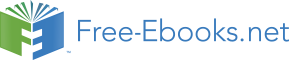

These are various routines that you may find useful :
Value Wrapping and limiting range
FUNCTION wrapF:Value,minValue,maxValue
LOCAL diff
IF Value<minValue OR Value>maxValue
diff=maxValue-minValue
IF Value<minValue
INC Value,diff
ELSE
IF Value>maxValue
DEC Value,diff
ENDIF
ENDIF
ENDIF
RETURN Value
ENDFUNCTION
FUNCTION wrapI:value%,minValue%,maxValue%
LOCAL diff%
IF value%<minValue% OR value%>maxValue%
diff%=maxValue%-minValue%
IF value%<minValue%
INC value%,diff%
ELSE
IF value%>maxValue%
DEC value%,diff%
ENDIF
ENDIF
ENDIF
RETURN value%
ENDFUNCTION
FUNCTION constrainF:value,minV,maxV
INLINE
value=(value<=minV ? minV : \
value>=maxV ? maxV : value);
ENDINLINE
RETURN value
ENDFUNCTION
Various routines for interfacing with the GLBasic window application. It also includes routines for splitting a RGB value to individual components :
INLINE
}
#ifndef WIN32
#undef main
#endif
#ifdef WIN32
// Windows stuff
typedef struct __RECT {
long left;
long top;
long right;
long bottom;
} __RECT;
#define SM_CXSCREEN 0
#define SM_CYSCREEN 1
#define HWND_BOTTOM ((HWND)1)
#define HWND_NOTOPMOST ((HWND)(-2))
#define HWND_TOP ((HWND)0)
#define HWND_TOPMOST ((HWND)(-1))
#define HWND_DESKTOP (HWND)0
#define HWND_MESSAGE ((HWND)(-3))
#define SWP_DRAWFRAME 0x0020
#define SWP_FRAMECHANGED 0x0020
#define SWP_HIDEWINDOW 0x0080
#define SWP_NOACTIVATE 0x0010
#define SWP_NOCOPYBITS 0x0100
#define SWP_NOMOVE 0x0002
#define SWP_NOSIZE 0x0001
#define SWP_NOREDRAW 0x0008
#define SWP_NOZORDER 0x0004
#define SWP_SHOWWINDOW 0x0040
#define SWP_NOOWNERZORDER 0x0200
#define SWP_NOREPOSITION SWP_NOOWNERZORDER
#define SWP_NOSENDCHANGING 0x0400
#define SWP_DEFERERASE 0x2000
#define SWP_ASYNCWINDOWPOS 0x4000
extern "C" __stdcall int GetSystemMetrics(int);
extern "C" __stdcall int GetWindowRect(HWND hWnd,struct __RECT *lpRect);
extern "C" __stdcall int SetWindowTextA(HWND hWnd,const char *lpString);
extern "C" __stdcall HWND GetDesktopWindow(void);
extern "C" __stdcall int SetWindowPos(HWND hWnd,HWND hWndInsertAfter,int X,int Y,int cx,int cy,int uFlags);
extern "C" __stdcall int EnumDisplaySettingsA(const char*, unsigned int, void*);
extern "C" __stdcall HWND GetForegroundWindow(void);
extern "C" __stdcall int GetLastError(void);
#else
#ifndef IPHONE
// This is for non-windows platforms
extern "C" __stdcall void SDL_WM_SetCaption(const char *,const char *);
#endif
#endif
extern "C" int rename(const char *_old, const char *_new);
namespace __GLBASIC__{
ENDINLINE
FUNCTION ChangeWindowText: t$
INLINE
#ifdef IPHONE
// Do nothing on the iPhone/iPod
#elif WIN32
::SetWindowTextA((HWND) GLBASIC_HWND(),t_Str.c_str());
#else
::SDL_WM_SetCaption(t_Str.c_str(), t_Str.c_str());
#endif
ENDINLINE
ENDFUNCTION
FUNCTION CentreWindow:deskWidth%,deskHeight%
DEBUG "desktop : "+deskWidth%+" "+deskHeight%+"\n"
INLINE
#ifdef WIN32
struct __RECT window;
HWND hWnd;
hWnd=(HWND) GLBASIC_HWND();
// DEBUG((int) GLBASIC_HWND());
if (::GetWindowRect(hWnd,&window))
{
