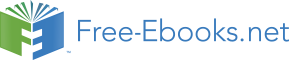

9. Garbage Collector
When programming in older languages like C or C++, programmers had to keep track of objects and release them when necessary. If an object is created and not released then this object is using resources from the system that will never be freed. The most common symptom is memory leaks. A program that is leaking memory will contentiously use more memory till the system runs out of memory and probably crashes. Those bugs are usually very difficult to find in code.
Modern languages have garbage collector's that keeps track of used objects. When the system runs low on memory resources, the garbage collector jumps in and searches through all objects and frees the ones with no “references”. Do you remember how we created objects before using the “new” keyword and then we assigned the object to a “reference”? An object can have multiple references and the garbage collector will not remove the object till it has zero references.
Note that the object is not removed immediately. When needed, the Garbage collector will run and remove the object. This can be an issue in some rare cases because the garbage collector needs some time to search and remove objects. It will only be few milliseconds but what if your application can't afford that? If so, the garbage collector can be forced to run at a desired anytime.
9.1. Losing Resources
The garbage collector ease's object allocation but it can also cause problems if we are not careful. A good example would be on using digital output pins. Lets say we need a pin to be high. We create an OutputPort object and set the pin high. Later on we lose the “reference” for that object for some reason. The pin will still be high when the reference is lost so all is good so far. After a few minutes, the garbage collector kicks in and it finds this unreferenced object, so it will be removed. Freeing an OutputPort will cause the pin to change its state to input. Now, the pin is not high anymore!
An important thing to note is that if we make a reference for an object inside a method and the method returns then we have already lost the reference. Here is an example
To solve this, we need a reference that is always available. Here is the correct code
Another good example is using timers. NETMF provides a way to create timers that handle work after a determined time. If the reference for the timer is lost and the garbage collector runs, the timer is now lost and it will not run as expected.
Important note: If you have a program that is working fine but then right after you see the GC running in the “Output Window”, and the program stops working or raises an exception, then this is because the GC has removed an object that you need. Again, that is because you didn't keep references to the object you wanted to keep alive.
9.2. Dispose
The garbage collector will free objects at some point but what if we need to free one particular object immediately? Most objects have a Dispose method. If an object needs to be freed at anytime, we can “dispose” of it.
Disposing an object is very important in NETMF. When we create a new InputPort object, the assigned pin is reserved. What if we want to use the same pin as an output? Or even use the same pin as an analog input? We will first need to free the pin and then create the new object.
9.3. GC Output Messages
When the garbage collector runs, it outputs a lot of useful information to the output window. These messages give you an idea of what is using resources in the system. Although not recommended, you may want to disable those messages to free up the output window for your own usage. This is easily achievable using this line of code.