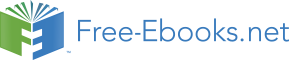

5.2 Exploiting Heartbleed Vulnerability with Metasploit
5.4 C# Code Segments for Predicting Severity/Threat Of Exploitation using Gaussian Naive Bayes Approach
5.4.1 Populating DataTable & Classifying Data Using Classify Function
5.4.2 Calculating Probabilities
namespace ProbabilityFunctions
{
public class Classifier
{
private DataSet dataSet = new DataSet();
public DataSet DataSet
{
get { return dataSet; }
set { dataSet = value; }
}
public void TrainClassifier(DataTable table)
{
dataSet.Tables.Add(table);
//table
DataTable GaussianDistribution = dataSet.Tables.Add("Gaussian");
GaussianDistribution.Columns.Add(table.Columns[0].ColumnName);
//columns
for (int i = 1; i < table.Columns.Count; i++)
{
GaussianDistribution.Columns.Add(table.Columns[i].ColumnName +
"Mean");
GaussianDistribution.Columns.Add(table.Columns[i].ColumnName +
"Variance");
}
//calculate data
var results = (from myRow in table.AsEnumerable()
group myRow by
myRow.Field<string>(table.Columns[0].ColumnName) into g
select new { Name = g.Key, Count = g.Count()
}).ToList();
for (int j = 0; j < results.Count; j++)
{
DataRow row = GaussianDistribution.Rows.Add();
row[0] = results[j].Name;
int a = 1;
for (int i = 1; i < table.Columns.Count; i++)
{
row[a] = Helper.Mean(SelectRows(table, i,
string.Format("{0} = '{1}'", table.Columns[0].ColumnName, results[j].Name)));
row[++a] = Helper.Variance(SelectRows(table, i,
string.Format("{0} = '{1}'", table.Columns[0].ColumnName, results[j].Name)));
a++;
}
}
}
public string Classify(double[] obj)
{
Dictionary<string, double> score = new Dictionary<string,
double>();
var results = (from myRow in dataSet.Tables[0].AsEnumerable()
group myRow by
myRow.Field<string>(dataSet.Tables[0].Columns[0].ColumnName) into g
select new { Name = g.Key, Count = g.Count()
}).ToList();
for (int i = 0; i < results.Count; i++)
{
List<double> subScoreList = new List<double>();
int a = 1, b = 1;
for (int k = 1; k < dataSet.Tables["Gaussian"].Columns.Count; k
= k + 2)
{
double mean = Convert.ToDouble
(dataSet.Tables["Gaussian"].Rows[i][a].ToString ());
double variance = Convert.ToDouble
(dataSet.Tables["Gaussian"].Rows[i][++a].ToString ());
double result = Convert.ToDouble (Helper.NormalDist(obj[b -
1], mean, Helper.SquareRoot(variance)));
subScoreList.Add(result);
a++; b++;
}
double finalScore = 0.0;
for (int z = 0; z < subScoreList.Count; z++)
{
if (finalScore == 0.0)
{
finalScore = subScoreList[z];
continue;
}
finalScore = finalScore * subScoreList[z];
}
score.Add(results[i].Name, finalScore * 0.5);
}
double maxOne = score.Max(c => c.Value);
var name = (from c in score
where c.Value == maxOne
select c.Key).First();
return name;
}
#region Helper Function
public IEnumerable<double> SelectRows(DataTable table, int column,
string filter)
{
List<double> _doubleList = new List<double>();
DataRow[] rows = table.Select(filter);
for (int i = 0; i < rows.Length; i++)
{
_doubleList.Add(Convert.ToDouble (rows[i][column]));
}
return _doubleList;
}
public void Clear()
{
dataSet = new DataSet();
}
#endregion
}
}
5.4.3 Helper Class
public static class Helper
{
public static double Variance(this IEnumerable<double> source)
{
double avg = source.Average();
double d = source.Aggregate(0.0, (total, next) => total +=
Math.Pow(next - avg, 2));
if (d == 0) d = 1;
return d / (source.Count() - 1);
}
public static double Mean(this IEnumerable<double> source)
{
if (source.Count() < 1)
return 0.0;
double length = source.Count();
double sum = source.Sum();
return sum / length;
}
public static double NormalDist(double x, double mean, double
standard_dev)
{
double fact = standard_dev * Math.Sqrt(2.0 * Math.PI);
double expo = (x - mean) * (x - mean) / (2.0 * standard_dev *
standard_dev);
return Math.Exp(-expo) / fact;
}
public static double NORMDIST(double x, double mean, double
standard_dev, bool cumulative)
{
const double parts = 50000.0; //large enough to make the trapzoids
small enough
double lowBound = 0.0;
if (cumulative) //do integration: trapezoidal rule used here
{
double width = (x - lowBound) / (parts - 1.0);
double integral = 0.0;
for (int i = 1; i < parts - 1; i++)
{
integral += 0.5 * width * (NormalDist(lowBound + width * i,
mean, standard_dev) +
(NormalDist(lowBound + width * (i + 1), mean,
standard_dev)));
}
return integral;
}
else //return function value
{
return NormalDist(x, mean, standard_dev);
}
}
public static double SquareRoot(double source)
{
double dblResult = Math.Sqrt(source);
return (dblResult);
}
}
}