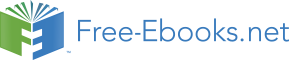

Table of Content
Assembly Programming Tutorial .............................................. 2
Audience.................................................................................. 2
Prerequisites ............................................................................ 2
Copyright & Disclaimer Notice.................................................. 3
Assembly Introduction.............................................................. 8
What is Assembly Language?................................................. 8
Advantages of Assembly Language ...........................................................8
Basic Features of PC Hardware .................................................................9
The Binary Number System .......................................................................9
The Hexadecimal Number System.............................................................9
Binary Arithmetic ......................................................................................10
Addressing Data in Memory .....................................................................11
Assembly Environment Setup ................................................ 13
Installing NASM........................................................................................13
Assembly Basic Syntax .......................................................... 15
The data Section ......................................................................................15
The bss Section .......................................................................................15
The text section ........................................................................................15
Comments ................................................................................................15
Assembly Language Statements..............................................................16
Syntax of Assembly Language Statements ..............................................16
The Hello World Program in Assembly.....................................................16
Compiling and Linking an Assembly Program in NASM ...........................17
Assembly Memory Segments................................................. 18
Memory Segments ...................................................................................18
Assembly Registers ............................................................... 20
Processor Registers .................................................................................20
Data Registers .........................................................................................20
Pointer Registers......................................................................................21
Index Registers ........................................................................................21
Control Registers .....................................................................................22
Segment Registers...................................................................................22
Example: ..................................................................................................23
Assembly System Calls.......................................................... 24
Linux System Calls...................................................................................24
Example ...................................................................................................25
Addressing Modes ................................................................. 27
Register Addressing .................................................................................27
Immediate Addressing..............................................................................27
Direct Memory Addressing .......................................................................28
Direct-Offset Addressing ..........................................................................28
Indirect Memory Addressing.....................................................................28
The MOV Instruction ................................................................................28
SYNTAX: ..................................................................................................28
E XAMPLE: ............................... ................................ ............................... 29
Assembly Variables ............................................................... 31
Allocating Storage Space for Initialized Data ...........................................31
Allocating Storage Space for Uninitialized Data .......................................32
Multiple Definitions ...................................................................................32
Multiple Initiali zations ...............................................................................33
Assembly Constants .............................................................. 34
The EQU Directive ...................................................................................34
Example: ..................................................................................................34
The %assign Directive..............................................................................35
The %define Directive ..............................................................................35
Arithmetic Instructions............................................................ 37
SY NT A X : ............................... ................................ ................................ .. 37
E XAMPLE: ............................... ................................ ............................... 37
The DEC Instruction .................................................................................37
SY NT A X : ............................... ................................ ................................ .. 37
E XAMPLE: ............................... ................................ ............................... 37
The ADD and SUB Instructions ................................................................38
SYNTAX: ..................................................................................................38
EXAMPLE: ...............................................................................................38
The MUL/IMUL Instruction .......................................................................40
SYNTAX: ..................................................................................................40
EXAMPLE: ...............................................................................................41
E XAMPLE: ............................... ................................ ............................... 41
The DIV/IDIV Instructions.........................................................................42
SY NT A X : ............................... ................................ ................................ .. 42
E XAMPLE: ............................... ................................ ............................... 43
Logical Instructions ................................................................ 45
The AND Instruction .................................................................................45
Example: ..................................................................................................46
The OR Instruction ...................................................................................46
Example: ..................................................................................................47
The XOR Instruction.................................................................................47
The TEST Instruction ...............................................................................48
The NOT Instruction .................................................................................48
Assembly Conditions.............................................................. 49
The CMP Instruction.................................................................................49
SYNTAX...................................................................................................49
EXAMPLE: ...............................................................................................49
Unconditional Jump..................................................................................50
SYNTAX: ..................................................................................................50
EXAMPLE: ...............................................................................................50
Conditional Jump .....................................................................................50
Example: ..................................................................................................51
Assembly Loops..................................................................... 53
Example: ..................................................................................................53
Assembly Numbers ................................................................ 55
ASCII Representation...............................................................................56
BCD Representation ................................................................................57
Example: ..................................................................................................57
Assembly Strings ................................................................... 59
String Instructions ....................................................................................59
MOVS.......................................................................................................60
LODS .......................................................................................................61
CMPS.......................................................................................................62
SCAS .......................................................................................................63
Repetition Prefixes ...................................................................................64
Assembly Arrays .................................................................... 65
Example: ..................................................................................................66
Assembly Procedures ............................................................ 67
Syntax: .....................................................................................................67
Example: ..................................................................................................67
Stacks Data Structure: .............................................................................68
E XAMPLE: ............................... ................................ ............................... 69
Assembly Recursion .............................................................. 70
Assembly Macros................................................................... 72
Example: ..................................................................................................73
Assembly File Management ................................................... 74
File Descriptor ..........................................................................................74
File Pointer ...............................................................................................74
File Handling System Calls ......................................................................74
Creating and Opening a File ....................................................................75
Openi ng an Existing File ..........................................................................75
Reading from a File ..................................................................................75
Writing to a File ........................................................................................76
Closing a File ...........................................................................................76
Updating a File .........................................................................................76
Example: ..................................................................................................77
Memory Management ............................................................ 79
Example: ..................................................................................................79