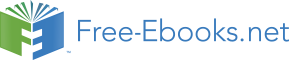

>>> 2 + 3
5
>>> 3 * 5
15
>>>
+ Plus
Explanation
Adds the two objects
- Minus
* Multiply
** Power
/ Divide Either gives a negative number or gives the subtraction of one number from the other
Gives the multiplication of the two numbers or returns the string repeated that many times.
Returns x to the power of yDivide x by y
Examples
3 + 5 gives8.'a' + 'b' gives'ab'.
-5.2 gives a negative number. 50 - 24 gives26.
2 * 3 gives6.'la' * 3 gives'lalala'.3 ** 4 gives81 (i.e.3 * 3 * 3 * 3)
4/3 gives1 (division of integers gives an integer). 4.0/3 or 4/3.0 gives 1.33333333333333 33
// Floor Division% Modulo Returns the floor of the quotient
Returns the remainder of the division<< Left Shift
>> Right Shift Shifts the bits of the number to the left by the number of bits specified. (Each number is represented in memory by bits or binary digits i.e. 0 and 1)
Shifts the bits of the number to the right by the number of bits specified.
& Bitwise AND| Bit-wise OR
^ Bit-wise XOR ~ Bit-wise invert
< Less Than
> Greater Than Bitwise AND of the numbers
Bitwise OR of the numbers
5 ^ 3 gives6
Returns whether x is less than y. All comparison operators return 1 for true and 0 for false. This is equivalent to the special variablesTrue and False respectively. Note the capitalization of these variables' names.
Returns whether x is greater than y<= Less Than or Equal To
>= Greater Than or Equal To
== Equal To
Returns whether x is less than or equal to y
4 // 3.0 gives1.0
8%3 gives 2. -
25.5%2.25 gives1.5 .
2 << 2 gives8. 2 is represented by 10 in bits. Left shifting by 2 bits gives 1000 which represents the decimal8.
11 >> 1 gives5
11 is represented in bits by
1011 which when right shifted by 1 bit gives
101 which is nothing but decimal5.
5 | 3 gives7
5 < 3 gives 0 (i.e. False) and 3 < 5 gives 1 (i.e. True). Comparisons can be chained arbitrarily: 3 < 5 < 7 givesTrue.
5 < 3 returnsTrue. If both operands are numbers, they are first converted to a common type. Otherwise, it always returnsFalse. x = 3; y = 6; x <= y returnsTrue.
x = 4; y = 3; x >= 3 returnsTrue.x = 2; y = 2; x == y returns True. x = 'str'; y = 'stR'; x == y returns False. x = 'str'; y = 'str'; x == y re
!= Not Equal Tonot Boolean NOT
and Boolean AND Compares if the objects are not equal
If x is True, it returns False. If x isFalse, it returnsTrue.
x and y returns False if x is False, else it returns evaluation of y
or Boolean OR If x is True, it returns True, else it returns evaluation of yturnsTrue.
x = 2; y = 3; x != y returnsTrue. x = True; not y returnsFalse.
x = False; y = True; x and y returns False since x is False. In this case, Python will not evaluate y since it knows that the value of the expression will has to be false (since x is False). This is called short-circuit evaluation.
x = True; y = False; x or y returns True. Short-circuit evaluation applies here as well.