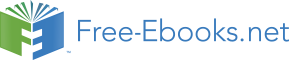

12. Programming standards
Before proceeding, it’s time for a test. It’s actually an exercise to challenge your brain. The problem is this. We have a balance scale and 8 pills that are all the same color. One of the pills is poison and the others are plain aspirin. The poison pill weighs slightly more than the others and can only be discerned by using the scale. How do you positively determine which is the bad pill in exactly two weighings? A weighing is defined as loading a set number of pills on each side of the scale and making a determination. Think about it and I will reveal the answer at the end of the next chapter.
As I have pointed out, we have certain rules in our P language. These have to do with keywords and their meaning, the way we define variables and use them and how we put together keywords and variables to form meaningful statements to the computer. From the sample programs you will note that I indented certain lines but that was not really necessary. For example the statements
if acct-status = 0
go to read-account
else
go to end-program
end-if
could just as well have been written as
if acct-status = 0
go to read-account
else
go to end-program
end-if
and the program would have functioned properly just as with the first set of lines. The indentation is done for readability, so that someone looking at the program can more easily follow the logic of the program. I also mentioned that instead of calling the variable
last-name
for the name of the person corresponding to the account we could just as well have used the variable
x.
It’s just that the latter would give us no clue as to what it represents without further digging into the program. The variable
last-name
is more meaningful.
Earlier I mentioned that one of the first languages I studied in graduate school was APL. In that language you could eventually reduce any program to a single continuous statement. It might extend over one line but one statement would do. If you wrote the program as a series of statements and thoroughly tested it so that you were completely satisfied that it worked and then converted those statements to one single entity, that might be fine. But if you had to come back six months later to change it, or if someone else wrote the program and you had to modify that single statement condensation, I’m sure you wouldn’t be too pleased.
Our goal in programming is to make things understandable and easily modified. I gave some thought to a certain approach to the update program of the last chapter and decided that my original design would be too confusing and involve more variables than I really needed. As a result I came up with a better way of doing things that would be more easily comprehended. It’s true that the other approach would have worked but why not have a design that works and can be both understood easily and modified with few repercussions. Even if you do your best in simplifying a situation, it will still have enough complexity so there is no need to make it more mystifying.
Every programming language has certain rules but there are other guidelines that can be taken to make life easier when we have to make changes to a program. The idea of indentation is one and structured code is another. Some languages or companies have a rule that
go to
statements aren’t allowed. If you ask how you can program without it, there are certain situations that can’t be avoided because of specific keywords and processes. In general you can somehow replace the
go to
with a
perform
statement. I argue against this if the latter is more difficult to understand than the use of the
go to.
First and foremost the goal of any program is simplicity.
Another guideline has to do with repeating lines of code. If you have five statements in a program that occur in two or three different places, why not put them into one procedure and simply perform that procedure when it is needed. This approach will accomplish two things: first, your program will have fewer lines of code and second, if you have to modify those lines, the change will be in a single place rather than two or three. Changing the code this way means that you won’t change the code in one area while forgetting to do it in the other area since it is only in one place. As far as the number of lines of code goes, generally speaking the fewer lines of code you have in a program, the more easily can it be maintained.
I will talk more about guidelines as we progress but for now let us look at more examples of the index keyword. Consider the statements
define x character(8) value “01234567”
define y character
define z character(3)
define answer integer
and the statements
y = “8”
answer = index(y, x)
which will result in
answer
with the value of 0 because the character 8 is not in the string
01234567.
Before proceeding recall that
integer
represents a one position numeric field just as the use of
character
for middle initial in an earlier program involved a single character.
The statements
z = “222”
answer = index(z, x)
will yield a value of 1 for
answer
since each character in the variable
z,
namely the 2 three times, is in the variable
x.
If we have the statements
z = “70”
answer = index(z, x)
note that the result will be 0. Certainly the 7 and the 0 are in the variable
x
but the third character is not. The last character is a space since
z
is defined as 3 characters and the statement
z = “70”
results in
z
consisting of three characters with the last one being a space. Since the space is not found in the string
x,
the result of the
index
statement is 0. If you say that z is only two positions long since it has the value “70”, I need to remind you that previously we had defined z as a field having 3 characters.
What will the statements
z = “70”
answer = index(x, z)
yield? What we are asking is if the string
01234567
can be found in the string
70.
Well the 0 and the 7 can but that’s about it so the result for
answer
is 0.
What will the statements
define x character(8) value “01234567”
define y character value “2”
define z character(3) value “789”
define answer integer
answer = index(index(z, y), x)
yield? First note that
index(z, y)
will be evaluated first and the result will be 0 since none of the characters 7, 8 or 9 can be found in the string
y,
which has a value of 2. Thus the line reduces to
answer = index(“0”, x)
and this is asking if 0 is in the string
x.
It certainly is so the result is that
answer
winds up with the value 1. This is a farfetched example that you probably will never encounter but if you understand it, you have a good grasp of the
index
keyword.
For your assignment, assume your boss likes to get involved in what you’re doing – like constantly looking over your shoulder. This may seem like an outlandish request, but just pretend you’re working in the department of information technology – or whatever it’s called today – at a corporation in America. The manager asks you for this:
Write a program that will allow someone to set up some accounts for the account number file. The user will enter last name, first name, middle initial and an account number will be generated from the system. A report listing all these fields is required. Later, someone else can key in the other data for these file records.
As you can see, the specifications aren’t very detailed, but at least they’re not written on toilet paper. If so, I’d refuse the request if my manager believed in recycling. The solution will be presented in a later chapter and illustrates what has to be done to add records – in some small way, you can figure out the rest – to a file.