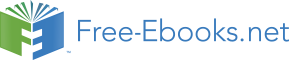

10. C# Level 3
This section will cover all the C# materials we wanted to include in this book. A good and free eBook to continue learning about C# is available at http://www.programmersheaven.com/2/CSharpBook
10.1. Byte
We learned how int is useful to store numbers. They can store very large numbers but every int consumes four bytes of memory. You can think of a byte as a single memory cell. A byte can hold any value from 0 to 255. It doesn't sound like much but this is enough for a lot of things. In C# bytes are declared using “byte” just like how we use “int”.
The maximum number that a byte can hold is 256 [0..255], What’s going to happen if we increment it over 255? Incrementing 255 by one would overlap the value back to zero.
You will probably want to use int for most of your variables but we will learn later where bytes are very important when we start using arrays.
10.2. Char
To represent a language like English, we need 26 values for lower case and 26 for upper case then 10 for numbers and maybe another 10 for symbols. Adding all these up will give us a number that is well less than 255. So a byte will work for us. If we create a table of letters, numbers and symbols, we can represent everything with a numerical value. Actually, this table already exists and is called the ASCII table.
So far a byte is sufficient to store all “characters” we have in English. Modern computer systems have expanded to include other languages, some use very complex non-Latin characters. The new characters are called Unicode characters. Those new Unicode characters can be more than 255 and so a byte is not sufficient and an integer (four bytes) is too much. We need a type that uses 2-bytes of memory. 2-bytes is good to store numbers from 0 to over 64,000. This 2-byte type is called “short”, which we are not using in this book.
Systems can represent characters using 1-byte or using 2-bytes. Programmers decided to create a new type called char where char can be 1-byte or 2-bytes, depending on the system. Since NETMF is made for smaller systems, its char is actually a byte! This is not the case on a PC where a char is a 2-byte variable!
Do not worry about all this mess, do not use char if you do not have to and if you use it, remember that it is 1-byte on NETMF.
10.3. Array
If we are reading an analog input 100 times and we want to pass the values to a method, it is not practical to pass 100 variables in 100 arguments. Instead, we create an “array” of our variable type. You can create an array of any object. We will mainly be using byte arrays. When you start interfacing to devices or accessing files, you will always be using byte arrays.
Arrays are declared similar to objects.
The code above creates a “reference” of an object of type “byte array”. This is a reference but it doesn't have an object yet, it is null. If you forgot what is a reference then go back and read more in the C# Level 2 chapter.
To create the object we use the “new” keyword and then we need to tell it the size of our array. This size is the count of how many elements of the type we will have in an array. Our type is a byte, so the number is how many bytes we are allocating in memory.
We have created a byte array with 10 elements in it. The array object is referenced from “MyArray”.
We now can store/read any of the 10 values in the array by indicating which “index“ we want to access.
A very important note here is that indexes start from zero. So, if we have an array of size 10, then we have indexes from 0 to 9. Accessing index 10 will NOT work and will raise an exception.
We can assign values to elements in an array at the time of initialization. This example will store the numbers 1 to 10 in indexes 0 to 9.
To copy an array, use the Array class as follows
One important and useful property of an array is the Length property. We can use it to determine the length of an array.
10.4. String
We have already used strings in many places. We will review what we have learned and add more details.
Programs usually need to construct messages. Those messages can be human readable text. Because this is useful and a commonly used feature in programs, C# supports strings natively. C# knows if the text in a program is a string if it is enclosed by double-quotes.
This is a string example.
Whatever is inside the double quotes is colored in red and considered to be a string. Note how in the second line I purposely used the same text in the string to match what I used to assign the string. C# doesn't compile anything in quotes (red text) but only takes it as it is; a string.
You may still have confusion on what the difference between an integer variable that has 5 in it and a string that has 5 in it is. Here is example code
What do you think the actual value of the variables now? For integer, it is 10 as 5+5=10. But for string this is not true. Strings do not know anything about what is in it, text or numbers make no difference. When adding two strings together, a new string is constructed to combine both. And so “5”+”5”=”55” and not 10 like integers.
Almost all objects have a ToString method that converts the object information to a printable text. This demonstration shows how ToString works
Running the above code will print:
The value of MyInteger is: 10
Strings can be converted to byte arrays if desired. This is important if we want to use a method that only accepts bytes and we want to pass our string to it. If we do that, every character in the string will be converted to its equivalent byte value and stored in the array
10.5. For-Loop
Using the while-loop is enough to serve all our loop needs but for-loop can be easier to use in some cases. The simplest example is to write a program that counts from 1 to 10. Similarly, we can blink an LED 10 times as well. The for-loop takes three arguments on a variable. It needs the initial value, how to end the loop and what to do in every loop
We first need to declare a variable to use. Then in the for-loop, we need to give it three arguments (initial, rule, action). In the very first loop, we asked it to set variable “i” to zero. Then the loop will keep running as long as the variable “i” is less then 10. Finally, the for-loop will increment variable i in every loop. Let us make a full program and test it.
If we run the program above, we will see that it is printing i from 0 to 9 but not 10. But, we wanted it to run from 1 to 10 and not 0 to 9! To start from 1 and not 0, we need to set i to 1 in the initial loop. Also, to run to 10, we need to tell the for-loop to turn all the way to 10 and not less than 10 so we will change the less than (“<”) with less than or equal (“<=”)
Can we make the 'for' loop count only even numbers (increment by two)?
The best way to understand for-loops is by stepping in code and seeing how C# will execute it.
10.6. Switch Statement
You probably won't use the switch statement for beginner applications but you will find it very useful when making large programs, especially when handling state-machines. The switchstatement will compare a variable to a list of constants (only constants) and make an appropriate jump accordingly. In this example, we will read the current “DayOfWeek” value and then from its value we will print the day as a string. We can do all this using if-statement but you can see how much easier switch-statement is, in this case.
One important note about switch-statements is that it compares a variable to a list of constants. After every “case” we must have a constant and not a variable.
We can also change the code to switch on the enumeration of days as the following shows:
Try to step in the code to see how switch is handled in details.