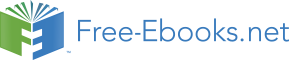

7. C# Level 2
7.1. Boolean Variables
We learned how integer variables hold numbers. In contrast, Boolean variables can only be true or false. A light can only be on or off, representing this using an integer doesn't make a lot of sense but using Boolean, it is true for on-state and false for off-state. We have already used those variables to set digital pins high and low, LED.Write(true);
To store the value of a button in a variable we use
We also used while-loops and we asked it to loop forever, when we used true for the statement
Take the last code we did and modify it to use a boolean, so it is easier to read. Instead of passing the Button state directly to the LED state, we read the button state into button_state boolean then we pass the button_state to set the LED accordingly.
Can you make an LED blink as long as the button is pressed?
Important note: The == is used to check for eqality in C#. This is different from = which is used to assign values.
7.2. if-statement
An important part of programming is checking some state and taking action accordingly. For example, “if” the temperature is over 80, turn on the fan.
To try the if-statement with our simple setup, we want to turn on the LED “if” the button is pressed. Note this is the opposite from what we had before since in our setup, the button is low when it is pressed. So, to achieve this we want to invert the state of the LED from the state of the button. If the button is pressed (low) then we want to turn the LED on (high). The LED needs to be checked repeatedly so we will do it once every 10ms.
7.3. if-else-statements
We learned how if-statements work. Now, we want to use the else-statement. Basically, “if” a statement is true, the code inside the if-statement runs or “else” the code inside the elsestatement will run. With this new statement, we can optimize the code above to be like this
I will let you in on a secret! We only used the if-statement and the else-statement in this example as a demonstration purpose. We can write the code this way.
Or even this way!
Usually, there are many way to write the code. Use what makes you comfortable and with more experience, you will learn how to optimize the code.
7.4. Methods and Arguments
Methods are actions taken by an object. It can also be called a function of an object. We have already seen methods and have used them. Do you remember the object Debug which has a Print method? We have already used Debug.Print many times before, where we gave it a “string” to display in the output window. The “string” we passed is called an argument.
Methods can take one or more optional arguments but it can only return one optional value.
The Print method in the Debug object only takes one string argument. Other methods may not require any arguments or may require more than one argument. For example, a method to draw a circle can take four arguments, DrawCircle(posx, posy, diam, color). An example for returning values can be a method that returns the temperature.
So far, we have learned of three variable types, int, string and bool. We will cover other types later but remember that everything we talk about here applies to other variable types.
The returned value can be an optional variable type. If there is no returned value then we replace the variable type with “void”. The “return” keyword is used to return values at the end of a method.
Here is a very simple method that “returns” the sum of two integers.
We started the method with the return value type, “int” followed by the method name. Then we have the argument list. Arguments are always grouped by parenthesis and separated by commas.
Inside the Add method, a local integer variable has been declared, var3. Local variables are created inside a method and die once we exit the method. Now, we add our two variables and finally return the result.
What if we want to return a string representing the sum of two numbers? Remember that a string containing the number 123 is not the same as an integer containing 123. An integer is a number but a string is an array or characters that represent text or numbers. To humans these are the same thing, but in the computer world, this is totally different.
Here is the code to return a string.
You can see how the returned type was changed to a string. We couldn't return var3 because it is an integer variable, so we had to convert it to a string. To do that, we create a new variable object named MyString. Then convert var3 "ToString" and place the new string in MyString.
The question now is why we called a "ToString" method on a variable of type integer? In reality, everything in C# is an object, even the built in variable types. This book is not going into these details as it is only meant to get you started.
This is all done in multiple steps to show you how it is done but we can compact everything and results will be exactly the same.
I recommend you do not write code that is extremely compact, like the example above, until you are very familiar with the programming language. Even then, there should be limits on how much you compact the code. You still want to be able to maintain the code after sometime and someone else may need to read and understand your code.
7.5. Classes
All objects we talked about so far are actually “classes” in C#. In modern object oriented programming languages, everything is an object and methods always belong to one object. This allows for having methods of the same name but they can be for completely different objects. A “human” can “walk” and a “cat” can also “walk” but do they walk the same way? When you call the “walk” method in C# it is not clear if the cat or the human will walk but using human.walk or cat.walk makes it more clear.
Creating classes is beyond the scope of this book. Here is a very simple class to get you started
7.6. Public vs. Private
Methods can be private to a class or publicly accessible. This is only useful to make the objects more robust from programmer misuse. If you create an object (class) and this object has methods that you do not want anyone to use externally then add the keyword “private” before the method return type; otherwise, add the “public” keyword.
Here is a quick example
7.7. Static vs. non-static
Some objects in life have multiple instances but others only exist once. The objects with multiple instances are non-static. For example, an object representing a human doesn't mean much. You will need an “instance” of this object to represent one human. So this will be something like human Mike;
We now have a “reference” called Mike of type human. It is important to note this reference at this point is not referencing any object (no instance assigned) just yet, so it is referencing NULL.
To create the “new” object instance and reference it from Mike
Mike = new human();
We now can use any of the human methods on our “instance” of Mike
Mike.Run(distance);
Mike.Eat();
bool hungry = Mike.IsHungry();
We have used those non-static methods already when we controlled input and output pins.
When creating a new non-static object, the “new” keyword is used with the “constructor” of the object. The constructor is a special type of method that returns no value and is only used when creating (construction) new objects.
Static methods are easier to handle because there is only one object that is used directly without creating instances. The easiest example is our Debug object. There is only one debug object in the NETMF system so using its methods, like Print, is used directly. Debug.Print(“string”);
I may not have used the exact definitions of static and instances but I wanted to describe it in the simplest way.
7.8. Constants
Some variables may have fixed values that never change. What if you accidentally change the value of this variable in your code? To protect it from changing, add the “const” keyword before the variable declaration.
7.9. Enumeration
An Enumeration is very similar to a constant. Let's say we have a device that accepts four commands, those are MOVE, STOP, LEFT, RIGHT. This device is not a human, so these commands are actually numbers. We can create constants (variables) for those four commands like the following:
The names are all upper case because this is how programmers usually name constants. Any other programmer seeing an upper case variable would know that this is a constant.
The code above is okay and will work but it will be nicer if we can group those commands
With this new approach, there is no need to remember what commands exist and what the command numbers are. Once the word “Command” is typed in, Visual Studio will give you a list of available commands.
C# is also smart enough to increment the numbers for enumerations so the code can be like this listing and will work exactly the same way