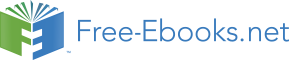

24. A program in action
We spent a great deal of time on concepts of programming. Before continuing, let’s present an easier way to sort a file than by the bubble sort of the last chapter. In this case, we want to sort the account number file in ascending order by zip code, then by last name, first name and middle initial, producing a report with all those fields and the account number.
program-name: sortedlist
define main-heading structure
print-month character(2)
field character value “/”
print-day character(2)
field character value “/”
print-year character(42)
field character(74) value “Sorted account number file report”
field character(5) value “Page”
page-number integer(3)
define sub-heading structure
field character(13) value spaces
field character(19) value “zip code”
field character(32) value “last name”
field character(29) value “first name”
field character(15) value “initial”
field character(22) value “account number”
define print-line structure
field character(13) value spaces
print-zip-code integer(5)
field character(14) value spaces
print-last-name character(18)
field character(14) value spaces
print-first-name character(15)
field character(14) value spaces
print-middle-initial character
field character(14) value spaces
print-account-number integer(9)
define file acctfile record account-record status acct-status structure
field character(100)
define file sortfile record sorted-record status sort-status key account-number structure
account-number integer(9)
last-name character(18)
first-name character(15)
middle-initial character
field character(42)
zip-code integer(5)
field character(10)
define work-date character(8)
define record-counter integer(5) value 0
define page-counter integer(3) value 0
define line-counter integer(2) value 54
define error-msg character(60) value spaces
work-date = date
print-month = work-date(5:2)
print-day = work-date(7:2)
print-year = work-date(3:2)
sort acctfile ascending zip-code last-name first-name middle-initial into sorted-record
if sort-status > 0
error-msg = “sort problem – program ending”
go to end-program
end-if
account-number = 0
read-file: readnext sortfile
if sort-status = 0
record-counter = record-counter + 1
print-account-number = account-number
print-last-name = last-name
print-first-name = first-name
print-middle-initial = middle-initial
print-zip-code = zip-code
line-counter = line-counter + 1
if line-counter > 54
perform print-headings
end-if
print print-line
go to read-file
else
if acct-status not = 9
error-msg = “There was a problem with the account file – program ending”
end-if
end-if
go to end-program
print-headings: page-counter = page-counter + 1
page-number = page-counter
line-counter = 5
print page main-heading
print skip(2) sub-heading
print skip
end-program: print skip(2)
print error-msg
end
You will note that this is a great deal easier than the bubble sort records we used in the last chapter. I also made a few other modifications to handle sort or read problems. A new keyword here is what you might expect,
sort.
You might say it magically sorts the account number file in ascending order by the four fields, zip code, last name, first name and middle initial – in that order – into a new file,
sortfile.
Each of these two lines,
define file acctfile record account-record status acct-status structure
and
define file sortfile record sorted-record status sort-status key account-number structure
spells out the record layout, status and structure, enabling the
sort
to work in the proper manner. The keyword,
ascending
leaves the records sorted from lowest order to highest by those four fields in the way requested. To obtain the data starting from the bottom of the alphabet, you would use the keyword,
descending.
From the sorted file, we just produce the report as before. Since there could be problems with the sort or the read, we have provided for each of those, which you can see at the sort and read procedures respectively. If there is a problem with the printing of the report, it’s time for a new computer. Are you glad that we don’t need that bubble sort?
Now let us see how we can take advantage of these ideas in the real world. We deal with a program or two that can be helpful in ordering goods in the grocery store, with little intervention on the part of any human being. We will not actually do the programming but merely indicate what could be done to have orders for stock automatically placed.
The front of the store already has an automated system for pricing of groceries and producing totals for the customer to pay for the goods purchased. This involves scanning the product to get a price for the item. The system works well but we shall now add an online program to the process. This will trigger ordering of cases of soup, pickles, cereal or whatever else is needed to guarantee that we don’t run out of stock for a particular product. To illustrate what will be done, I will deal with one product alone, dill pickle relish. Of course the procedure will work for any item in the store.
When the jar of relish is scanned at the register, besides the normal routine, an online program will be running which will add 1 (or 2 if that many jars of the relish are purchased) to a counter. This counter represents the number of jars of dill pickle relish that could be added to completely fill the space on the shelf that this product occupies. Besides this counter, the product code for this relish has a few other fields in the record on a file that we need. One is the number of jars in a case of dill pickle relish. Another is the number of jars that will fit on the shelf in the store in the assigned area in the pickle aisle.
There could be other fields as well such as the description of the product. However, I think you will agree that we really don’t need that specific field to accomplish what needs to be done regarding ordering. For argument sake let us say that one case of relish holds 12 jars and the shelf has room for 30 jars of the condiment. If the counter is currently at 11, when one jar of dill relish is scanned at any checkout, the counter will be incremented to 12 and this will cause an action to be taken which will update an order file and the counter reset. One case of this relish can be ordered, so it is.
