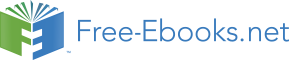

7. The online account display
Looking at the account number file report but you may ask why we didn’t save paper and display the fields on the screen. To save the destruction of trees for paper for our reports, our system will create the report but not actually print it to paper. Instead it will display data on the screen. You can get a full report printed but it will cost you. The intent is to make the cost so prohibitive that people will think twice about getting it on paper. For a lesser amount you can have just the page you need printed. This will cause people to think about the reports they request and be more concerned for the environment. At the end of the chapter, I will talk briefly about going paperless.
Obviously we need to look at data on files but we can do that without looking at a piece of paper. I never want to read a novel online but data in the office is an entirely different story. How to get the report on the screen will depend on the system you have so I won’t get into the details. We can peek at records on the account file if we have some kind of inquiry program online. Since our file is indexed, we can look at a specific record if we know the account number.
Before getting into the actual program, let me talk about program specifications. These are nothing more a detailed summary of what the program is supposed to do. They can be very long, encompassing not only what is to take place but also how that should be done. On the other hand specifications can be brief, to the point and it is up to the programmer to do the rest. There’s a joke in the computing business about specs written on the back of a napkin and I’m sure that some have used that medium.
Our specifications can be written as
Write a program to display account file fields based on the account number.
It could also be
Transfer the original account file report to the screen but limit it to one record at a time depending on the account number input.
Vague as these specs may be, in either case you get the idea of what needs to be done and how it is to be done is up to the programmer as well as where to place the fields on the screen. There’s quite a bit of freedom in getting the data to appear but you can understand that we don’t want a cluttered screen and it should be user friendly. After all, we don’t want to do the programming and later have some person say they are not happy with the way it looks. Actually, our goal is to have the user be so thrilled that she holds a party to celebrate the occasion.
In order to get data on the screen we need the keyword,
screen.
The computer monitor in most cases has 24 rows and 80 columns to display characters. Reducing the size of the characters can increase the number of rows and columns on the screen, but you need to be able to read the data. In order to print Account Number Inquiry on the first line and centered we need the line,
screen(1,30) “Account Number Inquiry”,
which will display the above heading in the first row starting at position 30. Any data in those positions on the line when this command is executed will be deleted. Note that if there happened to be something on the screen in positions 1 through 29, it will not be removed. To clear this trash, you can use the line
screen(1,1) “ ” (1,30) “Account Number Inquiry”
or
screen(1,1) “ Account Number Inquiry”
and the result in either case will be only those three words on the first line. This centers the heading in the line.
The first number after the left parenthesis indicates the row where the field will start while the second number represents the column. It should be noted that only the first line will be effected by our screen command. If we use either line above in our program we may run into some difficulties since our screen could already have information all over it on other lines. Our data would still be displayed but so would much of the data that was there before. We will need to clear the screen and this can be done with the line
screen erase
where the combination of these two keywords will remove all previous data from the screen so that we will be ready to display just what we want and nothing more. If we use the line
screen(20,20) “error”
the literal error will print on line 20 starting in the 20th column but nothing will be erased before column 20. Adding the line
screen erase(20,1)
before that screen line with the literal error will erase whatever is on line 20. Thus
screen erase(10,18)
will erase everything on line 10 starting in column 18.
The complete program would look like the following:
program-name: acctinq
define file acctfile record account-record status acct-status key account-number structure
account-number integer(9)
last-name character(18)
first-name character(15)
middle-initial character
street-address character(25)
city character(15)
state character(2)
zip-code integer(5)
balance signed decimal(6.2)
define error-msg character(60) value “ ”
screen erase
screen(1,30) “Account Number Inquiry”
screen(4,20) “account number:”
screen(6,20) “last name:”
screen(8,20) “first name:”
screen(10,20) “middle initial:”
screen(12,20) “street address:”
screen(14,20) “city:”
screen(16,20) “state:”
screen(18,20) “zip code:”
screen(20,20) “account balance:”
screen(22,20) “to exit, enter 0 for the account number”
input-number: input(4,36) account-number
screen(24,1) erase
if account-number = 0
go to end-program
end-if
read acctfile key account-number
if acct-status = 0
screen(4,36) account-number
screen(6,36) last-name
screen(8,36) first-name
screen(10,36) middle-initial
screen(12,36) street-address
screen(14,36) city
screen(16,36) state
screen(18,36) zip-code
screen(20,36) balance, mask($$$$,$$9.99-)
else
if acct-status = 5
screen(24,20) “The account # “ account-number “ is not on the file.”
else
error-msg = “Problem with the account file; program ending – press enter”
go to end-program
end-if
end-if
go to input-number
end-program: screen(24,1) erase screen(24,20) error-msg input
end
Some of the statements should be familiar to you. Note first that the title of the account number inquiry program is
acctinq
and our second statement
define file acctfile record account-record status acct-status key account-number structure
introduces the keyword
file,
which we should have had in the earlier programs. It lets us know that we are defining a file. The file status is also included in our definition of the file – we don’t need another line for that, though we could have had a separate definition of it – and so are the record layout or structure, and the key of the file, since we are inquiring on the account. As mentioned earlier, all the files in our system are indexed files, so we’ll read them with a keyed read, even if we process the file one record at a time.
