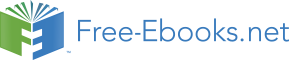

For example, int type is the set of 32-bit integers within the range -2,147,483,648 to 2,147,483,647
together with the operations described in the following table.
Table 2.3.
Operations
Symbol
Opposite
-
Addition
+
Subtraction
-
Multiplication *
Division
/
Modulus
%
Equal to
= =
Greater than
>
Less than
<
…
A data type can also be thought of as a constraint placed upon the interpretation of data in a type
system in computer programming.
Common types of data in programming languages include primitive types (such as integers,
floating point numbers or characters), tuples, records, algebraic data types, abstract data types,
reference types, classes and function types. A data type describes representation, interpretation
and structure of values manipulated by algorithms or objects stored in computer memory or other
storage device. The type system uses data type information to check correctness of computer
programs that access or manipulate the
data.
Constants
In general, a constant is a specific quantity that does not or cannot change or vary. A constant’s
value is fixed at compile-time and cannot change during program execution. C supports three
types of constants : numeric, character, string.
Numeric constants of C are usually just the written version of numbers. For example 1, 0, 56.78,
12.3e-4. We can specify our constant in octal or hexadecimal, or force them to be treated as long
integers.
Octal constants are written with a leading zero : -0.15
Hexadecimal constants are written with a leading 0x : 0x1ae
Long constants are written with a trailing L : 890L or 890l
Character constants are usually just the character enclosed in single quotes; ‘a’, ‘b’, ‘c’. Some
characters can’t be represented in this way, so we use a 2 character sequence (escape sequence).
Table 2.4.
‘\n’ newline
‘\t’ horizontal tab
‘\v’ vertical tab
‘\b’ backspace
‘\r’ carriage return
‘\\’ backslash
‘\’’ single quote
‘\”’ double quotes
‘\0’ null (used automatically to terminate character strings)
Character constants participate in numeric operations just as any other integers (they are
represented by their order in the ASCII character set), although they are most often used in
comparison with other characters.
Character constants are rarely used, since string constants are more convenient. A string constant
is a sequence of characters surrounded by double quotes e.g. “Brian and Dennis”.
A character is a different type to a single character string. This is important.
It is helpful to assign a descriptive name to a value that does not change later in the program. That is the value associated with the name is constant rather than variable, and thus such a name is
referred to as symbolic constant or simply a constant.
Variables
Variables are the names that refer to sections of memory into which data can be stored.
Let’s imagine that memory is a series of different size boxes. The box size is memory storage area
required in bytes.In order to use a box to store data, the box must be given a name, this process is known as declaration. It helps if you give a box a meaningful name that relates to the type of information and it is easier to find the data.The boxes must be of the correct size for the data type you are going to put into it. An integer number such as 2 requires a smaller box than a floating
point number as 123e12.
Data is placed into a box by assigning the data to the box. By using the name of the box you can
retrieve the box contents, some kind of data.
Variable named by an identifier. The conventions of identifiers were shown in 1.3.3.
Names should be meaningful or descriptive, for example, studentAge or student_age is more
meaningful than age, and much more meaniful than a single letter such as a.
Operators
Programming languages have a set of operators that perform arithmetical operations , and others
such as Boolean operations on truth values, and string operators manipulating strings of text.
Computers are mathematical devices , but compilers and interpreters require a full syntactic
theory of all operation in order to parse formulae involving any combination correctly.
Expressions
An expression in a programming language is a combination of values, functions, etc. interpreted
according to the particular rules of precedence and association for a particular programming
language, which computes and returns another value.
C expressions are arranged in the following groups based on the operators they contain and how
you use them:
Arithmetic expression
Conditional expression
Assignment expression
Comma expression
lvalue
Constant expression
Expressions are used as
Right hands of assignment statements
Actual parameters of functions
Conditions of if statements
Indexes of while statements
Operands of other expressions . . . .
