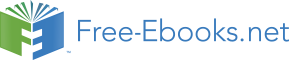

11. Updating fields
Before we proceed into updating fields in the account file, let us talk about some possibilities. We could create a second program that could be used for updating the fields or we could incorporate the options into the inquiry program. The advantage of two programs is that we would keep each process separate and thus the logic for each program would be less complex. On the other hand, many of the same processes will be in each program so perhaps a single program would be better. With the latter option, all the code would be in one place, which means that if changes have to be made they would be done in a single place. If we had two programs then we could miss changing one when there were changes to the other.
Either having one or two programs is an acceptable choice. Obviously any system should be designed to make the person using it as comfortable as possible and it should be able to be easily maintained. The choice is not an easy one and having both user-friendly and easily maintained systems may not always be possible. One feature may have to sacrificed for the other and much thought needs to go into how things will be handled.
In our system, account numbers will be generated by the computer. They could have been created by people at the computer as they entered all the fields. The reason for using the machine and not people is because this approach will be easier for the user insofar as the computer will have knowledge of what numbers are available, as they are needed. If ten people are entering data at ten different places for the account file and need to put in a new account number, they may try one only to find that that value has already been used. This effort could result in the person inputting information getting delayed because he cannot find an unused account number.
What this choice means is that to accommodate this decision, we will have to spend more time developing our program. There will be more work for the programmers, but this will be well appreciated by the users. Since we do have computers, there’s no sense in not taking advantage of their power. In the process we should make every effort to guarantee that our systems are maintained as easily as possible. We will talk more about the assignment of account numbers at a later time.
Using a single program, our old inquiry program will now give us the ability to change the values of certain fields on the account file. For now, there will be no update of the account number field because this would equate to a delete of a record and an add of a new one. Only the fields last name, first name and balance will be allowed to be changed for discussion purposes. The other remaining fields could be changed in the same manner so there is no need to show all of them in this program. You have to do it yourself but you will get the idea from these three fields alone.
The beginning will not change as the user will enter the account number and the account file will be checked to see if it exists. Entering a 0 will enable the user to exit. This is the same as in our original inquiry program. Assuming the record exists, the fields on the record will be displayed along with an additional message at the bottom of the screen and there will be numbers to the left of the three fields that can be changed. In the event that no changes are to be made, the operator can enter a 0 and now another account number can be entered. All three fields can be modified or as few as one, but once a change is made the account file will have to be reread in update mode to see if anyone else has that record. If so, the record cannot be updated at this time. If the record is available, the program will then write the account record with all the changes. Whether this is done or the record is busy, the program will then return so that another account number can be keyed.
This doesn’t sound too difficult but it is more complex than described here. To begin with, we don’t want someone to enter characters into either name that are not allowed there, such as $ or %. Thus we should try to eliminate this possibility and warn the operator of this error with some kind of message. Fortunately by defining the account balance as
signed decimal
we won’t have to worry about what is keyed since any character that is not acceptable as this type of field will not be allowed by the input statement. We can check for certain characters in either name, but the user could enter “h-o-s-e-r-s” and that would be fine, with no objection. We will assume that the operator knows what he is doing. In many companies, that belief can be dangerous.
Most of the inquiry / update program will look familiar but there will be a few new keywords and some of the logic may be troubling or puzzling or both. After going through it, you should have a good grasp of what an update is all about. Assuming the operator enters a valid account number of
391842091,
the new screen will look like the following:
Account number inquiry / update
Account number: 391842091
1. last name: Smith
2. first name: John
middle initial: L
street address: 3910 Main Street
city: East Aurora
state: NY
zip code: 14052
3. account balance: $27.89
key 1 (last name), 2 (first name), 3 (balance) or 0 (next)
To change the first name, enter 1. To update last name, enter 2 and enter 3 to update the account balance. Entering 0 will give you the opportunity to enter another account number. The new program will now turn into the following:
program-name: acctupd
define file acctfile record account-record status acct-status key account-number structure
account-number integer(9)
last-name character(18)
first-name character(15)
middle-initial character
street-address character(25)
city character(15)
state character(2)
zip-code integer(5)
balance signed decimal(6.2)
define name-string structure
field character(26) value “ABCDEFGHIJKLMNOPQRSTUVWXYZ”
field character(30) value “abcdefghijklmnopqrstuvwxyz ‘-.”
define field-no integer
define valid-input integer
define update-switch integer
define new-last-name character(18)
define new-first-name character(15)
define new-balance signed decimal(6.2)
define error-msg character(60) value spaces
screen erase
screen(1,25) “Account number inquiry / update”
screen(3,20) “account number:”
screen(5,17) “1. last name:”
screen(7,17) “2. first name:”
screen(9,20) “middle initial:”
screen(11,20) “street address:”
screen(13,20) “city:”
screen(15,20) “state:”
screen(17,20) “zip code:”
screen(19,17) “3. balance:”
input-number: screen(21,1) erase
screen(21,1) “enter the account number or 0 to exit”
screen(3,36) input account-number
screen(24,1) erase
if account-number = 0
go to end-program
end-if
read acctfile
if acct-status = 0
screen(3,36) account-number
screen5,36) last-name
screen(7,36) first-name
screen(9,36) middle-initial
screen(11,36) street-address
screen(13,36) city
screen(15,36) state
screen(17,36) zip-code
screen(19,36) balance, mask($$$$,$$9.99-)
new-last-name = last-name
new-first-name = first-name
new-balance = balance
update-switch = 0
field-no = 9
perform get-change until field-no = 0
go to input-number
else
if acct-status = 5
screen(24,20) “account number “ account-number “ is not on the file.”
go to input-number
else
error-msg = “account file read problem; program ending – press enter”
go to end-program
end-if
end-if
get-change: screen(21,1) erase “key 1 (last name), 2 (first name), 3 (balance) or 0 (next)”
screen(21,60) input field-no
screen(24,1) erase
valid-input = 0
if field-no = 1
perform input-last-name until valid-input = 1
else
if field-no = 2
perform input-first-name until valid-input = 1
else
if field-no = 3
screen(19,36) input new-balance
update-switch =1
else
if field-no = 0
perform update-check
else
screen(24,20) field-no “ is invalid”
end-if
end-if
end-if
end-if
update-check: if update-switch = 1
read acctfile update
if acct-status = 3
screen(24,20) “that record is unavailable – no update done”
else
if acct-status > 0
error-msg = “update problem; program ending – press enter”
go to end-program
else
balance = new-balance
first-name = new-first-name
last-name = new-last-name
write acctfile
if acct-status > 0
error-msg = “update problem; program ending –
press enter”
go to end-program
end-if
end-if
end-if
end-if
input-last-name: screen(5,36) input new-last-name
screen(24,1) erase
if index(new-last-name, name-string) = 1
valid-input = 1
update-switch = 1
else
screen(24,20) “invalid characters in the last name – try again”
end-if
input-first-name: screen(7,36) input new-first-name
screen(24,1) erase
if index(new-first-name, name-string) = 1
valid-input = 1
update-switch = 1
else
screen(24,20) “invalid characters in the first name – try again”
end-if
end-program: screen(24,20) error-msg input
end
To start with, we have the new variables,
field-no,
new-last-name,
new-first-name,
new-balance,
update-switch,
valid-input,
and the structure
name-string.
To indicate which of the three fields will be changed, we need