We clear the screen, but only once, and print the title on line 1 in column 30, followed by all the other headings on the appropriate lines. The next line introduces a new keyword:
input-number: input(4,36) account-number
giving the person at the screen the chance to enter a nine-digit account number. The keyword
input
halts all activity until something is entered. If there is input, we clear the error message at the bottom of the screen if one is displayed. It was on the screen a sufficient amount of time. This is necessary for two reasons: first, we need to give the person entering data a chance to look things over; second, it’s impossible to read the screen if the data is erased too fast. This reminds me of one of my college professors who wrote with one hand and erased what he wrote with the other – not my idea of a good teacher. When it comes to important messages at the bottom of the screen, a good practice is to leave the error message on the screen until something is input. We have defined
account-number
as a nine-digit integer and whatever is entered to the right of the literal
account number:
has to be a number from 0 to 999999999. If it is, the account number file can be read. You will note that entry for the account number begins at position 36 in row number 4 but if we had omitted the (4,36), it would start in the position right after the colon following the literal. This mention of the specific row and column allows for a space.
You will not be able to enter letters of the alphabet or any special characters into the input field. If you enter 9 for the account number, you would not need to key the leading zeros, since that one digit would suffice. The next line is a decision, which allows the user to end the program, which is done by entering 0 for the account number. Zero is certainly an integer but not a valid account number. As we have pointed out, account numbers have to be greater than 9. Entering 0 terminates the program and forces a branch to the label
end-program
which ends all activity. The next statement
read acctfile key account-number
should be familiar. The only difference involves the keyword
key.
This statement takes the value that was entered for the account number, and uses it as a key to read the account file into the structure. The statement could be replaced with
read acctfile
since the definition of the file points to the key as
account-number.
The file status is checked as in our original program. Here we have an indexed read. If the read doesn’t find that particular record, the result is a not found condition – status being a 5. If the read is successful, the program will display the values of each field for the record that was read and the user can now enter another account number or exit the program. For invalid account numbers entered, a message will print on line 24 starting in column 20 listing the invalid input. The keyword
else
gives us options so we will either read another record or be at the end of the program. This is another keyword which we have not seen with if statements. We actually cover three cases for the file status, 0, 5 and anything else. The error message
Problem with the account file; program ending – press enter
could result if the file didn’t exist or there was some other problem.
You may ask why we need to restrict the account number input to numeric as the read of the file would take care of that. We could take whatever was entered and try to access the file. If the field keyed is not numeric we would get a not found and that wouldn’t be a problem, except the operator will have to input another account number, slowing down the input process. If the field is numeric, the record desired may be on the file, but maybe not. Each case would be handled. We could allow
character(9)
but then the user would have to key in leading zeros. Being defined as
integer(9)
is a better choice since it saves keying.
If you have just an if statement or a combination of if and else, you only need one
end-if.
We could also have written the code for checking the status as
if acct-status = 0
screen(4,36) account-number
screen(6,36) last-name
screen(8,36) first-name
screen(10,36) middle-initial
screen(12,36) street-address
screen(14,36) city
screen(16,36) state
screen(18,36) zip-code
screen(20,36) balance, mask($$$$,$$9.99-)
go to input-number
end-if
if acct-status = 5
screen(24,20) “The account number ” account-number “ is not on the file.”
go to input-number
end-if
error-msg = “Problem with the account file; program ending – press enter”
and no else statements would have been necessary.
There is one other difference from the first program and that is
balance, mask($$$$,$$9.99-).
The minus sign allows for negative numbers to be displayed. For a negative balance, the sign prints to the right of the second decimal digit. If the amount is positive, no sign will print. The negative sign is printed on the right because we have the dollar sign on the left.
A new field is defined by a new keyword in the line
define error-msg character(60) value “ ”
which says the field is sixty spaces, even though you only see one. This is the same as
define error-msg character(60) value “ ”.
At the end of the program, that error message is
printed. If everything went smoothly, we just print nothing on line 24.
Otherwise, an error message is moved to the message and it will be displayed at
program’s end. The error message will remain on the screen until the operator
presses