Functions
A subprogram (also known as a procedure or subroutine) is nothing more than a collection of
instructions forming a program unit written independently of the main program yet associated
with it through a transfer/return process. Control is passed to the subprogram at the time its
services are required, and then control is returned to the main program after the subprogram has
finished.
The syntax used to represent the request of subprogram varies among the different language. The
techniques used to describe a subprogram also varies from language to language. Many systems
allow such program units to be written in languages other than that of the main program.
In most procedural programming languages, a subprogram is implemented as though it were
completely separate entity with its own data and algorithm so that an item of data in either the
main program or the subprogram is not automatically accessible from within the other. With this
arrangement, any transfer of data between the two program parts must be specified by the
programmer. This is usually done by listing the items called parameters to be transferred in the
same syntactic structure used to request the subprogram’s execution.
The names used for the parameters within the subprogram can be thought of as merely standing in
for the actual data values that are supplied when the subprogram is requested. As a result, you
often hear them called formal parameters, whereas the data values supplied from the main
program are refereed to actual parameters.
Figure 2.6.
C only accept one kind of subprogram, function. A function is a sub program in which input
values are transferred through a parameter list. However, information is returned from a function
to the main program in the form of the “value of the function”. That is the value returned by a
function is associated with the name of the function in a manner similar to the association
between a value and a variable name. The difference is that the value associated with a function
name is computed (according to the function’s definition) each time it is required, whereas when a
variable ‘s value is required, it is merely retrieve from memory.
C also provide a rich collection of built-in functions.There are more than twenty functions
declared in <math.h>. Here are some of the more frequently used.
Table 2.5.
Math
Name
Description
Example
Symbols
sqrt(x)
square root
sqrt(16.0) is 4.0
compute a value taken to an exponent,
pow(x,y)
xy
pow(2,3) is 8
xy
exp(x)
exponential function, computes ex
ey
exp(1.0) is 2.718282
log(x)
natural logarithm
ln x
log(2.718282) is 1.0
log10(x) base-10 logarithm
log x
log10(100) is 2
sin(x)
sine
sin x
sin(0.0) is 0.0
cos(x)
cosine
cos x
cos(0.0) is 1.0
tan(x)
tangent
tg x
tan(0.0) is 0.0
smallest integer not less than
ceil(x)
⌈ x ⌉
ceil(2.5) is 3; ceil(-2.5) is –2
parameter
largest integer not greater than
floor(2.5) is 2; floor(-2.5) is
floor(x)
⌊ x⌋
parameter
–3
Library
The library is not part of the C language proper, but an environment that support C will provide
the function declarations and type and macro definitions of this library.The functions, types and
macro of the library are declared in headers.
C header files have extensions .h. Header files should not contain any source code. They are used
purely to store function prototypes, common #define constants, and any other information you
wish to export from the C file that the header file belongs to.
A header can be accessed by
#include <header>
Here are some headers of Turbo C library
stdio.h Provides functions for performing input and output.
stdlib.h Defines several general operation functions and macros.
conio.h Declares several useful library functions for performing "console input and output" from a program.
math.h Defines several mathematic functions.
string.h Provides many functions useful for manipulating strings (character arrays).
io.h Defines the file handle and low-level input and output functions
graphics.h Includes graphics functions
Statements
A statement specifies one or more action to be perform during the execution of a program.
C requires a semicolon at the end of every statement.
Comments
Comments are marked by symbol “/*” and “*/”. C also use // to mark the start of a comment and
the end of a line to indicate the end of a comment.
Example 2.1.
1. The Hello program written using the first commenting style of C
/* A simple program to demonstrate
C style comments
The following line is essential
in the C version of the hello HUT program
*/
#include <stdio.h>
main()
{
printf /* just print */ ("Hello HUT\n");
}
The Hello program written using the second commenting style of C
// A simple program to demonstrate
// C style comments
//
// The following line is essential
// in the C version of the hello HUT program
#include <stdio.h>
main()
{
printf("Hello HUT\n"); //print the string and then go to a new line
}
By the first way, a program may have a multi-line comments and comments in the middle of a
line of code.However, you shouldn’t mix the two style in the same program.
