modify your Webpack configuration
const BundleAnalyzerPlugin = require('webpack-bundle-analyzer').BundleAnalyzerPlugin; module.exports = {
// Your existing Webpack config
plugins: [
new BundleAnalyzerPlugin()
]
};
[Result]
When you run your build script, Webpack Bundle Analyzer will open a web page displaying a visual map of your bundle, showing which modules are taking up space.
The Webpack Bundle Analyzer plugin integrates into your build process to provide a visual re presentation of the size of each module in your bundle. This visualization helps identify large libraries or chunks that may be optimized or lazy-loaded. Configuration is straightforward; af ter installing the plugin, you import it in your Webpack configuration file and add it to the pl ugins array. When you run the build, the analyzer shows a visual representation of your bund le in an interactive treemap, allowing you to spot large dependencies easily. This tool is inval uable for keeping your application's performance in check, especially for complex application s intended to run in a browser environment.
[Trivia]
Large JavaScript bundles can significantly affect page load times, particularly on mobile devic es with slower network connections. Efficiently managing bundle size not only improves loadi ng times but also enhances the user experience by reducing the time to interactive (TTI). Tec hniques such as code splitting, tree shaking, and using more efficient libraries can further hel p in reducing the overall size of your application.
CSS Modules in React
Using CSS Modules in React helps you manage styles in your components more effectively, e nsuring that styles do not conflict with each other.
Here, we create a React component and apply CSS Modules to encapsulate its styles.
[Code]
// App.js
import React from 'react';
import styles from './App.module.css';
function App() {
return <div className={styles.container}>Hello, CSS Modules!</div>;
}
export default App;
// App.module.css
.container {
background-color: lightblue;
padding: 20px;
border: 1px solid blue;
}
[Result]
A web page displaying "Hello, CSS Modules!" with a light blue background, 20px padding, an d a blue border.
CSS Modules are a technique in React that scopes CSS by automatically creating a unique cla ssname for each style definition. This encapsulation prevents styles from leaking across comp onents, avoiding unintended styling conflicts. When you import a CSS Module, like import st yles from './App.module.css';, it imports an object where properties are class names mapped to their corresponding scoped names generated at build time.For instance, .container might be converted to something like .container__3zyFi, ensuring its styles only apply to componen ts using styles.container. This makes it very easy to manage styles in large applications, as ea ch component's styles are isolated, reducing the risk of conflicting styles.
[Trivia]
CSS Modules work by appending a unique identifier to class names at build time. This identifi er can be customized using the webpack or other build tools' configuration to follow a specif ic pattern, such as including the file name or a hash of the content.
Functional Programming in React
Adopting functional programming principles in React can enhance your components' predict ability and maintainability.
Here, we will demonstrate a simple React component that utilizes functional programming c oncepts such as pure functions and immutability.
[Code]
// App.js
import React, { useState } from 'react';
function useCounter(initialCount = 0) {
const [count, setCount] = useState(initialCount);
function increment() {
setCount(prevCount => prevCount + 1);
}
function decrement() {
setCount(prevCount => prevCount - 1);
}
return { count, increment, decrement };
}
function Counter() {
const { count, increment, decrement } = useCounter();
return (
<h1>Count: {count}</h1>
<button onClick={increment}>Increment</button>
<button onClick={decrement}>Decrement</button>
</div>
);
}
export default Counter;
[Result]
A web page with a counter displaying "Count: 0" and two buttons labeled "Increment" and "
Decrement".
Functional programming (FP) in React involves using pure functions and managing state with out side effects. Pure functions are those that, for the same input, always return the same out put and do not cause any observable side effects. The useState hook is used to manage state in functional components, adhering to FP principles by not directly mutating state, but by usi ng a setter function that enforces the immutability of state.The custom hook useCounter de monstrates FP by encapsulating state logic in a reusable function that manages the state wit hout side effects, allowing for more predictable and testable code. By structuring your code t his way, components become easier to understand, test, and maintain over time, fostering a cleaner and more modular codebase.
[Trivia]
React's functional components and hooks were introduced to facilitate the use of functional programming patterns within components. Hooks, such as useState and useEffect, allow dev elopers to write side-effect-free code by clearly segmenting effects from the main logic, align ing with the core principles of functional programming.
◆
As we close the pages on this guide, we hope that the focused essentials provided here hav e empowered you in your journey through React. Designed specifically for individuals alread y familiar with fundamental programming concepts, this book aimed to deliver just what is n ecessary for beginners to gain a robust understanding of React, without overwhelming detail.
For seasoned professionals, this resource serves as an efficient refresher on the latest essenti als in React development. We have tailored the content to be concise and directly relevant, al lowing for quick assimilation and application of key React features.
If this book has been helpful to you, please consider leaving a review or comment. Sharing y our experiences can greatly assist other engineers in similar situations to discover and utilize this resource. Your feedback not only enriches your fellow readers' learning experiences but also supports the continuous improvement of this guide.
Thank you for choosing to advance your skills with us. We look forward to your insights and wish you continued success in your React endeavors.
Reads:
38
Pages:
216
Published:
Apr 2024
Dive into the world of earning without the daily grind with our "Passive Income Encyclopedia". This e-book is crafted specifically for beginners, providing a ...
Formats: PDF, Epub, Kindle, TXT