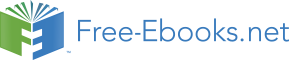

14. Programming creativity
That last challenge with the pills was meant for two purposes: first to keep the creative juices flowing and second to point out the fact that sometimes the normal way of doing things may not always work. We may have to find a different way of accomplishing a task. Even as we progress from day to day you will note that methods of getting a task done on the computer will change from what they were. It’s true that the technique of yesterday may still work today, but it may be beneficial to improve on the method just as the technology itself has been improved.
The obvious reference here is to the days when we had more limits on what we could do as computer programmers. We may have had space limitations, which forced us to think in a different way, or there may have been a limit on the number of variables in a program or the size of a table, which meant that the approach we thought about couldn’t be used. We would have to come up with an alternative method. A particular situation could be handled in two or three ways but perhaps only one of these would allow us to get certain tasks finished in a required timeframe. Many of these early restrictions are gone now, but some are still with us.
I recall a specific instance when I was working on a modification to a program to add checking for a new transaction. Unfortunately the software wouldn’t let me do this by increasing the table to accommodate the additional three characters. It seems there was a limit on the size of this field and it had been reached. Fortunately there was no repercussion if I added more lines of code, but there could have been. The way the program worked before with this table was that it started at some position in the string of values and obtained that number and the next two. If the string was
001002003004,
a pointer would start at position 1 for a length of 3, with the result being
001.
This value would represent a transaction number. We could then bump up the pointer by 3 to get 4 and then take the string starting at position 4 for a length of 3 and the result would be
002.
These three position fields would represent transaction numbers and by looping through the values in the same fashion, we could check to see if the three digit number we had was a valid transaction.
My task was to add another three-digit number that represented a new transaction to this string. Unfortunately the limit on the size of this variable had been reached. Since my new transaction was
010,
what I did was to add a slight modification to the program that checked the value of the pointer and if it was 1, I would only increase it by 1 rather than by 3. Then from that point in the string if I took the next three digits I would get
010,
which would account for the new transaction possibility. I would then check the pointer and if it was 2, I would increase it by 2 and then proceed. In this way I would still have available all the possible values I had originally as well as the
010.
Thus I added logic but didn’t have to increase the size of the variable, which I couldn’t do anyway.
I talked about adding a read to the zip code file but also mentioned that the added read of the new file could result in a slightly longer run time. This could be remedied by storing the city and state on the account file even though it could also be found on the zip code file. If this still caused a problem because of the lack of space, I suggested putting the zip code data into a more easily available table, which would mean less time needed for file access since you wouldn’t have to read the zip code file, and less space required on the account file since you wouldn’t have to store city and state on it. In making any of these decisions, you need a good knowledge of the capabilities of your system as well as what your options are.
You will run into challenges of all sorts despite the improvements of computers in what they can do and how fast they can do it. No matter what you do, you may not be able to speed up some process, especially if it depends on reading a file. You will have to make some decisions such as getting rid of old records by moving them to a history file and that could save you some time. This would mean less file access and it might save the day for you. Another consideration may be to change the way the file is accessed. This may not work so you will have to come upon another solution. Despite the speed of today’s computers, it still will take some time if you have to read through 10,000,000 records.
Some time ago I added logic to a program to do some type of string manipulation using some keyword. I forgot what it was or involved but I do remember that it took a great deal more time than I wanted it to despite the fact that it worked. I spent some time thinking about it and tried another method to get the same results. It also worked and fortunately only took a fraction of the time of the initial approach. You will run into situations just like these and your brain will be challenged.
As you know, there are many different ways in the world of PCs to accomplish a given task. That is true in programming, as I have already pointed out. The question might be what is the best way to accomplish a specific task? Obviously if you can do it by a simple process and keep the user happy as well as provide a program that is easily maintained, you have found the best way to get the job done. As you might guess, all three of these may not always be done together. So if you have a chance to provide someone who requested a program more than she wanted and at the same time the program can be easily maintained as well as not that much of a headache in producing, all the better. Intelligence and consideration are necessary.
Recall not too long ago I mentioned that the update program would not allow for changing an account number as that would be done by a deletion and an add. Since people close accounts out every so often, we will still need to be able to delete an account. Once we have added that functionality along with that of adding an account, which we shall get to shortly, we will have all we need to maintain the account file. Different circumstances in other systems may require the ability to change an account number, and you will encounter these challenges in your assignments.
