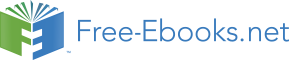

8. Program flow and compiles
So far the two programs that have been discussed have taken an input file and produced output. In each case the input was the account file and the output was a report, even though one was paper and the other was on the screen. We still have to deal with updating a file, but once we do that, we will have covered every possible scenario as to what a computer program can do. We won’t get to the update feature for some time but right now let me summarize the way computer programs process line of code.
A computer program will execute one statement after another until finally it hits the end. In our P language, that happens to be the
end
keyword. It can get to different places because of branches to labels, triggered by
go to
statements. These can be conditional, that is based on an
if
statement, but they could also be unconditional. The latter would be seen if we had a group of print statements followed by a
go to
which did not have any
if
statement preceding it. Consider the following statements:
If file-switch = 0
go to next-step
end-if
go to start-read
screen(1,7) “file switch: ” file-switch
The second
go to
statement is an unconditional branch. Note that the next statement can never be executed because of this
go to
statement. Let’s look at another set of statements:
If file-switch = 0
go to next-step
else
go to start-read
end-if
screen(1,7) “file switch: ” file-switch
The field
file-switch
is interrogated and if it has a value of 0, program control is transferred to the label
next-step.
Otherwise control goes to the label
start-read.
Because of this logic, the next statement can never be reached as the if-then-else statement has forced a branch to one of two places and it will either get to
next-step
or
start-read.
It can never get to the statement following the
end-if.
This line is dead code and there is no reason to leave it in the program. Sometimes people write code this way but the extra line only confuses those looking at the program. On other occasions a program may have been written without any dead code but someone modifies it and in so doing creates these lines of meaningless logic, which can never be executed. Changes were made that forced this scenario and whoever did them should also have deleted the dead code. It’s not necessary to keep it there since it will only take someone longer to read the program and realize that this code is unreachable.
Now consider another group of statements:
If file-switch = 0
go to next-step
else
if file-switch = 1
go to start-read
end-if
end-if
screen(1,7) “file switch: ” file-switch
In this case you will agree that the last line can be executed. This would be true if the field
file-switch
has a value greater than 1. But now suppose that in our program this field will only be 0 or 1. At this point the last line is once again unreachable as before. You couldn’t tell this fact unless you had knowledge of what values the field
file-switch
could be.
Let us consider one more set of statements.
read acctfile
start: if acct-status = 0
print “account number ” account-number
go to start
else
go to end-program
end-if
end-program: end
At first glance this may look similar to what we had earlier and it appears to be a valid set of statements. Note though that we can never get to the label
end-program
if the file has any records because the variable
acct-status
will always be 0. If the file was empty, then and only then would the program end because the status would not be 0 and the branch to the last statement would be taken. If there is at least one record on the file, the status would be 0 and the print would be done and then a branch to the label
start
would take place. At this point, no new record will be read and the field
file-status
would still have a value of 0 and once again we would print out the same account number preceded by the appropriate label.
What we have is an infinite loop, as the program will continue to print out this same account number with the literal
account number:
preceding it. The program would never end unless we somehow interrupted it. The problem is we need to do the read of the file each time we get to the label
start.
A simple change will get us out of this mess. All we need is to move the label up one line to the read statement and then we would eliminate this looping. Thus our statements become:
start: read acctfile
if acct-status = 0
print “account number ” account-number
go to start
else
go to end-program
end-if
end-program: end
and now there is no difficulty and the program will eventually end without us having to interrupt it.
This program could also have been written as
start: read acctfile
if acct-status = 0
print “account number ” account-number
go to start
end-program: end
which I prefer since it’s fewer lines of code.
We don’t want any program to loop indefinitely. You may ask if there is some way to check the lines of the program before we actually run it. There certainly is. There are two possibilities for this. In the P language as in a few others, we write the program and then simply try to run it. If things are in order, all the fields are properly defined, every
if
statement has a matching
end-if,
each left parenthesis is matched by a corresponding right parenthesis and so on, then the program will do what it should. Well it may not do exactly what we want but at least it won’t abend and we will have made some progress.
On the other hand, if something is amiss such as an undefined field, then the program will try to run and pause because of that deficiency. At that point there will be some message indicating more or less what went wrong – at least in most languages. If the warning is not specific enough it may list the line with the problem and you can somehow figure out what’s wrong, change it and continue to run the program. Of course there could be another different problem and once again you would have the same situation. You could correct it and resume the program and eventually get to the end.
Any program that we write is referred to as source code. Whether the running of the program proceeds as above or in one other manner, it will still be a source program. The other possibility is that we will need to compile the program or source code. This process is nothing more than running the program through a compiler to find out if there are any errors such as we mentioned above. If there are problems, we will get a list of the trouble spots and we can change the code and then recompile the program. Once all the errors are cleared up, the compiler will create what is referred to as object code. This is what is actually executed when we run the program, at least if we compile the source code. If you were to try to view some object code it would probably look like obscenities. However, this is what the computer needs to run your program and object code is sometimes called machine code for that reason.
As far as compiling a program, it sounds complicated but it is just running your source code through another computer program to create object code or find basic program problems. With the P language we don’t have to worry about that process but we still need to take care of these errors when we run the program, provided there are oversights in the code. When you get to work with other people in a programming environment and you need to do a compile, you will be given enough information to proceed.
At this point you may think that you are home free if your program compiles and then runs to completion. Maybe so, but there could be logic problems. When you design a program you have a good idea what you want done so you code accordingly. The computer then follows your directions but if you unknowingly have the wrong code, the computer will still do what you tell it but it may not be exactly what you want. This is referred to as a logic error. What you then have to do if you see that the wrong thing is happening is check over the code and see what is causing the difficulty. That may take longer than it took you to write the program, but you need to do it.
So you may have thought that you would have an easy time but there could be problems. Just remember that the computer will do everything you tell it to do but it is up to you to dictate the proper instructions. This means you need to know the rules of the language and how everything proceeds. The clearer your understanding, the fewer difficulties you will have. When the program doesn’t do what you want it to, you have to do some debugging. This is the process of figuring where you went astray.