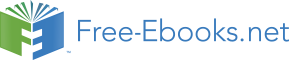

16. The called program and using
As already mentioned, the called program will have a new file called the next number file, which is defined with the key of account number and set up by the data base analyst. It consists of an indicator, which will be a blank or d, and the next available account number. Besides the next account number, there will also be records with account numbers that are available due to the deletion of records from the account number file. Once an account number is ready to be sent to the calling program, the next number file will be updated.
Even though the next number file is a keyed file, we will read it sequentially so we always read the first record on the file, taking the first available number and using it until the next number file eventually winds up with one record again. If there are many deletions, this may take a long time but at some point we should wind up with that single record. In any event, our access to get a new account number will work. In the previous chapter, I described what the called program will be doing, so I won’t repeat it here. The called program follows.
program-name: nextnumb link data-fields
define data-fields structure
new-account-number integer(9) value 0
process-sw integer value 0
define file numbfile record next-record status numb-status key account-number structure
account-number integer(9)
indicator character
account-number = 0
readnext numbfile update
if numb-status = 0
perform update-number
else
if numb-status = 3
process-sw = 3
else
process-sw = 9
end-if
end-if
go to end-program
update-number: process-sw = 0
new-account-number = account-number
delete numbfile
if numb-status > 0
process-sw = 9
else
if indicator = space
account-number = account-number + 1
indicator = space
write numbfile
if numb-status > 0
process-sw = 9
end-if
end-if
end-if
end-program: end
Notice that the program name is
nextnumb,
which appears to be a relatively simple program – for a change. The line
program-name: nextnumb link data-fields
has a new keyword,
link,
which enables the passing of data from the calling to the called program. We used the same data name, but they could be different variables as long as the size and characteristics are the same. When the calling program transferred control here, the field
data-fields
had a value of 0 for each field.
data-fields
will be used to pass new values for those fields back to whatever program calls it. Next we define the records on the next number file. It has only two fields, the account number and the indicator. Just as the calling program transferred data, as trivial as it was, we need to pass back data to that program, namely the new account number and a record status. We use
define data-fields structure
new-account-number integer(9) value 0
process-sw integer value 0.
The field
process-sw
will be 0 for a successful process of the next number file. This entire process involves reading the first record there, moving the new account number to the field
new-account-number,
deleting the record just read and adding a new one, unless the account number moved had been used before. If the next number file is busy, 3 will be returned in
process-sw
and if there’s a problem with the read, write or delete, a 9 will be passed back. In this case the new account number returned to the calling program will not be used. The new keyword
delete
will only delete the current record, the one we just read with the update to lock it out from anyone else. That’s the very first record on the file. It won’t delete the whole file, which would really be asking for trouble.
The rest of the program should be familiar but a few comments are in order. We want the first record on the next number file, so we do a sequential read of the first record whose key is larger than 0. That has to be the first record since any account numbers that were used before would appear on the file before the record with a space in the indicator. In the file, all the records are in ascending order in the file.
account-number
was obtained from the read of the next number file because of the
structure
keyword and we’ll use it to make sure we delete the correct record.
For any status we return in the field
process-sw,
other than 0, no error message will be displayed on the screen. That will happen in the calling program since we want people to see it. The updating depends on the indicator. If the indicator is d, we’ll merely delete the record. Otherwise we will delete the record and write another after incrementing the account number by 1. The latter situation means we actually read the last record in the file and so we need to delete it and add another record, which will now be the new last record.
I mentioned a new keyword that allows for a short pause, so let’s check it out. The statements
if inquiry-number = 9
screen(24,20) “Problem with the next number file – program ending”
pause 10
go to end-program
else
end-program: end
use the new keyword
pause,
which will delay so that the person at the screen can read the error message. The number after the
pause
represents the time in seconds. The line
pause 1
will only give a break of one second and that may not be enough. Of course, ten seconds may not be significant either if the operator is daydreaming.