As far as the account number goes, you may wonder how the system generates it. One way could be to generate a random nine-digit number and then read the account file. If the number created was not on the file we would then have a new account number to use. Initially this process may be all right but once the account file had a few records, it just might take a few random generations and corresponding reads of the file to find a free number. The process of generating a nine-digit number takes no time at all but reading the file a few times would.
Though this may work, we will use a different approach and eliminate some of the file accesses. We will still have a file to read but at most we will only read one record because our file will only have one record in it. That record will turn out to have the next available account number. What will happen is we will read this file and take the value on it for our account number and then 1 will be added to that number. We will then write the record. If someone else needs an account number, they will read this same file and get a number and the process will be repeated. Since each read of this file will be in update mode, the record will be locked so no one else can get it until we are through. The record they read will then have another account number and duplication should be eliminated.
What kind of problems could arise using this method? Suppose someone got into the program to add a record, got a new account number and then decided they didn’t want to have that record at all. Once in the program we may not give them the chance to delete the record but there would be another program for deleting closed or unwanted accounts. Even if we allowed the deletion in the add program, you can see that eventually we would have gaps in the file. That is to say, there would be numbers in the sequence that would not correspond to a valid account number. This may not be a problem at all as we have almost a billion numbers to choose from. However, if there were many deletions and the account number was only 6 digits and we had more customers that we anticipated, the possibility exists that we could run out of valid account numbers.
To get around this potential problem you could solve it by writing another record to the next available account number file every time a delete was done. This number could then be reused. The process would involve more complications in the logic as far as accessing the file for this number and it would cause problems if we moved the deleted account to a history file. This would result in two people having the same account number. Our apparent solution appears to be creating more problems than it is solving. Perhaps a much better and certainly simpler solution is to increase the size of the account number. Since our file will allow for almost a billion customers, I don’t think we will have a problem and we need not worry about recapturing deleted account numbers. As you can tell, making this decision requires some thought but in the long run the effort will pay off in fewer problems and less work.
Speaking of computer limits, Jerry Weinberg, our teacher in Binghamton, asked us to write a program to solve the traveling salesman problem. Starting at a home point, visiting one hundred places and returning home, we had to arrive at the route that would involve the shortest total distance covered.
My project mates and I figured we’d use the computer to look at all possible combinations, calculate the distances between points, two at a time – using geometric formulas – add them and arrive at the solution. It sounds like a murderous job, but we weren’t going to do it – the computer was. We’d start with one hundred random points on a graph, using A0 for the home point and A1, A2, A3, etc. up to A100 for the places to cover. The distance between A0 (x0, y0) and A1 (x1, y1) is the square root of the sum of (x1-x0)2 + (y1-y0)2. If you played hooky the day, we were taught that in math class, trust me. A similar calculation had to be done for all the remaining combinations.
For the number of combinations, just consider traveling to five places. There are five choices for the first location, four left for the second, then three, two and of course, one left. Using the asterisk to indicate multiplication, this becomes 5*4*3*2*1, which equals 120 possibilities. If we have one hundred places but consider only twenty, we have one hundred choices for the first location, then ninety-nine, ninety-eight on down, or
100*99*98*97*96*95*94*93*92*91*90*89*88*87*86*85*84*83*82*81. I’m not going to waste my time calculating that number, but instead use an approximation of 10020, which is larger than the value worked out, but since we still have eighty more locations, it should suffice, actually being a low estimate. The number, 10020 equals 1040, since 100 equals 102. Don’t tell me you cut math again.
According to a 2008 New York Times story, a super computer could perform 1.026 quadrillion calculations per second. This number is approximately 1015. In a year, there are 60*60*24*365, or 31,536,000 seconds. For a thousand years we have 31,536,000,000 seconds. Multiplying these two numbers (approximately 1015 calculations per second times about 1012) would result in fewer than 1027 calculations for a thousand years. Compare this to 1040. Since we’re only considering a fraction of the one hundred locations, we’re going to come up way short – by a long shot. We’ll need a faster computer – much, much faster. I think Jerry tricked us.
Indeed, a computer can’t do everything. It has limitations. This effects business applications as well. Assume that a business needs to shut down the online system for a short time to do some updating of files, say a half hour. If that process takes twenty minutes, that’s cutting it close. In today’s crazy, stressed out environment of 24/7, how can the corporation even afford to shut down for fifteen minutes? Perhaps the solution is to handle the updates without any shutdown. It will have to be figured out.
Speaking of solutions, specifically the traveling salesman problem, what if I hire ten go-getters to cover the one hundred locations, ten each. I implore that to really hustle for mucho dollars. I turn the dough over to my boss and he is overwhelmed and pleased, completely forgetting about the problem originally assigned.