C program structure
A C program basically has the following form:
Preprocessor Commands : Declare standard libraries used inside the program
Type definitions : Define new data types used inside the program
Function prototypes : Declare function types and variables passed to function.
Variables : State the names and data types of global variables
Functions: Include the main function(required) and the functions that their prototypes were
announced above.
Figure 2.7.
2.2. Data Types and Expressions*
Standard Data Types
The type of a variable determines how much space it occupies in storage and how the bit pattern
stored is interpreted. Standard data types in C are listed in the following table:
Table 2.6. Table of Variable types
Storage in
Variable Type
Keyword
Range
Bytes
Character
char
-127 to 127
1
Unsigned character
unsigned char
0 to 255
1
(Signed) integer
int
-32,768 to 32,767
2
Unsigned integer
unsigned int
0 to 65,535
2
Short integer
short
-32,768 to 32,767
2
Unsigned short
unsigned short
0 to 65,535
2
integer
Long integer
long
-2,147,483,648 to 2,147,483,647
4
integerunsigned
Unsigned long
0 to 4,294,967,295
4
long
Single precision
1.2E-38 to 3.4E38, approx. range
float
4
floating point
precision = 7 digits.
Double precision
2.2E-308 to 1.8E308, approx. range
double
8
floating point
precision = 19 digits.
Declaration and Usage of Variables and Constants
Variables
A variable is an object of a specified type whose value can be changed. In programming
languages, a variable is allocated a storage location that can contain data that can be modified
during program execution. Each variable has a name that uniquely identifies it within its level of
scope.
In C, a variable must be declared before use, although certain declarations can be made
implicitly by content. Variables can be declared at the start of any block of code, but most are
found at the start of each function. Most local variables are created when the function is called,
and are destroyed on return from that function.
A declaration begins with the type, followed by the name of one or more variables. Syntax of
declare statement is described as:
data_type list_of_variables;
A list of variables includes one or many variable names separated by commas.
Example 2.2.
Single declarations
int age; //integer variable
float amountOfMoney;//float variable
char initial;// character variable
Multiple declarations:
int age, houseNumber, quantity;
float distance, rateOfDiscount;
char firstInitial, secondInitial;
Variables can also be initialized when they are declared, this is done by adding an equals sign and
the required value after the declaration.
Example 2.3.
int high = 250; //Maximum Temperature
int low = -40; //Minimum Temperature
int results[20]; //Series of temperature readings
Constants
A constant is an object whose value cannot be changed. There are two method to define constant
in C:
By #define statement. Syntax of that statement is:
#define constant_name value
Example 2.4.
#define MAX_SALARY_LEVEL 15 //An integer constant
#define DEP_NAME “Computer Science”
// A string constant
By using const keyword
const data_type variable_name = value;
Example 2.5.
const double e = 2.71828182845905;
Functions printf, scanf
Usually i/o, input and output, form the most important part of any program. To do anything useful
your program needs to be able to accept input data and report back your results. In C, the standard
library (stdio.h) provides routines for input and output. The standard library has functions for i/o that handle input, output, and character and string manipulation. Standard input is usually means
input using the keyboard. Standard output is usually means output onto the monitor.
Input by using the scanf() function
Output by using the printf() function
To use printf and scanf functions, it is required to declare the header <stdio.h>
The printf() function
The standard library function printf is used for formatted output. It makes the user input a string
and an optional list of variables or strings to output. The variables and strings are output according to the specifications in the printf() function. Here is the general syntax of printf .
printf(“[string]”[,list of arguments]);
The list of arguments allow expressions, separated by commas.
The string is all-important because it specifies the type of each variable in the list and how you want it printed. The string is usually called the control string or the format string. The way that
this works is that printf scans the string from left to right and prints on the screen any characters it encounters - except when it reaches a % character.
