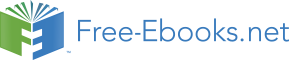

15. Adding records and calling a program
Now it’s time to discuss that program which I asked you to write a few pages ago. It will illustrate adding records to the account number file as well as calling a program to obtain a new account number. The calling program will have the operator enter last name, first name and middle initial. Once all three are entered and valid, an account number will be generated by the system, through the called program,
nextnumb,
which passes back the new account number to the calling program. The called program will read a new file called the next number file, which is defined with the key of account number and set up by the data base analyst. It consists of an indicator, which will be a blank or d, and the next available account number. Besides the next account number, there will also be records with account numbers that are available due to the deletion of records from the account number file.
Before any records exist on the account file, the next number file will have one record and the key or next available account number will be 10. If there are yet to be deletions to the account number file and the file has twenty records, the next number file will have one record with the key of 30, with the indicator equal to a space. At this point if someone deleted the record with account number equal to 15, the next number file would have two records, looking like this:
Key indicator
000000015 d
000000030
The called program giving us the next account number will be included in the next chapter. The procedure to add an account will be accomplished when the three data fields are correctly entered. To exit this program, the user should enter xx for the last name. The lines of the calling program follow.
program-name: acctcall
define main-heading structure
print-month character(2)
field character value “/”
print-day character(2)
field character value “/”
print-year character(42)
field character(74) value “New account number addition report”
field character(5) value “Page”
page-number integer(3)
define sub-heading structure
field character(54) value “account # last name first name”
field character(57) value “mi ”
define print-line structure
field character(18) value spaces
print-account-number integer(9)
field character(17) value spaces
print-last-name character(18)
field character(17) value spaces
print-first-name character(15)
field character(17) value spaces
print-middle-initial character
define total-line structure
field character(43) value spaces
field character(42) value “The number of account records added was”
print-count integer(3) mask(zz9)
define file acctfile record account-record status acct-status key account-number structure
account-number integer(9)
last-name character(18)
first-name character(15)
middle-initial character
field1 character(42)
zip-code integer(5)
balance signed decimal(6.2)
define data-fields structure
new-account-number integer(9) value 0
process-sw integer value 0
define error-msg character(60) value spaces
define in-last-name character(18)
define in-first-name character(15)
define in-middle-initial character
define name-string structure
field character(26) value “ABCDEFGHIJKLMNOPQRSTUVWXYZ”
field character(30) value “abcdefghijklmnopqrstuvwxyz ‘-.”
define work-date character(8)
define record-counter integer(5) value 0
define page-counter integer(3) value 0
define line-counter integer(2) value 54
work-date = date
print-month = work-date(5:2)
print-day = work-date(7:2)
print-year = work-date(3:2)
screen erase
screen(1,23) “new account number addition program”
screen(5,20) “account number:”
screen(10,20) “last name:”
screen(15,20) “first name:”
screen(20,20) “middle initial:”
screen(22,36) “enter xx for last name to exit”
input-data: valid-input = 0
perform input-last-name until valid-input = 1
valid-input = 0
perform input-first-name until valid-input = 1
valid-input = 0
perform input-middle-initial until valid-input = 1
perform call-process
go to input-data
input-last-name: screen(10,36) input in-last-name
screen(24,1) erase
if in-last-name = “xx”
go to end-program
end-if
if index(in-last-name, name-string) = 1
valid-input = 1
else
screen(24,20) “invalid characters in the last name – try again”
end-if
input-first-name: screen(15,36) input in-first-name
screen(24,1) erase
if index(in-first-name, name-string) = 1
valid-input = 1
else
screen(24,20) “invalid characters in the first name – try again”
end-if
input-middle-initial: screen(20,36) input in-middle-initial
screen(24,1) erase
if in-middle-initial = space or (>= “A” and <= “Z”) or (>= “a” and <= “z”)
valid-input = 1
else
screen(24,20) “invalid character in the middle initial – try again”
end-if
call-process: call nextnumb using data-fields
if process-sw = 0
account-number = new-account-number
last-name = in-last-name
first-name = in-first-name
middle-initial = in-middle-initial
field1 = spaces
zip-code = 0
balance = 0
perform write-record
else
if process-sw = 3
screen(24,1) “next number file is busy – try again later”
else
error-msg = “next number file error; program ending – press enter”
go to end-program
end-if
write-record: write acctfile
if acct-status = 0
record-counter = record-counter + 1
line-counter = line-counter + 1
if line-counter > 54
perform print-headings
end-if
print print-line
screen(10,36) last-name
screen(15,36) first-name
screen(20,36) middle-initial
screen(5,36) account-number
else
error-msg = “account number file problem; program – press enter”
go to end-program
end-if
print-headings: page-counter = page-counter + 1
page-number = page-counter
line-counter = 5
print page main-heading
print skip(2) sub-heading
print skip
end-program: print-count = record-counter
print skip(2) total-line
print skip error-msg
screen(24,20) error-msg input
end
The program is quite long but only a few lines should be unfamiliar. Note that the report will only display four fields, so we spaced it out across the page. We spread out the fields on the screen rather than bunching them all at the top. Whoever is inputting data will only key in last name, first name and the middle initial. Obviously, we have to check that they don’t key garbage so we use the same procedures for inputting the first two fields, which has a few checks. For verifying that the middle initial is valid, we use the line
if in-middle-initial = space or (>= “A” and <= “Z”) or (>= “a” and <= “z”),
which is a compound logical statement. It just checks to see if what was entered was either a blank or a letter of the alphabet, upper or lower case. The check
(>= “A” and <= “Z”)
will be satisfied if any of the letters from A to Z are entered and the second part of that check
(>= “a” and <= “z”),
you should be able to figure out. Both sets of
parentheses are needed here because we want the input to be between A and