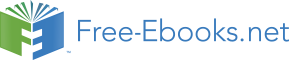

The interesting portion of this code is the parameter list for the two subroutines, change_it() and assign_it(), found at listing lines 3 and 7. You will notice that the actual code inside each subroutine is identical, but, as you can see from the output, drastically different things happen when the subroutines are called. (Any function which does not return a value is called a subroutine when programming in C.) We pass a pointer to a pointer as the first parameter in change_it(). When we subsequently change the value the pointer points to (remember * means “value at”) what we are really changing is the pointer in our main module. C# has this same issue if you force a reference. It just has trouble with strings.
We need to talk about two things here. First, when you want to pass a parameter by ref you have to actually use the ref (or out) keyword as shown on listing lines 11 and 24. C# is not like C++ when it comes to references, it will not automatically create one for you. The second thing to notice is that we really only got a copy of the string. Every shred of documentation I can find says string is a reference data type, but this certainly isn't reference behavior as is shown by the highlighted output lines.
What happens when you use a real reference class/object?
Our first class simply has a string member element (or property if you insist on using the new lingo.) Because we created this class without deriving it from any other class, any declarations of it will be an honest to goodness reference without any qualifiers spelled out in fine print like the string class has.
Notice that our Program class now contains the methods for changing and assigning. Instead of strings, most of the parameters are now an instance or reference of our TestClass. We still only force a reference with the assign_it() method, but look at the difference in output! The first call assigning tc3 to tc1 really did change the reference. We know this because the later change_it() call involving tc1 actually changed the value in tc3! The final assignment of tc1 to tc2 proves beyond a doubt we really changed tc3.
What about that object we created originally for tc1? It's just floating around out there waiting for our application to run long enough that garbage collection finds it, or that our entire region is wiped when our program ends. C#/Mono doesn't give us a method of deleting it under program control. It does provide the IDisposable Interface which would let our class implement Dispose(), however, that method is supposed to be used for releasing nonmanaged resources. It does not actually free the managed memory used by the object instance. (* see next section)
In short, the String class lugied up parameter passing because Microsoft tried to make C#/Mono easier to learn for newbies. While String is a reference class, the equality and inequality operators were overridden to compare object values instead of object references. If you come from a C++ or Java background, you have gotten used to String being a class which operates like all other classes. Since Microsoft wants to dumb down the IQ level required for programming, they made the class String behave somewhat like a native data type.
This is a throwback to the days of BASIC PLUS on the PDP 11/70 under RSTS/E. This early version of BASIC allowed a user to create a string variable by simply coding: