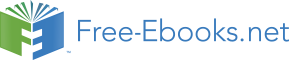

If you had an expression such as 2 + 3 * 4, is the addition done first or the multiplication? Our high school maths tells us that the multiplication should be done first - this means that the multiplication operator has higher precedence than the addition operator.
The following table gives the operator precedence table for Python, from the lowest precedence (least binding) to the highest precedence (most binding). This means that in a given expression, Python will first evaluate the operators lower in the table before the operators listed higher in the table.
The following table (same as the one in the Python reference manual) is provided for the sake of completeness. However, I advise you to use parentheses for grouping of operators and operands in order to explicitly specify the precedence and to make the program as readable as possible. For example,2 + (3 * 4) is definitely more clearer than2 + 3 * 4. As with everything else, the parentheses shold be used sensibly and should not be redundant (as in2 + (3 + 4)).
Operator
lambda
or
and
not x
in, not in
is, is not
<, <=, >, >=, !=, ==
Description
Lambda Expression Boolean OR
Boolean AND
Boolean NOT
Membership tests Identity tests
Comparisons
Operator
|
^
&
<<, >>
+,
*, /, %
+x, -x
~x
**
x.attribute
x[index]
x[index:index] f(arguments ...) (expressions, ...) [expressions, ...] {key:datum, ...} `expressions, ...`
Description
Bitwise OR
Bitwise XOR
Bitwise AND
Shifts
Addition and subtraction
Multiplication, Division and Remainder Positive, Negative
Bitwise NOT
Exponentiation
Attribute reference
Subscription
Slicing
Function call
Binding or tuple display
List display
Dictionary display
String conversion