::SetWindowPos(hWnd,HWND_NOTOPMOST,(deskWidth-(window.right-window.left)) / 2, (deskHeight-(window.bottom-window.top)) / 2, 0, 0, SWP_NOSIZE);
}
else
{
DEBUG("GetWindowRect failed");
}
#else
#endif
ENDINLINE
ENDFUNCTION
FUNCTION getCurrentDesktopSize:BYREF width%,BYREF height%
INLINE
#ifdef WIN32
struct __RECT desk;
if (::GetWindowRect(::GetDesktopWindow(),&desk))
{
width=desk.right-desk.left;
height=desk.bottom-desk.top;
return TRUE;
}
else
{
return FALSE;
}
#else
// Cant currently centre on Linux and Mac
width=-1;
height=-1;
return TRUE;
#endif
ENDINLINE
ENDFUNCTION
FUNCTION renameFile%:dir$,old$,new$
LOCAL ok%
LOCAL tempDir$
ok%=-1
INLINE
tempDir_Str=GETCURRENTDIR_Str();
if (SETCURRENTDIR(dir_Str.c_str())==TRUE)
{
ok=rename(old_Str.c_str(), new_Str.c_str());
}
SETCURRENTDIR(tempDir_Str);
return (ok==0 ? TRUE : FALSE);
ENDINLINE
ENDFUNCTION
FUNCTION RGBR%:colour%
INLINE
return (DGNat) colour & 255;
ENDINLINE
ENDFUNCTION
FUNCTION RGBG%:colour%
INLINE
return (DGNat) ((colour>>8) & 255);
ENDINLINE
ENDFUNCTION
FUNCTION RGBB%:colour%
INLINE
return (DGNat) ((colour>>16) & 255);
ENDINLINE
ENDFUNCTION
FUNCTION grabScreen%:
LOCAL temp%,sW%,sH%
temp%=GENSPRITE()
IF temp%<>-1
GETSCREENSIZE sW%,sH%
GRABSPRITE temp%,0,0,sW%-1,sH%-1
ENDIF
RETURN temp%
ENDFUNCTION
Swap variable values :
FUNCTION swapI:BYREF a%,BYREF b%
LOCAL c%
c%=a%
a%=b%
b%=c%
ENDFUNCTION
FUNCTION swapF:BYREF a,BYREF b
LOCAL c
c=a
a=b
b=c
ENDFUNCTION
FUNCTION swapS:BYREF a$,BYREF b$
LOCAL c$
c$=a$
a$=b$
b$=c$
ENDFUNCTION
Hexadecimal routine :
//! Convert a decimal number to a hexidecimal number
//\param value% - Number of be converted
//\param length% - Number of characters that the result will be padded to
//\return The hexidecimal value as a string
FUNCTION decToHex$:value%,length%=4
LOCAL digit%
LOCAL temp%
LOCAL result$
IF length%<=0
RETURN "0"
ENDIF
result$=""
FOR digit%=length% TO 1 STEP -1
temp%=MOD(value%,16)
IF temp%<10
result$=CHR$(temp%+48)+result$
ELSE
result$=CHR$((temp%-10)+65)+result$
ENDIF
value%=value%/16
NEXT
RETURN result$
ENDFUNCTION
//! Convert a hexidecimal number of an integer
//\param hex$ - Hexidecimal string
//\return The decimal value of the passed string
FUNCTION hexToDec%:hex$
LOCAL i%
LOCAL j%
LOCAL loop%
i%=0
j%=0
FOR loop%=0 TO LEN(hex$)-1
i%=ASC(MID$(hex$,loop%,1))-48
IF 9<i%
DEC i%,7
ENDIF
j%=j%*16
j%=bOR(j%,bAND(i,15))
NEXT
RETURN j
ENDFUNCTION
Various maths routines :
FUNCTION PI:
RETURN 3.14159265358979
ENDFUNCTION
FUNCTION PHI:
RETURN 1.6180339887
ENDFUNCTION
FUNCTION PHI2:
RETURN 0.6180339
ENDFUNCTION
FUNCTION factorial%:value%
LOCAL total%
IF value%<=1
total%=1
ELSE
total%=value%
ENDIF
WHILE value%>1
total%=total%*(value%-1)
DEC value%,1
WEND
RETURN total%
ENDFUNCTION
FUNCTION fcmp%:value1,value2,epsilon
IF ABS(value1-value2)<=ABS(value1)*epsilon
RETURN TRUE
ELSE
RETURN FALSE
ENDIF
ENDFUNCTION
Reads:
108
Pages:
176
Published:
Apr 2023
"No Filter, No Problem" by Famium is your ultimate guide to creating a visually stunning, engaging Instagram presence. Packed with insider secrets and practic...
Formats: PDF, Epub, Kindle, TXT