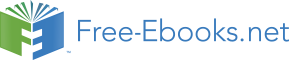

Anatomy of a Game Engine
By: Richard Baldwin
Online: < http://cnx.org/content/col11489/1.13>
This selection and arrangement of content as a collection is copyrighted by Richard Baldwin.
It is licensed under the Creative Commons Attribution License: http://creativecommons.org/licenses/by/3.0/
Collection structure revised: 2013/02/07
For copyright and attribution information for the modules contained in this collection, see the " Attributions" section at the end of the collection.
Anatomy of a Game Engine
Table of Contents
Chapter 1. Slick0100: Getting started with the Slick2D game library
1.4. Download the required software
1.5. Install the required software
1.6. Create, compile, and execute your first Slick2D program
Chapter 2. Slick0110: Overview
2.3. The bottom line at the top
2.7. Discussion and sample code
Behavior of an object of the AppGameContainer class
Behavior of an object that implements the Game interface
Beginning of the class named Slick0110a
Chapter 3. Slick0120: Starting your program
3.4. General background information
3.5. Discussion and sample code
Behavior of an object of the AppGameContainer class
Behavior of an object that implements the Game interface
The constructors for the AppGameContainer class
The setup method of the AppGameContainer class
The getDelta method of the GameContainer class
The gameLoop method of the AppGameContainer class
3.10. Complete program listing
Chapter 4. Slick0130: The game loop
4.4. General background information
Overall structure of a game program
4.5. Discussion and sample code
Beginning of the class named Slick0130a
4.10. Complete program listing
Chapter 5. Slick0140: A first look at Slick2D bitmap graphics
5.4. General background information
5.5. Discussion and sample code
Beginning of the class named Slick0140a
5.10. Complete program listing
Chapter 6. Slick0150: A first look at sprite motion, collision detection, and timing control
6.4. General background information
6.5. Discussion and sample code
A program with a relatively constant frame rate - Slick0150a
The screen output for Slick0150a
Beginning of the class named Slick0150a
The constructor and the main method
A program with a highly variable frame rate - Slick0150b
The screen output for Slick0150b
6.10. Complete program listings
Chapter 7. Slick0160: Using the draw and drawFlash methods.
7.4. General background information
7.5. Discussion and sample code
Beginning of the Slick0160a class
Beginning of the class named Slick0160b
7.10. Complete program listing
Chapter 8. Slick0170: Mouse and keyboard input
8.4. General background information
8.5. Discussion and sample code
8.10. Complete program listing
Chapter 9. Slick0180: Sprite sheet animation, part 1
9.4. General background information
9.5. Discussion and sample code
9.10. Complete program listing
Chapter 10. Slick0190: Sprite sheet animation, part 2
10.4. General background information
10.5. Discussion and sample code
10.10. Complete program listing
Chapter 11. Slick0200: Developing a sprite class
11.4. General background information
11.5. Discussion and sample code
11.10. Complete program listings
Chapter 12. Slick0210: Collision detection and sound
12.4. General background information
12.5. Discussion and sample code
The isCollision method of the Sprite01 class
12.10. Complete program listings
Chapter 13. Slick0220: Simulating a pandemic
13.4. General background information
13.5. Discussion and sample code
13.10. Complete program listing
Chapter 1. Slick0100: Getting started with the Slick2D
game library*
It is licensed under the Creative Commons Attribution License:
http://creativecommons.org/licenses/by/3.0/
2013/02/07 08:38:05 -0600
Summary
Learn how to install Slick2D in such a way that you can easily compile and execute Slick2D
programs from the command line with no need for a high level IDE.
1.1. Table of Contents
Download the required software
Create, compile, and execute your first Slick2D program
1.2. Preface
Turning the crank
As a professor of Computer Information Technology at Austin Community College, I teach
courses in game programming using both C++ and C#/XNA. I have long had a concern that
students enter my courses expecting to simply "turn the crank" on a game engine such as Dark
GDK or XNA and have great games emerge from the other end of the process. Unfortunately, it
isn't quite that easy.
Anatomy of a game engine
Given time limitations and other restrictions, it is not practical to teach those students much about
the inner working of such game engines. Therefore, I have decided to publish a series of modules
on the anatomy of a game engine that my students, (and other interested parties) can read to learn
about those inner workings.
First in a collection
Therefore, this module is the first in a collection of modules designed to teach you about the
anatomy of a typical game engine (sometimes called a game framework) .
The Slick2D library
I have chosen to concentrate on a free game library named Slick2D , (which is written in Java) for several reasons including the following:
Java is the language with which I am the most comfortable. Hence, I can probably do a better
job of explaining the anatomy of a game engine that uses Slick2D than would be the case for a
game engine written in C++, C#, Python, or some other programming language.
Java has proven in recent years to be a commercially successful game programming language.
For example, I cite the commercial game named Minecraft , written in Java, for which apparently millions of copies have been sold. Also, knowing Java is very beneficial for those
who might want to develop apps for Android.
Slick2D is free and the source code for Slick2D is readily available.
The overall structure of a basic Slick2D game engine is very similar to Dark GDK and XNA,
and is probably similar to other game engines as well.
Java is platform independent.
Applicable to other environments as well
Although the modules in this collection will concentrate on the Java game library named Slick2D,
the concepts involved and the knowledge that you will gain is applicable to other game engines
written in different programming languages.
Purpose
The purpose of this module is to get you started, including showing you how to download and
install Slick2D, and how to compile and execute your first Slick2D program. Future modules will
start digging into and explaining the inner workings of a basic Slick2D game engine.
What you should know
This series of modules is not intended for beginning programmers. As a minimum, you should
already know about fundamental programming concepts such as if statements, for loops, while
loops, method or function calls, parameter passing, etc. Ideally, you will have some object-
oriented programming knowledge in a modern programming language such as Java, C#, C++, or
possibly Python or JavaScript.
You should also be relatively comfortable with the command-line interface, directory or folder
trees, batch or script files, etc.
Finally, you should also be comfortable downloading and installing software on the machine and
operating system of your choice.
What you will learn
In this module, you will learn how to download and install Slick2D on a Windows XP, Vista, or
Windows 7 machine and how to compile and execute a very simple Slick2D program. (If you are
using a different operating system, you will need to translate this information to your system of
choice. However, since Java is platform in