Suppose that we didn’t have this keyword. We could put logic into our program to accomplish the same result. Assuming that our computer does one million operations per second, the following code would achieve the same delay as before:
define pause-counter integer(8)
pause-counter = 0
perform pause-loop until pause-counter = 10000000
where the procedure
pause-loop
would be
pause-loop: pause-counter = pause-counter + 1
which involves ten million additions. You could put these few lines in your program and then time the delay. If it still wasn’t enough or if it was longer than 10 seconds, you could change it accordingly by modifying the number of the additions. Another possibility is to add the keyword
blink,
which results in the message blinking.
Any of these choices has the possibility of problems – mostly the person keying data not paying attention or falling asleep from too big a lunch. People from outside organizations may be upset if somehow an electric shock is set up to be transmitted to the person keying data, so that’s not an option. If the error message is being sent from a called program, it’s a better idea to just transmit some value for a variable – as we did here – to eventually get some error message on the screen of the calling program. As you can guess, I’m not about to use the
pause
keyword since the approach of clearing the error message after the data is entered through the
input
keyword is a better idea.
Up until now, instruction was provided to accomplish certain results. Heuristic learning is another method of education that involves learning by making mistakes. Doing things the proper way may be fine until someone flounders and does it incorrectly. Had he done it wrong to begin with, he would only have made the mistake once. A computer can utilize this method to program the game of chess. I’m glad I don’t have to do it, but each series of moves – by both the computer and its opponent – is filed and when a move results in a defeat of the computer, the exact sequence is noted and the losing move is never repeated. This happens for every game. You can see that eventually, the computer won’t lose. Please note the word in italics – it might take a long, long time.
The way chess and Jeopardy are computer programmed is done by methods that involve specific strategies. This means the system will work in the programmer’s lifetime, even if the computer loses every so often. What this boils down to is when you play against the computer, you’re rally tangling with the person who designed the strategy. Naturally, that person has the advantage of numerous calculations done for him by a machine that a human just couldn’t manage so quickly. The strategies involve looking ahead a few moves and proceeding from there.
I programmed two mind games – you may have heard of both – but I’m not sure what language either was in. That’s not important. The first was JOTTO, a word game where you have to guess your opponent’s five-letter word before he guesses yours. This is done by offering other five-letter words with a response of from 0 to 5, depending on the jots, or common letters in the secret word and the word guessed. If no letters are in common, the response is 0. If one is, the response is 1. For multiple occurrences of a letter, it’s a one-to-one match. For example, if the secret word happens to be eerie, guesses of means and level would result in 1 and 2 jots respectively. JOTTO uses a dictionary of about 1800 words. I had two separate games, one in which you guessed the computer’s word and the other had the machine guessing yours – no cheating, please. The former wasn’t too exciting but the other is the one I’ll concentrate on here. In that approach, the computer could usually figure out the player’s word in eight guesses or less.
My strategy aimed at reducing the size of matrix of words as quickly as possible by eliminating any word that couldn’t possibly be the secret word. If the computer guessed a word with no letters in common, any word having any of those five letters would be discarded. If 3 was the response, any word without all three of those letters word would be tossed away as well. At the beginning of guessing, I had four words that the computer could choose from – all having different letters – hoping for a few 0 replies. This would really shrink the matrix of words. As you can imagine, it was a great strategy.
The other game was Score Four, three-dimensional Tic-Tac-Toe – almost. The more common game is 3 by 3, but this is 4 by 4 by 4, except that either player only had a maximum of 16 moves at any given time. There were 64 beads with holes so that they could be plopped down on 16 different thin poles, always dropping down as far as possible. Each pole held 4 beads and you could win the game by 4 in a row (length, width or height) or diagonally. The latter had 20 possibilities – you had to be on your guard. The strategy I used for the computer was 1) look for any move that produced a win and if there, end the game 2) look for any move that needed to be made to avoid losing on the opponent’s next move and take action and 3) make some offensive move. The program was quite good at suddenly coming up with victory. It didn’t lose very often. In either game, I avoided heuristic strategies.