The % character is a signal that what follows it is a specification for how the next variable in the list of variables should be printed. printf uses this information to convert and format the value that was passed to the function by the variable and then moves on to process the rest of the control
string and anymore variables it might specify.
For Example
printf("Hello World");
only has a control string and, as this contains no % characters it results in Hello World being
displayed and doesn't need to display any variable values. The specifier %d means convert the
next value to a signed decimal integer and so:
printf("Total = %d",total);
will print Total = and then the value passed by total as a decimal integer.
The % Format Specifiers
The % specifiers that you can use are:
Table 2.7.
Usual variable type Display
%c
char
single character
%d (%i)
int
signed integer
%e (%E) float or double
exponential format
%f
float or double
signed decimal
%g (%G) float or double
use %f or %e as required
%o
int
unsigned octal value
%s
array of char
sequence of characters
%u
int
unsigned decimal
%x (%X) int
unsigned hex value
Formatting Your Output
The type conversion specifier only does what you ask of it - it convert a given bit pattern into a
sequence of characters that a human can read. If you want to format the characters then you need
to know a little more about the printf function's control string.
Each specifier can be preceded by a modifier which determines how the value will be printed. The
most general modifier is of the form:
flag width.precision
The flag can be any of
Table 2.8.
flag
meaning
-
left justify
+
always display sign
space display space if there is no sign
0
pad with leading zeros
#
use alternate form of specifier
The width specifies the number of characters used in total to display the value and precision
indicates the number of characters used after the decimal point.
For example,
%10.3f will display the float using ten characters with three digits after the decimal point. Notice that the ten characters includes the decimal point, and a - sign if there is one. If the value needs more space than the width specifies then the additional space is used - width specifies the smallest space that will be used to display the value.
%-10d will display an int left justified in a ten character space.
The specifier %+5d will display an int using the next five character locations and will add a + or -
sign to the value.
Strings will be discussed later but for now remember: if you print a string using the %s specifier
then all of the characters stored in the array up to the first null will be printed. If you use a width specifier then the string will be right justified within the space. If you include a precision specifier then only that number of characters will be printed.
For Example
printf("%s,Hello")
will print Hello,
printf("%25s ,Hello")
will print 25 characters with Hello right justified
Also notice that it is fine to pass a constant value to printf as in printf("%s,Hello").
Finally there are the control codes:
Table 2.9.
\b backspace
\f formfeed
\n new line
\r carriage return
\t horizontal tab
\' single quote
\0 null
If you include any of these in the control string then the corresponding ASCII control code is sent
to the screen, or output device, which should produce the effect listed. In most cases you only
need to remember \n for new line.
The scanf() function
The scanf function works in much the same way as the printf. That is it has the general form:
scanf(“control string”,variable,variable,...)
In this case the control string specifies how strings of characters, usually typed on the keyboard,
should be converted into values and stored in the listed variables. However there are a number of
important differences as well as similarities between scanf and printf.
The most obvious is that scanf has to change the values stored in the parts of computers memory
that is associated with parameters (variables).
To understand this fully you will have to wait until we have covered functions in more detail. But,
just for now, bare with us when we say to do this the scanf function has to have the addresses of
the variables rather than just their values. This means that simple variables have to be passed with a preceding &.
The second difference is that the control string has some extra items to cope with the problems of
reading data in. However, all of the conversion specifiers listed in connection with printf can be
used with scanf.
The rule is that scanf processes the control string from left to right and each time it reaches a
specifier it tries to interpret what has been typed as a value. If you input multiple values then these are assumed to be separated by white space - i.e. spaces, newline or tabs. This means you can type:
3 4 5
or
3
4
5
and it doesn't matter how many spaces are included between items. For Example
scanf("%d %d",&i,&j);
will read in two integer values into i and j. The integer values can be typed on the same line or on different lines as long as there is at least one white space character between them.
The only exception to this rule is the %c specifier which always reads in the next character typed
no matter what it is. You can also use a width modifier in scanf. In this case its effect is to limit the number of characters accepted to the width.
For Example
scanf("%l0d",&i)