The order file will be an indexed file with the record key being the product code. At the time when that twelfth jar is scanned – triggering an order for another case of relish – the order file will be read for that specific code and if a record is found, that meant that at least one case of relish was ready to be ordered. Now another one is needed, so the number of cases will be bumped up by 1. That order record will be rewritten. A read of the order file resulting in no record being found means that only one case is ordered.
This will work for every product in the store and as any item is scanned, we may not write out any record to the order file but at least we will increment the counter on the file for that product. Eventually this file of orders will be transmitted to the warehouse and that probably will happen at the end of the day or maybe early the next morning. Of course it could happen twice a day or maybe only every other day. Once the transmission takes place, the order file will be cleared since we don’t need to have it duplicated. Before the order is transmitted, it will be backed up. This is done just in case there is a problem with the sending of the file to the warehouse.
You might wonder about the case where a customer somehow knocks a bottle of relish off the shelf and it winds up all over the floor of the pickle aisle. In that case the item will not be put through the scanner at one of the front registers but there will be one less bottle of relish on the shelf. This scenario could mess up the whole order process as a case of stock could fit on the shelf but the counter for that product would be at one less than the number of items in a case. To remedy this problem, we will have a scanner in the back room just for damaged goods. Some grocery clerk will have to get a bottle of the relish off the shelf and run it through the scanner for every broken bottle of relish. He or she also has to eventually put that jar back.
This will solve the reconciliation problem and at the end of the day you may even get a printout of all the damage for the day as well as the amount of the loss. But there is another potential problem having to do with shoplifting. If someone swipes one bottle of relish and it winds up in the car without going through the scanner up front, we have the same problem but no apparent way of reconciling it. Fortunately there is technology available where a product will not be able to get out the door without sounding an alarm. This could be what we need for stopping thieves. Another option is to have the ability to have an explosion triggered by the bottle leaving the store without being scanned. That option involves cleanup, however, and is not practical.
We may also find another solution to shoplifting so as to not mess up our ordering system but what about in-store thieves? Specifically what I am referring to is the bag of chips, which accidentally winds up as damaged in order to satisfy the salt cravings of the stock clerks. One rule of thumb is that a product found on the wrong shelf is fair game for starving grocery store workers. Maybe you’ve heard of it. This shouldn’t happen to a bottle of relish. Once again someone is going to have to run the bag of chips through the backroom scanner. In this way appetites will be satiated and we don’t have to worry about messing up the count. I am sure there are other scenarios that need consideration but there are probably ways to solve them as well.
Thus all the stock in the store can be ordered through the computer although it will still take manual intervention to fill the order at the warehouse, deliver it, unload it at the supermarket and put it on the shelf. Certain tasks will be eliminated but some will remain. In addition the system of keeping track of the stock can be used in a myriad of other ways. We can track how many cases of relish sell in a day, week, month, or during a specific season. When I worked in a supermarket I ordered stock for the glass aisle, which held soups, pickles and relishes. Now you know why I talked about dill pickle relish. At that time, we had averages for each product indicating how much would sell in a week so that we could order accordingly. So if the shelf held 3 cases of a particular item but 4 cases would be sold on an average week, we would order the latter amount for the week.
With our system there is no need to do that, as the system will order as needed. I recall my ordering days when the grocery manager used to order and we wound up with plenty of overstock in the back room. Apparently he used to dream of scenarios where the consumer would buy more than the average for some item. Obviously that didn’t happen and hence the surplus. I myself did my best to keep the overstock in the back room to a minimum even if the weekend found some items wanting. You could always order more next week.
Getting back to our automated system, note that we won’t have overstock and that means that we won’t need all that space in the back room. When a load comes in from the warehouse, we can unload it off the truck and take it directly to the aisles for stocking. This assumes that we have the manpower to do it but that is only a simple scheduling of help problem. Our system is turning out to be quite beneficial in making profits.
Another good thing about our system is all the information available. Getting back to our relish, suppose we notice that it sells two cases a week and the pickled watermelon rind next to it sells half that amount. At the same time the space for the rind holds more bottles that the dill relish. What the information can allow us to do is to change the allotment on the shelf for the two products, giving more space to the relish since it sells more each week. This will mean that we will be less likely to run out relish on the shelf since we can store more. This will also make the manager happier.
Obviously the computer can eliminate work and that means those laboring in the store won’t have to do certain things. These tasks could even be assignments that people take pleasure and pride in. At the same time the information available will not only keep stock clerks working, it will also allow them to be more productive and creative. They may have less of a physical challenge, but they will be required to perform in a cerebral way. When they get that bonus at the end of the year, they can say that they earned it.
That concludes our short trip into the basic ideas of computer programs and how they work. This is a only a very tiny piece of the world of computers as there is no mention of the architecture of a computer or how any of the keywords indicating action really work inside the computer. We just take it for granted that they do, but as we have seen, sometimes matters get fuzzy and complicated. It may be up to us to clear up some of the haze. Unfortunately, that may not be an easy matter. We need only do the best we can, remembering that computers are machines that are programmed by people. When rocket scientists get involved, who knows what could happen. Happy computing!